Imba: JavaScript-compatible language for quick work with DOM
- Transfer
Imba is an open source programming language created by Scrimba specifically for developing web applications. It compiles into JavaScript and is able to work inside an existing JS ecosystem. It is, for example, about Node.js, about npm, about Webpack.
Imba’s main strength is that it will allow you to create much faster applications than those based on libraries using Virtual DOM technology, like React and Vue. Application performance increases due to how Imba handles DOM updates. Details about this can be found here .

The author of this material, the co-founder of the Scrimba project, says that Imba has been using it for several years. According to him, writing in this language is a real pleasure, since it, in comparison with JavaScript, has a cleaner syntax, which improves the readability of the code.
This material is an Imba Beginner's Guide, which will enable you to start creating simple applications. First, the basics of the language will be revealed here, then the development of user interfaces with its help will be considered. The setup of the development environment for programming on Imba will also be shown here.
Before we go into the code, I would like to draw your attention to the fact that Imba is not something like another strange language compiled in JS and used exclusively in amateur projects. It is used in serious applications of large companies.
One example of its use is a platform supporting a fish auction in Iceland. In this country, fish trade accounts for about 1.6% of GDP, which is about $ 390 million.

Icelandic Fish Auction
Another example is the learning platform Scrimba.com , where Imba is used on the client and server parts of the project. The convenience of working with this platform, which is based on a complex application, depends heavily on Imba's ability to quickly synchronize changes to DOM.

Platform Scrimba.com
As a result, we can say that the language with which you will get acquainted today is suitable for developing projects of various scales.
Imba syntax is a lot like javascript, but other languages such as Ruby and Python also influenced it. Perhaps the features of syntax Imba will be convenient to consider an example. The following is a simple JS function that returns the largest of the two numbers passed to it, or, if these numbers are equal, a value
Now let's write the same on Imba.
Perhaps comparing these two examples, you can immediately see the differences between Imba and JavaScript. Namely, they are as follows:
This is not to say that the above syntax features are the most important aspect of Imba, but they make the code more concise than similar code written in JavaScript. This advantage will become more noticeable as we move through the material.
Let's talk about creating user interfaces using Imba. Strictly speaking, this language was created precisely for this. In particular, this means that the DOM nodes are built into the language in the form of so-called "first-class objects."
If you have experience with React development, you can consider this feature of Imba as if Imba has its own version of JSX built into the language.
Consider the following code, in which the React library is used to render a button and to display a message in the console when this button is clicked.
If this is rewritten on Imba, then we get the following.
Let's compare these two code fragments. Namely, pay attention to the following three features:
You may have noticed the features of event handlers in Imba. Namely, we connect the corresponding handler to the button using the construction
Now let's talk about working with data in Imba. The following example shows a React application, in the component state of
Here's how the same will look on Imba.
The first thing that catches your eye when comparing these two examples is the difference in code size.
The example on Imba is about two times shorter - both in the number of lines, and in the amount of code.
Although comparing the number of lines of code in the comparison of programming languages is not so important, it nevertheless affects the readability of the code, which, on the scale of a certain code base, already plays a certain role. Less code on Imba means better readability than React.
You may have noticed that in the above example, we are accessing an object instance variable directly, mentioning only its name
In our example on Imba, it would be possible to use the view construct
Another major difference between the two previous examples is how the state change is implemented in them. In the example written in Imba, the state is mutable, which allows changing the value of a variable
React uses a different approach in which the value is
If you prefer to work with an immune state, you can use the corresponding library with Imba. Imba language in this sense is not tied to any particular solution. We, in the Scrimba project, use the mutable state, since we believe that the unnecessary expenditure of system resources necessary to ensure immunity does not need us.
Now that you've learned the basics of Imba, it's time to experiment. To do this you will need to set up a development environment. In order to do this, it suffices to run the following commands.
After that, go to the browser at the address
If the local installation of Imba does not suit you, you can use the interactive online sandbox of the Scrimba project.
Let's talk about the performance of applications written on Imba. The reason Imba allows you to create extremely fast web applications is that this language does not use the concept of Virtual DOM, which has become very popular with the introduction of React. Imba uses Memoized DOM technology. It is simpler than the Virtual DOM, and uses fewer intermediate mechanisms for working with the DOM.
In this benchmark, which you can run at your place by simply pressing a button
In the test, the results of which are shown below, it turned out that Imba is 20-30 times faster than React and Vue. On different computers, test results will vary.
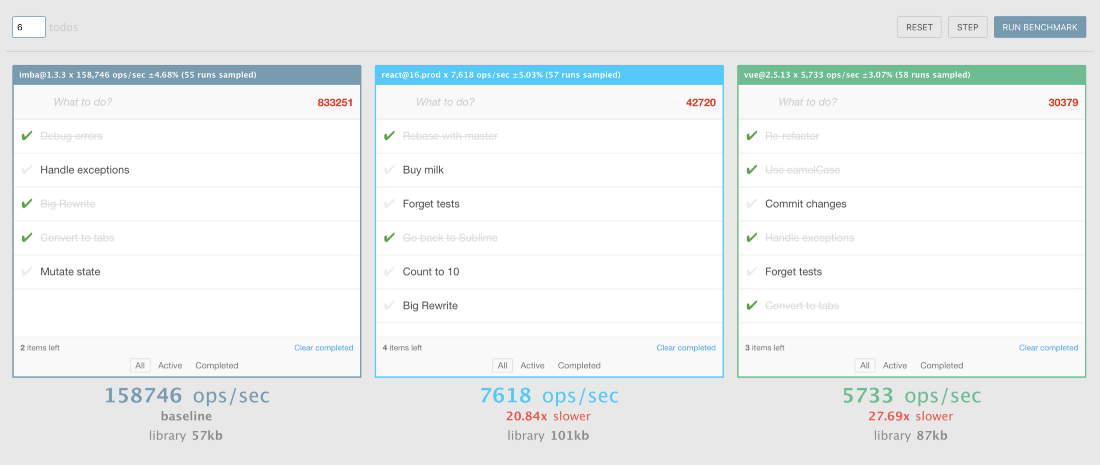
Performance results Imba, the React and Vue
As you can see, the use of Imba means that you can very quickly work with the DOM.
In this article, we looked only at the very basics of Imba. If you are interested in this language, check out its documentation . In particular, it will be useful to master his concept of using getters and setters and the mechanism of implicit calls. Here is a list of helpful resources related to Imba. It should be noted that at first this language may seem difficult, it will take some time to master it, but, as everyone knows, you cannot easily pull a fish out of the pond.
Dear readers! Do you plan to use the Imba language in your projects?

Imba’s main strength is that it will allow you to create much faster applications than those based on libraries using Virtual DOM technology, like React and Vue. Application performance increases due to how Imba handles DOM updates. Details about this can be found here .

The author of this material, the co-founder of the Scrimba project, says that Imba has been using it for several years. According to him, writing in this language is a real pleasure, since it, in comparison with JavaScript, has a cleaner syntax, which improves the readability of the code.
This material is an Imba Beginner's Guide, which will enable you to start creating simple applications. First, the basics of the language will be revealed here, then the development of user interfaces with its help will be considered. The setup of the development environment for programming on Imba will also be shown here.
About projects that use imba
Before we go into the code, I would like to draw your attention to the fact that Imba is not something like another strange language compiled in JS and used exclusively in amateur projects. It is used in serious applications of large companies.
One example of its use is a platform supporting a fish auction in Iceland. In this country, fish trade accounts for about 1.6% of GDP, which is about $ 390 million.

Icelandic Fish Auction
Another example is the learning platform Scrimba.com , where Imba is used on the client and server parts of the project. The convenience of working with this platform, which is based on a complex application, depends heavily on Imba's ability to quickly synchronize changes to DOM.

Platform Scrimba.com
As a result, we can say that the language with which you will get acquainted today is suitable for developing projects of various scales.
Syntax
Imba syntax is a lot like javascript, but other languages such as Ruby and Python also influenced it. Perhaps the features of syntax Imba will be convenient to consider an example. The following is a simple JS function that returns the largest of the two numbers passed to it, or, if these numbers are equal, a value
false
.functionfindGreatest(num1, num2) {
if (num1 > num2) {
return num1
} elseif (num2 > num1){
return num2
} else {
returnfalse
}
}
Now let's write the same on Imba.
def findGreatest num1, num2
if num1 > num2
num1
elif num2 > num1
num2
else
false
Perhaps comparing these two examples, you can immediately see the differences between Imba and JavaScript. Namely, they are as follows:
function
turns intodef
. Keywordfunction
replaced by keyworddef
.- Lack of brackets. Function parameters are not enclosed in brackets. In Imba, in fact, brackets are rarely needed, although you can use them if you like.
- Indentation. Indentations play a very important role in Imba. This means that braces are not needed here.
- Missing keyword
return
. In Imba, returning values from functions is performed implicitly, that is, there isreturn
no need in the keyword . Imba automatically returns the last function expression.
This is not to say that the above syntax features are the most important aspect of Imba, but they make the code more concise than similar code written in JavaScript. This advantage will become more noticeable as we move through the material.
User Interface Design
Let's talk about creating user interfaces using Imba. Strictly speaking, this language was created precisely for this. In particular, this means that the DOM nodes are built into the language in the form of so-called "first-class objects."
If you have experience with React development, you can consider this feature of Imba as if Imba has its own version of JSX built into the language.
Consider the following code, in which the React library is used to render a button and to display a message in the console when this button is clicked.
classAppextendsReact.Component{
logOut() {
console.log('button clicked!')
}
render() {
return (
<divclassName="container">
<buttononClick={this.logOut}>click me!</button>
</div>
)
}
}
If this is rewritten on Imba, then we get the following.
tag App
def logOut e
log 'button clicked!'
def render
<self.container>
<button :tap.logOut> 'Click me!'
Imba.mount <App>
Let's compare these two code fragments. Namely, pay attention to the following three features:
- Inline tags. Note that the view construct has
class App extends React.Component
been converted to a much simpler viewtag App
. The keyword istag
embedded in the language. DOM tags are also embedded. - No closing tags. Since the alignment of lines determines the structure of the program, it is
</button>
not necessary to close tags (for example, using a tag ). This speeds up the input of the program text and reduces its size. - Simple class syntax. Imba simplifies working with HTML classes. Namely, instead of a bulky construction,
className="container"
it suffices to add.container
to the tag itself.
You may have noticed the features of event handlers in Imba. Namely, we connect the corresponding handler to the button using the construction
:tap.logOut
used instead onClick={this.logOut}
. This is just one of several ways to handle user input. Details about this can be found here .Work with data
Now let's talk about working with data in Imba. The following example shows a React application, in the component state of
App
which there is a variable count
equal to 0. The value of this variable decreases or increases when you click on the corresponding buttons.classAppextendsReact.Component{
state = {
count: 0
}
increase = () => {
this.setState({
count: this.state.count + 1
})
}
decrease = () => {
this.setState({
count: this.state.count - 1
})
}
render() {
return (
<divclassName="container">
<buttononClick={this.increase}>Increase!</button>
<buttononClick={this.decrease}>Decrease!</button>
<p>{this.state.count}</p>
</div>
)
}
}
Here's how the same will look on Imba.
tag App
prop count default: 0
def increase
count += 1
def decrease
count -= 1
def render
<self.container>
<button :tap.increase> 'Increase!'
<button :tap.decrease> 'Decrease!'
<p> count
Imba.mount <App>
The first thing that catches your eye when comparing these two examples is the difference in code size.
The example on Imba is about two times shorter - both in the number of lines, and in the amount of code.
Although comparing the number of lines of code in the comparison of programming languages is not so important, it nevertheless affects the readability of the code, which, on the scale of a certain code base, already plays a certain role. Less code on Imba means better readability than React.
Implicit self call
You may have noticed that in the above example, we are accessing an object instance variable directly, mentioning only its name
count
. In React, the same thing is done using the construct this.state.count
. In our example on Imba, it would be possible to use the view construct
self.count
, however, self
it is implicitly referred to here, so it is self
not necessary to indicate . Imba, when accessing count
, finds out whether there is such a variable either in scope, or at the App instance itself.Mutability
Another major difference between the two previous examples is how the state change is implemented in them. In the example written in Imba, the state is mutable, which allows changing the value of a variable
count
directly. React uses a different approach in which the value is
this.state
considered to be immutable, with the result that the only way to change it is to use it this.setState
. If you prefer to work with an immune state, you can use the corresponding library with Imba. Imba language in this sense is not tied to any particular solution. We, in the Scrimba project, use the mutable state, since we believe that the unnecessary expenditure of system resources necessary to ensure immunity does not need us.
Setting up the development environment
Now that you've learned the basics of Imba, it's time to experiment. To do this you will need to set up a development environment. In order to do this, it suffices to run the following commands.
git clone https://github.com/somebee/hello-world-imba.git
cd hello-world-imba
npm install
npm run dev
After that, go to the browser at the address
http://localhost:8080/
and you will see the main page of the project. In order to modify the application - edit the file that can be found at src/client.imba
. If the local installation of Imba does not suit you, you can use the interactive online sandbox of the Scrimba project.
Imba performance
Let's talk about the performance of applications written on Imba. The reason Imba allows you to create extremely fast web applications is that this language does not use the concept of Virtual DOM, which has become very popular with the introduction of React. Imba uses Memoized DOM technology. It is simpler than the Virtual DOM, and uses fewer intermediate mechanisms for working with the DOM.
In this benchmark, which you can run at your place by simply pressing a button
RUN BENCHMARK
on its page, you can compare the number of operations with the DOM, which you can perform in a second in the process of modifying the TODO list using Imba, React and Vue.In the test, the results of which are shown below, it turned out that Imba is 20-30 times faster than React and Vue. On different computers, test results will vary.
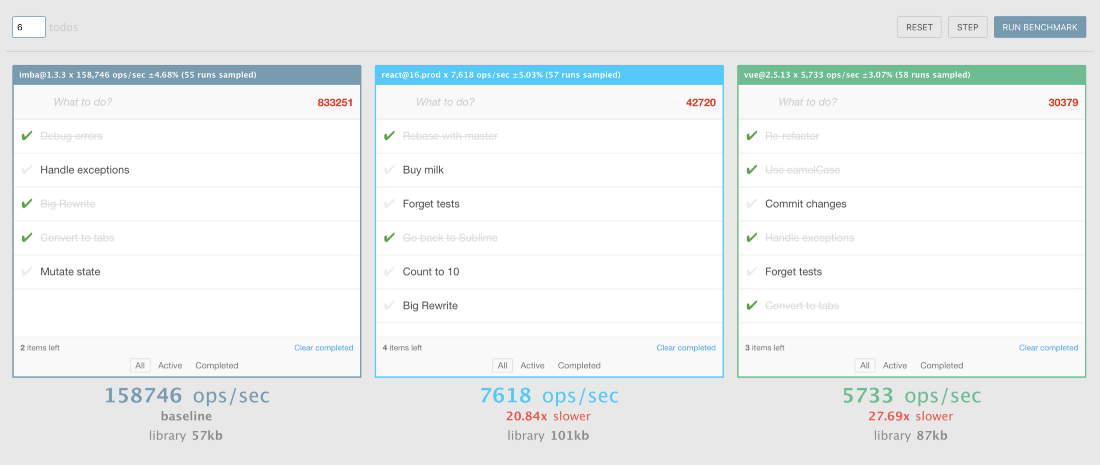
Performance results Imba, the React and Vue
As you can see, the use of Imba means that you can very quickly work with the DOM.
Results
In this article, we looked only at the very basics of Imba. If you are interested in this language, check out its documentation . In particular, it will be useful to master his concept of using getters and setters and the mechanism of implicit calls. Here is a list of helpful resources related to Imba. It should be noted that at first this language may seem difficult, it will take some time to master it, but, as everyone knows, you cannot easily pull a fish out of the pond.
Dear readers! Do you plan to use the Imba language in your projects?
