
3 in 1: Desktop, Mobile, Web. Cross-platform development

Development within the framework of one project has a number of advantages. Firstly, it allows you to use one implementation of the business logic of the program. Secondly, it is an opportunity to have a single set of unit tests. Thirdly, this is the use of the familiar language (C ++) and the development environment.
The article describes some programming methods and several libraries that help to create cross-platform applications.
Based on the experience of creating a small application such as a "calculator"
Cross-platform development
It is convenient to write one code instead of three, this significantly reduces the time it takes to create a program. But unfortunately each platform has its own dependent code (functionality). Often, the functionality provided by a particular platform is simply indispensable. A possible solution to this problem is design strategies (for more details, see Alexandrescu's book “Modern Design in C ++”). All dependent code is placed in the configuration classes, the so-called “policies” , which later configure the larger classes.The use of cross-platform libraries ( boost , stl and others) also simplifies development.
MVC
Using the MVC model greatly simplifies application design, as most platform dependencies are localized in the View component.Htmlayout
To organize the graphical interface, the Htmlayout library was used , which allows you to describe the graphical interface of the program using html and css . The implementation of the GUI using web-based tools carries a lot of positive aspects, starting with a large number of web designers and ending with a huge number of ready-made design options. And having “web” as one of the target platforms, this reduces the cost of implementing the design in the form of html + css .Htmlayout has an implementation for Windows Mobile, which allows you to use it also for a mobile platform.
Program calculator
A program like “calculator” was chosen because it allows you to fully try to implement the MVC model and involves the use of some components of the “boost” library that were interesting to me.Desktop
The implementation of the application for the desktop (my native platform), primarily involved writing data models used in the program and implementing the general architecture of the program. A new point for me was the use of the Htmlayout library . However, there were no problems with the implementation of the graphical interface with its help.The code for the main.cpp file in which the configuration and instantiation of the main classes takes place:
#include"stdafx.h"
//Calculator includes
#include"RegexNumberCheckModel.h"
#include"CalculatorModel.hpp"
#include"DesktopView.h"
#include"CalculatorController.hpp"
CAppModule _Module;
int APIENTRY WinMain( HINSTANCE hInstance
,HINSTANCE hPrevInstance
,LPSTR lpCmdLine
,int nShowCmd)
{
//MVC concept:
//Model
typedef CalculatorModel MyCalculatorModel;
MyCalculatorModel calcModel;
//View
typedef DesktopView MyCalculatorView;
MyCalculatorView calcView;
//Controller
typedef CalculatorController MyCalculatorController;
MyCalculatorController calcController(calcModel, calcView);
calcView.Run(hInstance);
return 0;
}
Mobile

Mobile version main.cpp code: Minimum changes: CalculatorModel is configured by the NoNumberCheckModel class , and the DesktopView is replaced by MobileView .
#include"stdafx.h"
//Calculator includes
//#include "RegexNumberCheckModel.h"
#include"NoNumberCheckModel.h"
#include"CalculatorModel.hpp"
#include"MobileView.h"
#include"CalculatorController.hpp"
CAppModule _Module;
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE, LPTSTR, int)
{
//MVC concept
//Model
typedef CalculatorModel MyCalculatorModel;
MyCalculatorModel calcModel;
//View
typedef MobileView MyCalculatorView;
MyCalculatorView calcView;
//Controller
typedef CalculatorController MyCalculatorController;
MyCalculatorController calcController(calcModel, calcView);
calcView.Run(hInstance);
return 0;
}
Web
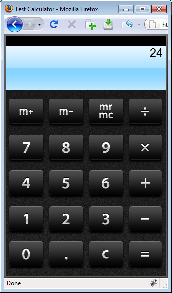
The implementation for the web was a little more complicated, too few articles and examples can be found on the Internet on this subject. The result of a little research was the article "Apache, fastcgi and c ++:" Hello, world " , published a week ago on the hub After choosing the main technology, there were no special problems with the implementation of the interface and the processing of AJAX requests.
The class that implements the "View" component of DesktopView is replaced by FastcgiView .
Conclusion
The right approach to application design allows you to develop programs that can work under most popular platforms without significant effort.And the presence of libraries like htmlayout , boost and stl allows you to do this quickly.
I think such a development technique is quite suitable for programs like torrent clients or smart home control systems ...