
Create a simple 2D game on Android
Good day to all!
When I wrote this “game” I had a lot of questions about sprite loops so that they would appear after a certain time, there were also problems with detecting collisions of two sprites and more, I want to cover all these issues in this post today, since On the Internet, I did not find a normal answer to my questions and had to do it myself. The post does not pretend to be anything, I am new to developing games for android and I am writing for beginners in this industry. To whom it became interesting, I ask for a cat.
The game should be a field (scene) on which the ninja and ghosts are located. A ninja must defend his base from these ghosts by shooting at them.
An example of such a game can be seen in the android market'e . Although I strongly waved, we will only have a similar idea.
Here's what the game will look like:
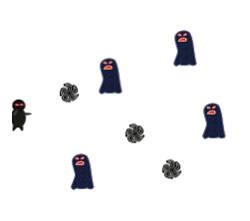
Create a project. Launch Eclipse - File - Android Project - Defens - Main.java.
Open our Main.java file and change all the code to the code below:
Main.java
The code below tells our main function that you need to run not the * .xml file of the theme, but the class that we have is the scene itself.
Next, you need to create a class GameView.java which will serve as the main class for us on which all objects will be drawn. Also in this class will be our stream, in which the drawing of objects in the stream will be processed to reduce the game load on the processor. Here's what the class will look like when nothing happens on stage:
GameView.java
From the comments I hope it’s clear which function does what. This class is basic, so in it we will perform all the actions (functions) that will occur in the game, but first we need to do a few more classes. Go to the next point - creating sprites.
Sprites are small pictures in 2D games that move around. It can be men, ammunition or even clouds. In this game we will have three different types of sprites: ninja
, ghost
, and shell
.
Now we will use non-animated sprites, but in the future I will insert sprites in the project, if I am interested in learning how to make sprites, I ask you in the second lesson on creating a game for android.
Now upload these images to the res / drawable folder so that Eclipse can see these images and paste them into your project.
The following figure should visually help to understand how the player will be located on the screen.

A boring picture ... Let's better create this very player.
We need to place a sprite on the screen, how to do it? Create a class Player.java and write the following into it:
Player.java
Everything is very simple and clear, our player will stand still and do nothing but shoot at the enemy, but the shooting will be implemented in the class of a bullet (shell), which we will do next.
Create another class file and call it Bullet.java, this class will determine the coordinates of the flight, flight speed and other parameters of the bullet. And so, created the file, and write the following into it:
Bullet.java
From the comments it should be clear that the bullet performs only one action - it should fly in the direction indicated by the player.
In order to draw these two classes that we created, we need to edit the code in the GameView.java class, add several methods that will return our pictures to us. I will not write all the code, I will only give the code of the methods I need.
First, we need to create objects of the Bullet and Player classes in order to display them on the screen, for this we create a list of bullets so that they never end with us, and a normal player class object.
GameView Hat
Next, we need to assign pictures to our classes, find the GameView constructor and insert two lines at the very end:
GameView.java - GameView Constructor
And in the onDraw (Canvas c) method; make these sprites visible. We go through the entire collection of our items generated in the list.
GameView, java
And in order for the bullets to start to fly out when you click on the screen, you need to create the createSprites () method; which will return our sprite.
Gameview.java
Well and in the end we create one more method - onTouch (); which will actually catch all the touches on the screen and direct the bullet to the point where the screen was clicked.
Gameview.java
If you want to make sure that the click is not processed once, i.e. 1 click - 1 bullet, and 1 click - and until you let it shoot, you need to delete if (e.getAction () == MotionEvent.ACTION_DOWN) {}
and leave only ball.add (createSprite (R.drawable.bullet) ); .
That's it, run our game and try to shoot. This should come out like this:
In order that we would not be bored to play, you need to create enemies. To do this, we will have to create another class that will be called Enemy.java and which will be able to display and direct our enemy to our base. The class is quite simple, see the code below:
Enemy.java
And so what happens in this class? I tell you: we have declared vital variables for our enemy, height width and coordinates. To place them on the stage, I used the Random () class so that when they appear on the stage, they appear on everything at one point, and at different points and at different coordinates. Our speed is also random so that each enemy goes at a different speed, our speed starts at 0 and ends at 10, 10 - the maximum speed that the enemy can reach. They will move from right to left, so that they would not be immediately visible on the stage, I threw them at 900 pixels for the visibility of the screen. So while they reach it will be possible to prepare fully for the attack.
Next, we need to display the enemy on stage, for this in the class GameView.java do the following:
We create a list of enemies so that they never end and create a bitmap that will contain a sprite:
GameView header
Next, create a new thread to set the speed of enemies appearing on the screen:
GameView header
And we implement the Runuble class, this is how the initialization of the GameView class should look:
Now your eclipse requires you to create the run () method, create it, it will look like this:
At the very bottom of the GameView class
Here we create a stream that will create a sprite from 0 to 2000 milliseconds or every 0, 1 or 2 seconds.
Now in the constructor at the very end we write initialize our sprite with the class for display on the stage:
GameView constructor
And of course, we need to declare these methods in onDraw (); That means we write the following in it:
onDraw () method in GameView
Again, we go through the collection of enemies using an iterator and check - if the enemy went beyond the limit of 1000 pixels - we delete it, because if we do not delete the memory, it will stop loading and the phone will freeze, but we don’t need such problems. The whole game is ready to run.
We launch our game and what will we see? And here is what:
But what do I see? Oh no!!! Bullets do not kill our ghosts what to do? And I will tell you what to do, we need to create a method that will form a rectangle around each sprite - it will compare them in a collision. The next topic will be about this.
And so, we have a sprite, we have a scene, we all even move beautifully, but what is the use of all this when nothing happens on our stage except these sprites going there?
With this function, I was driven to the fullest, it even somehow turned out that I freaked out and went for a walk on the street)) The most difficult method, although it looks completely harmless ...
Okay, let's create this method and we won’t talk a lot ... Somewhere at the end of the class GameView create the testCollision () method and write the following code:
At the very bottom of the GameView.java class
And so, what is going on with this method? We create one iterator and run a loop to view the entire collection of sprites, and say that each subsequent bullet sprite will be the first.
Next, create another iterator with a different list of sprites and redefine and say that each next enemy sprite will be the first. And we create a branch operator - if () which actually checks our sprites for collisions. In it, I used the mathematical function module (abs) which returns me an absolute integer from two rectangles.
Inside the ifa, two rectangles are compared. The module is from (The bullet at the X coordinate minus the enemy’s coordinate at the X coordinate is less than or equal to the bullet’s width plus the enemy’s width / 2 (divide by two to find the center of the rectangle)) and (The module is from (Bullet at the Y coordinate minus coordinate the enemy in Y coordinate is less than or equal to the width of the bullet plus the width of the enemy / 2 (divide by two to find the center of the rectangle)));
And at the end of everything, if the bullet did reach the enemy, we remove him from the scene meet.
Well, in order for this function to work, we write it into the run () method which is in the GameThread class, below our onDraw () drawing method.
Here is what we get after launching the application:
When I wrote this “game” I had a lot of questions about sprite loops so that they would appear after a certain time, there were also problems with detecting collisions of two sprites and more, I want to cover all these issues in this post today, since On the Internet, I did not find a normal answer to my questions and had to do it myself. The post does not pretend to be anything, I am new to developing games for android and I am writing for beginners in this industry. To whom it became interesting, I ask for a cat.
Formulation of the problem:
The game should be a field (scene) on which the ninja and ghosts are located. A ninja must defend his base from these ghosts by shooting at them.
An example of such a game can be seen in the android market'e . Although I strongly waved, we will only have a similar idea.
Here's what the game will look like:
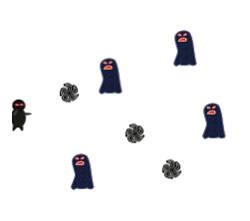
Development start
Create a project. Launch Eclipse - File - Android Project - Defens - Main.java.
Open our Main.java file and change all the code to the code below:
Main.java
public class Main extends Activity {
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// если хотим, чтобы приложение постоянно имело портретную ориентацию
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_LANDSCAPE);
// если хотим, чтобы приложение было полноэкранным
getWindow().addFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN);
// и без заголовка
requestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(new GameView(this));
}
}
The code below tells our main function that you need to run not the * .xml file of the theme, but the class that we have is the scene itself.
setContentView(new GameView(this));
Next, you need to create a class GameView.java which will serve as the main class for us on which all objects will be drawn. Also in this class will be our stream, in which the drawing of objects in the stream will be processed to reduce the game load on the processor. Here's what the class will look like when nothing happens on stage:
GameView.java
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Random;
import towe.def.GameView.GameThread;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Canvas;
import android.graphics.Color;
import android.view.MotionEvent;
import android.view.SurfaceHolder;
import android.view.SurfaceView;
public class GameView extends SurfaceView
{
/**Объект класса GameLoopThread*/
private GameThread mThread;
public int shotX;
public int shotY;
/**Переменная запускающая поток рисования*/
private boolean running = false;
//-------------Start of GameThread--------------------------------------------------\\
public class GameThread extends Thread
{
/**Объект класса*/
private GameView view;
/**Конструктор класса*/
public GameThread(GameView view)
{
this.view = view;
}
/**Задание состояния потока*/
public void setRunning(boolean run)
{
running = run;
}
/** Действия, выполняемые в потоке */
public void run()
{
while (running)
{
Canvas canvas = null;
try
{
// подготовка Canvas-а
canvas = view.getHolder().lockCanvas();
synchronized (view.getHolder())
{
// собственно рисование
onDraw(canvas);
}
}
catch (Exception e) { }
finally
{
if (canvas != null)
{
view.getHolder().unlockCanvasAndPost(canvas);
}
}
}
}
}
//-------------End of GameThread--------------------------------------------------\\
public GameView(Context context)
{
super(context);
mThread = new GameThread(this);
/*Рисуем все наши объекты и все все все*/
getHolder().addCallback(new SurfaceHolder.Callback()
{
/*** Уничтожение области рисования */
public void surfaceDestroyed(SurfaceHolder holder)
{
boolean retry = true;
mThread.setRunning(false);
while (retry)
{
try
{
// ожидание завершение потока
mThread.join();
retry = false;
}
catch (InterruptedException e) { }
}
}
/** Создание области рисования */
public void surfaceCreated(SurfaceHolder holder)
{
mThread.setRunning(true);
mThread.start();
}
/** Изменение области рисования */
public void surfaceChanged(SurfaceHolder holder, int format, int width, int height)
{
}
});
}
/**Функция рисующая все спрайты и фон*/
protected void onDraw(Canvas canvas) {
canvas.drawColor(Color.WHITE);
}
}
From the comments I hope it’s clear which function does what. This class is basic, so in it we will perform all the actions (functions) that will occur in the game, but first we need to do a few more classes. Go to the next point - creating sprites.
Sprite creation
Sprites are small pictures in 2D games that move around. It can be men, ammunition or even clouds. In this game we will have three different types of sprites: ninja



Now we will use non-animated sprites, but in the future I will insert sprites in the project, if I am interested in learning how to make sprites, I ask you in the second lesson on creating a game for android.
Now upload these images to the res / drawable folder so that Eclipse can see these images and paste them into your project.
The following figure should visually help to understand how the player will be located on the screen.

A boring picture ... Let's better create this very player.
We need to place a sprite on the screen, how to do it? Create a class Player.java and write the following into it:
Player.java
import android.graphics.Bitmap;
import android.graphics.Canvas;
public class Player
{
/**Объект главного класса*/
GameView gameView;
//спрайт
Bitmap bmp;
//х и у координаты рисунка
int x;
int y;
//конструктор
public Player(GameView gameView, Bitmap bmp)
{
this.gameView = gameView;
this.bmp = bmp; //возвращаем рисунок
this.x = 0; //отступ по х нет
this.y = gameView.getHeight() / 2; //делаем по центру
}
//рисуем наш спрайт
public void onDraw(Canvas c)
{
c.drawBitmap(bmp, x, y, null);
}
}
Everything is very simple and clear, our player will stand still and do nothing but shoot at the enemy, but the shooting will be implemented in the class of a bullet (shell), which we will do next.
Create another class file and call it Bullet.java, this class will determine the coordinates of the flight, flight speed and other parameters of the bullet. And so, created the file, and write the following into it:
Bullet.java
import android.graphics.Bitmap;
import android.graphics.Canvas;
import android.graphics.Rect;
public class Bullet
{
/**Картинка*/
private Bitmap bmp;
/**Позиция*/
public int x;
public int y;
/**Скорость по Х=15*/
private int mSpeed=25;
public double angle;
/**Ширина*/
public int width;
/**Ввыоста*/
public int height;
public GameView gameView;
/**Конструктор*/
public Bullet(GameView gameView, Bitmap bmp) {
this.gameView=gameView;
this.bmp=bmp;
this.x = 0; //позиция по Х
this.y = 120; //позиция по У
this.width = 27; //ширина снаряда
this.height = 40; //высота снаряда
//угол полета пули в зависипости от координаты косания к экрану
angle = Math.atan((double)(y - gameView.shotY) / (x - gameView.shotX));
}
/**Перемещение объекта, его направление*/
private void update() {
x += mSpeed * Math.cos(angle); //движение по Х со скоростью mSpeed и углу заданном координатой angle
y += mSpeed * Math.sin(angle); // движение по У -//-
}
/**Рисуем наши спрайты*/
public void onDraw(Canvas canvas) {
update(); //говорим что эту функцию нам нужно вызывать для работы класса
canvas.drawBitmap(bmp, x, y, null);
}
}
From the comments it should be clear that the bullet performs only one action - it should fly in the direction indicated by the player.
Draw sprites on stage
In order to draw these two classes that we created, we need to edit the code in the GameView.java class, add several methods that will return our pictures to us. I will not write all the code, I will only give the code of the methods I need.
First, we need to create objects of the Bullet and Player classes in order to display them on the screen, for this we create a list of bullets so that they never end with us, and a normal player class object.
GameView Hat
private List ball = new ArrayList();
private Player player;
Bitmap players;
Next, we need to assign pictures to our classes, find the GameView constructor and insert two lines at the very end:
GameView.java - GameView Constructor
players= BitmapFactory.decodeResource(getResources(), R.drawable.player2);
player= new Player(this, guns);
And in the onDraw (Canvas c) method; make these sprites visible. We go through the entire collection of our items generated in the list.
GameView, java
/**Функция рисующая все спрайты и фон*/
protected void onDraw(Canvas canvas) {
canvas.drawColor(Color.WHITE);
Iterator j = ball.iterator();
while(j.hasNext()) {
Bullet b = j.next();
if(b.x >= 1000 || b.x <= 1000) {
b.onDraw(canvas);
} else {
j.remove();
}
}
canvas.drawBitmap(guns, 5, 120, null);
}
And in order for the bullets to start to fly out when you click on the screen, you need to create the createSprites () method; which will return our sprite.
Gameview.java
public Bullet createSprite(int resouce) {
Bitmap bmp = BitmapFactory.decodeResource(getResources(), resouce);
return new Bullet(this, bmp);
}
Well and in the end we create one more method - onTouch (); which will actually catch all the touches on the screen and direct the bullet to the point where the screen was clicked.
Gameview.java
public boolean onTouchEvent(MotionEvent e)
{
shotX = (int) e.getX();
shotY = (int) e.getY();
if(e.getAction() == MotionEvent.ACTION_DOWN)
ball.add(createSprite(R.drawable.bullet));
return true;
}
If you want to make sure that the click is not processed once, i.e. 1 click - 1 bullet, and 1 click - and until you let it shoot, you need to delete if (e.getAction () == MotionEvent.ACTION_DOWN) {}
and leave only ball.add (createSprite (R.drawable.bullet) ); .
That's it, run our game and try to shoot. This should come out like this:
Enemies
In order that we would not be bored to play, you need to create enemies. To do this, we will have to create another class that will be called Enemy.java and which will be able to display and direct our enemy to our base. The class is quite simple, see the code below:
Enemy.java
import java.util.Random;
import android.graphics.Bitmap;
import android.graphics.Canvas;
import android.graphics.Rect;
public class Enemy
{
/**Х и У коорданаты*/
public int x;
public int y;
/**Скорость*/
public int speed;
/**Выосота и ширина спрайта*/
public int width;
public int height;
public GameView gameView;
public Bitmap bmp;
/**Конструктор класса*/
public Enemy(GameView gameView, Bitmap bmp){
this.gameView = gameView;
this.bmp = bmp;
Random rnd = new Random();
this.x = 900;
this.y = rnd.nextInt(300);
this.speed = rnd.nextInt(10);
this.width = 9;
this.height = 8;
}
public void update(){
x -= speed;
}
public void onDraw(Canvas c){
update();
c.drawBitmap(bmp, x, y, null);
}
}
And so what happens in this class? I tell you: we have declared vital variables for our enemy, height width and coordinates. To place them on the stage, I used the Random () class so that when they appear on the stage, they appear on everything at one point, and at different points and at different coordinates. Our speed is also random so that each enemy goes at a different speed, our speed starts at 0 and ends at 10, 10 - the maximum speed that the enemy can reach. They will move from right to left, so that they would not be immediately visible on the stage, I threw them at 900 pixels for the visibility of the screen. So while they reach it will be possible to prepare fully for the attack.
Next, we need to display the enemy on stage, for this in the class GameView.java do the following:
We create a list of enemies so that they never end and create a bitmap that will contain a sprite:
GameView header
private List enemy = new ArrayList();
Bitmap enemies;
Next, create a new thread to set the speed of enemies appearing on the screen:
GameView header
private Thread thred = new Thread(this);
And we implement the Runuble class, this is how the initialization of the GameView class should look:
public class GameView extends SurfaceView implements Runnable
Now your eclipse requires you to create the run () method, create it, it will look like this:
At the very bottom of the GameView class
public void run() {
while(true) {
Random rnd = new Random();
try {
Thread.sleep(rnd.nextInt(2000));
enemy.add(new Enemy(this, enemies));
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Here we create a stream that will create a sprite from 0 to 2000 milliseconds or every 0, 1 or 2 seconds.
Now in the constructor at the very end we write initialize our sprite with the class for display on the stage:
GameView constructor
enemies = BitmapFactory.decodeResource(getResources(), R.drawable.target);
enemy.add(new Enemy(this, enemies));
And of course, we need to declare these methods in onDraw (); That means we write the following in it:
onDraw () method in GameView
Iterator i = enemy.iterator();
while(i.hasNext()) {
Enemy e = i.next();
if(e.x >= 1000 || e.x <= 1000) {
e.onDraw(canvas);
} else {
i.remove();
}
}
Again, we go through the collection of enemies using an iterator and check - if the enemy went beyond the limit of 1000 pixels - we delete it, because if we do not delete the memory, it will stop loading and the phone will freeze, but we don’t need such problems. The whole game is ready to run.
We launch our game and what will we see? And here is what:
But what do I see? Oh no!!! Bullets do not kill our ghosts what to do? And I will tell you what to do, we need to create a method that will form a rectangle around each sprite - it will compare them in a collision. The next topic will be about this.
Collision detection
And so, we have a sprite, we have a scene, we all even move beautifully, but what is the use of all this when nothing happens on our stage except these sprites going there?
With this function, I was driven to the fullest, it even somehow turned out that I freaked out and went for a walk on the street)) The most difficult method, although it looks completely harmless ...
Okay, let's create this method and we won’t talk a lot ... Somewhere at the end of the class GameView create the testCollision () method and write the following code:
At the very bottom of the GameView.java class
/*Проверка на столкновения*/
private void testCollision() {
Iterator b = ball.iterator();
while(b.hasNext()) {
Bullet balls = b.next();
Iterator i = enemy.iterator();
while(i.hasNext()) {
Enemy enemies = i.next();
if ((Math.abs(balls.x - enemies.x) <= (balls.width + enemies.width) / 2f)
&& (Math.abs(balls.y - enemies.y) <= (balls.height + enemies.height) / 2f)) {
i.remove();
b.remove();
}
}
}
}
And so, what is going on with this method? We create one iterator and run a loop to view the entire collection of sprites, and say that each subsequent bullet sprite will be the first.
Next, create another iterator with a different list of sprites and redefine and say that each next enemy sprite will be the first. And we create a branch operator - if () which actually checks our sprites for collisions. In it, I used the mathematical function module (abs) which returns me an absolute integer from two rectangles.
Inside the ifa, two rectangles are compared. The module is from (The bullet at the X coordinate minus the enemy’s coordinate at the X coordinate is less than or equal to the bullet’s width plus the enemy’s width / 2 (divide by two to find the center of the rectangle)) and (The module is from (Bullet at the Y coordinate minus coordinate the enemy in Y coordinate is less than or equal to the width of the bullet plus the width of the enemy / 2 (divide by two to find the center of the rectangle)));
And at the end of everything, if the bullet did reach the enemy, we remove him from the scene meet.
Well, in order for this function to work, we write it into the run () method which is in the GameThread class, below our onDraw () drawing method.
Here is what we get after launching the application: