7 recommendations on the design of the JavaScript code
- Transfer
The author of the material, the translation of which we publish today, says that she is really obsessed with writing clean code. She believes that the code should be written so that, firstly, it would be convenient for other programmers to work with him, in the future, including his author, and secondly, given the possibility of extending this code. That is, you need to strive to ensure that the application is relatively easy to add new features, and that its code base would be convenient to accompany. If programs were written, taking into account only the needs of computers, then programmers could probably express their thoughts only with the help of zeros and ones, without worrying about anything else. This article provides a number of recommendations for writing high-quality code, illustrated with examples in JavaScript.
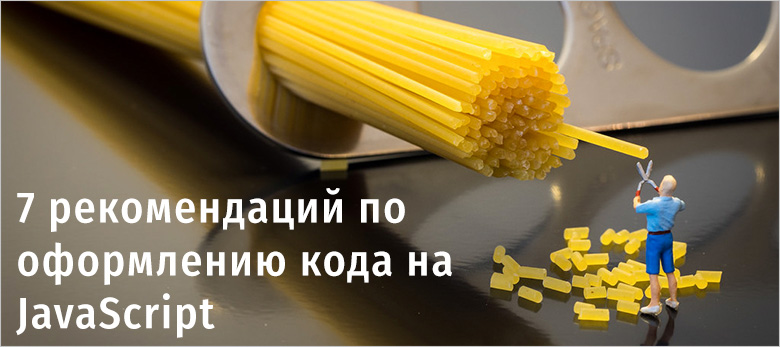
The code is much easier to read when using its written, descriptive names of functions and variables. Here is a not very clear code:
Its readability will be greatly improved if we use in it clear names of variables and functions reflecting their meaning.
Do not strive for minimalism when writing text programs. Use the full variable names that the one who will work with your code in the future can easily understand.
Functions are easier to maintain, they become much more understandable, readable, if they are aimed at solving only a single task. If we encounter an error, then using small functions, finding the source of this error becomes much easier. In addition, code reuse capabilities are improved. For example, the above function could be given a name
It can be divided into two functions, then the role of each code fragment will become clearer. In addition, if we create a large program, the presence of a function
A sign that the function can be divided into two is the possibility of using the word “and” in its name.
Write good documentation for your code - then the one who encounters it in the future will understand what is being done and why in this code. Here is an example of a failed function. Here are used some "magic numbers", their meaning is not explained anywhere.
Here you can add comments to make this code more understandable for someone who does not know the formula for calculating the area of a circle.
This code is just an example. Probably, in a similar situation, instead of introducing its own constant storing the pi number, it would be better to use the standard property
Comments on the code should answer the question why.
Please note that for documenting code, it makes sense to use special tools and the corresponding code commenting rules. I like Google Style Docstrings when applied to Python , and JSDoc as applied to JavaScript .
Sandy Metz programs Ruby well, gives interesting talks and writes books. She formulated four rules for writing clean code in object-oriented languages. Here they are.
I recommend to see her presentation on these rules.
I have been following these rules for about two years now, and they are so thoroughly entrenched in my mind that I follow them, literally, “on the machine”. I like them, and I believe that thanks to their use, the convenience of maintaining the code is enhanced.
Please note that these rules are only recommendations, but using them will make your code much better.
Consistent application of the rules of writing code is very important, regardless of whether you are writing the code of a certain project yourself or working in a team. In particular, this is expressed in the fact that those who read the code that is the result of team development should perceive it as a whole. With this approach, the authorship of individual lines of code can be established only by contacting Git. If you use a semicolon in JavaScript, put it wherever it is needed. The same goes for quotes - choose double or single quotes and, if there are no good reasons, always use what you chose.
I recommend using the code style guide and the linter, which allows you to convert the code to the selected standard. For example, I, for JavaScript, like the rules of Standard JSfor Python, the PEP8 rules .
In fact, the main thing here is to find the code design rules and follow them.
One of the first ideas that they are trying to convey to someone who wants to become a programmer is: “Do not repeat” (Don't Repeat Yourself, DRY). If you notice repetitive fragments in your projects, use such software constructs that will reduce repetitions of the same code. I often advise my students to play the SET game in order to improve their pattern recognition skills.
However, if you are fanatically applied the principle of DRY or decide to abstract the unsuccessfully chosen templates, the readability of the code can seriously deteriorate, and, later, you may need to resort to creating copies of the same constructions more often. Sandy Metz, by the way, has an excellent article dedicated to the fact that code duplication is a lesser evil thanunsuccessful abstraction .
As a result, you should not repeat, but you should not, in the struggle to comply with the DRY principle, modify the code to such an extent that it can become difficult to understand.
Group related variables and functions to make your code clearer and better in terms of its reuse. Here is an example of not very well-organized code, in which information about a person is presented in the form of separate variables.
If in such a program you need to process the data of many people, then it would be better to use something like the following construct.
And if the program only needs to work with data about one person, then they can be arranged as shown below.
In a similar way, one should approach the splitting of long programs into modules, into separate files. This will facilitate the use of code, separated into separate files, in different projects. In large files with program code it is often difficult to navigate, and small clear modules are easy to use in the project for which they were created, and, if necessary, in other projects. Therefore, strive to write a clear modular code, combining logically related elements.
In this article we shared with you some recommendations for writing clean and clear code. However, these are only recommendations. These are tips to help write quality program texts. If we systematically apply rules similar to those described here, then in comparison with the code, when writing something similar does not apply, the code written using the rules will be more understandable, it will be easier to expand, maintain and reuse.
Dear readers! What code rules do you follow?

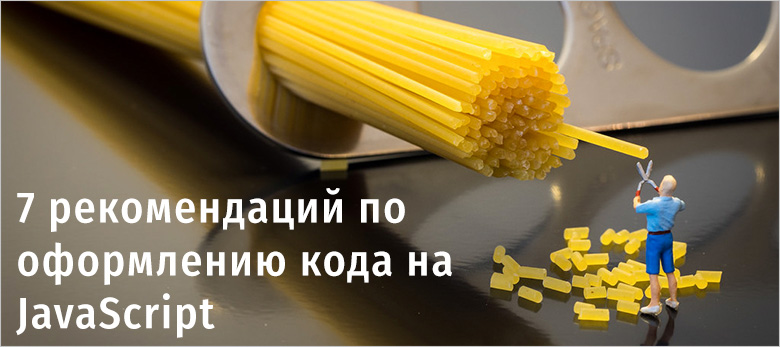
1. Use clear variable and function names.
The code is much easier to read when using its written, descriptive names of functions and variables. Here is a not very clear code:
functionavg (a) {
let s = a.reduce((x, y) => x + y)
return s / a.length
}
Its readability will be greatly improved if we use in it clear names of variables and functions reflecting their meaning.
functionaverageArray (array) {
let sum = array.reduce((number, currentSum) => number + currentSum)
return sum / array.length
}
Do not strive for minimalism when writing text programs. Use the full variable names that the one who will work with your code in the future can easily understand.
2. Write short functions that solve one problem.
Functions are easier to maintain, they become much more understandable, readable, if they are aimed at solving only a single task. If we encounter an error, then using small functions, finding the source of this error becomes much easier. In addition, code reuse capabilities are improved. For example, the above function could be given a name
sumAndAverageArray
, since in it we calculate the sum of the values of the array elements using the method reduce
, and then we find the average value, dividing the resulting sum by the number of array elements. This is the function.functionsumAndAverageArray(array) {
let sum = array.reduce((number, currentSum) => number + currentSum)
return sum / array.length
}
It can be divided into two functions, then the role of each code fragment will become clearer. In addition, if we create a large program, the presence of a function
sumArray
can be very useful. Here is the code for two new features. One calculates the sum of the elements of the array, the second returns their average value.functionsumArray(array){
returnarray.reduce((number, currentSum) => number + currentSum)
}
functionaverageArray(array){
return sumArray(array) / array.length
}
A sign that the function can be divided into two is the possibility of using the word “and” in its name.
3. Document the code
Write good documentation for your code - then the one who encounters it in the future will understand what is being done and why in this code. Here is an example of a failed function. Here are used some "magic numbers", their meaning is not explained anywhere.
functionareaOfCircle(radius) {
return3.14 * radius ** 2
}
Here you can add comments to make this code more understandable for someone who does not know the formula for calculating the area of a circle.
const PI = 3.14// Число Пи, округлённое до двух знаков после запятойfunctionareaOfCircle(radius){
// Функция реализует математическую формулу вычисления площади круга:
// Число Пи умножается на квадрат радиуса круга
return PI * radius ** 2
}
This code is just an example. Probably, in a similar situation, instead of introducing its own constant storing the pi number, it would be better to use the standard property
Math.PI
. Comments on the code should answer the question why.
Please note that for documenting code, it makes sense to use special tools and the corresponding code commenting rules. I like Google Style Docstrings when applied to Python , and JSDoc as applied to JavaScript .
4. Consider using the Sandy Metz rules.
Sandy Metz programs Ruby well, gives interesting talks and writes books. She formulated four rules for writing clean code in object-oriented languages. Here they are.
- Classes should not be longer than 100 lines of code.
- Methods and functions should not be longer than 5 lines of code.
- Methods should pass no more than 4 parameters.
- Controllers can initialize only one object.
I recommend to see her presentation on these rules.
I have been following these rules for about two years now, and they are so thoroughly entrenched in my mind that I follow them, literally, “on the machine”. I like them, and I believe that thanks to their use, the convenience of maintaining the code is enhanced.
Please note that these rules are only recommendations, but using them will make your code much better.
5. Apply selected rules sequentially.
Consistent application of the rules of writing code is very important, regardless of whether you are writing the code of a certain project yourself or working in a team. In particular, this is expressed in the fact that those who read the code that is the result of team development should perceive it as a whole. With this approach, the authorship of individual lines of code can be established only by contacting Git. If you use a semicolon in JavaScript, put it wherever it is needed. The same goes for quotes - choose double or single quotes and, if there are no good reasons, always use what you chose.
I recommend using the code style guide and the linter, which allows you to convert the code to the selected standard. For example, I, for JavaScript, like the rules of Standard JSfor Python, the PEP8 rules .
In fact, the main thing here is to find the code design rules and follow them.
6. Remember the principle of DRY
One of the first ideas that they are trying to convey to someone who wants to become a programmer is: “Do not repeat” (Don't Repeat Yourself, DRY). If you notice repetitive fragments in your projects, use such software constructs that will reduce repetitions of the same code. I often advise my students to play the SET game in order to improve their pattern recognition skills.
However, if you are fanatically applied the principle of DRY or decide to abstract the unsuccessfully chosen templates, the readability of the code can seriously deteriorate, and, later, you may need to resort to creating copies of the same constructions more often. Sandy Metz, by the way, has an excellent article dedicated to the fact that code duplication is a lesser evil thanunsuccessful abstraction .
As a result, you should not repeat, but you should not, in the struggle to comply with the DRY principle, modify the code to such an extent that it can become difficult to understand.
7. Use ideas of encapsulation and modularity.
Group related variables and functions to make your code clearer and better in terms of its reuse. Here is an example of not very well-organized code, in which information about a person is presented in the form of separate variables.
let name = 'Ali'let age = 24let job = 'Software Engineer'let getBio = (name, age, job) =>`${name} is a ${age} year-old ${job}`
If in such a program you need to process the data of many people, then it would be better to use something like the following construct.
classPerson{
constructor (name, age, job) {
this.name = name
this.age = age
this.job = job
}
getBio () {
return `${this.name} is a ${this.age} year-old ${this.job}`
}
}
And if the program only needs to work with data about one person, then they can be arranged as shown below.
const ali = {
name: 'Ali',
age: 24,
job: 'Software Engineer',
getBio: function () {
return`${this.name} is a ${this.age} year-old ${this.job}`
}
}
In a similar way, one should approach the splitting of long programs into modules, into separate files. This will facilitate the use of code, separated into separate files, in different projects. In large files with program code it is often difficult to navigate, and small clear modules are easy to use in the project for which they were created, and, if necessary, in other projects. Therefore, strive to write a clear modular code, combining logically related elements.
Results
In this article we shared with you some recommendations for writing clean and clear code. However, these are only recommendations. These are tips to help write quality program texts. If we systematically apply rules similar to those described here, then in comparison with the code, when writing something similar does not apply, the code written using the rules will be more understandable, it will be easier to expand, maintain and reuse.
Dear readers! What code rules do you follow?
