Working with the Facebook API from UWP Applications
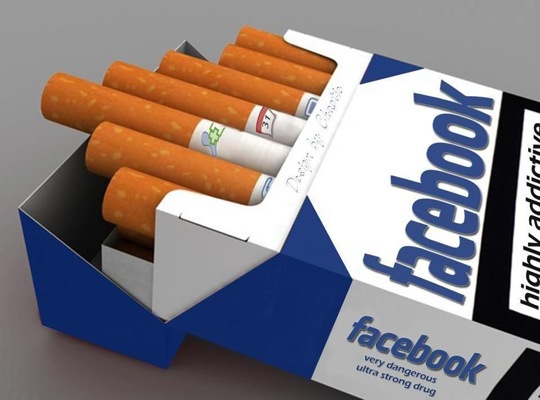
People, as a rule, creatures are very forgetful. In order not to force users to remember a new password when registering in your UWP application, you can use third-party platform accounts. At the same time, it is possible without forcing to fill out long questionnaires to gain access to any useful information and user characteristics. The fact that the publication of content on social networks increases conversion will not even be mentioned, this is understandable. If you want to understand how to work with the most popular network in the world from C # / XAML UWP applications without smoking manuals, then welcome to cat.
Link Facebook application to UWP application
In order for the UWP application to work with the Facevook API, you need to create a Facebook application. This is done through the https://developers.facebook.com/apps/ portal by clicking the "+ Add a new application" button.
Fill in the fields for the application name and contact address, and also classify the application by selecting a category.
Next, we need the input function with facebook
are building switches in such a way that at least the client OAuth authentication , Web authentication OAuth and OAuth authorization, the built-in browser included
and choose the platform by pressing the platform settings
Get the window:
in which we chooseApplication for Windows
Now you can make some settings, but they are not critical. But then you need the identifier of the facebook application.
What we really need to fill out is a little lower:
The value of the Windows Store Security Identifier (SID) can be obtained in different ways.
An easier way is from code with:
WebAuthenticationBroker.GetCurrentApplicationCallbackUri().ToString();
This line will return us ms-app: // SID (ms-app: // will need to be deleted) The
second method requires the application to be associated with the Store. In this case, you can go to the control panel , then in the App management application and select App identity. The value of PackageSID is what you need.
Work with the Facebook API using the Windows SDK for Facebook
We use the library from Microsoft with the unpronounceable abbreviated name winsdkfb . The full name is much easier to pronounce - Windows SDK for Facebook. This is the most convenient way to use the Facebook API from UWP applications. You can download and install it with NuGet.
In C #, add a namespace to the XAML page code
using winsdkfb;
The authentication code is:
string[] requested_permissions = {"public_profile", "email", "user_friends"};
FBSession session = FBSession.ActiveSession;
session.WinAppId = WebAuthenticationBroker.GetCurrentApplicationCallbackUri().ToString();
session.FBAppId = "229583744102151";
FBPermissions permissions = new FBPermissions(requested_permissions);
FBResult result = await session.LoginAsync(permissions);
if (result.Succeeded)
{
// здесь мы можем что-то полезное сделать
}
else
{
// не получилось получить доступ к аккаунту facebook
}
The first line sets the permissions accessed by the application. In the example, 3 permissions are mentioned, for which facebook does not ask for additional user confirmations.
The full list can be found at Permission Help: “Log in with Facebook”.
Two parameters that must be set for connection are the already mentioned SID and the identifier of the created facebook application.
As a result, we get the following built-in window:
Knowing how to enter, you need to know how to exit. This can be done using similar code:
FBSession sess = FBSession.ActiveSession;
await sess.LogoutAsync();
Simple user data can be obtained from the session.User object
string username = session.User.Name; // имя пользователяstring locale = session.User.Locale; // локаль пользователя
What can be useful? For example, you can add to your application the ability to post a message with the result / record of the game or an invitation to join
PropertySet parameters = new PropertySet();
parameters.Add("title", "Угадай MP3");
parameters.Add("link", "https://www.microsoft.com/ru-ru/store/p/Угадай-mp3/9wzdncrdkwgv");
parameters.Add("description", "Прикольная игра а-ля угадай мелодию для компании");
parameters.Add("message", "Попробуй тоже эту прикольную игру");
string path = "/" + session.User.Id + "/feed";
var factory = new FBJsonClassFactory(s =>
{
return JsonConvert.DeserializeObject<FBReturnObject>(s);
});
var singleValue = new FBSingleValue(path, parameters, factory);
var fbresult = await singleValue.PostAsync();
if (fbresult.Succeeded)
{
var response = fbresult.Object as FBReturnObject;
}
else
{
// Попытка запостить оказалась неудачной
}
This snippet is executed if if (result.Succeeded) returns true (that is, after successful authentication). It uses the popular JSON parser called NewtonSoft.JSON, which must be installed from NuGet .
In addition, it is necessary that the following class be present in the project, which characterizes the result of the operation:
publicclassFBReturnObject
{
publicstring Id { get; set; }
publicstring Post_Id { get; set; }
}
There is an option to post a message in the feed, while displaying a dialog box in which the user can add a comment. The code is a bit simpler:
PropertySet parameters = new PropertySet();
parameters.Add("title", "ТесТ");
parameters.Add("link", "https://www.microsoft.com/ru-RU/store/p/take-a-test/9wzdncrdkwgx");
parameters.Add("description", "Нескучная программа для проведения тестирований");
parameters.Add("message", "Я прошел тест с отличным результатом");
FBResult fbresult = await session.ShowFeedDialogAsync(parameters);
if (fbresult.Succeeded)
{
var response = fbresult.Object as FBReturnObject;
}
else
{
// Попытка запостить оказалась неудачной
}
Using the following small snippet, a user can send a link to one of his friends and add a message to it:
PropertySet parameters = new PropertySet();
parameters.Add("link", "http://www.habrahabr.ru");
FBResult fbresult = await session.ShowSendDialogAsync(parameters);
Examples of applications using winsdkfb are located on GitHub , but for C # there is only one example that is not very clear.
More or less normal documentation can be found by the link.
In particular, in addition to the already discussed ways of interacting with fb, the following options are considered: uploading photos / videos, sending invitations to friends (only for games), like any object or link.