
Using environment variables in Node.js
- Transfer
The material on environment variables in Node.js, the translation of which we are publishing today, was written by Burke Holland , a web developer whose interests include JavaScript, Node.js and VS Code. In addition, it is worth noting that he has developed a difficult relationship with Java. Here is his story.
Environment variables are one of the fundamental constructs of Node.js, but for some reason I never tried to learn how to use them correctly. Perhaps this happened because of their name - "Environment Variables". This name caused me something like a post-traumatic syndrome, unpleasant memories of how I tried to add the path to the Java home directory on Windows. I then really could not understand whether to add this path to the PATH variable, to the JAVA_HOME variable, or both. It was also unclear whether a semicolon should be at the end of this path. Actually, then I had a question about why I use Java. Be that as it may, I finally found the strength in myself and began to get acquainted with the environment variables of Node.
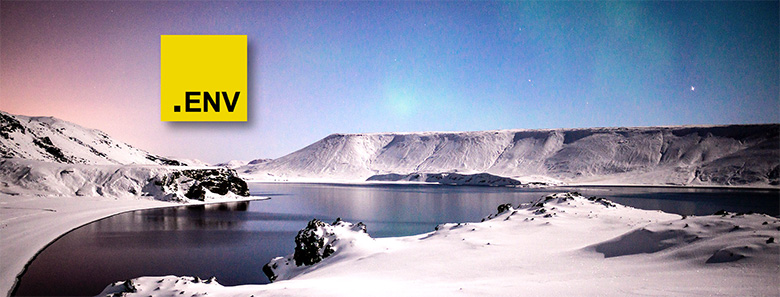
If you write for the Node.js platform, and just like me, it doesn’t matter - for what reasons, you are still not particularly familiar with environment variables - I propose to fix this.
In Node, environment variables can be global (as in Windows), but they are often used when applied to a specific process in which the developer is interested. For example, if you have a web application, this means that the following environment variables can be used in it:
In this context, environment variables are actually more like “Configuration Settings” - for me, it sounds much better than “Environment Variables”.
If you previously programmed for .NET, you may be familiar with something like a file
By the way, on the topic “hard code” - assigning certain values in the code instead of receiving them from external sources, I want to share my own tweet.

I quote myself at the peak of insanity.
How to use environment variables in Node.js applications? I had to work hard to find good material on environment variables in Node, with an indispensable condition for this material to be enough jokes about Java. I did not find such material, so I decided to write it myself.
Here are some ways to use environment variables in applications for Node.js.
You can specify environment variables in the terminal where you plan to run Node. For example, if you have an application that uses Express and want to pass port information to it, you can do this like this:
By the way, an interesting thing. It turns out that the greatest value that a port number can take is 65535. How did I find out? Of course I found it on StackOverflow . How does anyone know anything? But in Node, the largest port number is 65534. Why? I have no idea. I can’t know absolutely everything.
So, to use the environment variable in the code, you need to use the object
However, the use of the approach described here may end badly. If you have, for example, a connection string to the database, you will probably not be particularly pleased with the prospects of entering it into the terminal. In fact, entering long values in the terminal is like a painful addiction, and we don’t need it at all. See for yourself:
This approach does not scale, but everyone wants to scale. According to each architect, next to whom I have sat at different events, “scaling” is even more important than the fact that the application is working.
Therefore, consider another approach, which is to use files
Files
You can read these values in different ways. Perhaps the easiest way is with a package
After installing the package, you need to connect it to the project, and then you can use it to work with environment variables. This package will find the file
By the way, I want to share important advice. Do not upload files
So, so far so good, but what we were talking about here is not very convenient. You have
What to do? Fortunately, you use VS Code (I am absolutely sure of this), which means that you have a few more options.
To get started, you can install the DotENV extension for VS Code, which will give a nice highlight of the file syntax

Here's what an .env file looks like without syntax highlighting and highlighting
In addition, the VS Code debugger, if you use it, offers some more convenient options for loading values from files
The Node.js debugger for VS Code (it is installed by default) supports downloading files
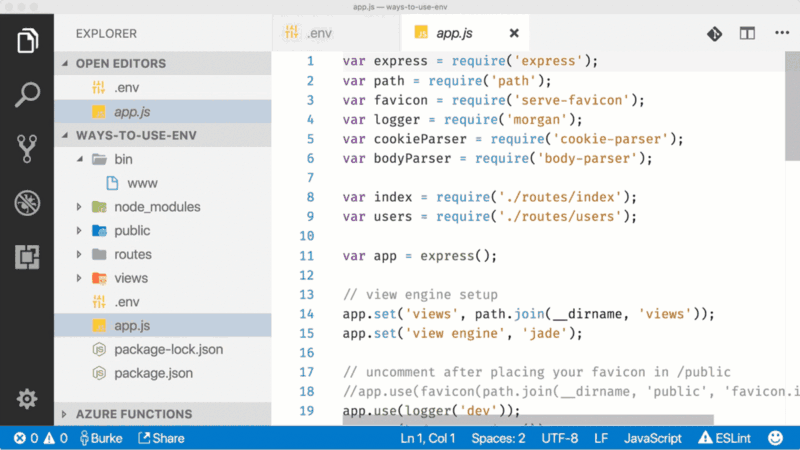
Creating a basic launch configuration for Node
After you create a basic launch configuration for Node (click on the gear icon and select Node), you can do one of the following, or do both.
The first option is to include the variables in the configuration file.
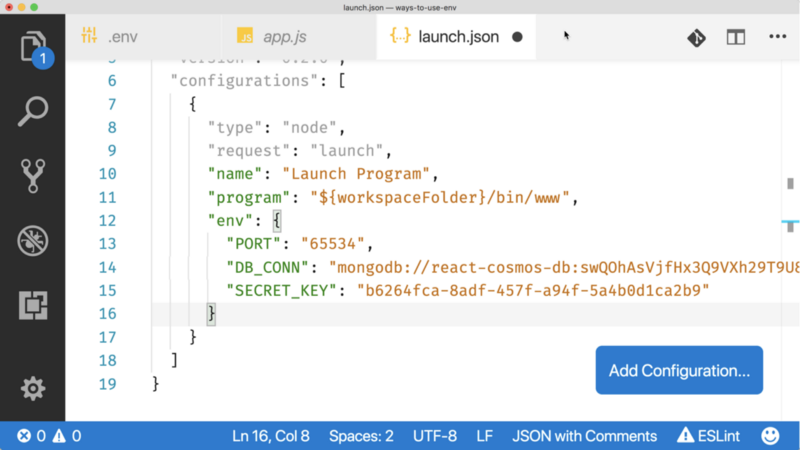
Variables in the configuration file
This is a perfectly acceptable option, but it bothers me a little that each value should be a string. Still, some values are numbers, not strings, and in JavaScript there are only, let's say, three main data types, and I would not want to lose one of them.
Environment variables can be transferred to VS Code in a simpler way. We have already found out that the files

The path to the .env file in the configuration file
As long as we start the Node.js process from VS Code, the file with environment variables will be transferred to this process. In this case, we do not have to squeeze the numbers in quotation marks, making strings out of them, and we do not have to deploy unnecessary code in production. Well, at least you won’t have to do this.
You may have come to this place and thought that you never run Node projects with commands of the form
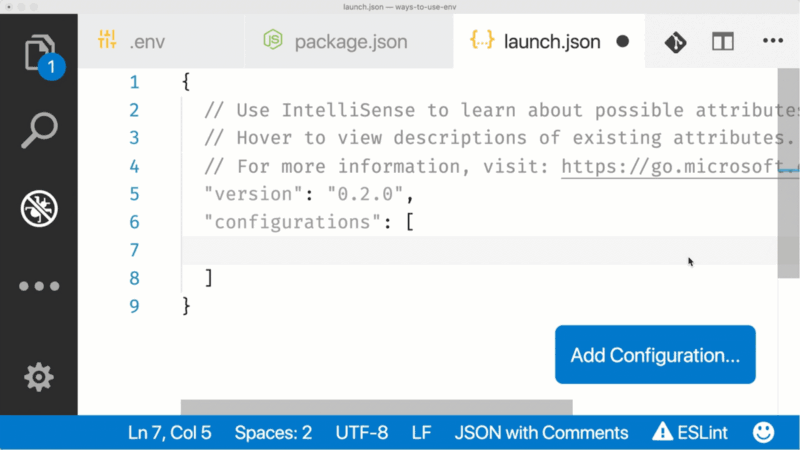
Launch Via NPM task
After that, you can configure the value of the parameter

Configuring the launch of the project using npm
Note that

--Inspect flag in package.json
So, we figured out how to use environment variables during development. You most likely will not use the files
In production, the definition of environment variables will depend on the features of the platform used. For example, in the case of Azure, there are three ways to declare and manage such variables.
The first way is to use the Azure CLI .
It works, but it doesn’t look very good. Another way is to use the Azure web portal. I do not often use the web portal, but when this happens, I turn to it for setting environment variables.
Here, what we call “environment variables” is called “Application Settings.”

Setting environment variables in Azure
Another option, considering that you use VS Code, is to install the App Service extension and configure the Application Settings described above directly from the editor.

Setting environment variables from VS Code
I like to do absolutely everything in VS Code, and if I could write emails there, I would do so. By the way , it seems my dream has come true.
Now you know the same thing that I know (not so much, let me say), and I feel that I have fulfilled my goal through jokes on the subject of Java. If all of a sudden they aren’t enough here, here’s another one whose author is unknown: “Java is a very powerful tool for turning XML into stack traces.”
We hope this material made it possible for those who had not used them before to fill the knowledge gaps in environment variables in Node, and those who already knew about them, allowed to learn something new about .env files, about the use of environment variables on battle servers , and how to make your life easier by developing Node projects in VS Code. If you are interested in the topic of Node environment variables, here is one of our previous publications on this topic dedicated to the features of working with process.env.
Dear readers! How do you solve the problems of using Node environment variables during development and production?

Environment variables are one of the fundamental constructs of Node.js, but for some reason I never tried to learn how to use them correctly. Perhaps this happened because of their name - "Environment Variables". This name caused me something like a post-traumatic syndrome, unpleasant memories of how I tried to add the path to the Java home directory on Windows. I then really could not understand whether to add this path to the PATH variable, to the JAVA_HOME variable, or both. It was also unclear whether a semicolon should be at the end of this path. Actually, then I had a question about why I use Java. Be that as it may, I finally found the strength in myself and began to get acquainted with the environment variables of Node.
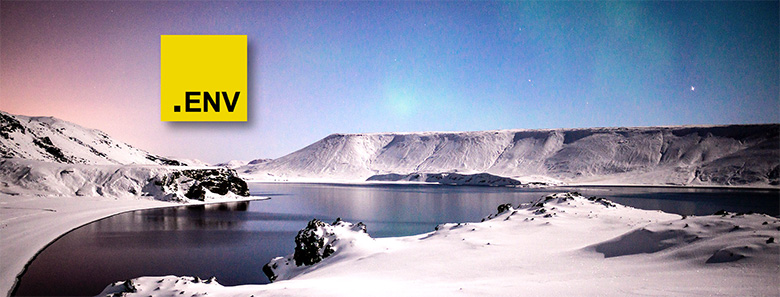
If you write for the Node.js platform, and just like me, it doesn’t matter - for what reasons, you are still not particularly familiar with environment variables - I propose to fix this.
Environment Variables in Node.js
In Node, environment variables can be global (as in Windows), but they are often used when applied to a specific process in which the developer is interested. For example, if you have a web application, this means that the following environment variables can be used in it:
- The HTTP port that this application will listen on.
- Database connection string.
- The path to JAVA_HOME, oh wait, it's past. Please note that recovery takes time.
In this context, environment variables are actually more like “Configuration Settings” - for me, it sounds much better than “Environment Variables”.
If you previously programmed for .NET, you may be familiar with something like a file
web.config
. Node environment variables play almost the same role as settings from web.config
- they represent a mechanism for transferring information to the application that the developer does not want to set hard in the code. By the way, on the topic “hard code” - assigning certain values in the code instead of receiving them from external sources, I want to share my own tweet.

I quote myself at the peak of insanity.
How to use environment variables in Node.js applications? I had to work hard to find good material on environment variables in Node, with an indispensable condition for this material to be enough jokes about Java. I did not find such material, so I decided to write it myself.
Here are some ways to use environment variables in applications for Node.js.
Indication of environment variables in the terminal
You can specify environment variables in the terminal where you plan to run Node. For example, if you have an application that uses Express and want to pass port information to it, you can do this like this:
PORT=65534 node bin/www
By the way, an interesting thing. It turns out that the greatest value that a port number can take is 65535. How did I find out? Of course I found it on StackOverflow . How does anyone know anything? But in Node, the largest port number is 65534. Why? I have no idea. I can’t know absolutely everything.
So, to use the environment variable in the code, you need to use the object
process.env
. It looks like this:var port = process.env.PORT;
However, the use of the approach described here may end badly. If you have, for example, a connection string to the database, you will probably not be particularly pleased with the prospects of entering it into the terminal. In fact, entering long values in the terminal is like a painful addiction, and we don’t need it at all. See for yourself:
PORT=65534 DB_CONN="mongodb://react-cosmos-db:swQOhAsVjfHx3Q9VXh29T9U8xQNVGQ78lEQaL6yMNq3rOSA1WhUXHTOcmDf38Q8rg14NHtQLcUuMA==@react-cosmos-db.documents.azure.com:19373/?ssl=true&replicaSet=globaldb" SECRET_KEY=b6264fca-8adf-457f-a94f-5a4b0d1ca2b9 node bin/www
This approach does not scale, but everyone wants to scale. According to each architect, next to whom I have sat at different events, “scaling” is even more important than the fact that the application is working.
Therefore, consider another approach, which is to use files
.env
.Using .env Files
Files
.env
are intended for storage of environment variables. To use this technology, it is enough to create a file with a name in the project .env
and add environment variables into it, starting each with a new line:PORT=65534
DB_CONN="mongodb://react-cosmos-db:swQOhAsVjfHx3Q9VXh29T9U8xQNVGQ78lEQaL6yMNq3rOSA1WhUXHTOcmDf38Q8rg14NHtQLcUuMA==@react-cosmos-db.documents.azure.com:10255/?ssl=true&replicaSet=globaldb"
SECRET_KEY="b6264fca-8adf-457f-a94f-5a4b0d1ca2b9"
You can read these values in different ways. Perhaps the easiest way is with a package
dotenv
from npm
:npm install dotenv --save
After installing the package, you need to connect it to the project, and then you can use it to work with environment variables. This package will find the file
.env
and load the variables described in it into Node. Here's what it looks like://Использование пакета dotenv для чтения переменных из файла .env в Node
require('dotenv').config();
var MongoClient = require('mongodb').MongoClient;
// Обращение к переменным из .env, которые теперь доступны в process.env
MongoClient.connect(process.env.DB_CONN, function(err, db) {
if(!err) {
console.log("We are connected");
}
});
By the way, I want to share important advice. Do not upload files
.env
to GitHub. They contain information not intended for prying eyes. Do not repeat my mistakes. So, so far so good, but what we were talking about here is not very convenient. You have
dotenv
to connect in every file where you need to use environment variables, and you have dotenv
to use it in production when, in essence, you do not need it. I am not a fan of deploying useless code in production, although, it seems to me, so far I have only done this. What to do? Fortunately, you use VS Code (I am absolutely sure of this), which means that you have a few more options.
Working with .env files in VS Code
To get started, you can install the DotENV extension for VS Code, which will give a nice highlight of the file syntax
.env
.
Here's what an .env file looks like without syntax highlighting and highlighting
In addition, the VS Code debugger, if you use it, offers some more convenient options for loading values from files
.env
.VS Code Launch Configuration
The Node.js debugger for VS Code (it is installed by default) supports downloading files
.env
through launch configurations. Details on launch configurations can be found here .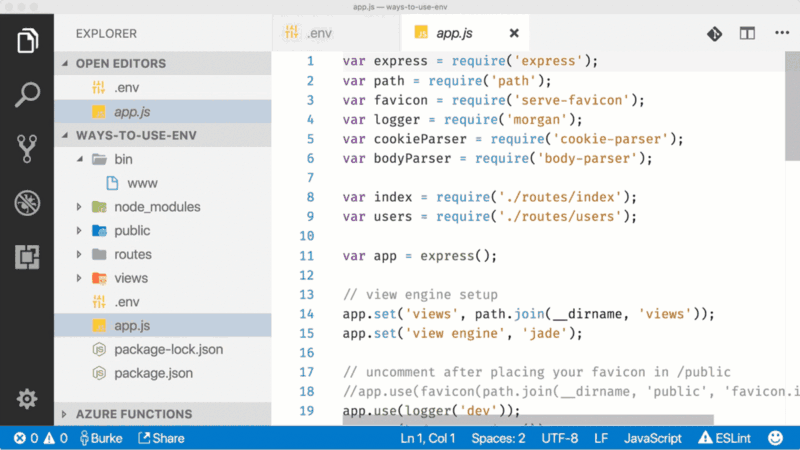
Creating a basic launch configuration for Node
After you create a basic launch configuration for Node (click on the gear icon and select Node), you can do one of the following, or do both.
The first option is to include the variables in the configuration file.
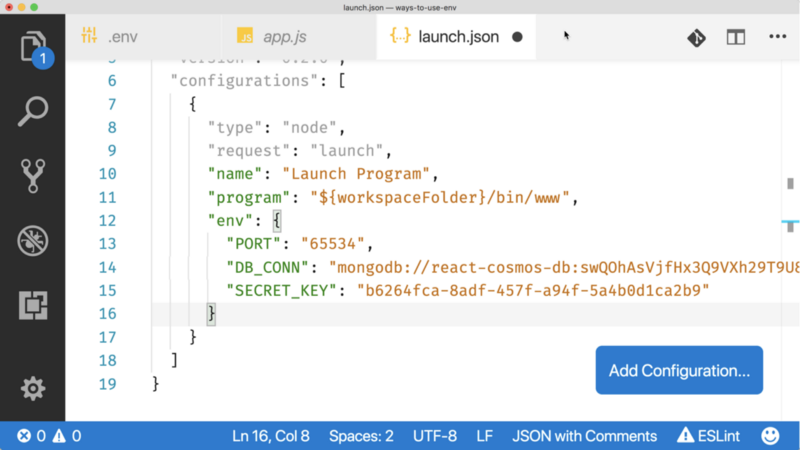
Variables in the configuration file
This is a perfectly acceptable option, but it bothers me a little that each value should be a string. Still, some values are numbers, not strings, and in JavaScript there are only, let's say, three main data types, and I would not want to lose one of them.
Environment variables can be transferred to VS Code in a simpler way. We have already found out that the files
.env
are our friends, so instead of entering the values of the variables in the configuration file, we simply indicate the path to the file there .env
.
The path to the .env file in the configuration file
As long as we start the Node.js process from VS Code, the file with environment variables will be transferred to this process. In this case, we do not have to squeeze the numbers in quotation marks, making strings out of them, and we do not have to deploy unnecessary code in production. Well, at least you won’t have to do this.
Running node scripts through NPM
You may have come to this place and thought that you never run Node projects with commands of the form
node …
, always using npm scripts like npm start
. VS Code launch configurations can also be used in this case. However, instead of using the standard Node launcher, you can configure the Launch Via NPM task.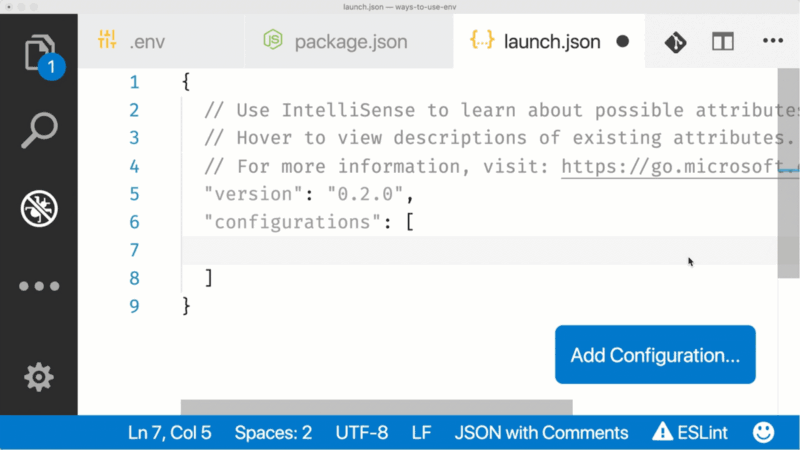
Launch Via NPM task
After that, you can configure the value of the parameter
envFile
by specifying the path to the file .env
and the parameter runtimeArgs
to run the desired script. Usually, start
or acts as a script debug
.
Configuring the launch of the project using npm
Note that
package.json
you need to add a flag to the npm script --inspect
so that VS Code can connect a debugger to the process. Otherwise, although the task starts, the debugger cannot do anything useful.
--Inspect flag in package.json
Environment Variables in Production
So, we figured out how to use environment variables during development. You most likely will not use the files
.env
in production, and the VS Code launch configuration on the server will not bring much benefit. In production, the definition of environment variables will depend on the features of the platform used. For example, in the case of Azure, there are three ways to declare and manage such variables.
The first way is to use the Azure CLI .
az webapp config appsettings set -g MyResourceGroup -n MyApp --settings PORT=65534
It works, but it doesn’t look very good. Another way is to use the Azure web portal. I do not often use the web portal, but when this happens, I turn to it for setting environment variables.
Here, what we call “environment variables” is called “Application Settings.”

Setting environment variables in Azure
Another option, considering that you use VS Code, is to install the App Service extension and configure the Application Settings described above directly from the editor.

Setting environment variables from VS Code
I like to do absolutely everything in VS Code, and if I could write emails there, I would do so. By the way , it seems my dream has come true.
Summary
Now you know the same thing that I know (not so much, let me say), and I feel that I have fulfilled my goal through jokes on the subject of Java. If all of a sudden they aren’t enough here, here’s another one whose author is unknown: “Java is a very powerful tool for turning XML into stack traces.”
We hope this material made it possible for those who had not used them before to fill the knowledge gaps in environment variables in Node, and those who already knew about them, allowed to learn something new about .env files, about the use of environment variables on battle servers , and how to make your life easier by developing Node projects in VS Code. If you are interested in the topic of Node environment variables, here is one of our previous publications on this topic dedicated to the features of working with process.env.
Dear readers! How do you solve the problems of using Node environment variables during development and production?
