React Tutorial, Part 2: Functional Components
- Transfer
- Tutorial
In one of our previous materials we asked you whether it would be advisable to make a series of traditional publications on the basis of this course on React. You supported our idea. Therefore, today we present to your attention the continuation of the course. Here we talk about the functional components.
→ Part 1: course overview, reasons for the popularity of React, ReactDOM and JSX
→ Part 2: functional components
→ Part 3: component files, project structure
→ Part 4: parent and child components
→ Part 5: starting work on a TODO application, basics of styling
→ Part 6: Some features of the course, JSX and JavaScript
→
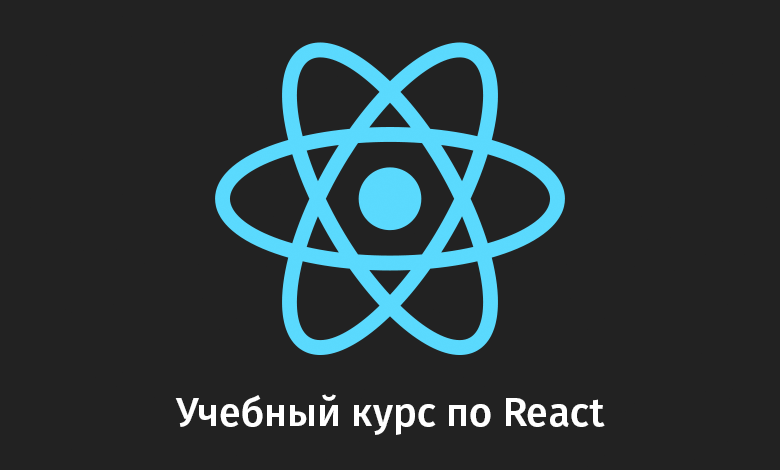
Part 7: Built-in Styles
→ Part 8: Continuing Work on a TODO Application, Familiarity with Component Properties
→ Part 9: Component Properties
→ Part 10: A Workshop on Working with Component Properties and Styling
→ Part 11: Dynamic Layout Formation and the Array Method map
→ Part 12: workshop, third stage of work on the TODO application
→ Part 13: class-based components
→ Part 14: workshop on class-based components, component condition
→ Part 15: workshops on working with the state of components
→ Part 16: fourth tap work on TODO-application event handling
→ Part 17: the fifth stage of working on a TODO application, modifying the state of components
→ Part 18: the sixth stage of working on a TODO application
→ Part 19: methods of the component life cycle
→ Part 20: the first lesson on conditional rendering
→ Part 21: the second lesson and Workshop on conditional rendering
→ Part 22: the seventh stage of working on a TODO application, loading data from external sources
→ Part 23: the first session on working with forms
→ Part 24: the second session on working with forms
→ Part 25: a workshop on working with forms
→ Part 26: Application Architecture , Container / Component pattern
→ Part 27: course project
→ Original
At the previous practical lesson, we talked about the fact that it is not necessary to place all the JSX-code that forms the HTML elements in the argument of the method
Imagine that you need to output, using the same approach, a whole web page with hundreds of elements. If you do this, then normally it will be almost impossible to maintain such code. When we talked about the reasons for the popularity of React, one of them was the support of this library of components suitable for reuse. Now we will talk about how to create functional components of React.
These components are called "functional" because they are created by constructing special functions.
Create a new function and give it a name
The name of the function is made exactly for the reason that it uses the naming scheme of function constructors. Their names (in fact - the names of the components) are written in the camel style - the first letters of the words they are made of are capitalized, including the first letter of the first word. You should strictly adhere to this naming convention for such functions.
The functional components are fairly simple. Namely, there should be a command in the function body that returns the JSX code that represents the corresponding component.
In our example, it is enough to take the code of the bulleted list and arrange the return of this code from the functional component. Here is what it might look like:
And although in this case everything will work as expected, that is, the team
With this approach, the markup returned from the component turns out to be very similar to ordinary HTML code.
Now, in the method
You may notice that a self-closing tag is used here. In some cases, when it is necessary to create components that have a more complex structure, such constructions are constructed differently, but for the time being we will use such self-closing tags.
If you update the page formed by the above code, then its appearance will be the same as it was before moving the marked list to the functional component.
The markup that the functional components return is subject to the same rules that we considered when applied to the first parameter of the method
Perhaps you have already begun to feel the power of using functional components. In particular, we are talking about creating your own components that contain fragments of JSX code, which is a description of the HTML markup that will appear on the web page. Such components can be linked together.
In our example, there is a component that displays a simple HTML list, but as we create more and more complex applications, we will develop components that derive the other components we have created. As a result, all this will turn into ordinary HTML elements, but sometimes it will be necessary to form dozens of your own components to form these elements.
As a result, when we will create more and more components, we will place them in separate files, but for now it is important for you to master what we have just discussed, to get used to the functional components. During the course, you will create more and more complex file structures.
In this lesson, we sorted out the basics of functional components, and in the following we apply the knowledge gained in practice.
→ Original
Stylize page elements by learning how to do it yourself (look in Google). It should be noted that we will talk more about styling components in this course.
Note: roll block
Here we are satisfied with the same HTML page that we used earlier. A file with a React-code will also look quite standard. Namely, we import libraries into it, create a skeleton of a functional component
Now you need to return from the
Notice that the structure returned from
In this article, we learned about the functional components of React. Next time we will talk about the component files and the structure of React-projects.
Dear readers! If you are taking this course, please tell us about the environment in which you are doing the exercises.

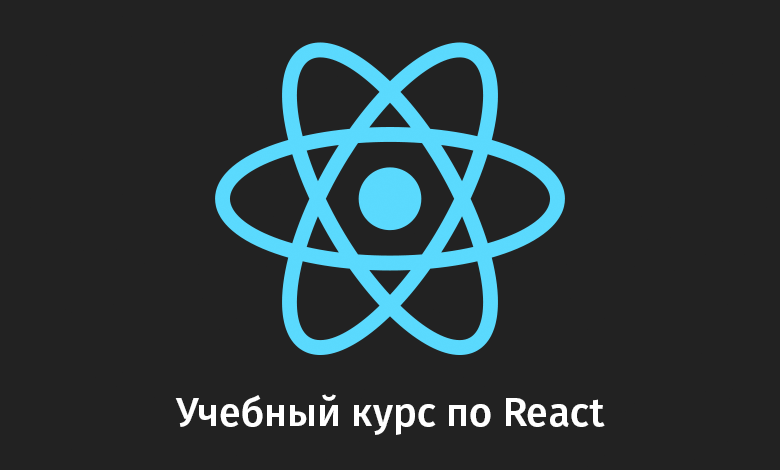
Part 7: Built-in Styles
→ Part 8: Continuing Work on a TODO Application, Familiarity with Component Properties
→ Part 9: Component Properties
→ Part 10: A Workshop on Working with Component Properties and Styling
→ Part 11: Dynamic Layout Formation and the Array Method map
→ Part 12: workshop, third stage of work on the TODO application
→ Part 13: class-based components
→ Part 14: workshop on class-based components, component condition
→ Part 15: workshops on working with the state of components
→ Part 16: fourth tap work on TODO-application event handling
→ Part 17: the fifth stage of working on a TODO application, modifying the state of components
→ Part 18: the sixth stage of working on a TODO application
→ Part 19: methods of the component life cycle
→ Part 20: the first lesson on conditional rendering
→ Part 21: the second lesson and Workshop on conditional rendering
→ Part 22: the seventh stage of working on a TODO application, loading data from external sources
→ Part 23: the first session on working with forms
→ Part 24: the second session on working with forms
→ Part 25: a workshop on working with forms
→ Part 26: Application Architecture , Container / Component pattern
→ Part 27: course project
Lesson 6: Functional Components
→ Original
At the previous practical lesson, we talked about the fact that it is not necessary to place all the JSX-code that forms the HTML elements in the argument of the method
ReactDOM.render()
. In our case, we are talking about a bulleted list, one that is described below.import React from"react"import ReactDOM from"react-dom"
ReactDOM.render(
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>,
document.getElementById("root")
)
Imagine that you need to output, using the same approach, a whole web page with hundreds of elements. If you do this, then normally it will be almost impossible to maintain such code. When we talked about the reasons for the popularity of React, one of them was the support of this library of components suitable for reuse. Now we will talk about how to create functional components of React.
These components are called "functional" because they are created by constructing special functions.
Create a new function and give it a name
MyApp
:import React from"react"import ReactDOM from"react-dom"functionMyApp() {
}
ReactDOM.render(
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>,
document.getElementById("root")
)
The name of the function is made exactly for the reason that it uses the naming scheme of function constructors. Their names (in fact - the names of the components) are written in the camel style - the first letters of the words they are made of are capitalized, including the first letter of the first word. You should strictly adhere to this naming convention for such functions.
The functional components are fairly simple. Namely, there should be a command in the function body that returns the JSX code that represents the corresponding component.
In our example, it is enough to take the code of the bulleted list and arrange the return of this code from the functional component. Here is what it might look like:
functionMyApp() {
return<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>
}
And although in this case everything will work as expected, that is, the team
return
will return all this code, it is recommended to conclude similar constructions in parentheses and apply another agreement adopted in React when formatting the program code. It lies in the fact that the individual elements are placed on separate lines and aligned accordingly. As a result of applying the above ideas, the code of our functional component will look like this:functionMyApp() {
return (
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>
)
}
With this approach, the markup returned from the component turns out to be very similar to ordinary HTML code.
Now, in the method
ReactDOM.render()
, you can create an instance of our functional component, passing it to this method as the first argument and wrapping it in a JSX tag.import React from"react"import ReactDOM from"react-dom"functionMyApp() {
return (
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>
)
}
ReactDOM.render(
<MyApp />,
document.getElementById("root")
)
You may notice that a self-closing tag is used here. In some cases, when it is necessary to create components that have a more complex structure, such constructions are constructed differently, but for the time being we will use such self-closing tags.
If you update the page formed by the above code, then its appearance will be the same as it was before moving the marked list to the functional component.
The markup that the functional components return is subject to the same rules that we considered when applied to the first parameter of the method
ReactDOM.render()
. That is - it is impossible for JSX elements to be present in it, following each other. Attempt to place in the previous example after the element <ul>
any other element, say -<ol>
, will lead to an error. You can avoid this problem, for example, by simply wrapping everything that returns a functional component into an element <div>
. Perhaps you have already begun to feel the power of using functional components. In particular, we are talking about creating your own components that contain fragments of JSX code, which is a description of the HTML markup that will appear on the web page. Such components can be linked together.
In our example, there is a component that displays a simple HTML list, but as we create more and more complex applications, we will develop components that derive the other components we have created. As a result, all this will turn into ordinary HTML elements, but sometimes it will be necessary to form dozens of your own components to form these elements.
As a result, when we will create more and more components, we will place them in separate files, but for now it is important for you to master what we have just discussed, to get used to the functional components. During the course, you will create more and more complex file structures.
In this lesson, we sorted out the basics of functional components, and in the following we apply the knowledge gained in practice.
Session 7. Workshop. Functional components
→ Original
▍Job
- Prepare a basic React project.
- Create a functional component
MyInfo
that generates the following HTML elements:- An element
<h1>
with your name. - A text paragraph (element
<p>
) containing your short story about yourself. - List marked (
<ul>
) or numbered (<ol>
), with a list of three places that you would like to visit.
- An element
- Bring an instance of the component
MyInfo
to a web page.
▍Advanced task
Stylize page elements by learning how to do it yourself (look in Google). It should be noted that we will talk more about styling components in this course.
Note: roll block
▍Decision
Here we are satisfied with the same HTML page that we used earlier. A file with a React-code will also look quite standard. Namely, we import libraries into it, create a skeleton of a functional component
MyInfo
and call the render()
object method ReactDOM
, passing it the component to be output to the page, and a link to the element of the page in which this component should be displayed. At this stage, the code will look like this:import React from"react"import ReactDOM from"react-dom"functionMyInfo() {
}
ReactDOM.render(<MyInfo />, document.getElementById("root"))
Now you need to return from the
MyInfo
JSX-code that forms the HTML markup in accordance with the task. Here is the complete solution code.import React from"react"import ReactDOM from"react-dom"functionMyInfo() {
return (
<div>
<h1>Bob Ziroll</h1>
<p>This is a paragraph about me...</p>
<ul>
<li>Thailand</li>
<li>Japan</li>
<li>Nordic Countries</li>
</ul>
</div>
)
}
ReactDOM.render(
<MyInfo />,
document.getElementById("root")
)
Notice that the structure returned from
MyInfo
is enclosed in parentheses, and that the elements to be displayed are inside the auxiliary element <div>
.Results
In this article, we learned about the functional components of React. Next time we will talk about the component files and the structure of React-projects.
Dear readers! If you are taking this course, please tell us about the environment in which you are doing the exercises.
