
Small PHP-GTK program

PHP-GTK is a toolkit binding for creating the GTK + GUI to the PHP programming language . About it already wrote on Habré . Many people are skeptical of this library, not even so much to it as to the use of PHP on the desktop. But, one way or another, the library exists and, moreover, develops, as evidenced by recent news on the official website . Therefore, I propose to get acquainted with PHP-GTK in more detail and write a small program.
The program creates a window and displays a text widget in it containing an integer (by default, 0), the “Increase” button, when clicked, the number will be increased by one, and the “Reset” button, resetting the value of the text widget to zero.
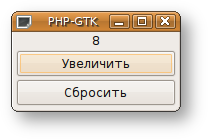
Creating a base window
The window is created using the constructor of the GtkWindow () class . To determine the position occupied by the window after creation, use the method set_position () , set_title () sets the header and set_size_request () - window size (-1 indicates that the height will be chosen automatically based on the size of the content). When the window is closed, the signal 'destroy' is emitted. At this point, to correctly complete the program, you must call Gtk :: main_quit (), for this the connect_simple () method is used .
$ window = new GtkWindow ();
$ window-> set_position (Gtk :: WIN_POS_CENTER);
$ window-> set_size_request (160, -1);
$ window-> set_title ('PHP-GTK');
$ window-> connect_simple ('destroy', 'Gtk :: main_quit');
Widgets
As mentioned earlier, the program uses one text widget GtkLabel () and two buttons GtkButton () . In order to place them in a window, it is necessary to use a special vertical container GtkVBox () , because GtkWindow () allows you to place only one widget.
$ vbox = new GtkVBox ();
$ label = new GtkLabel ('0');
$ btn_inc = new GtkButton ('Zoom In');
$ btn_cancel = new GtkButton ('Reset');
$ vbox-> pack_start ($ label);
$ vbox-> pack_start ($ btn_inc);
$ vbox-> pack_start ($ btn_cancel);
User interaction
When the buttons are pressed, a 'clicked' signal is emitted, “hearing” which we call the function increment () to increase the number and cancel () to reset the value. In the connect_simple () method, after the function name, there is a list of parameters passed to the called function. In this case, we pass the GtkLabel () widget to both functions. In order to get the text currently in GtkLabel (), you must use the get_label () method , and to set a new value, set_label () .
$ btn_inc-> connect_simple ('clicked', 'increment', $ label);
$ btn_cancel-> connect_simple ('clicked', 'cancel', $ label);
function increment ($ label)
{
$ int = $ label-> get_text ();
$ int ++;
$ label-> set_text ($ int);
}
function cancel ($ label)
{
$ label-> set_text ('0');
}
Now all that remains is to add the container to the window and display the window on the screen. Full program code:
$ window = new GtkWindow ();
$ window-> set_position (Gtk :: WIN_POS_CENTER);
$ window-> set_size_request (160, -1);
$ window-> set_title ('PHP-GTK');
$ window-> connect_simple ('destroy', 'Gtk :: main_quit');
$ vbox = new GtkVBox ();
$ label = new GtkLabel ('0');
$ btn_inc = new GtkButton ('Zoom In');
$ btn_cancel = new GtkButton ('Reset');
$ vbox-> pack_start ($ label);
$ vbox-> pack_start ($ btn_inc);
$ vbox-> pack_start ($ btn_cancel);
$ btn_inc-> connect_simple ('clicked', 'increment', $ label);
$ btn_cancel-> connect_simple ('clicked', 'cancel', $ label);
function increment ($ label)
{
$ int = $ label-> get_text ();
$ int ++;
$ label-> set_text ($ int);
}
function cancel ($ label)
{
$ label-> set_text ('0');
}
$ window-> add ($ vbox);
$ window-> show_all ();
Gtk :: main ();
?>
Official site PHP-GTK
Manual