
Classes are objects
Those who study Ruby know that all entities in it are objects, but sometimes they don’t know how much. So, classes in Ruby are also objects.
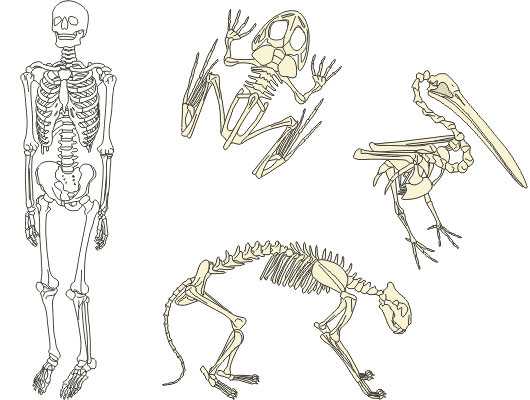
In Ruby, classes are objects that are instances of the Class class.
A class is a type that describes the structure of objects.
An object is an entity in the address space of a computer that appears when an instance of a class is created.
In the future, sometimes I will use the term for convenience instance (instance) for the facility
and the term type to class .
Since each described class has various properties and executable code, they need to be stored somewhere. Where does Ruby store data? That's right, in objects. And it is logical that objects describing a certain class must have their own type. Indeed, the type (class) of objects describing classes is Class .
The mechanism for creating a class in Ruby can be understood in such an unpopular way.
We have now created an absolutely normal, but anonymous class (the name method returns an empty string) and
placed it in the myklass variable . What is myklass ? This is the object in which the description of our class is stored; the class of this object is Class .
Our anonymous class, all its methods and variables exist only in the myklass object .
An instance of an anonymous class (that is, its object) is created just like for any other:
An anonymous class can be inherited in exactly the same way as a non-anonymous class.
There's a bit of magic from Ruby. The name of the class appears at the moment when the object describing the class is assigned to the Constant.
As you know, in Ruby, constants start with a capital letter, hence the names of the classes.
Please note MyKlass is not a class name, it is a constant that stores an object describing the class. But at the moment when we assigned it to this constant, our class got a name that matches the name of the constant.
In general, the class name is often just informative in nature. After all, you use the class not by name, but by using the same variable or Constant in which it is stored.
The Class type , like the other classes, is inherited from the Object type . But, after this:
figure out what comes first - Object or Class is impossible. How can a Class be a constant that stores the object of the class that it is an instance of? In fact, Object , Class, and Module are the primary structures embedded in the interpreter, and they are not formed from each other by ruby code, although Ruby successfully pretends to be so.
In fact, there are no class methods in Ruby (which we think we describe through def self.aaa ). There are only methods of objects, including objects of type Class.
But more on that next Thursday ...
rhg.rubyforge.org/chapter02.html
rbdev.ru/2010/07/izuchaem-yadro-ruby-klassy-i-obekty
stackoverflow.com/questions/1219691/why-object-class-class-in-ruby
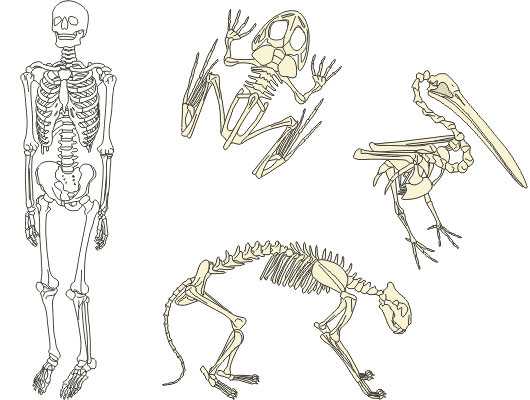
In Ruby, classes are objects that are instances of the Class class.
OOP Reminder
A class is a type that describes the structure of objects.
An object is an entity in the address space of a computer that appears when an instance of a class is created.
In the future, sometimes I will use the term for convenience instance (instance) for the facility
and the term type to class .
Class properties need to be stored somewhere.
Since each described class has various properties and executable code, they need to be stored somewhere. Where does Ruby store data? That's right, in objects. And it is logical that objects describing a certain class must have their own type. Indeed, the type (class) of objects describing classes is Class .
Anonymous class
The mechanism for creating a class in Ruby can be understood in such an unpopular way.
myklass = Class.new do
def test
puts "TEST"
end
def self.ctest
puts "CLASS TEST"
end
end
myklass # => #
myklass.class # => Class
myklass.name # => ""
myklass.ctest # => "CLASS TEST"
We have now created an absolutely normal, but anonymous class (the name method returns an empty string) and
placed it in the myklass variable . What is myklass ? This is the object in which the description of our class is stored; the class of this object is Class .
Our anonymous class, all its methods and variables exist only in the myklass object .
An instance of an anonymous class
An instance of an anonymous class (that is, its object) is created just like for any other:
m = myklass.new
m.test # => 'TEST'
m.class # => #
m.class==myklass # => true
Inheriting an Anonymous Class
An anonymous class can be inherited in exactly the same way as a non-anonymous class.
class MyKlass2 < myklass
# ...
end
MyKlass2.superclass == myklass # => true
# или опять анонимно:
mk2 = Class.new myklass do
# ..
end
mk2.superclass == myklass
mk2.name # => ""
Call me by name
There's a bit of magic from Ruby. The name of the class appears at the moment when the object describing the class is assigned to the Constant.
As you know, in Ruby, constants start with a capital letter, hence the names of the classes.
MyKlass = myklass
MyKlass # => 'MyKlass'
MyKlass.name # => 'MyKlass'
myklass.name # => 'MyKlass'
Please note MyKlass is not a class name, it is a constant that stores an object describing the class. But at the moment when we assigned it to this constant, our class got a name that matches the name of the constant.
In general, the class name is often just informative in nature. After all, you use the class not by name, but by using the same variable or Constant in which it is stored.
A chicken or an egg?
The Class type , like the other classes, is inherited from the Object type . But, after this:
Object.class # => Class
Class.class # => Class
Class.superclass # => Module
Module.class # => Class
Module.superclass # => Object
figure out what comes first - Object or Class is impossible. How can a Class be a constant that stores the object of the class that it is an instance of? In fact, Object , Class, and Module are the primary structures embedded in the interpreter, and they are not formed from each other by ruby code, although Ruby successfully pretends to be so.
Interesting fact.
In fact, there are no class methods in Ruby (which we think we describe through def self.aaa ). There are only methods of objects, including objects of type Class.
But more on that next Thursday ...
Related Links
rhg.rubyforge.org/chapter02.html
rbdev.ru/2010/07/izuchaem-yadro-ruby-klassy-i-obekty
stackoverflow.com/questions/1219691/why-object-class-class-in-ruby