
How to speed up build with Maven
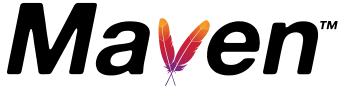
What if the build with Maven is too slow? After all, when the assembly takes too long, anyone, even the most patient developer, can get bored and distracted.
For a quick search on Google or for bookmarks, I immediately offer the final solution:
mvn package -am -o -Dmaven.test.skip -T 1C
- to build a project without tests .
This story began with the fact that I downloaded a rather large Java project from our corporate repository. I think that many, like me, would immediately assemble it with a team:
mvn clean package
Well, many of the team:
mvn clean install
My project was assembled in 25 minutes, and each time it was going to take as long as the first time.
package instead of install
According to the project build life cycle , after the package phase, in which we get a full-fledged jar file, there is a verify phase, and then install. Often it is necessary to get a jar file, which does not need to be placed in a local repository, so in this situation I stopped at package and saved a little time.
Parallel assembly
During normal startup, Maven does not use all the capabilities of modern processors to parallelize computations on different cores, assembling modules one by one. Fortunately, you can use the -T option to tell Maven that you need to build a dependency graph and build modules in parallel.
The parameter can be used in different ways:
mvn -T 4 package
or
mvn -T 1C package
In the first case, you specify the number of threads to use Maven, and in the second - that you need to use one thread for each CPU core.
The second method of setting the parameter, tied to the number of cores, I intend to use in my final team.
Having experimented with the number of threads per core, trying the following options:
mvn -T 1C package
mvn -T 1.5C package
mvn -T 2C package
- I did not get any noticeable increase in the case of more than 1 thread per core on the project being built on the Core i7 processor.
I also note that parallel assembly is an experimental feature of Maven 3. During assembly, problems may arise when using plug-ins other than @threadSafe. In particular, these are plugins:
- Surefire with the parameter forkMode = never, surefire [2.6,) warns (assert) about this.
- maven-modello-plugin, fixed since version 1.4,
- All maven-archiver clients (EAR, EJB, JAR, WAR etc), fixed in latest versions
and libraries:
- plexus-utils 2.0.5
- maven-archiver 2.4.1
- plexus-archiver 1.0
- plexus-io 1.0
Incremental assembly
As we discussed above, the project is most often assembled by the team:
mvn clean package
The clean command is used to clean the project, but is it necessary every time you build it? No, usually we want an incremental project update, and Maven can do this with the command:
mvn package -am
Maven builds the module and updates those modules on which this module depends, if something has changed in them.
This option significantly accelerates the assembly of projects from many modules, in which, as a rule, point changes are made in 1-2 modules.
Offline assembly
It often happens that artifacts on external repositories are not updated very often, especially when these are artifacts of a certain version, or these artifacts should be supported by your efforts (- Welcome to a new project!). It’s quite logical in this situation to tell Maven that you do not need to download them again from the repositories every time:
mvn package -o
or
mvn package --offline
For brevity, we will focus on the first option.
Skipping Tests
Perhaps the most controversial proposal for optimizing the assembly speed I left in the end. For those who fully share the values of TDD, I just suggest scrolling through this paragraph and removing the -Dmaven.test.skip parameter from our final command.
I will not deny that tests are certainly needed, and the programmer must understand the part of the responsibility that he takes upon himself by disabling them. But if you suddenly come across a new gigantic project in which someone once wrote tests, and they do not work, then you already have to turn them off for a start.
As for the Maven launch option, I want to note only that, as a rule, they use the command to skip tests:
mvn package -DskipTests
But you can also skip the test compilation if you execute the command in the following form:
mvn package -Dmaven.test.skip
To summarize
So, once again the command that we got to speed up the build with Maven:
mvn package -am -o -Dmaven.test.skip -T 1C
The result shown on the project I am assembling is pleasing: the assembly instead of 25 minutes began to take place in 30 seconds.
Authors of some other articles on optimizing build speed with Maven also recommend that you use compiler startup optimization options:
MAVEN_OPTS= -XX:+TieredCompilation -XX:TieredStopAtLevel=1
mvn package
- but, firstly, I did not feel any real acceleration of the assembly, apparently, due to the fact that I already used the incremental assembly and the compilation process did not take so much time in this case, and secondly, there are enough references to the fact that these parameters can cause OutOfMemory errors and other problems.
I hope that in the comments you will also share statistics on accelerating the assembly of your projects!