
Android + Arduino = ♥
- Tutorial
Not so long ago, Google opened an API for working Android devices with USB. Today it's time to feel what it is and find out what opportunities are opening up for us.

So, we have Arduino Mega 2560, USB Host Shield and HTC Desire with cyanogen 7.1.0 RC1 firmware (Android 2.3.4). Just in case, I’ll remind you that everything that will be described later works only starting with Android 2.3.4 for phones and Android 3.1 for tablets.
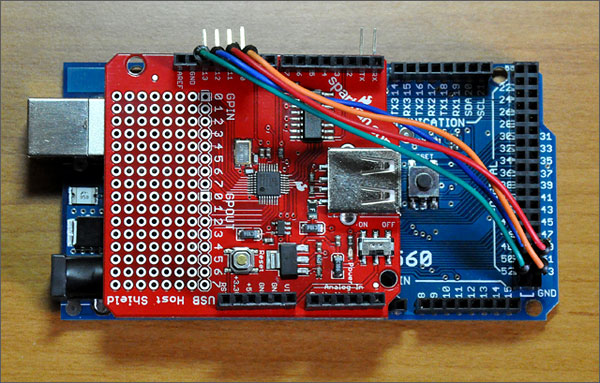
The board with the thrown SPI contacts
1. If not already installed, then download and install the software for Arduino .
2. Download and unzip the ADK package (contains the DemoKit application). Folder should appear
3. Download the CapSense library
4. Copy
5. Create a CapSense directory in
Google kindly provides its DemoKit sketch for Arduino. All that is needed is to open it from
The DemoKit package also contains the source code of the Android application to demonstrate the capabilities. Google offers us to independently create an Android project and build this application. First we need to install API Level 10 . Then everything is simple - we create an Android project and specify the path to the folder
Just connect our phone to the USB Host Shield. If we did everything correctly, then the screen will ask you to launch the DemoKit application. The application itself contains two tabs - In (buttons, joystick and sensors) and Out (LEDs, relays and servos). I decided that a pair of LEDs and a button are enough to demonstrate. How all this miracle works can be observed on video.
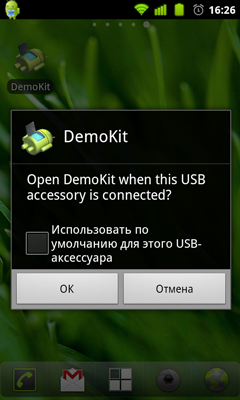


In this example, messages transmitted via USB consist of three bytes:
1st byte defines a command or group of devices, for example LEDs - 0x2
2nd byte indicates a specific device, for example green LED - 0x1
3rd byte contains the value transmitted device, for example, maximum brightness - 0xff
Discovering such opportunities, Google in the first place, of course, expects a large number of active Android accessories to appear, but by no means the last place is taken by the fact that in fact we get a convenient device for interacting with various sensors, sensors and drives. Such a device can easily become the brain of something robotic.
Also, we must not forget that the Android device for Arduino can act as an expansion card, which has GPS, Bluetooth, WiFi, an accelerometer and much more.
1. Android USB Accessory
2. Android Open Accessory Development Kit

So, we have Arduino Mega 2560, USB Host Shield and HTC Desire with cyanogen 7.1.0 RC1 firmware (Android 2.3.4). Just in case, I’ll remind you that everything that will be described later works only starting with Android 2.3.4 for phones and Android 3.1 for tablets.
It is worth noting that this USB Host Shield is not entirely successful, especially in combination with the Arduino Mega 2560. The first problem was that this expansion board was made for Arduino UNO, and it differs from Mega in the positions of the SPI contacts, so I had to throw jumpers (see. a photo). The second problem, although quite expected, was the need for an external power source for this expansion card to work. The USB Host Shield 2.0 from Circuits @ Home is considered more successful , but it is also more expensive.
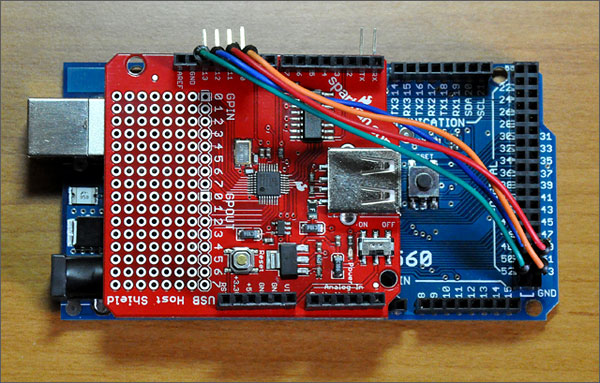
The board with the thrown SPI contacts
Arduino software setup
1. If not already installed, then download and install the software for Arduino .
2. Download and unzip the ADK package (contains the DemoKit application). Folder should appear
app
, firmware
and hardware
. 3. Download the CapSense library
4. Copy
firmware/arduino_libs/AndroidAccessory
and firmware/arduino_libs/USB_Host_Shield
in /libraries/
. 5. Create a CapSense directory in
/libraries/
and copy to CapSense.cpp
and CapSense.h
from the CapSense archive.Firmware Download
Google kindly provides its DemoKit sketch for Arduino. All that is needed is to open it from
firmware/demokit/demokit.pde
, compile and upload it to the board.Android test application
The DemoKit package also contains the source code of the Android application to demonstrate the capabilities. Google offers us to independently create an Android project and build this application. First we need to install API Level 10 . Then everything is simple - we create an Android project and specify the path to the folder
app
, in the Build Target we specify Google APIs (Platform 2.3.3 , API Level 10). We collect the application and install it on the phone. Who does not want to mess with the assembly - can download the finished APK .Launch
Just connect our phone to the USB Host Shield. If we did everything correctly, then the screen will ask you to launch the DemoKit application. The application itself contains two tabs - In (buttons, joystick and sensors) and Out (LEDs, relays and servos). I decided that a pair of LEDs and a button are enough to demonstrate. How all this miracle works can be observed on video.
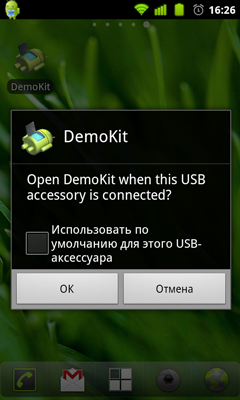


Some code
In this example, messages transmitted via USB consist of three bytes:
1st byte defines a command or group of devices, for example LEDs - 0x2
2nd byte indicates a specific device, for example green LED - 0x1
3rd byte contains the value transmitted device, for example, maximum brightness - 0xff
Arduino
...
/* инициализация */
AndroidAccessory acc("Google, Inc.",
"DemoKit",
"DemoKit Arduino Board",
"1.0",
"http://www.android.com",
"0000000012345678");
void setup()
{
....
acc.powerOn();
}
void loop()
{
byte msg[3];
/* проверка подключения */
if (acc.isConnected()) {
/* получение сообщения от Android устройства */
int len = acc.read(msg, sizeof(msg), 1);
if (len > 0) {
/* сообщение для светодиодов */
if (msg[0] == 0x2) {
if (msg[1] == 0x0)
analogWrite(LED3_RED, msg[2]);
else if (msg[1] == 0x1)
analogWrite(LED3_GREEN, msg[2]);
else if (msg[1] == 0x2)
analogWrite(LED3_BLUE, msg[2]);
}
}
msg[0] = 0x1;
b = digitalRead(BUTTON1);
if (b != b1) {
msg[1] = 0;
msg[2] = b ? 1 : 0;
/* отправка состояния кнопки */
acc.write(msg, 3);
b1 = b;
}
}
}
Android
import com.android.future.usb.UsbAccessory;
import com.android.future.usb.UsbManager;
...
public class DemoKitActivity extends Activity implements Runnable {
private UsbManager mUsbManager;
UsbAccessory mAccessory;
FileInputStream mInputStream;
FileOutputStream mOutputStream;
...
private void openAccessory(UsbAccessory accessory) {
mFileDescriptor = mUsbManager.openAccessory(accessory);
if (mFileDescriptor != null) {
mAccessory = accessory;
FileDescriptor fd = mFileDescriptor.getFileDescriptor();
mInputStream = new FileInputStream(fd);
mOutputStream = new FileOutputStream(fd);
Thread thread = new Thread(null, this, "AccessoryThread");
thread.start();
}
}
public void run() {
int ret = 0;
byte[] buffer = new byte[16384];
int i;
while (ret >= 0) {
// получение входящих сообщений
ret = mInputStream.read(buffer);
i = 0;
while (i < ret) {
int len = ret - i;
switch (buffer[i]) {
case 0x1: // сообщение от кнопки
if (len >= 3) {
Message m = Message.obtain(mHandler, MESSAGE_SWITCH);
m.obj = new SwitchMsg(buffer[i + 1], buffer[i + 2]);
mHandler.sendMessage(m);
}
i += 3;
break;
}
}
}
}
// пример использования - включить красный светодиод на полную яркость:
// mActivity.sendCommand((byte)2, (byte)0, (byte)255)
public void sendCommand(byte command, byte target, int value) {
byte[] buffer = new byte[3];
if (value > 255)
value = 255;
buffer[0] = command;
buffer[1] = target;
buffer[2] = (byte) value;
if (mOutputStream != null && buffer[1] != -1) {
try {
mOutputStream.write(buffer);
} catch (IOException e) {
...
}
}
}
}
conclusions
Discovering such opportunities, Google in the first place, of course, expects a large number of active Android accessories to appear, but by no means the last place is taken by the fact that in fact we get a convenient device for interacting with various sensors, sensors and drives. Such a device can easily become the brain of something robotic.
Also, we must not forget that the Android device for Arduino can act as an expansion card, which has GPS, Bluetooth, WiFi, an accelerometer and much more.
References
1. Android USB Accessory
2. Android Open Accessory Development Kit