Ultimate ++ Impressions
If you are a freelancer or like to experiment with exotic C ++ environments, then you should pay attention to the Ultimate ++ ecosystem , which I learned recently through a series of articles by Semyon Esilevsky ( part 1 , part 2 , part 3 , part 4 - everything is on the wiki ) with such a final parting word:
Under the cut are those U ++ nuances that we managed to unearth on the forum and in the manuals for the month of daily work on an edited directory of documents stored in the database. I ’ll note right away that there will be no comparison with the “wonderful trinity”, because earlier for the simplest gui I used WTL and looked with hope at the eGUI ++ library , which, unfortunately, the author abandoned, but there was no one to pick up.
First of all, Ultimate ++ is an ultimatum: either TheIDE’s own environment, or, for example, such a set under Windows as Visual Studio + STL + POCO + WTL. As a result, you have to forget about exceptions, although the rest is decent, which is illustrated by fragments of the asynchronous client-server file transfer code I wrote.
Just look here and here . Php / Yii fans should appreciate it.
QTF is a native U ++ format for advanced text formatting. Used in RichEdit and in generating reports. TheIDE has a special designer for experiments:
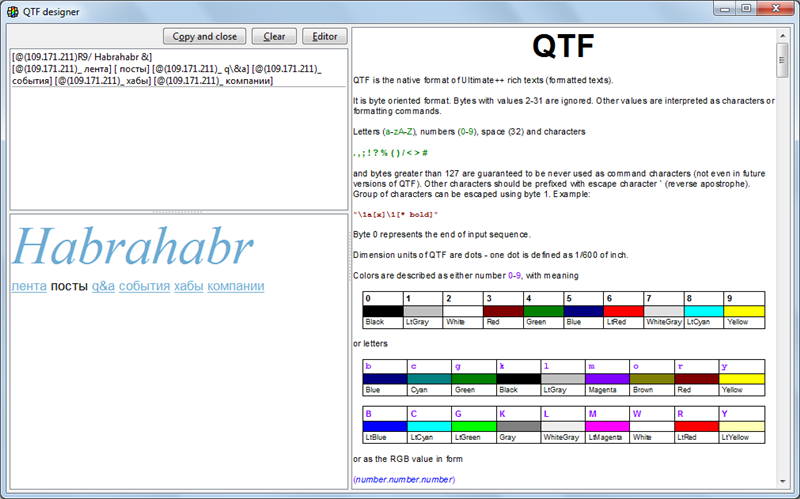
There is also a special dialog for printing a report (saving in pdf is in the bowels of ReportWindow):

The style of the text is framed by tags [and], and first, behind the bracket [are the QTF identifiers, and then only through the space text. In general, the principle is like in HTML, the difference is only in the names.
Bazar is a set of user libraries for specific needs, among which the cross-platform work with Word / Excel in Office Automation was very useful to me :
Join in! Many points that I did not write out require active improvement. 32 mb with a small distribution deserve it.
“Do the benefits of U ++ outweigh its unusualness and high“ entry barrier ”? In my opinion, yes. U ++ is an excellent choice for cross-platform projects that are developed from scratch, especially if heavy use of databases is expected. ”
Under the cut are those U ++ nuances that we managed to unearth on the forum and in the manuals for the month of daily work on an edited directory of documents stored in the database. I ’ll note right away that there will be no comparison with the “wonderful trinity”, because earlier for the simplest gui I used WTL and looked with hope at the eGUI ++ library , which, unfortunately, the author abandoned, but there was no one to pick up.
First of all, Ultimate ++ is an ultimatum: either TheIDE’s own environment, or, for example, such a set under Windows as Visual Studio + STL + POCO + WTL. As a result, you have to forget about exceptions, although the rest is decent, which is illustrated by fragments of the asynchronous client-server file transfer code I wrote.
Core
- Delegates (the main tool for binding events in the GUI; personally, they hooked me the most)
//ограничения: //функция не должна возвращать значений, а также иметь больше 4х входных параметров void Func() {}; void Func4(int, double, String, Value) {} //делегаты для функций GUI_APP_MAIN { Callback cb = callback(Func); cb(); //stateful Callback cb4 = callback4(Func4, 1, 2, AsString(3), 4); cb4(); //stateless Callback4
cb1 = callback(Func4); cb1(1, 2, AsString(3), 4); } //делегаты в классе class AppMain { public: //для THISBACK typedef AppMain CLASSNAME; Callback cb; Callback cb4; AppMain() { cb = THISBACK(Func); cb4 = THISBACK4(Func4, 1, 2, AsString(3), 4); } void Func() {}; void Func4(int, double, String, Value) {} }; //делегаты для объектов GUI_APP_MAIN { AppMain m; m.cb = callback(&m, &AppMain::Func); m.cb4 = callback4(&m, &AppMain::Func4, 1, 2, AsString(3), 4); } - Singleton
//класс SomeClass должен иметь конструктор по-умолчанию Single
().SomeMethod(); - Logging (keep in mind that for real numbers,% f is used instead of% lf)
//вывод на экран и в файл StdLogSetup(LOG_COUT|LOG_FILE); ... LOG(Format("Total %d files have sended\n", n)); LOG("Total " << n << " files have sended");
- Ini files
VectorMap
config = LoadIniFile(GetExeDirFile("config.ini")); String host = config.Get("HOST"); int port = ScanInt(config.Get("PORT")); for (int i = 0; i < config.GetCount(); i++) { if ("FILE" == config.GetKey(i)) { Cout() << config[i] << "\n"; } } - Multithreading
//без ожидания завершения Thread::Start(callback(Func)); //c ожиданием завершения Thread th; th.Run(callback(Func)); th.Wait(); Thread::ShutdownThreads();
As I understand it, OpenMP is not supported; instead, CoWork , working with delegates, is suggested , which is not convenient for parallelizing loops. - Text JSON (binary data is supported by a pair of Base64Encode / Base64Decode functions)
FileIn fp(fname); Json json; json("fname", fname)("fsize", (int)fp.GetSize())("fdata", Base64Encode(LoadFile(fname))); ValueArray jsonAr = ParseJSON(json.ToString()); fname = jsonAr[0]; int fsize = jsonAr[1]; String fdata = Base64Decode(jsonAr[2]);
- Sockets
TcpSocket server; if (!server.Listen(port)) { LOG(Format("Can't open server port %d for listening\n", port)); return; } for(;;) { LOG("Waiting..."); TcpSocket socket; if (socket.Accept(server)) { String msg = ""; for (int c = socket.Get(); c > 0 && c != '\n'; c = socket.Get()) { msg.Cat(c); } } }
- RegExp (PCRE)
RegExp reg("(\\\\)"); String path = "D:\\test.txt"; if (reg.Match(path)) { int last; int first; reg.GetMatchPos(0, first, last); String drive = path.Mid(0, last); String fname = path.Mid(last); }
- Built-in memory leak check (malloc is not tracked)
GUI_APP_MAIN { double *d = new double(0); }
SQL
Just look here and here . Php / Yii fans should appreciate it.
QTF (ReportView)
QTF is a native U ++ format for advanced text formatting. Used in RichEdit and in generating reports. TheIDE has a special designer for experiments:
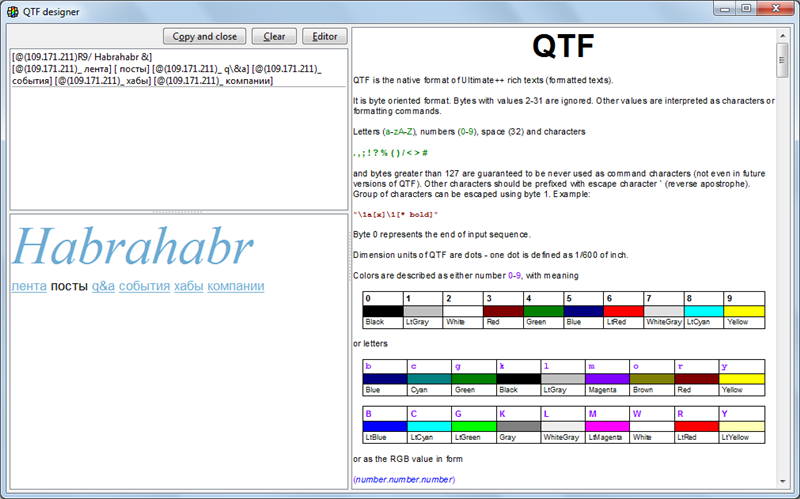
There is also a special dialog for printing a report (saving in pdf is in the bowels of ReportWindow):
Color rgb_color = Color(109, 171, 211);
String qtf_color = Format("@(%d.%d.%d)", rgb_color.GetR(), rgb_color.GetG(), rgb_color.GetB());
String qtf;
qtf.Cat(Format("[R9/%s Habrahabr &]", qtf_color));
qtf.Cat(Format("[_%s лента] [ посты] [_%s q\\&a] [_%s события] [_%s хабы] [_%s компании]",
qtf_color, qtf_color, qtf_color, qtf_color, qtf_color));
Report rep;
rep << qtf;
ReportWindow().Perform(rep);

The style of the text is framed by tags [and], and first, behind the bracket [are the QTF identifiers, and then only through the space text. In general, the principle is like in HTML, the difference is only in the names.
Bazaar
Bazar is a set of user libraries for specific needs, among which the cross-platform work with Word / Excel in Office Automation was very useful to me :
#include
GUI_APP_MAIN
{
//Excel
OfficeSheet sheet;
bool xlsOn = sheet.IsAvailable("Microsoft");
if (xlsOn)
sheet.Init("Microsoft");
//Open Office Calc
if (!xlsOn) {
xlsOn = sheet.IsAvailable("Open");
if (xlsOn)
sheet.Init("Open");
}
if (xlsOn) {
FileSel fs;
fs.Type("Файлы таблиц", "*.xls *.xlsx");
fs.AllFilesType();
if (fs.ExecuteOpen("Выберите Excel файл")) {
sheet.OpenSheet(~fs, true);
sheet.AddSheet(true);
}
}
//Word
OfficeDoc doc;
//Если установлен Word2003 и Word2007, открывается почему-то Word2003. Разработчик разводит руками.
bool docOn = doc.IsAvailable("Microsoft");
if (docOn)
doc.Init("Microsoft");
//Open Office Writer
if (!docOn) {
docOn = doc.IsAvailable("Open");
if (docOn)
doc.Init("Open");
}
if (docOn) {
FileSel fs;
fs.Type("Файлы Word", "*.doc *.docx *.rtf");
fs.AllFilesType();
if (fs.ExecuteOpen("Выберите Word файл")) {
doc.OpenDoc(~fs, true);
doc.AddDoc(true);
}
}
}
Nuances
- The Russian-language file selection dialog does not translate subfolders, even if Windows itself is Russified:
SetLanguage(SetLNGCharset(GetSystemLNG(), CHARSET_UTF8));
- If you look at examples of real applications , you can see that there are no screenshots with icons and text in the menu, because this cannot be done - icons with text can only have sub-sections of the menu, and the main menu is always text.
- Buttons do not have default properties for resizing.
- Convenient block line shift of lines through Alt + Shift + stitches + tab is not supported, which is annoying after Visual Studio and Notepad ++.
- The biggest problem the editor does not always correctly cancel the last operation. It manifests itself in two ways: with manual cancellation (or Ctrl + Z), adjacent and not only lines can begin to mix (it is caught once or twice); when launched through F5 or Ctrl + F5, it is canceled, again sometimes, the last edit (happens more often).
- There are problems with Intellisense, which is more likely to display the available signatures of an existing variable in the code than the one just written. I would also like to recognize situations when, when calling a procedure from an object from a list, the code
obj.f()
would not transform intoobj.f() ()
Summary
Join in! Many points that I did not write out require active improvement. 32 mb with a small distribution deserve it.