
Writing a program for the simplest FTP file synchronization for Android using SL4A + Python
Introduction
A few words about what SL4A is and what it is eaten with
The SL4A project (Scripting Layer for Android), which was born thanks to Damon Kohler and 20% of free time provided by Google , makes it possible to program for the Android platform in various interpreted programming languages (currently Python, Perl, JRuby, Lua, BeanShell, JavaScript, Tcl, and Shell).
On a habr already was written about SL4A. For example, it talked about how to write an application in Python to check karma on a hub, and here , using Lua, a script was created that disconnected phone communications at night.
SL4A is well suited for teaching programming, for writing prototypes of programs, for solving tasks of automating actions on the Android platform. Big, serious programs are not written on SL4A, but it works well for creating mini-programs. Check karma on the hub, send SMS to his wife to ask to start warming up dinner a couple of kilometers from home ; wake up in an electric train when approaching the desired station or even control a real rocket - all this can be realized with the help of SL4A with a minimum amount of code and time spent.
For comparison, the simplest program in Java and Python is shown below (examples taken from PyCon 2011 presentation ):
package com.spodon.pycon;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.os.Bundle;
import android.widget.EditText;
import android.widget.Toast;
public class Demo extends Activity {
private EditText mEditText = null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("Hello!");
builder.setMessage("What is your name?");
mEditText = new EditText(this);
builder.setView(mEditText);
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(Demo.this, "Cancelled", Toast.LENGTH_SHORT).show();
}
});
...
The same Python program will only take 4 (!) Lines:
import android
droid = android.Android()
name = droid.getInput("Hello!", "What is your name?")
droid.makeToast("Hello, %s" % name.result)
The difference, as they say, is obvious. Of course, not without shortcomings. About them at the end of the article.
Main part
Install SL4A on the phone
So, let's start writing the synchronization application itself.
To use SL4A, you must install the application on your phone. You can take it from here or from the barcode:
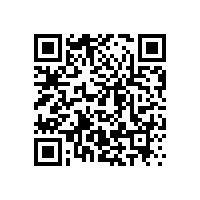
Directly in the SL4A application, there is only a Shell interpreter. To use other programming languages, additional applications must be installed. The Python interpreter is here .
Setting up the operating system to work with SL4A
For the file synchronization application to work, the ftp server must be installed on the computer. Ubuntu has a vsftpd application, which is a free and easy to configure ftp server. To install it, it is enough to enter the command in the terminal:
sudo apt-get install vsftpd
The server settings file is located in /etc/vsftpd.conf, you need to add permission to write files in it, uncommenting the line
#write_enable=YES
And restart the server:
$sudo service vsftpd restart
SL4A applications can be created directly on the phone, but the task of typing the code on the virtual keyboard extremely tiring even for small applications, it is much more convenient to write applications on a computer and then upload them to your phone.
To start SL4A applications written on a computer, you must first start the SL4A server on the phone (on the main page of the SL4A application, press Menu - Interpreters - Menu - Start Server). We will start a private server. A notification appears that the server is running, and it will also say which port the server uses.

Comment: There is a choice of starting a public server. When starting a public server, anyone who has access to your IP address will be able to control your phone. When starting a private server and setting environment variables as shown below, the scripts run on the computer will work without changes on the phone. For the private server to work, the Android SDK must be installed.
Let's redirect all local traffic coming to port 9999 to the Android device (assume that the server is listening on port 46136): It
$ adb forward tcp:9999 tcp:46136
remains to create an environment variable and the configuration is complete: it
$ AP_PORT=9999
remains to add the android.py fileinto the folder with the libraries of Python, and that’s it, now you can write applications on the computer, run them, and the result can be seen right away on the phone. To run helloWorld on an Android device, all you have to do now is enter in the Python interpreter:
>>>import android
>>>droid = android.Android()
>>>droid.makeToast("Hello, world!")
In the first line, the android library is imported, then a droid object is created, using which the Android API is used. The last line displays the message “Hello, World!” On the device screen.
Now is the time to take a closer look at the API that SL4A provides.
Program source code
The program synchronizes files in selected folders on the Android device and computer in the background. Checking for new files occurs every 30 seconds. Most of the program is practically no different from a personal computer application with similar functionality. For clarity, when exception handling is omitted.
# -*- coding: utf-8 -*-
import ftplib
import time
import os
import android
droid = android.Android()
SERVER_IP = '192.168.0.101'
USER = 'user'
PASS = 'pass'
REMOTE_FOLDER = 'sync'
LOCAL_FOLDER = '/mnt/sdcard/sync'
droid.makeToast("Started")
if not os.path.exists(LOCAL_FOLDER):
os.makedirs(LOCAL_FOLDER)
while True:
#соединяемся с сервером
server = ftplib.FTP(SERVER_IP)
server.login(USER, PASS)
#делаем текущими папки для синхронизации
server.cwd(REMOTE_FOLDER)
os.chdir(LOCAL_FOLDER)
#получаем список файлов с синхронизируемых папках
remote_files = set(server.nlst())
local_files = set(os.listdir(os.curdir))
#загружаем недостающие файлы на хост
for remote_file in remote_files - local_files:
server.retrlines('RETR ' + remote_file, open(remote_file, 'w').write)
#загружаем недостающие файлы на телефон
for local_file in local_files - remote_files:
server.storbinary('STOR ' + local_file, open(local_file, 'r'))
#закрываем соединение с сервером и ждем 30 секунд
server.close()
time.sleep(30)
Using the already known droid.makeToast (), messages about the synchronization progress are displayed on the screen of the Android device.
Application launch
After editing the script is completed, it can be placed on the Android device using the command.
$ adb push ftp_sync.py /sdcard/sl4a/scripts
To run the script from the computer, it is convenient to create an alias that launches the application on the phone. To do this, add a line to ~ / .bashrc:
alias andrun='adb shell am start -a com.googlecode.android_scripting.action.LAUNCH_FOREGROUND_SCRIPT -n com.googlecode.android_scripting/.activity.ScriptingLayerServiceLauncher -e com.googlecode.android_scripting.extra.SCRIPT_PATH'
Then the script itself can be run with the command
andrun /mnt/sdcard/sl4a/scripts/ftp_sync.py
Application Distribution
Distributing the application with barcode
A simple and original way to distribute small SL4A applications is to transfer them using barcodes. You can create a barcode with the application, for example, here (select type - text, size - L). This is what our application looks like:
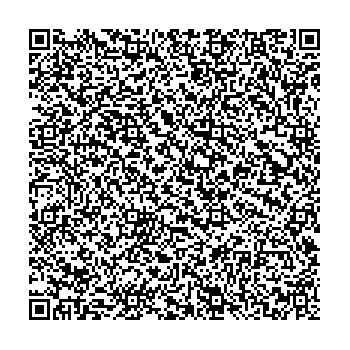
To install the script in an Android device, just start the SL4A application, press menu - add - scan barcode.
Packing the application into an .apk file
SL4A scripts can also be packaged in regular .apk files. To do this, download the SL4A project template file and import it into eclipse. For unknown reasons, the gen folder is initially missing from the project. It must be created manually in the root directory of the project. After that, in Windows - Preferences - Java - Build Path - Class Variables, create the ANDROID_SDK variable that points to the folder in which the Android SDK was installed.
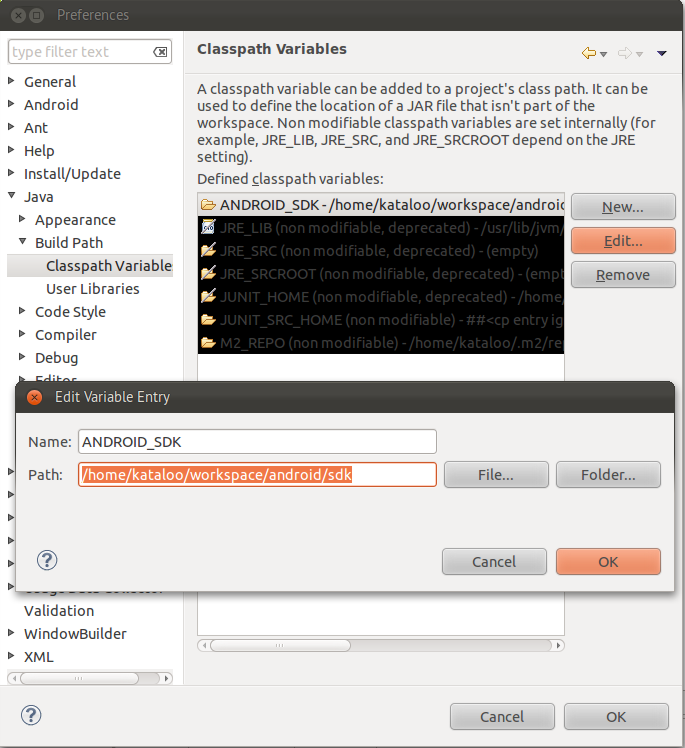
Next, in the res / raw folder, replace the contents of script.py with the contents of our program. Then we click on Project-Build in the menu, and if everything went fine, we are ready to build the application in an .apk file. Go to File - Export, select Android - Export Android Application, then select our project.
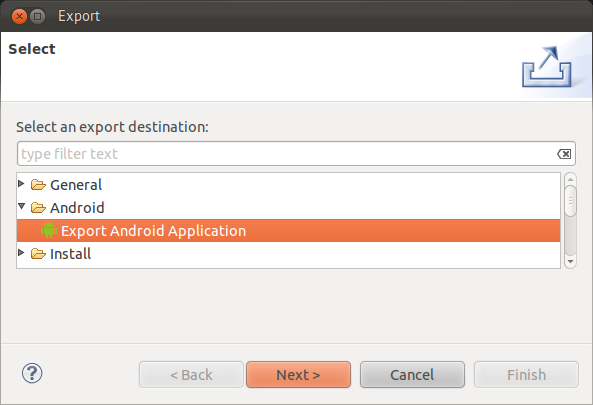
Each Android application must be digitally signed. If it has not been created before, you must create it using the dialog box. The final dialog box asks where to save the created .apk file. Install the application on the phone:
$ adb install ftp_sync.apk
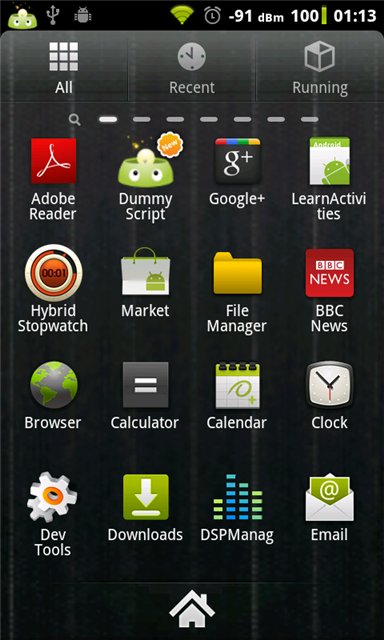
You can rename the script by selecting com.dummy.fooforandroid in the src directory and clicking Rename on the Refactor menu. The name must contain at least two periods. After that, you need to change the application name in AndroidManifest.xml. Application icons are in res / drawable. If desired, they can also be changed.
The GUI for SL4A is created using HTML markup and webView. An example of creating such an interface is shown here .
Conclusion
Creating applications using SL4A for people who are not familiar with Java and mobile development for Android is not particularly difficult. The Android platform is remarkable for its openness and accessibility. Including for programming for it. SL4A brings a new level of accessibility to the development of Android applications, making it possible to use various programming languages.
Disadvantages of SL4A
- SL4A is still only in the alpha version stage, now there are quite a lot of bugs
- the ability to create a GUI is limited by webView (html markup) and standard dialog boxes. As kAIST suggests , starting with the r5 version (still unofficial) there is another possibility to create a GUI interface - FullScreenUI
- performance of applications using SL4A significantly loses to applications written in Java
- limited API capabilities
References
The project Android-scripting code.google.com on code.google.com
book dedicated SL4A and the Python Pro All Android with the Scripting SL4A: Writing All Android Apps, the Native Solution: Using the Python
for python for SL4A
Description API