ACS, raspberries and teapot
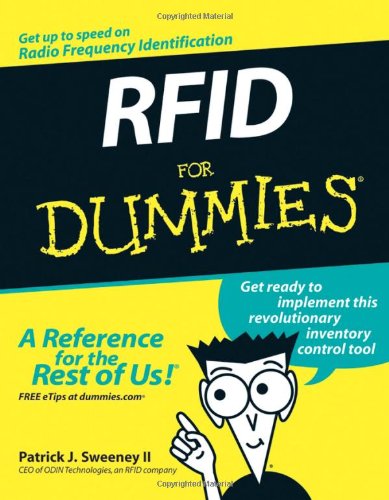
In the form of having a lot of diverse information on the Internet with not quite working examples, I will try to describe everything step by step and in detail. This module works with RFID cards at a frequency of 13.56 MHz and easily understands, in addition to cards and chips with such a frequency, Moscow uniform tickets (I used the used one-time single ticket), as well as NFC tags.
Hardware
To implement the project, you need to connect the module and several additional components.
Module connection diagram

To check the operation of the module, I used several diodes and piezodynamics. Red and green diodes - to indicate the status of the conditional lock (red - the lock is closed, green - the lock is open), the yellow diode - to indicate the control signal (in real conditions it will be replaced by a lock), the piezoelectric speaker - gives an audible signal when the lock is opened and signals an error. In a real circuit with a lock, it is advisable to use a relay to control the power circuit of the lock.
Diode and speaker connection diagram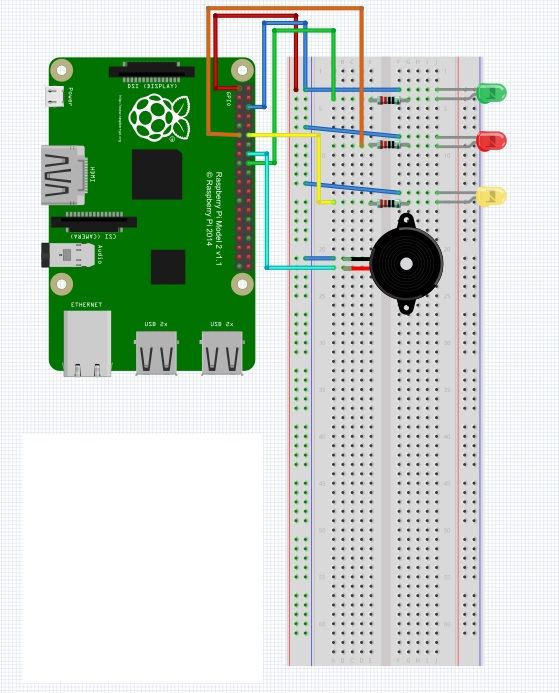
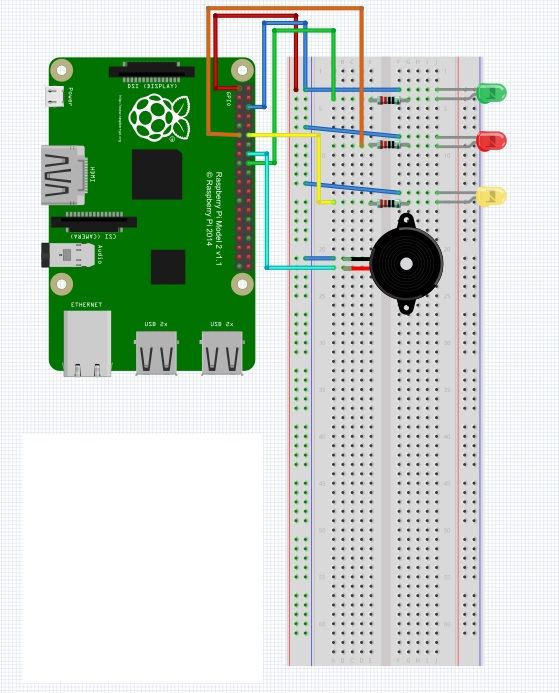
Software
First you need to enable SPI on Raspberry, if not enabled. How to do this is written here .
Now you can download the library to work with our module and check the reading and writing of cards. For us, reading is important, in particular reading the UID card, for this we enter in the console:
git clone https://github.com/mxgxw/MFRC522-python
For the downloaded code to work correctly, you must also download and install SPI-py:
git clone https://github.com/lthiery/SPI-Py
sudo python /SPI-Py/setup.py install
Now you can read the UID of the card and the information recorded in it. To do this, run their downloaded files example:
cd MFRC522-python
sudo python Read.py
The following should appear on the screen:
Welcome to the MFRC522 data read example
Press Ctrl-C to stop.
After that, you can bring a card or tag to the reader and we will see its UID
Card detected
Card read UID: 60,56,197,101
Size: 8
Sector 8 [12, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
In this case, we are interested in this part: UID: 60,56,197,101 .
And we can change this part
Spoiler heading
, replacing zeros with the values we need: Sector 8 [12, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0] , but this does not interest us now.
Further, for the operation of our module and other components, I wrote a simple python script based on various sources.
Script with my comments
#!/usr/bin/env python
import RPi.GPIO as GPIO
import MFRC522
import signal
import time
red = 11
green = 18
speaker = 16
doorlock = 12
# Список UID карт (UID карты узнаем с помощью sudo python /MFRC522-python/Read.py
card_01 = '6056197101' #white
card_02 = '148167101184' #fob
card_03 = '13652116101' #единый
#Настройка портов вывода
GPIO.setmode(GPIO.BOARD) # Это значит, что считаем пины по порядку с левого верхнего (3v3 - первый)
GPIO.setup(red, GPIO.OUT, initial=1) # Устанавливаем пин 18 на вывод
GPIO.setup(green, GPIO.OUT, initial=0) # тоже самое с пином 11
GPIO.setup(speaker, GPIO.OUT, initial=0) # пин 16
GPIO.setup(doorlock, GPIO.OUT, initial=0) # пин 12
continue_reading = True
def end_read(signal,frame): # что делать, если программу прервать и как её прервать
global continue_reading
print "Ctrl+C captured, ending read."
continue_reading = False
GPIO.cleanup()
# Create an object of the class MFRC522 (??)
MIFAREReader = MFRC522.MFRC522()
while continue_reading:
# Сканируем карты - считываем их UID
(status,TagType) = MIFAREReader.MFRC522_Request(MIFAREReader.PICC_REQIDL)
# Если карту удалось считать, пишем "карта найдена"
if status == MIFAREReader.MI_OK:
print "Card detected"
# Считываем UID карты
(status,uid) = MIFAREReader.MFRC522_Anticoll()
# Если считали UID, то идем дальше
if status == MIFAREReader.MI_OK:
# выводим UID карты на экран
UIDcode = str(uid[0])+str(uid[1])+str(uid[2])+str(uid[3])
print UIDcode
# Если карта есть в списке
if UIDcode == card_01 or UIDcode == card_03 or UIDcode == card_02:
# то дверь открывается
# предполагается, что замок открывается при подаче на
# него (на реле, управляющее замком), напряжения
# т.е. им управляет переключаемое реле
# т.е. замок открывается при высоком значении пина doorlock
# при этом, горит зеленая, тухнет красная и пищит динамик
GPIO.output((red, green, speaker, doorlock), (0,1,1,1))
print "Door open"
# успеть дернуть за 1 секунду
time.sleep(1)
GPIO.output((red, green, speaker, doorlock), (1,0,0,0))
# потом дверь закрывается, о чем нас извещают
print "Door closed"
# А если карты в списке нет, то моргаем и пищим
else:
GPIO.output((red, green, speaker, doorlock), (1,0,0,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (1,0,1,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (1,0,0,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (1,0,1,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (0,1,0,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (0,1,1,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (0,1,0,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (0,1,1,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (1,0,0,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (1,0,1,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (1,0,0,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (1,0,1,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (0,1,0,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (0,1,1,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (0,1,0,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (0,1,1,0))
time.sleep(0.05)
GPIO.output((red, green, speaker, doorlock), (1,0,0,0))
print "Unrecognised Card"
Create a file and copy the code into it:
nano rf.py
save the file (CTRL + x, y).
Run the script with the command
sudo python rf.py
After that, the following will happen: the
red diode will light up, indicating that our “lock” is closed.
If a card is brought to the reader whose number (without commas) is listed, then the red diode will go out, the green diode will light up, signaling that the “lock” is open, the yellow diode will light up - it is in this case “control”, indicating that “ lock ”there is a control signal, the piezodynamic speaker will beep. After a specified time (in our case 1 second), the diodes and speaker will return to their original state, the reader will wait for the card to be read.
If a card not from the list is brought to the reader, then the red and green diodes will blink, the piezoelectric speaker will be intermittently beeping, signaling an error, the yellow diode will not light up (ie, the signal to open the “lock” does not go). In a little less than a second, everything will return to its original state.
A short video illustrating the work: