
Google AI Challenge - Quick Start
- Tutorial
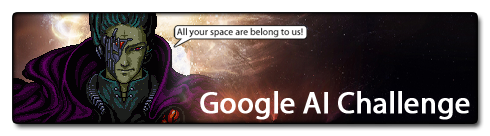
1. We get into the essence
1.1. Gameplay from a bot perspective
First, I’ll tell you how the interaction of bots and the verification system (checker) works. The tournament organizers decided that the best way to arrange this interaction is to declare each robot a separate process, and communicate with it using standard input and output. We will not discuss whether this is a good decision or not, but just take it for granted. The game, in contrast to how it may seem, is turn-based. For each turn, the bot receives a description of the current state of the game on stdin. It includes:
- Description of all the planets on the map; for each planet the following is known:
- Unique ID of the planet
- Its Cartesian coordinates
- ID of the owner of the planet ( NB: if the planet is neutral, then the ID is zero , if it belongs to the player, then the ID is one )
- The number of ships on the planet
- Ship Growth Rate
- Description of all fleets in flight:
- Fleet Owner ID
- The number of ships in the fleet
- ID of the planet the fleet is flying from
- ID of the planet the fleet is flying to
- How many more moves the fleet will fly ( NB: The number of moves the fleet will spend moving from planet A to planet B is equal to the Euclidean distance rounded up between A and B )
In response to this, the bot writes a sequence of commands to stdout, and then says that it has finished its turn. The team has only one: send X ships from planet A to planet Bed and . Please note that if planet A does not belong to you, or planet A or B does not exist, or there are less than X ships on planet A or X is less than zero, then you will be disqualified from the match.
1.2. How is the rating considered
2. Getting down to business
2.1. registration
There is nothing special to say, except that it is impossible to have several accounts and that it is also impossible to change them, even the password. If you want to play in a team, you need to indicate this in the biography immediately.
2.2. Recognizing the starter package
For the convenience of the participants, the organizers made special starter packages for the main languages. These packages include a checker, a replay watcher, some simple example bots and a template for creating your own bot. You can download the starter kit for your favorite language from here . I love java, and therefore I will continue to talk on the example of this language. To the delight of those who do not share my passions, everything differs little for other languages.
After you download the .zip archive and unzip it to a place you like, you will see the following folder structure:
java_starter_package/
|-- example_bots/
|-- maps/
|-- tools/
|-- Fleet.java
|-- MyBot.java
|-- Planet.java
|-- PlanetWars.java
In the example_bots folder, as you might guess, there are simple example bots along with the source codes, and in the maps folder, as someone suggests, there are maps. The tools folder is already more interesting (by the way, its contents are the same for starter kits in all languages): there are two jar files there, without source. The first one is PlayGame.jar, which is a checker. You need to use it like this:
java -jar tools/PlayGame.jar maps/map1.txt 1000 1000 log.txt "java -jar my_bots/trunk/MyBot.jar" "java -jar example_bots/ProspectorBot.jar"
Just in case, I’ll explain: the first argument is the path to the map, the second argument is the maximum length of the move in milliseconds ( yes, the move is limited in time : if one of the bots thinks too long, it will automatically lose ), the third argument is the maximum number of moves. Next in the arguments is the name of the file for the logs, the command that you need to execute to start the first bot, and the command for the second. In principle, the engine supports a larger number of players; you can also specify them on the command line. However, in a tournament all matches are held face to face.
The second file in the tools folder is ShowGame.jar, which renders the match, the description of which he needs to submit to standard input. This is done specifically so that you can transfer the output of the checker directly to the visualizer:
java -jar tools/PlayGame.jar params | java -jar tools/ShowGame.jar
Next, the root * .java files remain. The purpose of Fleet.java and Planet.java is obvious - they contain classes that describe the fleet and planet, respectively. The PlanetWars class is already more substantial: it can parse what the checker sends to the bot on stdin and present the result in a more or less user-friendly way. The interface (which, however, is not explicitly indicated) is something like this:
public interface IPlanetWars {
int NumPlanets();
Planet GetPlanet(int planetID);
int NumFleets();
Fleet GetFleet(int fleetID);
List Planets();
List Fleets();
int Distance(int sourcePlanet, int destinationPlanet);
void IssueOrder(int sourcePlanet, int destinationPlanet, int numShips);
void FinishTurn();
}
I said “about” because I provided only the main part of the interface necessary for understanding the mechanism of interaction; actually a few more methods are available, but they are for the most part already for convenience. By the way, given how little code corresponds to Java Coding Conventions, it is highly likely that it was semi-automatically translated from language to language, and therefore did not differ between them at all.
All files that were reviewed earlier, it is not necessary to leave. You can safely change them (by the way, I highly recommend: they are written from the point of view of user convenience) or even delete. But the file MyBot.java cannot be deleted, and the main method must also be in it, since it will be called by the checker. All the logic in the example is in the DoTurn method, which has an instance of the PlanetWars class at the input.
The code in the starter package is more or less decently commented, so you can easily figure out how to use it and write your first simple bot. I just want to say that it is not allowed to create streams and write to a file.
2.3. Submit a solution
To send a solution, you need to take all (all!) Source (!) Files that are needed for your bot to compile, pack them in a zip archive, and download them using this link . Please note that the size of the archive should not exceed two megabytes. Although you, of course, are unlikely to write so much code;). After some time, you will receive a letter in the mail in which the Compilation Script will share with you the result of its work. If everything goes well, the text will be something like this:
This is just to let you know that your latest submissions to the Google AI Challenge has successfully compiled. Here is the output of the compile script, in case you're curious:
Found entry.zip. Attempting to unpack.unzip -u entry.zip > /dev/null 2> /dev/null
Found MyBot.java. Compiling this entry as Javajavac Bot.java Fleet.java MyBot.java Planet.java PlanetWars.java SimpleBot.java jar cfe MyBot.jar MyBot Bot.class PlanetWars.class Planet.class Fleet.class MyBot.class SimpleBot$1.class SimpleBot.class
Sincerely,
The Compile Script
Some time after that, a note will appear in your personal account that you sent the bot:
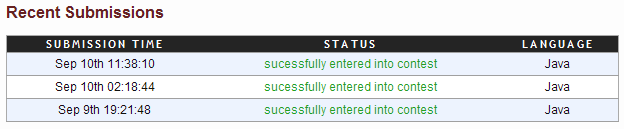
And after some time your brainchild will begin to valiantly fight with the other bots:

Each such battle can be viewed by clicking on the “View Game” link on right. You can see your rating either in the Current Rankings section , or in your profile.
I also want to add that you should not send bots immediately, it is much more advisable to test it first on your own on different cards. To do this, local craftsmen (including your humble servant) created a script that automatically runs the bot on all cards and all bots from the examples (and your other bots, if any) and gives statistics on victories. You can take this script here .
That's all. I will be glad to questions, clarifications and corrections.
Bloody battles and sweet dreams! ©
UPD: Sadly, the competition site is. For sufferers, I posted in this comment links to scripts for testing bots. The starter package for Java can be downloaded here . If someone shares links to starter kits for other languages, it will be cool! ;)
UPD2: Raised, but so far partially. They promise to fix everything by the end of the weekend. By the way, now the contest has become fully open !