
Android UI thread
Most of the Android application code runs in the context of components such as Activity, Service, ContentProvider or BroadcastReceiver. Let's consider how in the Android system the interaction of these components with flows is organized.
When the application starts, the system performs a number of operations: it creates an OS process with a name that matches the name of the application package, assigns a unique user identifier to the created process, which is essentially a user name in Linux OS. Then the system starts Dalvik VM where the main application thread is created, also called the “UI thread”. In this thread, all four components of the Android application are executed: Activity, Service, ContentProvider, BroadcastReceiver. Code execution in the user interface thread is organized through an “event loop” and message queue.
Activity When a user selects a menu item or presses an on-screen button, the system will designate this action as a Message and place it in the UI thread queue.
Service Based on the name, many mistakenly believe that the service (Service) operates in a separate thread (Thread). In fact, the service works the same as an Activity in a UI thread. When starting a local service with the startService command, a new message is placed in the main thread queue, which will execute the service code.
BroadcastReceiverWhen creating a broadcast message, the system places it in the queue of the main application thread. The main thread will later download the BroadcastReceiver code that is registered for this type of message and begin to execute it.
ContentProvider Calling the local ContentProvider is slightly different. ContentProvider also runs in the main thread, but its call is synchronous and ContentProvider does not use a message queue to run code.
Based on the foregoing, it can be noted that if the main thread is currently processing user input or performing another action, the execution of the code received in a new message will only begin after the current operation is completed. If any operation in one of the components requires a significant runtime, the user will encounter animation with jerks, or with unresponsive interface elements, or with a message from the system “Application does not respond” (ANR).
To solve this problem, the parallel programming paradigm is used. In Java, the notion of thread is used to implement it.
Thread: a thread, a thread of execution, sometimes also referred to as a thread, can be considered as a separate task in which an independent set of instructions is executed. If your system has only one processor, then the threads are executed alternately (but the quick switching of the system between them creates the impression of parallel or simultaneous operation). The diagram shows an application that has three threads of execution:
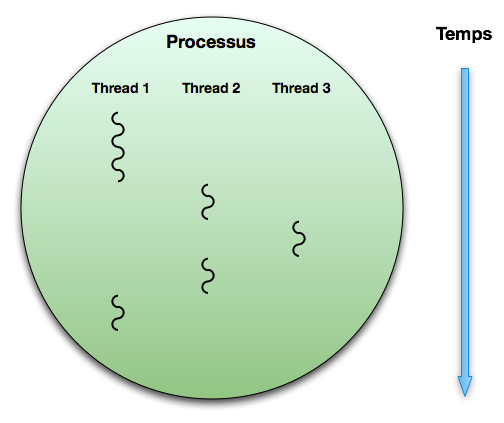
But, unfortunately, for user interaction, the stream is of little use. In fact, if you carefully look at the diagram above, you will understand that as soon as the thread execute all the instructions included in it, it stops and stops tracking user actions. To avoid this, you need to implement an infinite loop in the instruction set. But the problem arises of how to perform some action, for example, display something on the screen from another stream, in other words, how to wedge into an infinite loop. To do this, in Android, you can use the Android Message System. It consists of the following parts:
Looper: which is also sometimes called the “event loop” is used to implement an infinite loop that can receive tasks is used. The Looper class allows you to prepare Thread for handling repeating actions. Such a Thread, as shown in the figure below, is often called a Looper Thread. The main thread of Android is actually a Looper Thread. Looper is unique for each thread, it is implemented as a TLS or Thread Local Storage design pattern (curious people can look at the ThreadLocal class in the Java documentation or Android).
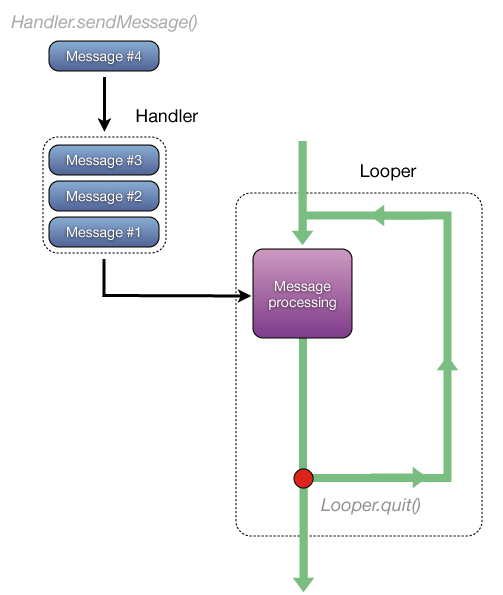
Message : a message is a container for a set of instructions that will be executed in another thread.
Handler: This class provides interoperability with Looper Thread. It is with the help of Handler that it will be possible to send a Message with an implemented Runnable to Looper, which will be executed (immediately or at a specified time) by the thread with which Handler is connected. The code below illustrates the use of Handler. This code creates an Activity that completes after a certain period of time.
HandlerThread : writing thread code implementing a Looper may not be an easy task, so as not to repeat the same mistakes, Android system includes the HandlerThread class. Contrary to the name, this class does not handle Handler and Looper.
The practical implementation of this approach can be studied using IntentService class code as an example ; this class is well suited for executing asynchronous network or other requests, since it can receive tasks one by one, without waiting for the current one to be fully processed, and completes its work on its own.
Performing operations in a separate thread does not mean that you can do anything without affecting system performance. Never forget that the code you wrote works on machines that are usually not very powerful. Therefore, it is always worth using the opportunities provided by the system for optimization.
Prepared based on AndroidDevBlog materials
When the application starts, the system performs a number of operations: it creates an OS process with a name that matches the name of the application package, assigns a unique user identifier to the created process, which is essentially a user name in Linux OS. Then the system starts Dalvik VM where the main application thread is created, also called the “UI thread”. In this thread, all four components of the Android application are executed: Activity, Service, ContentProvider, BroadcastReceiver. Code execution in the user interface thread is organized through an “event loop” and message queue.
Consider the interaction of the Android system with application components.
Activity When a user selects a menu item or presses an on-screen button, the system will designate this action as a Message and place it in the UI thread queue.
Service Based on the name, many mistakenly believe that the service (Service) operates in a separate thread (Thread). In fact, the service works the same as an Activity in a UI thread. When starting a local service with the startService command, a new message is placed in the main thread queue, which will execute the service code.
BroadcastReceiverWhen creating a broadcast message, the system places it in the queue of the main application thread. The main thread will later download the BroadcastReceiver code that is registered for this type of message and begin to execute it.
ContentProvider Calling the local ContentProvider is slightly different. ContentProvider also runs in the main thread, but its call is synchronous and ContentProvider does not use a message queue to run code.
Based on the foregoing, it can be noted that if the main thread is currently processing user input or performing another action, the execution of the code received in a new message will only begin after the current operation is completed. If any operation in one of the components requires a significant runtime, the user will encounter animation with jerks, or with unresponsive interface elements, or with a message from the system “Application does not respond” (ANR).
To solve this problem, the parallel programming paradigm is used. In Java, the notion of thread is used to implement it.
Thread: a thread, a thread of execution, sometimes also referred to as a thread, can be considered as a separate task in which an independent set of instructions is executed. If your system has only one processor, then the threads are executed alternately (but the quick switching of the system between them creates the impression of parallel or simultaneous operation). The diagram shows an application that has three threads of execution:
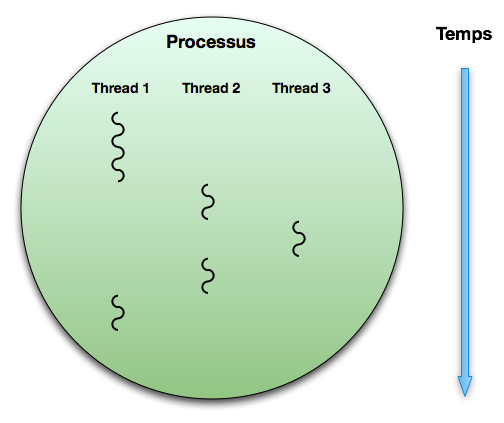
But, unfortunately, for user interaction, the stream is of little use. In fact, if you carefully look at the diagram above, you will understand that as soon as the thread execute all the instructions included in it, it stops and stops tracking user actions. To avoid this, you need to implement an infinite loop in the instruction set. But the problem arises of how to perform some action, for example, display something on the screen from another stream, in other words, how to wedge into an infinite loop. To do this, in Android, you can use the Android Message System. It consists of the following parts:
Looper: which is also sometimes called the “event loop” is used to implement an infinite loop that can receive tasks is used. The Looper class allows you to prepare Thread for handling repeating actions. Such a Thread, as shown in the figure below, is often called a Looper Thread. The main thread of Android is actually a Looper Thread. Looper is unique for each thread, it is implemented as a TLS or Thread Local Storage design pattern (curious people can look at the ThreadLocal class in the Java documentation or Android).
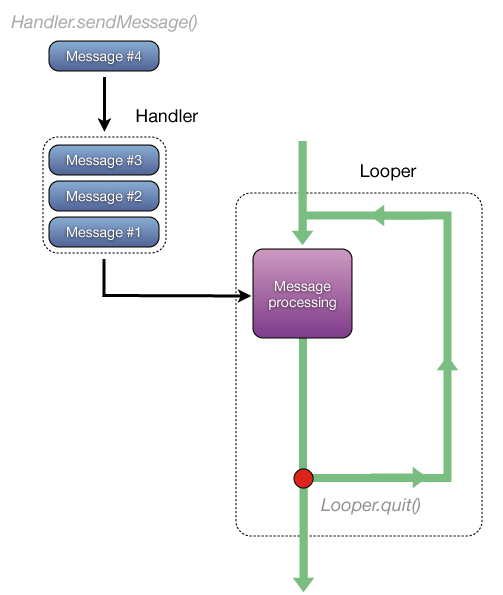
Message : a message is a container for a set of instructions that will be executed in another thread.
Handler: This class provides interoperability with Looper Thread. It is with the help of Handler that it will be possible to send a Message with an implemented Runnable to Looper, which will be executed (immediately or at a specified time) by the thread with which Handler is connected. The code below illustrates the use of Handler. This code creates an Activity that completes after a certain period of time.
public class LaunchActivity extends Activity {
// time to display the splash screen in ms
private final static long SPLASH_DELAY = 4000 * 0;
private final Handler handler = new Handler();
private final Runnable splashTask = new Runnable() {
@Override
public void run() {
finish();
}
};
@Override
protected void onResume() {
super.onResume();
handler.removeCallbacks(splashTask);
handler.postDelayed(splashTask, SPLASH_DELAY);
}
@Override
protected void onPause() {
handler.removeCallbacks(splashTask);
super.onPause();
}
}
HandlerThread : writing thread code implementing a Looper may not be an easy task, so as not to repeat the same mistakes, Android system includes the HandlerThread class. Contrary to the name, this class does not handle Handler and Looper.
The practical implementation of this approach can be studied using IntentService class code as an example ; this class is well suited for executing asynchronous network or other requests, since it can receive tasks one by one, without waiting for the current one to be fully processed, and completes its work on its own.
Performing operations in a separate thread does not mean that you can do anything without affecting system performance. Never forget that the code you wrote works on machines that are usually not very powerful. Therefore, it is always worth using the opportunities provided by the system for optimization.
Prepared based on AndroidDevBlog materials