React Tutorial, Part 3: Component Files, Project Structure
- Transfer
- Tutorial
In this article we will talk about the component files and the structure of React-projects.
→ Part 1: course overview, reasons for the popularity of React, ReactDOM and JSX
→ Part 2: functional components
→ Part 3: component files, project structure
→ Part 4: parent and child components
→ Part 5: starting work on a TODO application, basics of styling
→ Part 6: about some features of the course, JSX and JavaScript
→ Part 7: inline styles
→ Part 8: continued work on a TODO application, familiarity with the properties of components
→ Part 9: properties of components
→
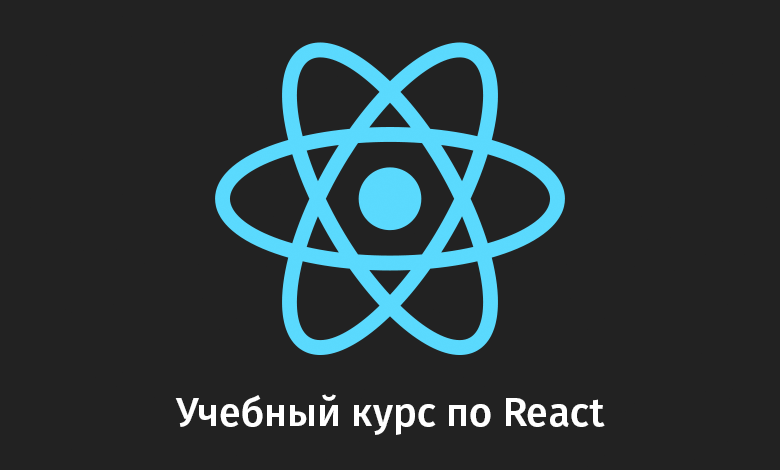
Part 10: workshop on working with component properties and styling
→ Part 11: dynamic markup generation and array method map
→ Part 12: workshop, third stage of working on a TODO application
→ Part 13: class-based components
→ Part 14: workshop on class-based components, component state
→ Part 15: workshops on working with component state
→ Part 16: fourth stage of working on a TODO application, event handling
→ Part 17: fifth stage of working on a TODO application, modifying component state
→ Part 18 : over that phase of work on TODO-application
→Part 19: component life cycle methods
→ Part 20: first lesson on conditional rendering
→ Part 21: second lesson and practice on conditional rendering
→ Part 22: seventh stage of working on a TODO application, loading data from external sources
→ Part 23: first lesson on working with forms
→ Part 24: second lesson on working with forms
→ Part 25: a workshop on working with forms
→ Part 26: application architecture, Container / Component pattern
→ Part 27: course project
→ Original
If we assume that you performed the task from the previous practical lesson using the standard project created
Please note that the function component code is
Component files are given names that correspond to the names of the components whose code they store. Place them, in the case of
Create a file
At this stage, the work
The code
Here is how it all looks in VSCode.
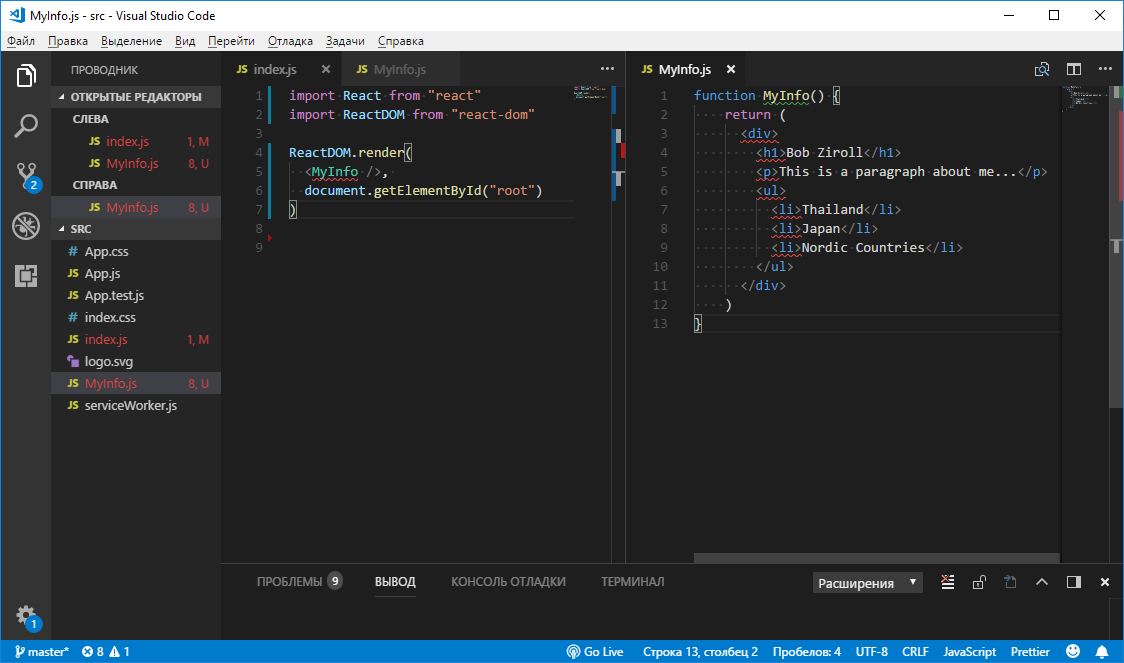
Transferring the component code to a new file
The component code
First of all, remember what the
Secondly, we need to make the function
Now let's work on the file
What if you try to write down the component import command based on the import command
The system, seeing such a command, in particular, based on the fact that it lacks information about the relative path to the file, will look for a project dependency - a module with the name specified when calling this command ( here's how to install dependencies in projects created by means
In addition, if we talk about the team
Usually, such JS file import commands are written this way.
Here is the complete file code
With the growth of the size and complexity of React-projects it is very important to maintain their structure in good condition. In our case, although our project is now small, it is possible, in a folder
Create such a folder and move the file into it
Namely, now the path to
And this is how it all looks in VSCode.
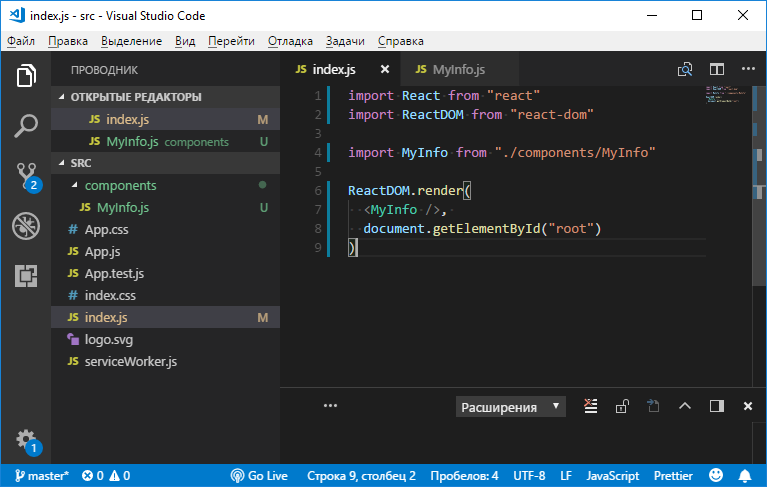
Folder for storing components and importing a component from this folder into VSCode
In fact, one folder
It is recommended to experiment with everything that you have learned today. For example, you can try to move a file.
In this lesson, we talked about the design of the component code as separate files, about exporting and importing code using ES6 tools, and about the structure of React projects. Next time we will continue to get acquainted with the capabilities of the components.
Dear readers! We ask experienced React-developers to share with newbies ideas about the organization of the project structure.

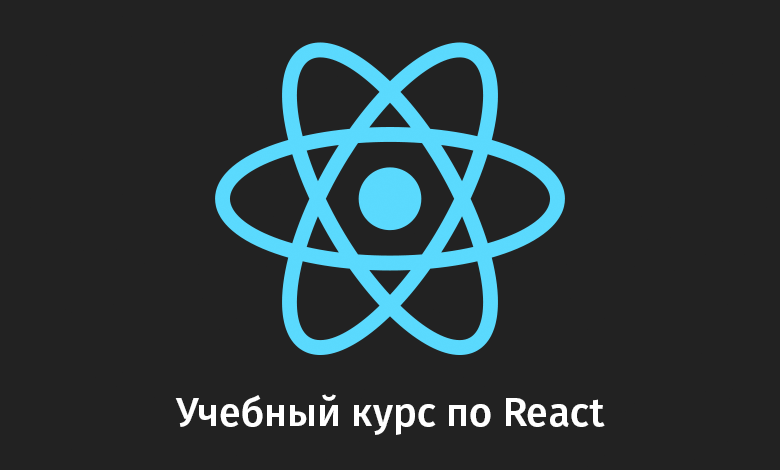
Part 10: workshop on working with component properties and styling
→ Part 11: dynamic markup generation and array method map
→ Part 12: workshop, third stage of working on a TODO application
→ Part 13: class-based components
→ Part 14: workshop on class-based components, component state
→ Part 15: workshops on working with component state
→ Part 16: fourth stage of working on a TODO application, event handling
→ Part 17: fifth stage of working on a TODO application, modifying component state
→ Part 18 : over that phase of work on TODO-application
→Part 19: component life cycle methods
→ Part 20: first lesson on conditional rendering
→ Part 21: second lesson and practice on conditional rendering
→ Part 22: seventh stage of working on a TODO application, loading data from external sources
→ Part 23: first lesson on working with forms
→ Part 24: second lesson on working with forms
→ Part 25: a workshop on working with forms
→ Part 26: application architecture, Container / Component pattern
→ Part 27: course project
Lesson 8: Component Files, React Project Structure
→ Original
Компонентов Component Files
If we assume that you performed the task from the previous practical lesson using the standard project created
create-react-app
, then now the file index.html
from the folder public
whose contents we are comfortable with and the file index.js
from the folder src
in which we write the code are involved in it . In particular, index.js
it now looks like this:import React from"react"import ReactDOM from"react-dom"functionMyInfo() {
return (
<div>
<h1>Bob Ziroll</h1>
<p>This is a paragraph about me...</p>
<ul>
<li>Thailand</li>
<li>Japan</li>
<li>Nordic Countries</li>
</ul>
</div>
)
}
ReactDOM.render(
<MyInfo />,
document.getElementById("root")
)
Please note that the function component code is
MyInfo
contained in this file. As you remember, React allows you to create many components, this is one of its strengths. It is clear that to place the code of a large number of components in a single file, although technically feasible, in practice means great inconvenience. Therefore, the code of components, even small in size, is usually made up as separate files. This approach is recommended to follow when developing React-applications. Component files are given names that correspond to the names of the components whose code they store. Place them, in the case of
create-react-app
, in the same folder src
where the file is located index.js
. With this approach, the file with the component MyInfo
will receive a name MyInfo.js
.Create a file
MyInfo.js
and transfer the component code to it by MyInfo
removing it from index.js
. At this stage, the work
index.js
will look like this:import React from"react"import ReactDOM from"react-dom"
ReactDOM.render(
<MyInfo />,
document.getElementById("root")
)
The code
MyInfo.js
will be:functionMyInfo() {
return (
<div>
<h1>Bob Ziroll</h1>
<p>This is a paragraph about me...</p>
<ul>
<li>Thailand</li>
<li>Japan</li>
<li>Nordic Countries</li>
</ul>
</div>
)
}
Here is how it all looks in VSCode.
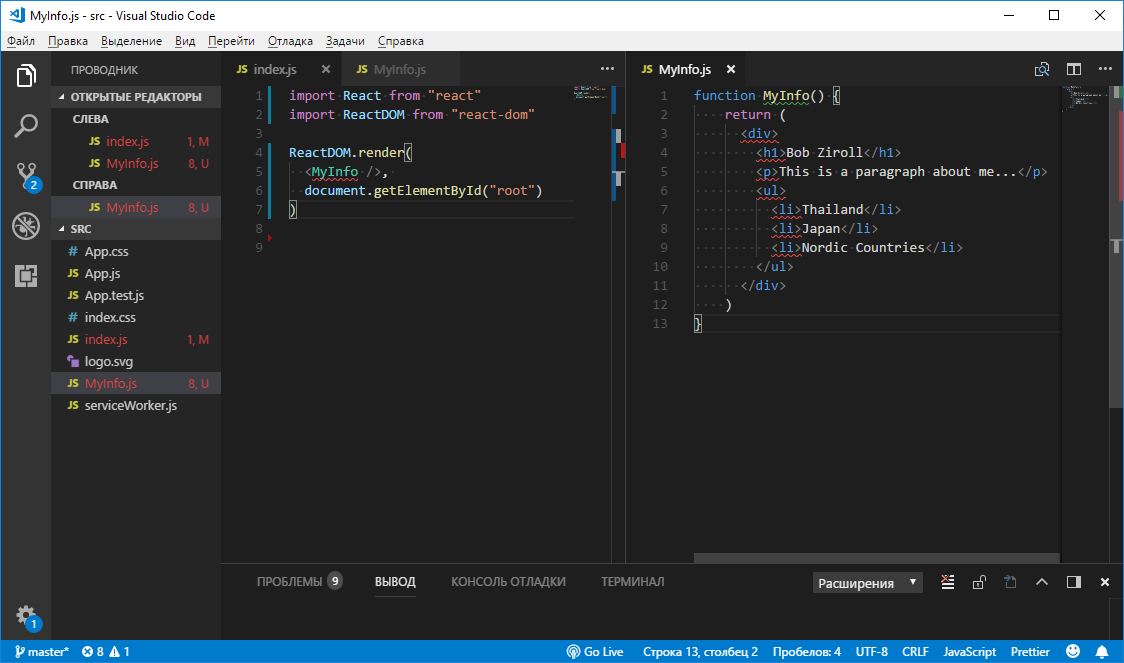
Transferring the component code to a new file
The component code
index.js
we transferred from, but the structure we got now is not working yet. First of all, remember what the
index.js
team is forimport React from "react"
, even considering that weReact
do notdirectly contact ushere. The reason for this is that without import theReact
mechanisms of this library will not work, in particular - JSX. Thanks to this import command, we could, in previous lessons, pass theReactDOM.render()
JSX-codeto the methodand output the HTML markup created on its basis to the page. All this means that in the fileMyInfo.js
we also need to import React. This is a common practice for component files. Secondly, we need to make the function
MyInfo
from the fileMyInfo.js
could be used in other application files. It needs to be exported. ES6 standard features are used here. As a result, the updated code MyInfo.js
takes the following form:import React from"react"functionMyInfo() {
return (
<div>
<h1>Bob Ziroll</h1>
<p>This is a paragraph about me...</p>
<ul>
<li>Thailand</li>
<li>Japan</li>
<li>Nordic Countries</li>
</ul>
</div>
)
}
exportdefault MyInfo
Now let's work on the file
index.js
. Namely, we need the component MyInfo
to be available in this file. You can make it available by index.js
importing it. What if you try to write down the component import command based on the import command
react
and react-dom
in index.js
? For example, add the following import command to the file:import MyInfo from"MyInfo.js"// неправильно
The system, seeing such a command, in particular, based on the fact that it lacks information about the relative path to the file, will look for a project dependency - a module with the name specified when calling this command ( here's how to install dependencies in projects created by means
create-react-app
; dependencies can then be imported into React projects in the same way as the React library was imported). It will not find such a module, as a result the import command will not work. Therefore, the file import command must be rewritten with the path to it. In this case, we are satisfied with the indication of the current directory ( ./
) and the import command will take the following form:import MyInfo from"./MyInfo.js"// правильно
In addition, if we talk about the team
import
, it is important to bear in mind that it implies that JavaScript files are imported with its help. That is, the extension .js
is quite possible to remove, and the team import
, acquiring the form shown below, will not lose its working capacity.import MyInfo from"./MyInfo"// правильно
Usually, such JS file import commands are written this way.
Here is the complete file code
index.js
.import React from"react"import ReactDOM from"react-dom"import MyInfo from"./MyInfo"
ReactDOM.render(
<MyInfo />,
document.getElementById("root")
)
ПроектаProject structure
With the growth of the size and complexity of React-projects it is very important to maintain their structure in good condition. In our case, although our project is now small, it is possible, in a folder
src
, to create a folder components
for storing files with a component code. Create such a folder and move the file into it
MyInfo.js
. After that you will need to edit the import command of this file in index.js
. Namely, now the path to
MyInfo.js
indicates that this file is located in the same place where index.js
, but in fact this file is now in a folder components
located in the same folder as index.js
. As a result, the relative path to it index.js
will look like ./components/MyInfo
. This will be the updated code index.js
:import React from"react"import ReactDOM from"react-dom"import MyInfo from"./components/MyInfo"
ReactDOM.render(
<MyInfo />,
document.getElementById("root")
)
And this is how it all looks in VSCode.
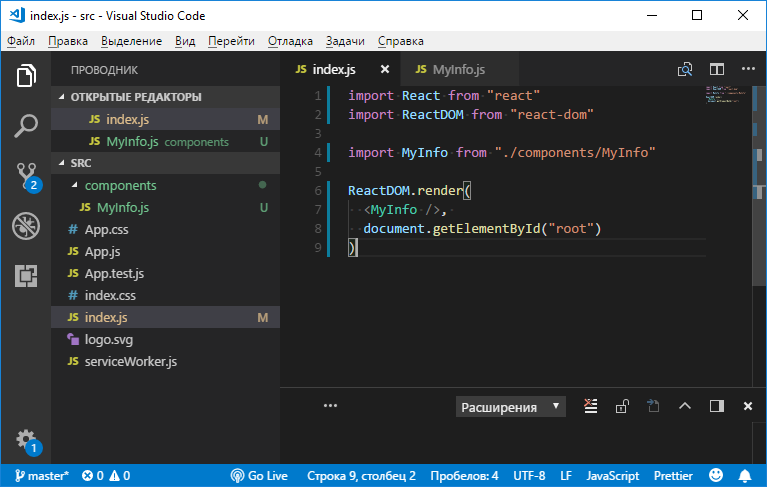
Folder for storing components and importing a component from this folder into VSCode
In fact, one folder
components
intended for placing the code of all components is an example of an extremely simplified project structure. In real projects, to provide the convenience of working with a large number of entities, much more complex folder structures are used. What exactly these structures will be depends on the needs of the project and on the personal preferences of the programmer. It is recommended to experiment with everything that you have learned today. For example, you can try to move a file.
MyInfo.js
in any folder and see what comes of it, you can try to rename it, change some code in it. When in the course of such experiments, the correct work of the project will be disrupted - it will be useful to understand the problem and bring the project back to working condition.Results
In this lesson, we talked about the design of the component code as separate files, about exporting and importing code using ES6 tools, and about the structure of React projects. Next time we will continue to get acquainted with the capabilities of the components.
Dear readers! We ask experienced React-developers to share with newbies ideas about the organization of the project structure.
