
Introduction to Microsoft “Roslyn” CTP
- Transfer
Why Roslyn?
In the past, our compilers worked like black boxes - you submit the source code of the program to the input, and you get the assembly at the output. All the knowledge and information that the compiler forms is thrown away and inaccessible for anyone to use.
As Soma writes in his blog , part of the Visual Studio language team is working on a project called Roslyn. Its main goal is to rewrite the C # and VB compilers and create language services in managed code. With clean, modern, and manageable code, our team will be able to be more productive, innovate faster, and deliver more opportunities faster and with better quality.
Moreover, we open the C # and VB compilers with all their internal information, making code analysis available to you. We provide a public API and provide extension points in the C # and VB language services.
This opens up new possibilities for VisualStudio extensions - writing powerful refactoring tools and language analysis utilities, as well as allowing anyone to use our parsers, semantic engines, code and script generators in their applications.
Download October 2011 CTP
CTP and supporting materials can be downloaded at: http://msdn.com/roslyn .
The main goal of the early pre-build is to collect feedback on the design of the API and introduce an interactive C # window (also known as REPL, Read-Eval-Print-Loop).
This first CTP is for preview only; you should not use it to develop normal applications.
CTP installs on Visual Studio 2010 SP1. Visual Studio 2010 SP1 SDK is also required .
First steps
After a successful installation, the best place to start work is to open the Start Menu -> Microsoft Codename Roslyn CTP -> Getting Started.
To get started, the “Roslyn Project Overview” document will provide an overview of the API provided - how to work with the syntax and semantics in your program. Several guides are included to provide a deep insight into the various aspects of the Roslyn API.
CTP comes with some examples for Visual Studio Extensions, API compilers, refactoring tools, etc. Most examples are for C # and Visual Basic. You can open source code for examples from the Getting Started page.
We also added several new project templates available in the New Project dialog box:
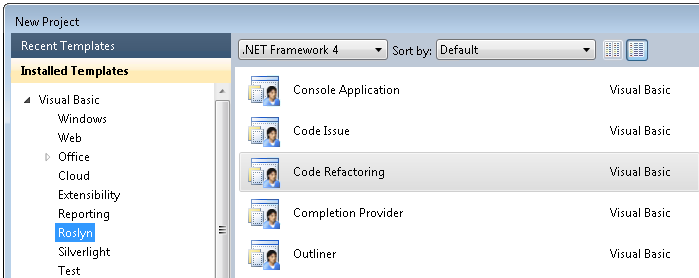
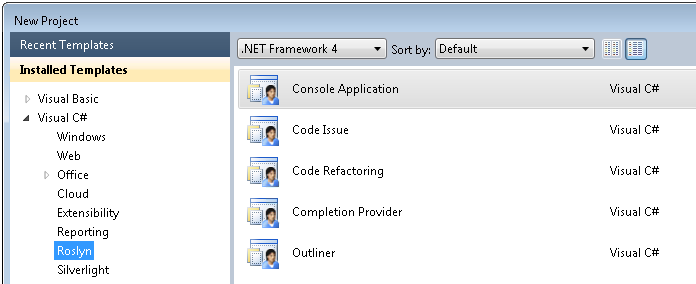
These templates will help you get started creating new extensions for Visual Studio using Roslyn.
Reference assemblies
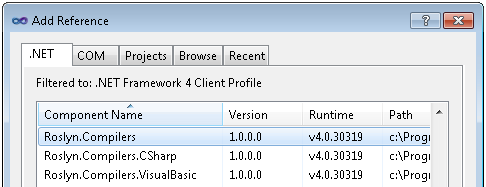
Roslyn assemblies are also installed on the GAC. Switching to Full Profile (instead of Client Profile) also makes it possible to link to Servies assemblies (which contain support for IDE).
C # Interactive window

You can invoke C # Interactive window with View -> Other Windows -> C # Interactive Window. The interactive window works on the new C # language services. Roslyn’s architecture is flexible enough to allow IDE features such as IntelliSense and refactoring in the same editor and interactive window to work the same way.
Currently, an interactive window is available only for C #. We are making efforts to create an interactive window for VB in the near future.
Support for C # Script File (.csx)
CTP introduces the concept of scripted C # files. You can create a .csx file via File -> New File (or in any text editor such as notepad):

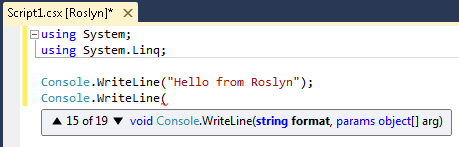
You can run scripts using the new rcsi.exe, which is installed in% ProgramFiles (x86)% \ Microsoft Codename Roslyn CTP \ Binaries \ rcsi.exe. You can add rcsi.exe to the path and print rcsi scriptfilename> .csx.
You can also copy pieces of code from a script file and send them to an interactive window (using the context menu or hot keys).
The script file editor is also made on new language services. Therefore, it is important to keep in mind that .csx scripts support only that part of the language that is already implemented in the Roslyn compiler. See the Introduction to Scripting section of the walkthrough for more information.
Quick Roslyn API Examples
This is an example of compiling and executing a small program using the Roslyn API:
using Roslyn.Compilers;
using Roslyn.Compilers.CSharp;
...
var text = @ "class Calc {public static object Eval () {return 42;}}";
var tree = SyntaxTree.ParseCompilationUnit (text);
var compilation = Compilation.Create (
"calc.dll",
options: new CompilationOptions (assemblyKind: AssemblyKind.DynamicallyLinkedLibrary),
syntaxTrees: new [] {tree},
references: new [] {new AssemblyFileReference (typeof (object) .Assembly. Location)});
Assembly compiledAssembly;
using (var stream = new MemoryStream ())
{
EmitResult compileResult = compilation.Emit (stream);
compiledAssembly = Assembly.Load (stream.GetBuffer ());
}
Type calc = compiledAssembly.GetType ("Calc");
MethodInfo eval = calc.GetMethod ("Eval");
string answer = eval.Invoke (null, null) .ToString ();
Assert.AreEqual ("42", answer);
Note: at this stage, only part of the language features has been implemented in the current CTP. We are moving forward at a fast pace, but functions such as LINQ queries, attributes, events, dynamic, async are not yet implemented. You can visit the Roslyn forums to view a complete list of things not implemented .
Although not all language features are supported, the public API form is more populated. Therefore, we advise you to write extensions and utilities using the Syntax, Symbols, and Flow and Region Analysis APIs.
We are very pleased to offer you an early preview of this technology and look forward to feedback, ideas and suggestions. Use the forums to ask questions and provide feedback, Microsoft Connectto register errors and suggestions. You can also use the hashtag #RoslynCTP on Twitter.