
Briefly about RTTI and attributes in Delphi 2010
- Transfer
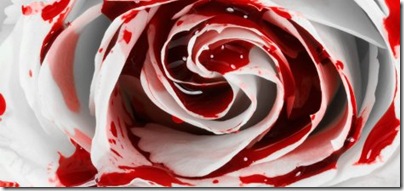
RTTI is the central element on which the Delphi IDE is written , it has existed since the first release, but I heard from some people over the years that they tried to use RTTI and found it too complicated and intricate, especially compared to the Reflection API in Java and. NET . This is a real shame, since the ability to write code to request detailed information about other objects without knowing their type in advance is a really powerful feature.
However, I think complaints will be a thing of the past with the new API.. It was not only expanded, but became much more accessible and easier to use.
One of the new features that I am very impressed with is the support of attributes in the Win32 environment . I am working on a great example, but here we will quickly look at creating and then requesting custom attributes.
Custom attributes are simply classes that inherit from TCustomAttribute . They can have properties, methods, etc., like any other class:
MyAttribute = class(TCustomAttribute)
private
FName: string;
FAge: Integer;
public
constructor Create(const Name : string; Age : Integer);
property Name : string read FName write FName;
property Age : Integer read FAge write FAge;
end;
There are no surprises here, the constructor is described exactly as you expected.
Next, we can apply our attribute to the class:
TMyClass = class
public
[MyAttribute('Malcolm', 39)]
procedure MyProc(const s : string);
[MyAttribute('Julie', 37)]
procedure MyOtherProc;
end;
Here I apply the attribute to both methods, but set different parameters to the attribute. The order of writing parameters corresponds to their order in the constructor. The use of attributes is not limited to one method, you can apply attributes in properties, classes, to any other entities.
Of course, it makes no sense to add attributes if you cannot read them from somewhere, so here are some examples of code that uses the new RTTI API to query our attributes, as well as display details in a ListBox :
procedure TForm3.Button1Click(Sender: TObject);
var
ctx : TRttiContext;
t : TRttiType;
m : TRttiMethod;
a : TCustomAttribute;
begin
ListBox1.Clear;
ctx := TRttiContext.Create;
try
t := ctx.GetType(TMyClass);
for m in t.GetMethods do
for a in m.GetAttributes do
if a is MyAttribute then
ListBox1.Items.Add(Format('Method = %s; Attribute = %s, Name = %s, Age = %d',
[m.Name, a.ClassName, MyAttribute(a).Name,
MyAttribute(a).Age]));
finally
ctx.Free;
end;
end;
First, I got a link to the RTTI context, and loaded it using the ClassType of my class. Then I executed for..in on the methods of my class, and for each method I executed for..in on the attributes. After that, I checked if the attribute is my custom attribute, got the Name and Age values for them , and displayed them in the ListBox .
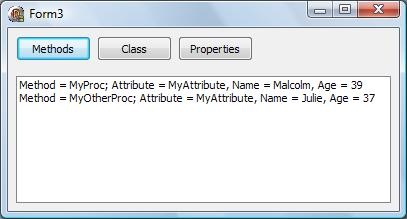
As I mentioned earlier, I am working on another example that is more useful, but hopefully this example will give you a taste of some of the new Delphi 2010 products .
You can help improve the translation.
translated.by/you/rtti-and-attributes-in-delphi-2010/into-ru
Translators: r3code