Get videos from vk.com using PHP
Get video from VK using PHP
I had a problem - how to get video from all the famous social networks vkontakte.ru. and install it on the site. The key point, I did not want to use IFRAME or some other VK services, I wanted the video to look like it was on my site. Having climbed a couple of dozens of sites, as much as I found, these are sites for downloading videos, such as VIDEOSAVER and so on. This all did not fit, because I needed code which I could integrate into my PHP engine.
There were a couple of self-written PHP scripts - which cost from 30 to 500 cu But half of them were encoded by Zend Encoder or simply used their servers to search for video files and did not have much value for me.
Thus began the inspiration. I decided to use the mobile version because it is much easier. The first thing I did was draw up a video algorithm from VC.
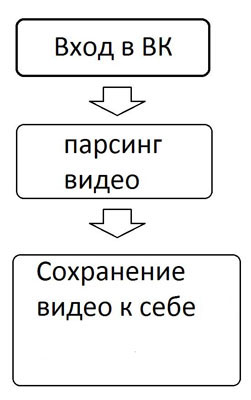
The first piece of code was the entrance to the VC. I rummaged through the Internet and found something similar:
(Code taking into account my corrections)
function curl_gets_exec is a function for more convenient work with CURL, redirect handling, etc.
Other parameters can be found - php.net/manual/ru/book.curl.php
Now it remained to find the video and parse it.
As you know, VK video files have the form - vk.com/video2409212_161721801
It is easy to guess that it consists of 2 pieces of “2409212” and “161721801”.
So I used 2 variables $ vid and $ oid, which started to accept GET requests
And formed such a link
Then we send the VK data and get the HTML text from the video page, all the HTML go into the variable $ answer
In order not to mess a lot, I used the simple_html_dom library for parsing, you can download it on the Internet, or I posted it here - v.plirex.com/simplehtmldom.rar
Create the Simple shell
We load HTML text in it
We find the first element of the DOM system "video", if you look at the page vk.com/video2409212_161721801 in HTML code, we see that the links to the video are stored in this element
Check if the video we need is there
inside video something like this HTML code
As you can see there are elements of 'source' which contain links to the video.
I want to note that these links are active only for this user, and only after viewing the main video document, those links are vk.com/video2409212_161721801 .
The links to the video look like - cs527213.userapi.com/u11174769/videos/dc3277461b.240.mp4
Where u11174769 is a unique cache that is new every time.
We take the first available link and download it
I described a short guide for downloading videos from VK. This code is not perfect and requires a lot of improvements. The first thing is to get rid of the storage of cookies in the FILE, and in the future make it possible to choose what quality to download video.
But I did not find a similar example on the Internet. So go for it.
Here is a link to the full source with the v.plirex.com/v.plirex.com.rar library .
I also made a demo on the v.plirex.com domain
I had a problem - how to get video from all the famous social networks vkontakte.ru. and install it on the site. The key point, I did not want to use IFRAME or some other VK services, I wanted the video to look like it was on my site. Having climbed a couple of dozens of sites, as much as I found, these are sites for downloading videos, such as VIDEOSAVER and so on. This all did not fit, because I needed code which I could integrate into my PHP engine.
There were a couple of self-written PHP scripts - which cost from 30 to 500 cu But half of them were encoded by Zend Encoder or simply used their servers to search for video files and did not have much value for me.
Thus began the inspiration. I decided to use the mobile version because it is much easier. The first thing I did was draw up a video algorithm from VC.
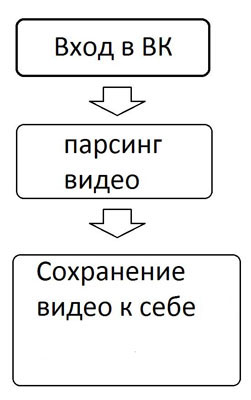
Entrance to VK
The first piece of code was the entrance to the VC. I rummaged through the Internet and found something similar:
(Code taking into account my corrections)
ini_set('error_reporting', E_ALL);
ini_set ('display_errors', 1);
$user_agent = 'Mozilla/5.0 (Windows; U; Windows NT 6.0; ru; rv:1.9.2.13) ' . 'Gecko/20101203 Firefox/3.6.13 ( .NET CLR 3.5.30729)';
$login = ‘логин’;
$password = ‘пароль’;
functioncurl_gets_exec($ch, $redirects = 0, $curlopt_returntransfer = true, $curlopt_maxredirs = 10, $curlopt_header = false){
curl_setopt($ch, CURLOPT_HEADER, true);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$data = curl_exec($ch);
$header=substr($data,0,curl_getinfo($ch,CURLINFO_HEADER_SIZE));
$http_code = curl_getinfo($ch, CURLINFO_HTTP_CODE);
$exceeded_max_redirects = $curlopt_maxredirs > $redirects;
$exist_more_redirects = false;
if ($http_code == 301 || $http_code == 302) {
if ($exceeded_max_redirects) {
list($header) = explode("\r\n\r\n", $data, 2);
$matches = array();
preg_match('/(Location:|URI:)(.*?)\n/', $header, $matches);
$url = trim(array_pop($matches));
$url_parsed = parse_url($url);
if (isset($url_parsed)) {
curl_setopt($ch, CURLOPT_URL, $url);
$redirects++;
return curl_gets_exec($ch, $redirects, $curlopt_returntransfer, $curlopt_maxredirs, $curlopt_header);
}
}
else {
$exist_more_redirects = true;
}
}
if ($data !== false) {
if (!$curlopt_header)
list(,$data) = explode("\r\n\r\n", $data, 2);
if ($exist_more_redirects) returnfalse;
if ($curlopt_returntransfer) {
return $data;
}
else {
echo $data;
if (curl_errno($ch) === 0) returntrue;
elsereturnfalse;
}
}
else {
returnfalse;
}
}
$ch = curl_init();
curl_setopt($ch, CURLOPT_USERAGENT, $user_agent);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_TIMEOUT, 10);
curl_setopt($ch,CURLOPT_REFERER,'http://m.vk.com/login?fast=1&hash=&s=0&to=');
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($ch, CURLOPT_COOKIEFILE,'cookie.txt');
curl_setopt($ch, CURLOPT_COOKIEJAR, 'cookie.txt');
curl_setopt($ch, CURLOPT_POST, false);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_URL, 'https://login.vk.com/?act=login&from_host=m.vk.com&from_protocol=http&ip_h=&pda=1');
$answer = curl_gets_exec($ch);
$post = array(
'email' => $login,
'pass' => $password
);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($post));
$answer = curl_gets_exec($ch);
</code>
Данный кусок кода делает довольно несложные действия – создаётся запрос , такой как будто бы его делал ваш браузер эмулируя вход пользователя в систему ВК,
Более детально :
<source lang="php">
curl_setopt($ch,CURLOPT_REFERER,'http://m.vk.com/login?fast=1&hash=&s=0&to='); // от куда отправляться данные
curl_setopt($ch, CURLOPT_COOKIEFILE,'cookie.txt'); // куда упадут кукисы (не забудьте создать этот файл на сервере и назначить ему права 777 , - 'cookie.txt' )
curl_setopt($ch, CURLOPT_URL, 'https://login.vk.com/?act=login&from_host=m.vk.com&from_protocol=http&ip_h=&pda=1'); // куда
отправить данные
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($post)); //Данные для пост запроса (в нашем случае – это ваши пароль и логин)
function curl_gets_exec is a function for more convenient work with CURL, redirect handling, etc.
Other parameters can be found - php.net/manual/ru/book.curl.php
Parsig Video
Now it remained to find the video and parse it.
As you know, VK video files have the form - vk.com/video2409212_161721801
It is easy to guess that it consists of 2 pieces of “2409212” and “161721801”.
So I used 2 variables $ vid and $ oid, which started to accept GET requests
$vid=$_GET['vid'];
$oid=$_GET['oid'];
And formed such a link
$link="http://m.vk.com/video{$vid}_{$oid}";
Then we send the VK data and get the HTML text from the video page, all the HTML go into the variable $ answer
curl_setopt($ch, CURLOPT_URL, $link);
$answer = curl_gets_exec($ch);
In order not to mess a lot, I used the simple_html_dom library for parsing, you can download it on the Internet, or I posted it here - v.plirex.com/simplehtmldom.rar
Create the Simple shell
include"simplehtmldom/simple_html_dom.php";
$html = new simple_html_dom();
We load HTML text in it
$html->load($answer);
We find the first element of the DOM system "video", if you look at the page vk.com/video2409212_161721801 in HTML code, we see that the links to the video are stored in this element
$div_video=$html->find('video',0);
Check if the video we need is there
if($div_video->outertext=="")die(“Video not found”);
inside video something like this HTML code
<videopreload="none"controls="controls"poster="http://cs527213.userapi.com/u11174769/video/l_18a03288.jpg"><sourcesrc="http://cs527213.userapi.com/u11174769/videos/dc3277461b.720.mp4"type="video/mp4"></source><sourcesrc="http://cs527213.userapi.com/u11174769/videos/dc3277461b.480.mp4"type="video/mp4"></source><sourcesrc="http://cs527213.userapi.com/u11174769/videos/dc3277461b.360.mp4"type="video/mp4"></source><sourcesrc="http://cs527213.userapi.com/u11174769/videos/dc3277461b.240.mp4"type="video/mp4"></source><divclass="img"><imgsrc="http://cs527213.userapi.com/u11174769/video/l_18a03288.jpg"alt=""></div><divclass="no_pl"> Название </div></video>
As you can see there are elements of 'source' which contain links to the video.
I want to note that these links are active only for this user, and only after viewing the main video document, those links are vk.com/video2409212_161721801 .
The links to the video look like - cs527213.userapi.com/u11174769/videos/dc3277461b.240.mp4
Where u11174769 is a unique cache that is new every time.
$sVideo=$div_video->find('source');
//Преобразуем полученные данные в массив ссылок на видео
$aBuf="";
$j=0;
foreach($sVideo as $post) {
/*$aBuf[$j]=$post->outertext;
$aBuf[$j]=substr($aBuf[$j],stripos($aBuf[$j],'src="')+5,strlen($aBuf[$j]));
$aBuf[$j]=substr($aBuf[$j],0,stripos($aBuf[$j],'" type'));
*/
$aBuf[$j]=$post->src;
$j++;
//echo "{$aBuf[$j]} \n |";
}
Preservation
We take the first available link and download it
$link= $aBuf[0];
header('Content-type: content=video/mp4');
header("Content-Disposition: attachment; filename=vPlirex.mpg");
header("Pragma: no-cache");
header("Expires: 0");
$tFile = fopen($link,'r');
while (!feof($tFile))
{
echo( fgets($tFile));
}
fclose($tFile);
Conclusion
I described a short guide for downloading videos from VK. This code is not perfect and requires a lot of improvements. The first thing is to get rid of the storage of cookies in the FILE, and in the future make it possible to choose what quality to download video.
But I did not find a similar example on the Internet. So go for it.
Here is a link to the full source with the v.plirex.com/v.plirex.com.rar library .
I also made a demo on the v.plirex.com domain