
How to turn your Telegram avatar into a watch
- From the sandbox
- Tutorial
I recently sat in a community of programmers in Telegram and noticed one very interesting profile. The following was curious - in the main photo he depicted the current time. I was terribly interested in how he achieved this, and I decided to write the same program at all costs.

Before that, I often worked with Telegram bots using the PyTelegramBotAPI library , so I suggested that such a function is in the bot API. Surely, this was a dumb assumption in my life, because the photo was on the profile picture of the user , not the bot - and soon I became convinced of this, not finding any prerequisites for the ability to change the profile photo.
I started surfing the Internet and came across a rather convenient tool - telethon . He just allowed me to enter Telegram as a user , and not as a bot. Well, I guess it was the very first and biggest step towards the goal. Next, let's look at how to recreate the “clock” on our profile picture.
We follow the link , enter the phone number, after which we get a confirmation code. Read more about this here . So, we get two things that are important to us from there - api_id and api_hash. We create the config.py file and enter the following code there:
Now create the main.py file in which we write:
It is important that main.py and config.py are on the same file level, i.e. in one folder, otherwise the line from .config import * will give an error. The TelegramClient class is exactly what will allow us to log into Telegram as a regular user. Further in the same file we write the following:
Thus, we can assume that we are logged in to Telegram. SESSION NAME you can choose any, at your discretion (for example, "pineapple" ). So, we are connected to the telegram. Now it's time to think about the photos ...
Perhaps this step was the easiest for me, because I have been developing various software that uses computer vision for a long time , and therefore, working with images has become something commonplace. Everything is simple here - we install the opencv library , which allows you to work wonders with photos. Create the utils.py file and write in it:
We need this function so that the time in the format H: MM is indicated on the photo (there were other ways to do this, but I preferred this one).
So, we begin to create the photos themselves. We create the generate_time_images.py file and write in it:
These libraries should be enough for us to create time-stamped photos. Next, we write a function to obtain a black background, on which we will write the time:
Now you will need to go through the cycle for every minute in days and generate a photo indicating the time. Create a time_images folder in advance / where you will save the photos. So, we write:
To create a collection of photos, there is only one thing left to do - run generate_time_images.py . After starting, we see that a lot of photos appeared in the time_images / folder . You should get something like these photos:
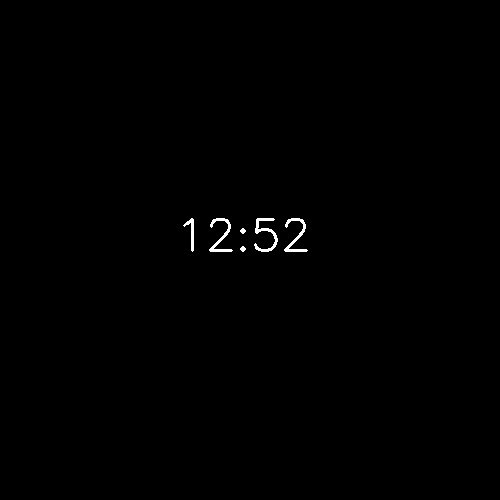
At the telethon there is a very handy thing - called UploadProfilePhotoRequest . We import it into our previously written main.py :
Of course, we should not update the avatar at any time - just do it once a minute. To do this, we must know the moment when the old minute ends and a new one begins - it is at this moment that we will change the photo. We write one more small function in utils.py :
Yes, yes, we pass a string to it with the time of the previous update of the avatar. Of course, there were other ways to implement it, but I wrote it all in a hurry, so I did not think much about optimization. We continue to fill our main.py :
We need DeletePhotosRequest in order to delete previous avatars, so that the effect is created that we do not add a new photo - it changes by itself. In order not to overload the processor, you can add time.sleep (1) to the end of the loop (of course, importing time.py first ).
Done! Run main.py and your avatar will turn into a clock. As a more original option, you can try changing the text on the photos, for example, to this:


Before that, I often worked with Telegram bots using the PyTelegramBotAPI library , so I suggested that such a function is in the bot API. Surely, this was a dumb assumption in my life, because the photo was on the profile picture of the user , not the bot - and soon I became convinced of this, not finding any prerequisites for the ability to change the profile photo.
I started surfing the Internet and came across a rather convenient tool - telethon . He just allowed me to enter Telegram as a user , and not as a bot. Well, I guess it was the very first and biggest step towards the goal. Next, let's look at how to recreate the “clock” on our profile picture.
Step one. Get access to Telegram login from code
We follow the link , enter the phone number, after which we get a confirmation code. Read more about this here . So, we get two things that are important to us from there - api_id and api_hash. We create the config.py file and enter the following code there:
api_id = <ВАШ API_ID>
api_hash = <ВАШ API_HASH>
Now create the main.py file in which we write:
from telethon import TelegramClient, sync
from .config import *
It is important that main.py and config.py are on the same file level, i.e. in one folder, otherwise the line from .config import * will give an error. The TelegramClient class is exactly what will allow us to log into Telegram as a regular user. Further in the same file we write the following:
client = TelegramClient(<ИМЯ СЕССИИ>, api_id, api_hash)
client.start()
Thus, we can assume that we are logged in to Telegram. SESSION NAME you can choose any, at your discretion (for example, "pineapple" ). So, we are connected to the telegram. Now it's time to think about the photos ...
Step Two Create a time source for photos
Perhaps this step was the easiest for me, because I have been developing various software that uses computer vision for a long time , and therefore, working with images has become something commonplace. Everything is simple here - we install the opencv library , which allows you to work wonders with photos. Create the utils.py file and write in it:
def convert_time_to_string(dt):
return f"{dt.hour}:{dt.minute:02}"
We need this function so that the time in the format H: MM is indicated on the photo (there were other ways to do this, but I preferred this one).
So, we begin to create the photos themselves. We create the generate_time_images.py file and write in it:
from .utils import *
import cv2
import numpy as np
from datetime import datetime, timedelta
These libraries should be enough for us to create time-stamped photos. Next, we write a function to obtain a black background, on which we will write the time:
def get_black_background():
return np.zeros((500, 500))
Now you will need to go through the cycle for every minute in days and generate a photo indicating the time. Create a time_images folder in advance / where you will save the photos. So, we write:
start_time = datetime.strptime("2019-01-01", "%Y-%m-%d") # Можете выбрать любую дату
end_time = start_time + timedelta(days=1)
def generate_image_with_text(text):
image = get_black_background()
font = cv2.FONT_HERSHEY_SIMPLEX
cv2.putText(image, text, (int(image.shape[0]*0.35), int(image.shape[1]*0.5)), font, 1.5, (255, 255, 0), 2, cv2.LINE_AA)
return image
while start_time < end_time:
text = convert_time_to_string(start_time)
image = generate_image_with_text(text)
cv2.imwrite(f"time_images/{text}.jpg", image)
start_time += timedelta(minutes=1)
To create a collection of photos, there is only one thing left to do - run generate_time_images.py . After starting, we see that a lot of photos appeared in the time_images / folder . You should get something like these photos:
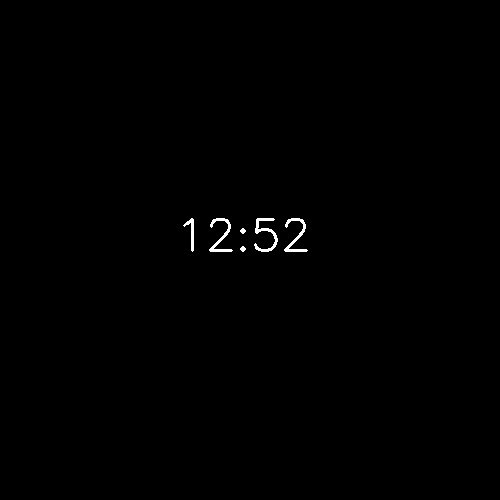
Step Three Updating photos every minute
At the telethon there is a very handy thing - called UploadProfilePhotoRequest . We import it into our previously written main.py :
from telethon.tl.functions.photos import UploadProfilePhotoRequest, DeletePhotosRequest
from datetime import datetime
from .utils import *
Of course, we should not update the avatar at any time - just do it once a minute. To do this, we must know the moment when the old minute ends and a new one begins - it is at this moment that we will change the photo. We write one more small function in utils.py :
def time_has_changed(prev_time):
return convert_time_to_string(datetime.now()) != prev_time
Yes, yes, we pass a string to it with the time of the previous update of the avatar. Of course, there were other ways to implement it, but I wrote it all in a hurry, so I did not think much about optimization. We continue to fill our main.py :
prev_update_time = ""
while True:
if time_has_changed(prev_update_time):
prev_update_time = convert_time_to_string(datetime.now())
client(DeletePhotosRequest(client.get_profile_photos('me')))
file = client.upload_file(f"time_images/{prev_update_time}.jpg")
client(UploadProfilePhotoRequest(file))
We need DeletePhotosRequest in order to delete previous avatars, so that the effect is created that we do not add a new photo - it changes by itself. In order not to overload the processor, you can add time.sleep (1) to the end of the loop (of course, importing time.py first ).
Done! Run main.py and your avatar will turn into a clock. As a more original option, you can try changing the text on the photos, for example, to this:
