
PLO - Palestine Liberation Organization
This article is a written statement of my personal perception of programming and Object-oriented programming in particular. Here are collected and emotional indignation, and worries for programmers around the world. Everything, of course, is backed up by source code.

How did I find out about programming?
- From 9 to 11 grade at school taught to program in Pascal.
What did my programs consist of?
- Of the procedures and functions, data and actions on them.
What didn’t you like?
- When I tried to write a large program, I struggled with errors for a very long time ... And the further the more they were ... I had to keep in mind a huge amount of information on how everything works. Who causes whom and where.
How did I deal with complexity?
- I highlighted independent modules in which I combined functions according to their semantic purpose. Separately functions for working with the mouse, separately for working with graphics, separately for working with BMP, etc.
How did you find out about OOP?
- In the first year at the institute.
What exactly did they tell me about OOP?
- It's hard to remember. I remember classes, inheritance, private , public and static .
How did I use the acquired knowledge?
“Well, I created classes as a reflection of real-world objects.” Inherited to extend the functionality of a certain class.
How has OOP helped me, has it become easier to write programs?
- At first it was convenient. It was possible to reuse old classes. Sometimes, if the requirements changed - I was inherited and changed the behavior in the heir as necessary.
What are the principles of SOLID?
What is a class interface and why explicitly describe it?
What is the relation general / particular and part / whole?
If you gave a positive answer to the two previous questions, you can no longer read. Further in the article, we will talk about interfaces, briefly describe the principles of SOLID and give 2 main types of relations between objects in an object-oriented program.
Let's look at the code for the Foo class:
We see several types of information:
1. Class information
- class fields: b
- class methods: b ()
2. Class instance information
- class instance fields: a
- class instance methods: a (), sum1 (), sum2 ( )
- constructor of the class instance: Foo ()
- destructor of the class instance: ~ Foo ()
So the class interface is the following data:
- class instance methods: a (), sum1 (), sum2 ()
What to do to explicitly describe the interface in C ++?
- The C ++ language has small problems with this (the syntax is poor)
Lord, what is it?
- Virtual destructor : ~ Foo ().
- Purely virtual methods: a (), sum1 (), sum2 ().
And now what to do with this IFoo?
- Inherit from him class Foo.
The Foo class interface is now explicitly described. The Foo class can be called the implementation (implementation) of the IFoo interface . It is necessary to use objects of the Foo class through the IFoo interface as follows:
What does this give us?
- Well, just look at the function:
In the function process can pass any implementation of the interface IFoo . It can be both Foo and SuperFoo and GiperPuperFoo .
In C #, Java, and others, there is an interface keyword, which is used to describe interfaces:
In Microsoft Visual C ++ has a keyword __interface , read more here .
“SOLID is an abbreviation of the five basic principles of class design in object-oriented design.” Wikipedia
I will try to give a brief description of each of them.
The principle of asingle division of responsibility states that each object in the program should have a single responsibility. If the object performs many different duties - it must be sawn. For example, the report printing entity is responsible for the format and content of the reports — this is incorrect. One object must be responsible for the format, another - for the content.
The principle of openness / closenessrequires classes, modules, functions to be open for extension, but closed for change. The bottom line is that once created classes / functions cannot be changed for the specific needs of a particular project. Allowed only to correct errors in them - nothing more. To change the behavior of a certain class / function, it is necessary to explicitly describe its interface and create another implementation of this interface.
The principle of Liskov substitution in general suggests that inheritance is correctly used to create program hierarchies of the general-private type , but not in some cases not a part-whole . Example: the wheels of a car are a part-whole, and the “wheel of a passenger car” relative to the generalized essence of “wheel” is a general-private relation.
The principle of interface isolation states that it is more correct to use many specialized interfaces than to group methods that are completely unrelated to each other into common interfaces.
The principle of dependency inversion is necessary to ensure replaceability of objects without corrections throughout the code and to weaken dependencies in the code. When you have a class A that has a pointer to a class B - the classes are considered strongly related. To replace class B with any other, you will have to correct the code of class A - which is not good. It is proposed to derive a class B interface, let's call it IB. Change pointer type in class A to IB. Thus dependency A -> B replaced by A -> IB <- Bed and . Now you can use any other implementation of the IB interface instead of B.
An object-oriented program consists of classes. In the process of the program, instances of classes are created, interact with other instances and end their existence. Interacting with self-similar classes form two types of hierarchies:
- Part / whole
relationship hierarchy - General / particular
relationship hierarchy What does the general / particular relationship hierarchy look like?
- Hierarchy of types of land transport:

- Hierarchy of types of wheels:
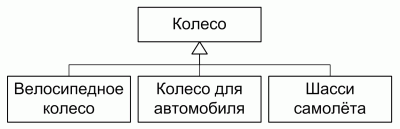
What does the part / whole relationship hierarchy look like?
- Passenger car hierarchy:
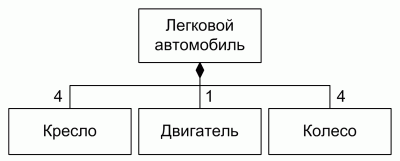
When designing a system, it is necessary to define hierarchies of both types.
OOP was created to "reduce the complexity of the program!" When used correctly, your programs can become extremely simple in their internal structure. You, as a programmer, simply must be lazy)) So here is a way to lower the complexity of your programs, reduce connectivity, etc.
PS In the bottom of the J2ME programming books, the chapter on the PLO included the phrase: “If for you the PLO is the Organization for the Liberation of Palestine, refer to another more fundamental book.” Now this phrase is associated with my misunderstanding of OOP.

What is programming?
How did I find out about programming?
- From 9 to 11 grade at school taught to program in Pascal.
What did my programs consist of?
- Of the procedures and functions, data and actions on them.
What didn’t you like?
- When I tried to write a large program, I struggled with errors for a very long time ... And the further the more they were ... I had to keep in mind a huge amount of information on how everything works. Who causes whom and where.
How did I deal with complexity?
- I highlighted independent modules in which I combined functions according to their semantic purpose. Separately functions for working with the mouse, separately for working with graphics, separately for working with BMP, etc.
What is OOP?
How did you find out about OOP?
- In the first year at the institute.
What exactly did they tell me about OOP?
- It's hard to remember. I remember classes, inheritance, private , public and static .
How did I use the acquired knowledge?
“Well, I created classes as a reflection of real-world objects.” Inherited to extend the functionality of a certain class.
How has OOP helped me, has it become easier to write programs?
- At first it was convenient. It was possible to reuse old classes. Sometimes, if the requirements changed - I was inherited and changed the behavior in the heir as necessary.
What are the principles of SOLID?
What is a class interface and why explicitly describe it?
What is the relation general / particular and part / whole?
If you gave a positive answer to the two previous questions, you can no longer read. Further in the article, we will talk about interfaces, briefly describe the principles of SOLID and give 2 main types of relations between objects in an object-oriented program.
What is an interface?
Let's look at the code for the Foo class:
class Foo
{
int a;
static int b;
public:
Foo() { a = 0; }
~Foo() { a = 0; }
int a() { return a; }
static int b() { return b; }
int sum1() { return a+b; }
int sum2() { return a()+b(); }
};
We see several types of information:
1. Class information
- class fields: b
- class methods: b ()
2. Class instance information
- class instance fields: a
- class instance methods: a (), sum1 (), sum2 ( )
- constructor of the class instance: Foo ()
- destructor of the class instance: ~ Foo ()
So the class interface is the following data:
- class instance methods: a (), sum1 (), sum2 ()
What to do to explicitly describe the interface in C ++?
- The C ++ language has small problems with this (the syntax is poor)
class IFoo
{
public:
virtual ~IFoo() {}
virtual int a() = 0;
virtual int sum1() = 0;
virtual int sum2() = 0;
};
Lord, what is it?
- Virtual destructor : ~ Foo ().
- Purely virtual methods: a (), sum1 (), sum2 ().
And now what to do with this IFoo?
- Inherit from him class Foo.
class Foo : public IFoo
{
int a;
static int b;
public:
Foo() { a = 0; }
~Foo() { a = 0; }
int a() { return a; }
static b() { return b; }
int sum1() { return a+b; }
int sum2() { return a()+b(); }
};
The Foo class interface is now explicitly described. The Foo class can be called the implementation (implementation) of the IFoo interface . It is necessary to use objects of the Foo class through the IFoo interface as follows:
IFoo * foo = new Foo();
int a = foo->a();
int sum1 = foo->sum1();
int sum2 = foo->sum2();
What does this give us?
- Well, just look at the function:
void process(IFoo * foo)
{
// ...
}
In the function process can pass any implementation of the interface IFoo . It can be both Foo and SuperFoo and GiperPuperFoo .
In C #, Java, and others, there is an interface keyword, which is used to describe interfaces:
interface IFoo
{
public int a();
public int sum1();
public int sum2();
};
In Microsoft Visual C ++ has a keyword __interface , read more here .
What is SOLID?
“SOLID is an abbreviation of the five basic principles of class design in object-oriented design.” Wikipedia
S | Srp | Single responsibility principle | The principle of a |
O | Opc | Open / closed principle | The principle of openness / closeness |
L | Lsp | Liskov Substitution Principle | Lisk substitution principle |
I | ISP | Interface segregation principle | Interface isolation principle |
D | Dip | Dependency Inversion Principle | Dependency Inversion Principle |
The principle of a
The principle of openness / closenessrequires classes, modules, functions to be open for extension, but closed for change. The bottom line is that once created classes / functions cannot be changed for the specific needs of a particular project. Allowed only to correct errors in them - nothing more. To change the behavior of a certain class / function, it is necessary to explicitly describe its interface and create another implementation of this interface.
The principle of Liskov substitution in general suggests that inheritance is correctly used to create program hierarchies of the general-private type , but not in some cases not a part-whole . Example: the wheels of a car are a part-whole, and the “wheel of a passenger car” relative to the generalized essence of “wheel” is a general-private relation.
The principle of interface isolation states that it is more correct to use many specialized interfaces than to group methods that are completely unrelated to each other into common interfaces.
The principle of dependency inversion is necessary to ensure replaceability of objects without corrections throughout the code and to weaken dependencies in the code. When you have a class A that has a pointer to a class B - the classes are considered strongly related. To replace class B with any other, you will have to correct the code of class A - which is not good. It is proposed to derive a class B interface, let's call it IB. Change pointer type in class A to IB. Thus dependency A -> B replaced by A -> IB <- Bed and . Now you can use any other implementation of the IB interface instead of B.
What is object oriented design?
An object-oriented program consists of classes. In the process of the program, instances of classes are created, interact with other instances and end their existence. Interacting with self-similar classes form two types of hierarchies:
- Part / whole
relationship hierarchy - General / particular
relationship hierarchy What does the general / particular relationship hierarchy look like?
- Hierarchy of types of land transport:

- Hierarchy of types of wheels:
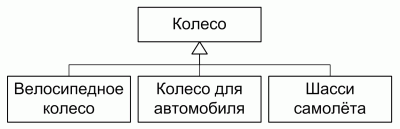
What does the part / whole relationship hierarchy look like?
- Passenger car hierarchy:
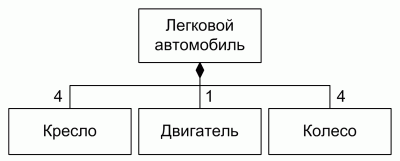
When designing a system, it is necessary to define hierarchies of both types.
Why is this necessary if everything works like that?
OOP was created to "reduce the complexity of the program!" When used correctly, your programs can become extremely simple in their internal structure. You, as a programmer, simply must be lazy)) So here is a way to lower the complexity of your programs, reduce connectivity, etc.
Recommended Reading
- Clean code. Robert Martin ( OZON.ru )
- Refactoring Improving existing code. Martin Fowler ( OZON.ru )
- Object-oriented analysis and design with sample applications. Grady Butch ( OZON.ru )
- Receptions of object-oriented design. Design patterns. E. Gamma, R. Helm, R. Johnson, J. Vlissides ( OZON.ru )
PS In the bottom of the J2ME programming books, the chapter on the PLO included the phrase: “If for you the PLO is the Organization for the Liberation of Palestine, refer to another more fundamental book.” Now this phrase is associated with my misunderstanding of OOP.