Office 365. Web application development. Authorization ADAL JS, the new Microsoft Graph API
- Tutorial

- register an application in corporate Azure
- authorize users
- obtain data from Office 365 services
The main goal: to learn how to make Office 365 applications that can be sold in the Microsoft AppSource Marketplace .
For example, let's create a minimalistic Single Page web-application in JavaScript that displays a list of letters. Without Angular, TypeScript, without collectors, without command lines and without server code.
If you want to immediately get working code - an example on GitHub .
The result will be like this:
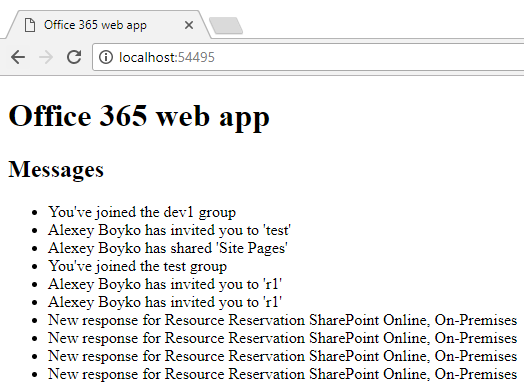
We will need
- subscription to Office 365
- local web server, for example, IIS Express in Visual Studio
Terminology what Office 365 apps come in
There is a lot of information on Office 365 applications, it’s hard to figure out where to start. Therefore, here are a few keywords and terms for googling.
Office 365 app launcher - shown in the image below. By clicking on “Get more apps” we get to the store .
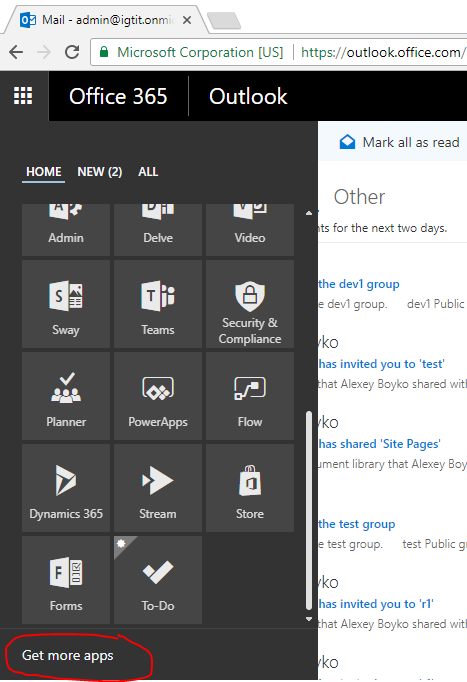
We want to make an application that can be published in this store.
Among the Office 365 applications, one can single out:
- Office 365 Add-in
- Office 365 web app, aka Azure AD web app
Office 365 Add-in - these are add-ons integrated into the Office 365 services UI. For example, this can be an additional panel with information viewing letters, SharePoint web part, button in Excel. Note that add-ons can work not only in web versions (for example, Outlook Web Access), but also in desktop applications (Outlook).
Office 365 web app - this
is a freestanding web application
- opens on its domain
- with its own hosting
- communicates with the Office 365 services (such as Outlook, SharePoint) using a Web-API (such as the Microsoft Graph the API , but not only - see below.)
That is, it is an independent separate site, it is connected with Office 365 by the fact that it receives / modifies data of users of Office 365. For this site to gain access to the Office 365 API, it must be registered in Office 365 (Azure AD). A link to the application can be placed in the app launcher.
The administrator can do the registration manually or by installing the application from the store. During registration, application rights to parts of the API (for example, access to mail) are indicated. Office 365 web apps are displayed in this section of the store:
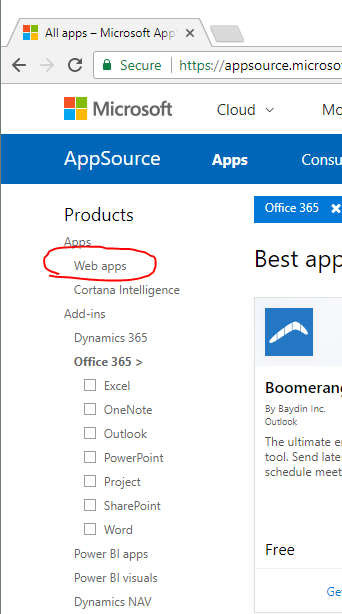
In this article we will make Office 365 web app, we will register manually.
Multi-Tenant, single organization (Single-Tenant) web-apps - Multi-Tenant are applications that several organizations can work with. Single organization - applications developed for one company. For the store, of course, only Multi-Tenant are suitable.
Register a web app in Azure
For our Office 365 web app (our site) to use the Office 365 API, it must be registered. The manual registration process is shown below.
We go to the Azure admin panel .

In the Sign-on URL field, specify the URL of the site, in our case, the test application is hosted on localhost:
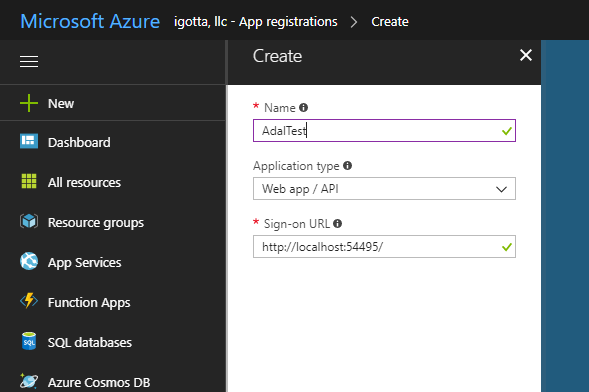
In the application manifest, you need to set the parameter "oauth2AllowImplicitFlow": true.

After registration, the application will receive an Application ID, we will need it later. Do not forget to give rights to Microsoft Graph.

Authorization, Microsoft Graph API
Office 365 offers many different web APIs. Microsoft Graph API is the most modern API, it allows you to work with all Office 365 services. Wherever you need to use Microsoft Graph, only if necessary resort to more specialized APIs.
Microsoft Graph API is a separate topic, here we just note that it is convenient and allows you to make simple REST requests.
Each request to the API must contain a secret token, thanks to which the Microsoft Graph API understands from which user the request came and what actions are allowed to be performed.
Token issues the “Azure AD Authorization Endpoint”. Token has a limited lifespan.
In our case, a single page web application, without server code, token is obtained as follows:
1. The user opens the site (we have http: // localhost: 54495 /)
2. JavaScript sends the user to the Microsoft authorization page (let's call it “Azure AD Authorization Endpoint”), while transmitting the application ID (see Application ID earlier)
3 . “Azure AD Authorization Endpoint” generates a token, redirects the user back to the site in the url after # indicates token.
4. JavaScript site saves token (for example, in local storage). Now you can make requests to the Office 365 API by passing a token.
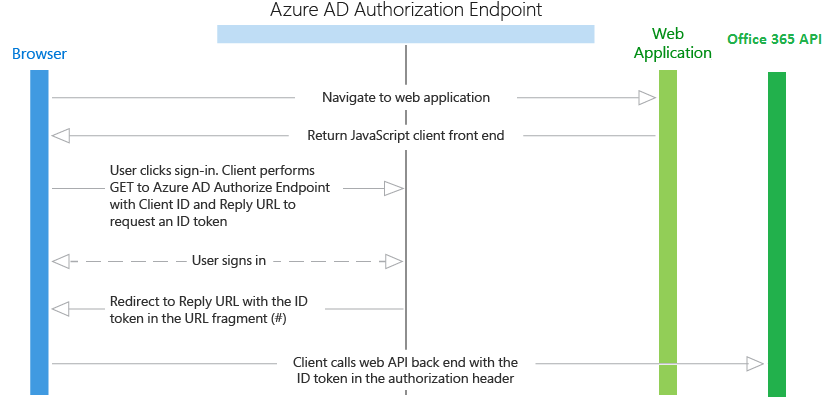
The process is described in more detail in the article Authentication Scenarios for Azure AD .
Our application does not contain server code. Pay attention to the Browser scheme only once calls the Web Application to get HTML and JS code, the rest of the requests the Browser makes to the Office 365 API.
But you can make your own ASP.NET MVC application, which, like the Office 365 API, will check the same token. And not in the browser, but on the server to use the Office 365 API. You don’t have to manually parse the HTTP headers - Microsoft has taken care of everything. An example of such an application is “ Integrating Azure AD into an AngularJS single page app ”.
ADAL JS
Above, for understanding, we examined the process of obtaining and using token. The Active Directory Authentication Library (ADAL) for JavaScript (ADAL JS) does all this for us. You just need to connect it to the page. An example of using ADAL JS:
// create config and get AuthenticationContext
window.config = {
tenant: "igtit.onmicrosoft.com",
clientId: "21XXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
postLogoutRedirectUri: window.location.origin,
endpoints: {
graphApiUri: "https://graph.microsoft.com",
},
cacheLocation: "localStorage"
};
var authContext = new AuthenticationContext(config);
var isCallback = authContext.isCallback(window.location.hash);
authContext.handleWindowCallback();
if (isCallback && !authContext.getLoginError()) {
window.location = authContext._getItem(authContext.CONSTANTS.STORAGE.LOGIN_REQUEST);
}
// check if user need to login
var user = authContext.getCachedUser();
var token = authContext.getCachedToken(config.clientId);
if (!user || !token) {
authContext.login();
}
// use token
authContext.acquireToken(config.endpoints.graphApiUri, function (error, token) {
if (error || !token) {
// TODO: for some error call authContext.login()
console.log("ADAL error: " + error);
return;
}
// TODO: USE token here, request graph Api
});
In our example, token will be stored in local storage.
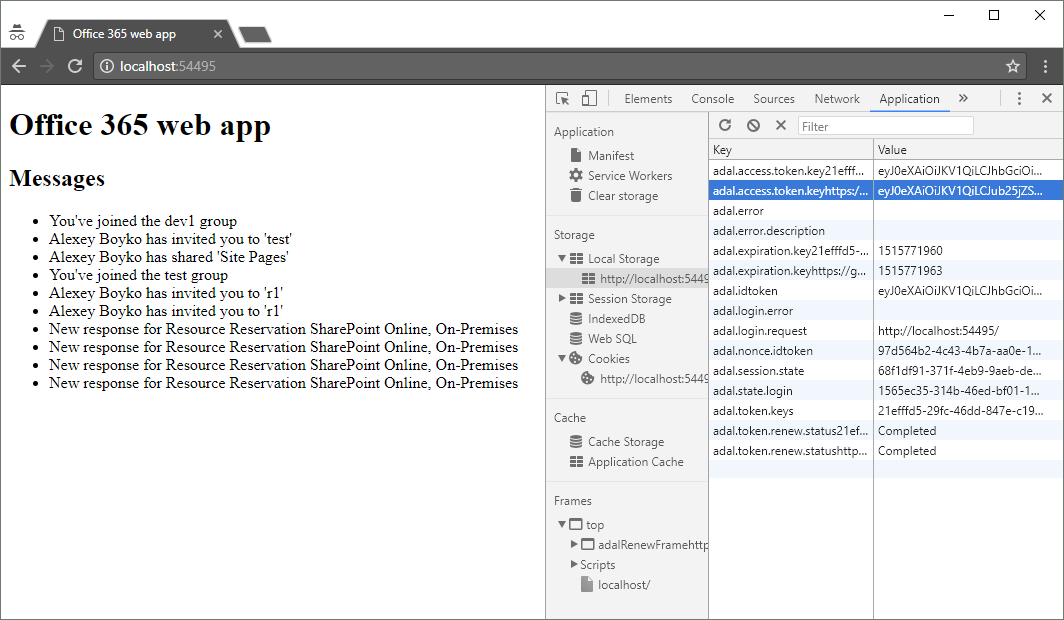
At request of Graph API it is necessary to transfer token
var url = config.endpoints.graphApiUri + "/v1.0/me/messages";
$.ajax({
type: "GET",
url: url,
headers: {
"Authorization": "Bearer " + token
}
}).done(function (response) {
// messages
var items = response.value;
console.log(items);
}).fail(function () {
console.log("Error");
});
Full example on github .
References
Have your app appear in the Office 365 app launcher
Authentication Scenarios for Azure AD
ADAL.js with Multi-Tenant Azure Active Directory
Active Directory Authentication Library (ADAL) for JavaScript
Authenticate an Office 365 user with ADAL JS
Microsoft Graph API