10 skills and knowledge necessary for a beginner iOS developer
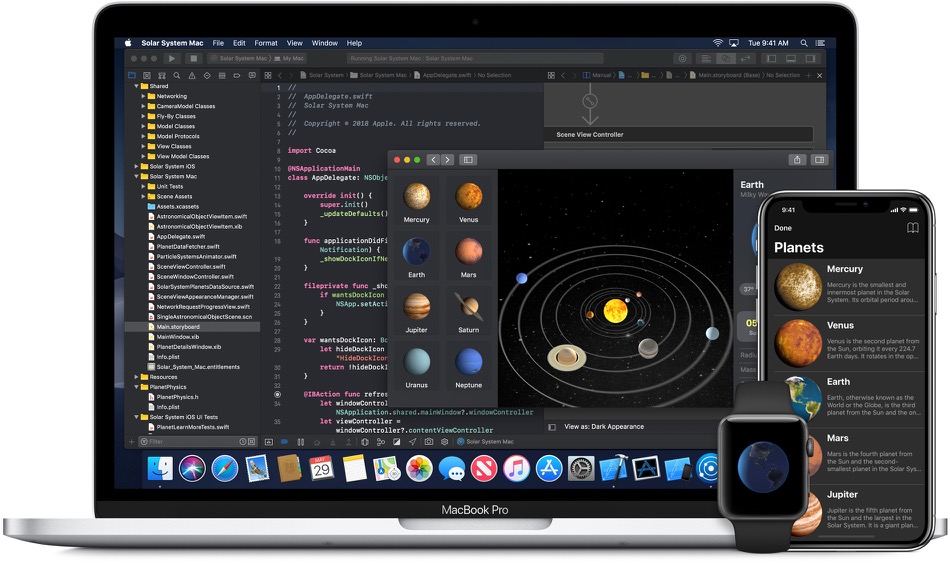
The profession of iOS developer is now quite in demand, well paid, and possibly even fashionable, and therefore attracts many people.
Many candidates come to me and colleagues for interviews for vacancies that are open at our place, and I clearly see many newcomers lacking basic knowledge or simply unsure knowledge of them. Although there are many articles, books and courses on the Internet (both free and paid) from which you can obtain the necessary knowledge, it is not always easy to understand which topics should be concentrated on.
In this article I will share information about what skills and knowledge I consider important for a beginner iOS developer, try to explain why they are needed and give links to materials for their study.
The material is addressed primarily to those who want to start training, fill in the gaps or understand their willingness to work as an iOS developer. I will try to explain everything in simple words.
I must say right away that most of the materials to which I will provide links are in English. I understand that learning English can be harder and slower at the beginning, but it will bear fruit later - you cannot always find good relevant materials in Russian.
First steps
Before delving into the following topics, I recommend preparing the foundation, namely, taking (listening to and reproducing all the assignments) the free CS 193P course from Stanford University and reading the Swift documentation on the Apple website .
It is absolutely normal if you do not understand or remember all the topics, but you will have a basis - structured basic understanding of the device’s iOS applications and Swift language constructs. Taking the time to these things at the start, it will be much easier for you to add knowledge on each topic.
1. Auto Layout, Storyboard, layout from code
Creating a layout-based application interface is a basic skill for an iOS programmer. It’s worth noting not only the main components from which the interface is assembled (UIView, UILabel, UIButton, UIImageView, etc.), but also how to arrange them on the screen to achieve the desired position and size on different sized screens, including when turning the device. For simplicity, I would highlight 3 ways to create an interface:
- visual way through Interface Builder
- fully describe the interface from code
- a combined option, when part of the contents of the screens is entered through Interface Builder, and their settings are moved to the code.
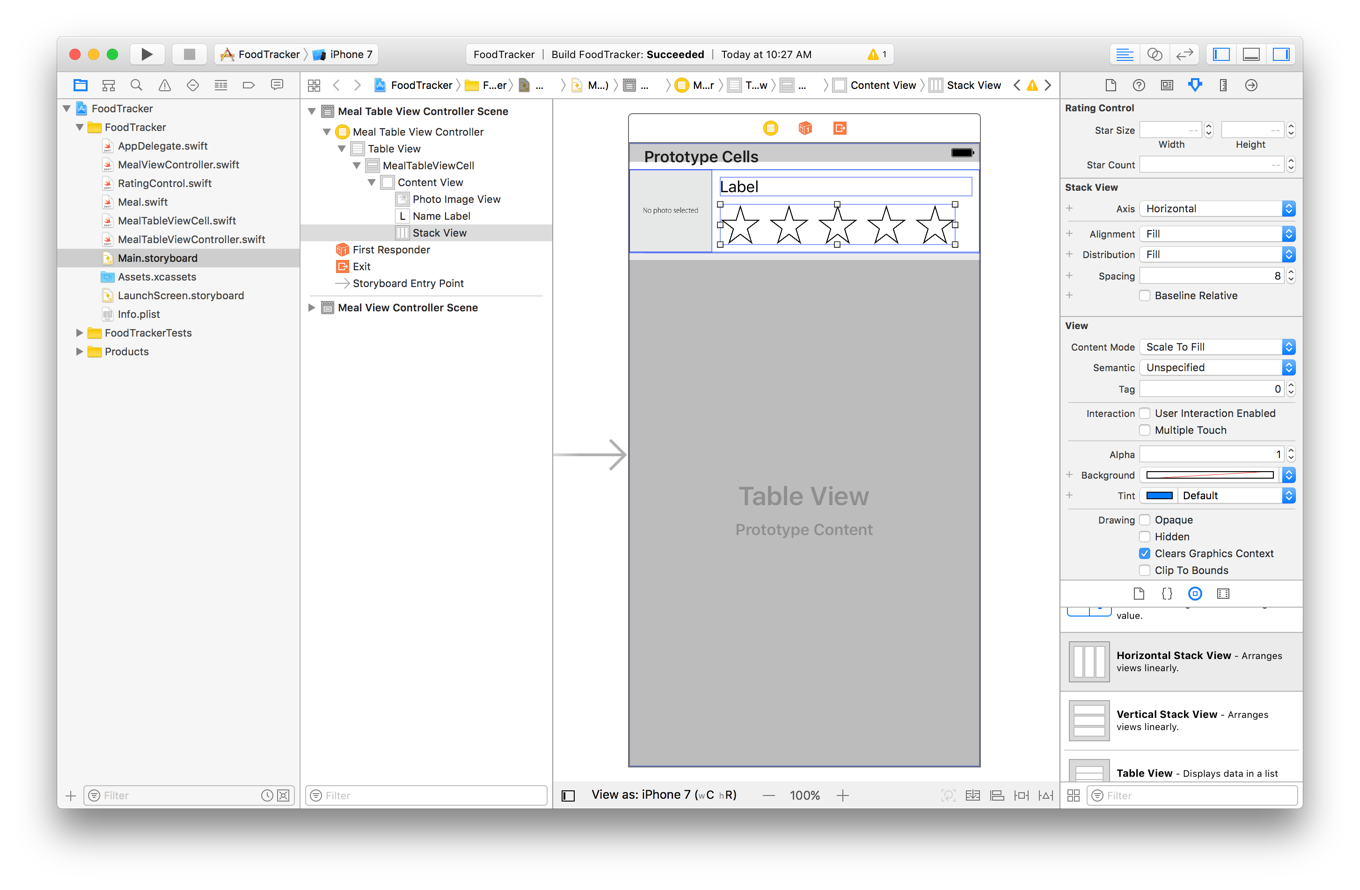
Interface layout skills only through the Interface Builder on the StoryBoard may not be enough for you. In addition to the fact that you can not do everything through StoryBoard, you need to understand that several developers are working on large applications, and a version control system (most often this is Git) is used to combine the results of their work. When several people work on one piece of code, the result of their work is not always possible to combine automatically. If the interface was designed by the programmer from the code, merging is simpler and faster than when the code was generated by Interface Builder.
To master the topic, I recommend:
- take a detailed lesson from Ryan Ackermann and Matthijs Hollemans
- take a free mini course from Brian Voong
- watch two videos in Russian video 1 and video 2 on The Swift Developers channel
How to understand that you have mastered the topic sufficiently? Take any of the apps you use on your iPhone. You can start with standard ones, for example, Apple Music. Try to fully reproduce one or a couple of screens that you see, as well as their behavior when turning.
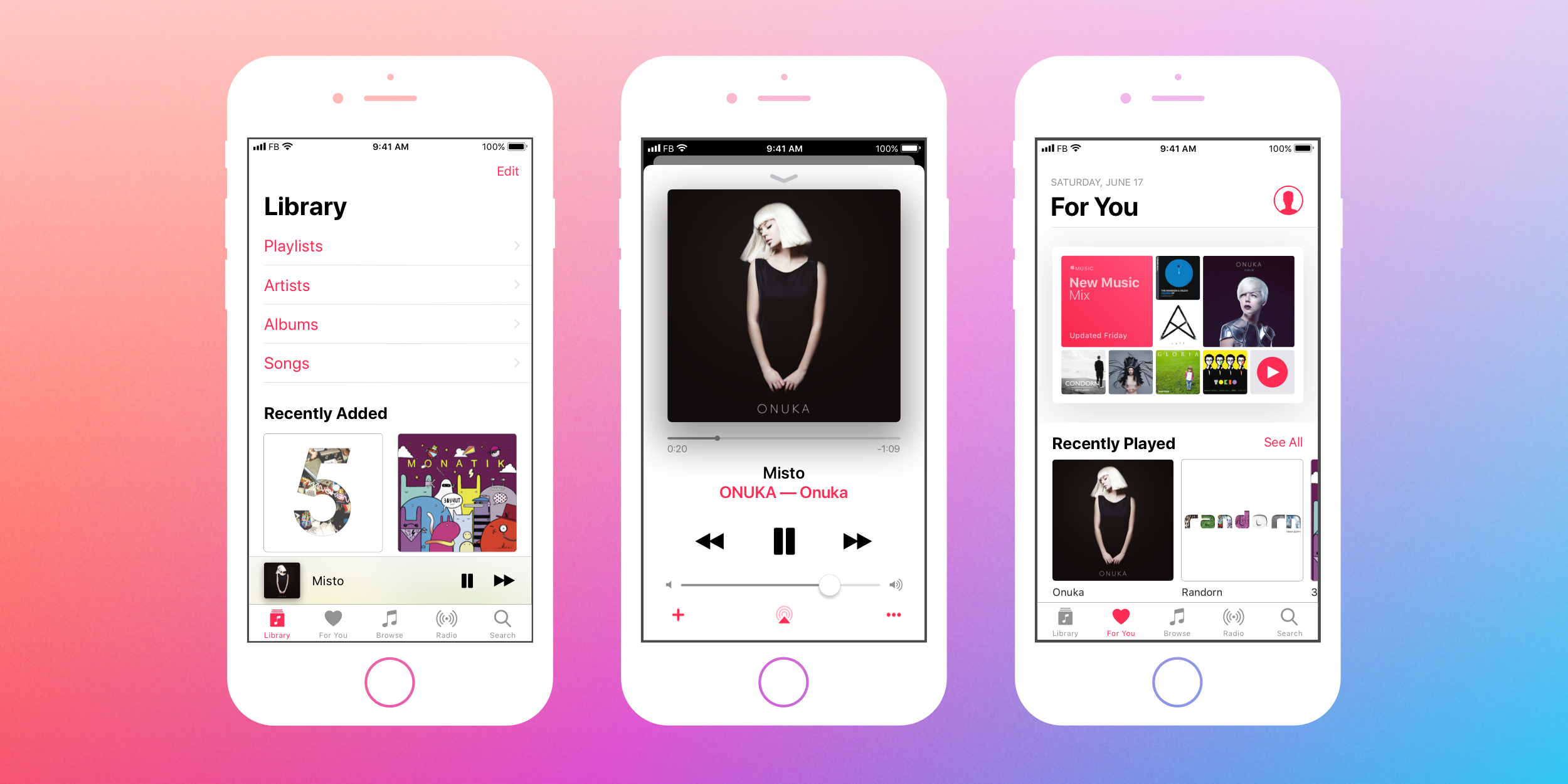
2. Life cycle of ViewController
For simplicity, suppose that each screen in a mobile application is a ViewContoller, or rather, it is a descendant of the UIViewContoller class. It is important for the iOS developer to know how the life cycle of the screen works, or rather, what methods will be performed during the preparation, appearance, rotation, destruction, and other screen conditions.
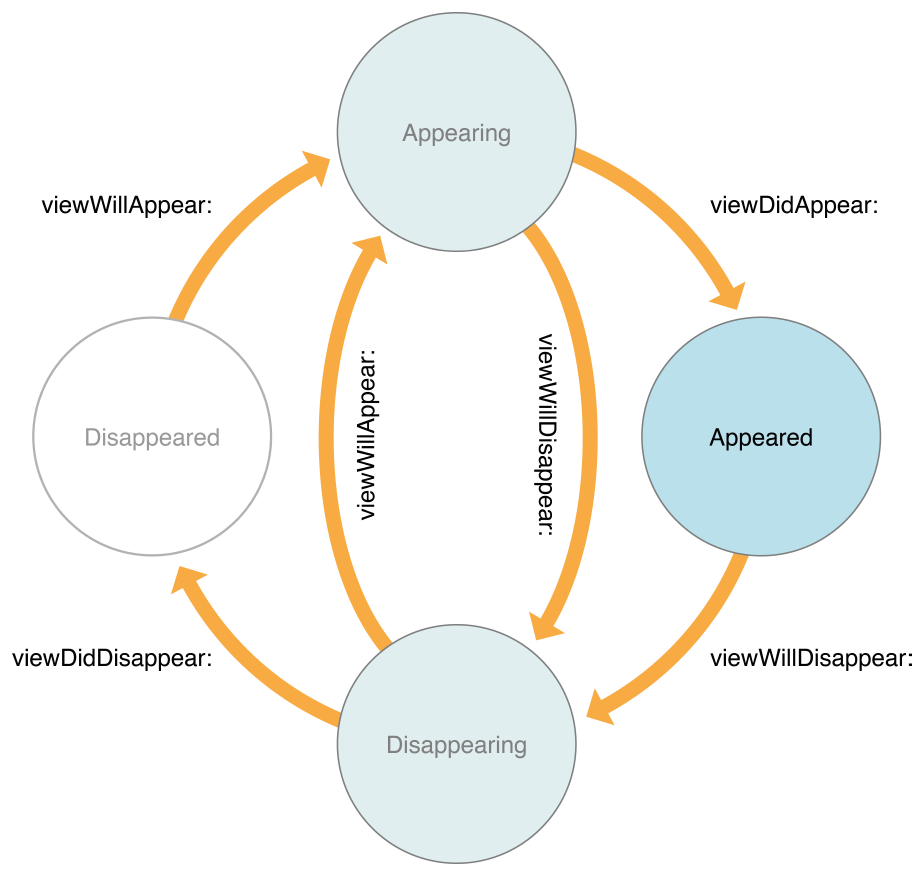
Related materials:
3. Work with UITableView and UICollectionView
Most of the mobile application interfaces are table-based. You should be able to add a table to the screen, prepare cells, implement the necessary protocols, assign a delegate and data source.
In addition, it is important to understand why identifiers are needed, and what is meant by cell reuse.
Related materials:
4. Grand Central Dispatch
Applications should be able to simultaneously solve several problems. For example, while a user flips through a news feed, data is downloaded from the network, and the interface is not blocked.
Grand Central Dispatch is one of the important and rather difficult topics that you should definitely know if you are going to program for iOS.
Related materials:
5. Networking, receiving data from JSON
Which of your favorite applications continue to work without an internet connection? I think that if you throw back the games, you will see that most of the applications work with data from the network. What, in this case, should be able to beginner iOS developer? I think that to create a network request, receive and process the data. Knowledge of URLSession should be enough for a start. This is the most basic approach, and I recommend first of all to master the application of it. It may not be out of place to know about the existence of such a popular library as Alamofire , but this should not be to the detriment of the URLSession.
Most often, data that comes to applications over the network is transmitted in JSON format. It is necessary to be able to receive and process this data, for example, to derive some list based on it. At the same time, it is advisable that you know how to do this not only with the help of one of the popular libraries, for example, SwiftyJSON , but also own the standard language tools, be familiar with Codable.
Quite a bit about working with URLSession is described in an article from Audrey Tam . There are a lot of materials on working with JSON; you can see the article by Anand Nimje .
To learn the topic in practice, I recommend making an application with a weather forecast, in which there is an input field for the name of the city, an API request is created ( OpenWeatherMap is suitable), and based on the received JSON, weather information is displayed.
6. Knowledge Swift
Reading the documentation will help a lot here . Learning Swift may take some time, but on the way out you should take along and be free to navigate the following topics:
- classes
- the structure
- transfers
- protocols
- collections
- methods of working with collections (enumeration, sorting, filtering, map, reduce, etc.)
- work with options and exceptions
These topics need to be known and mastered in the volume to which they are given in the documentation - all this will come in handy in the work. It is very good if for each topic you can give an example of a problem that can be solved with their help.
Carefully analyze the differences between structures and classes - not only because you will be asked this question at the interview with a high probability, it will just be necessary in your work.
In parallel with reading the documentation on Swift, you can watch the video marathon on Swift by Alexei Skutarenko. This marathon is not a substitute for reading documentation.due to the fact that not all topics are disclosed in it, and he also published 4 years ago, and during this time there have been small changes in Swift. Alexey did a tremendous job, there are a lot of useful material and thoughts.
7. Strong and weak links, ARC, memory leaks
How to make sure that the application does not have problems with lack of memory?
What are links, what are the types of links? What exactly does ARC do, and how to avoid the Retain Cycle, and what is it all about? The topic is not complicated, but extremely important. You can get acquainted, for example, in SwiftBook .
8. Protocols and delegates
When developing for iOS, you will often encounter delegation, or rather transfer of responsibility from one object to another. For example, the classic task is a table with cells, each of which has a button “add item to favorites”. You must be able to create a protocol for this situation, appoint a delegate, and implement protocol support for him.
Related materials:
9. Architectural approaches: MVC, MVVM, MVP, VIPER
For ease of creation and support of software products during development, they are divided into separate blocks. For example, one block is only responsible for working with data, and the other for appearance. This approach has a number of advantages, for example, you can change the appearance without touching the logic, or if necessary, you can change the data source, again without changing the rest of the application. Obviously, these approaches are used not only in iOS, I will say more - they came here from other platforms. There are several well-established approaches to sharing responsibility between different blocks; for working with iOS, I recommend a good understanding of MVC and MVVM.
Instead of a normal explanation of the division of responsibilities in architecture, I often hear in interviews, something like "MVC is bad because it is a Massive View Controller, and MVVM is much better."
Related materials:
- detailed article in Russian from Badoo
- two-part lesson from Lorenzo Boaro and Eli Ganem , revealing many architectural approaches in iOS
10. Data Storage, Core Data
As we said above, many applications work with data from the network. You must be able to save this data on the device, as well as save what the user entered. The basic set of ways to store data for a beginner iOS programmer, in my opinion, includes
- Keychain for something small and secret
- UserDefaults for small settings
- Core Data for storing objects, the relationships between them
There is no SQLite, Realm, Firebase in this list. You can master them if you wish, but certainly not to the detriment of the above methods. If you already have experience with SQL, then I recommend using SQLite on iOS as a reserve, it is very likely that you will find this approach to storage more convenient.
Related materials:
- translation of the KeyChain lesson into Russian (working with Keychain becomes much easier when using libraries like Keychain Swift )
- Hacking With Swift UserDefaults Quick Start Guide
- lesson with introduction to Core Data by Pietro Rea
- Core Data video course
Total
I believe that evenly distributing the above topics and devoting them to study from 20 hours a week, it’s really possible to master it all from scratch in a period of 4-5 months.
With this wealth of knowledge, you can start taking orders for small applications or go for interviews. For example, if there was a vacancy, we would have taken a person with this set of knowledge into our mobile team.
Write your finished application
I am sure that an iOS developer should have his own application. We are talking about a complete application that solves some necessary problem or problem (it’s okay if it solves only for the author of the application). In the process of writing your application, you will encounter creative tasks of finding suitable solutions, this will not only allow you to better consolidate theoretical knowledge, but also give you pleasure.
Do not be shy about your applications, show them at the interviews. Even if the application is simple, even if the design was done by ourselves, and it turned out not the most beautiful application (if the application is needed, the functionality can then be expanded, and the design can be made new).
PS
Please tell in the comments what topics you consider important for the development of new iOS developers