1.1 SFML and Visual Studio
- From the sandbox
- Tutorial
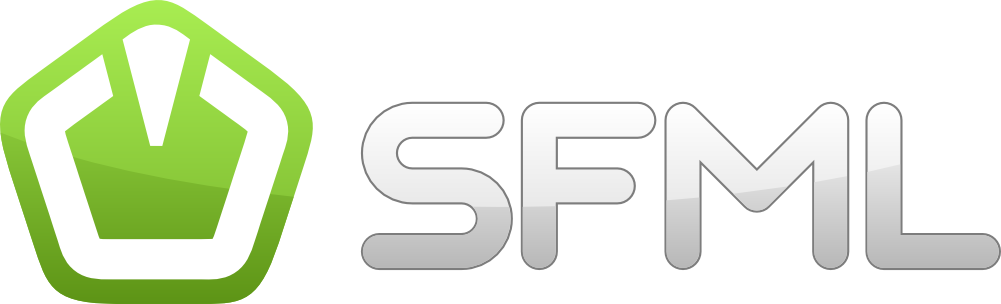
From the translator: this article is the first in the translation cycle of the official SFML library guide. This series of articles aims to provide people who do not know the original language with the opportunity to get acquainted with this library. SFML is a simple and cross-platform multimedia library. SFML provides a simple interface for developing games and other multimedia applications. The original article can be found here . Let's get started.
The SFML library provides a simple interface for the various components of your computer to facilitate the development of games and multimedia applications. It consists of five modules: system, window, graphics, audio and network.
Using SFML, your application can be compiled and run on the most common platforms: Windows, Linux, Mac OS X (planned to support Android and IOS).
Precompiled SDKs for your OS are available on the download page .
SFML officially supports C and .NET. Also, thanks to its active community, it is available in many other languages, such as Java, Ruby, Python, Go, etc.
Table of contents:
0.1 Introduction
1. Getting Started
2. System module
3. Window module
4. Graphics module
5. Audio module
6. Network module
1. Getting Started
- SFML and Visual Studio
- SFML and Code :: Blocks (MinGW)
- SFML and Linux
- SFML and Xcode (Mac OS X)
- Compiling SFML with CMake
2. System module
3. Window module
- Opening and managing windows
- Event handling
- Work with keyboard, mouse and joysticks
- Using OpenGL
4. Graphics module
- 2D drawing objects
- Sprites and textures
- Text and Fonts
- Forms
- Designing your own objects with vertex arrays
- Position, rotation, scale: transforming objects
- Adding special effects with shaders
- 2D camera and view control
5. Audio module
- Playing sounds and music
- Audio recording
- Custom audio streams
- Spatialization: 3D sounds
6. Network module
- Socket Communication
- Use and expansion of packages
- Web Requests Using HTTP
- FTP File Transfer
Introduction
This article is the first one you should read if you are using the Visual Studio development environment (Visual C ++ compiler). It will tell you how to set up your project.
Install SFML
First you need to download the SFML SDK from the download page .
The package you downloaded should match your version of Visual C ++. For example, a library compiled using VC ++ 10 (Visual Studio 2010) will not be compatible with VC ++ 12 (Visual Studio 2013). If you do not find the SFML package compiled for your version of Visual C ++ on the download page, you will have to compile SFML yourself .
Next, you must unzip the SFML archive to any directory convenient for you. Copying header files and libraries to your Visual Studio installation is not recommended. It is better to keep the libraries in a separate place, especially if you intend to use several versions of the same library or several compilers.
Creating and Configuring an SFML Project
The first thing you need to do is select the type of project you are creating: you must select “Win32 application”. The wizard will offer you several options for setting up the project: select “Console application” if you need a console, or “Windows application” otherwise. Select “Empty project” if you do not need the automatically generated code.
Create the main.cpp file and add it to the project. This will apply the C ++ settings (otherwise, Visual Studio will not know which language we will use for this project). The contents of the main.cpp file will be shown below.
Now you need to tell the compiler where to look for header files (files with the .hpp extension) and the linker where to look for SFML libraries (files with the .lib extension).
Add the following to the project properties:
- Path to SFML header files ( <path to install-SFML> / include ) in C / C ++ »General» Additional Include Directories
- Path to SFML libraries ( <path to install-SFML> / lib ) in Linker »General» Additional Library Directories
These paths are the same for Debug and Release configurations, so you can set them globally for your project (“All configurations”).
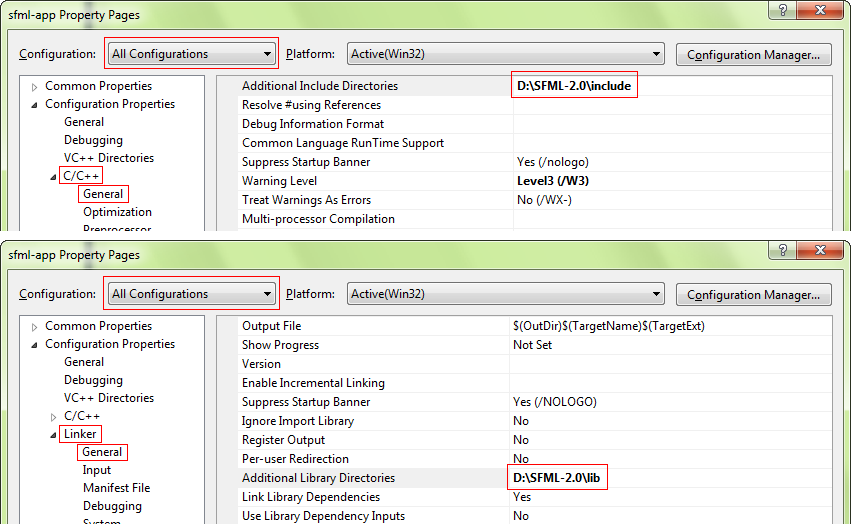
The next step is to build your application with SFML libraries (files with the .lib extension). SFML consists of five modules (system, window, graphics, network and audio) and libraries for each of them. Libraries must be added to project properties in Linker »Input» Additional Dependencies. Add the SFML libraries you need, such as sfml-graphics.lib, sfml-window.lib, and sfml-system.lib.
It is important to specify libraries that match the configuration: “sfml-xxx-d.lib” for Debug and “sfml-xxx.lib” for Release, otherwise errors may occur.
The settings above allow you to build your project with a dynamic version of SFML, which requires DLL files. If you want to directly integrate SFML into your executable file, and not use a dynamic library layout, you must build a static version of the library. SFML static libraries have the suffix "-s": "sfml-xxx-sd.lib" for Debug configuration and "sfml-xxx-s.lib" for Release.
You also need to define the SFML_STATIC macro in the preprocessor options of your project.
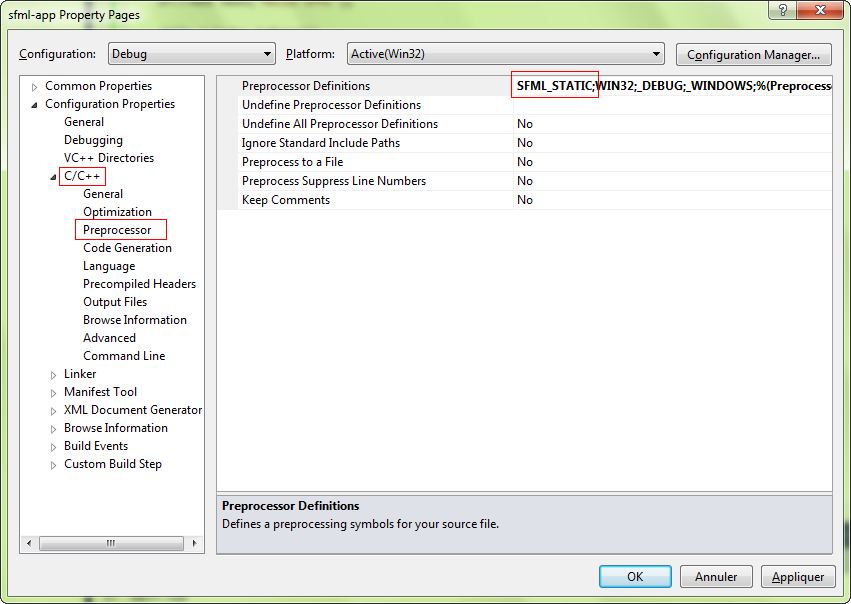
Starting with SFML 2.2 for static linking, you also need to build all the SFML dependencies. This means that if, for example, you build sfml-window-s.lib or sfml-window-sd.lib, you will also have to build opengl32.lib, winmm.lib and gdi32.lib. Some of these libraries may already be listed in the “Inherited values” section, but adding them should not cause any problems.
The table below shows the dependencies for each module, add -d if you want to build the SFML Debug libraries:
Module | Dependencies |
---|---|
sfml-graphics-s.lib |
|
sfml-window-s.lib |
|
sfml-audio-s.lib |
|
sfml-network-s.lib |
|
sfml-system-s.lib |
|
You may have noticed from the table that some SFML modules may depend on others, for example, sfml-graphics-s.lib depends on sfml-window-s.lib and sfml-system-s.lib. If you are building the SFML library statically, be sure that all dependencies in the dependency chain have been satisfied. If one of the dependencies is missing, you will get a linker error.
If you’re a bit confused, don’t worry, it’s completely normal for a beginner to be overwhelmed with all this static linking information. If something doesn’t work out the first time, you can try again with all of the above in mind. If you still have difficulty with static linking, you can try looking for a solution in the FAQ section or on the forum .
If you don’t know how dynamic (also called shared) and static libraries differ and what type of libraries to use, you can find more information on the Internet. There are many good articles / blogs / posts on this subject.
Your project is ready, let's write some code to verify that everything is working correctly. Put the following code in the main.cpp file:
#include
int main()
{
sf::RenderWindow window(sf::VideoMode(200, 200), "SFML works!");
sf::CircleShape shape(100.f);
shape.setFillColor(sf::Color::Green);
while (window.isOpen())
{
sf::Event event;
while (window.pollEvent(event))
{
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(shape);
window.display();
}
return 0;
}
If you selected the “Windows application” option when creating the project, then the entry point into your program should be the “WinMain” function instead of “main”. Since this is a Windows specificity, your code will not compile on Linux or Mac OS X. SFML provides a way to keep the main entry point as the default entry point if you link your project with the sfml-main module (“sfml-main-d. lib ”for Debug,“ sfml-main.lib ”for Release) in the same way that you compiled sfml-graphics, sfml-window and sfml-system.
Now compile the project, and if you compiled your program with a dynamic version of SFML, do not forget to copy the files with the extension .DLL (they are located in <path-to-install-SFML> / bin) to the directory in which the executable file of your program is located. Run the program, and if everything was done correctly, you should see this:
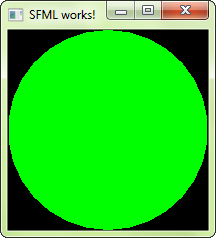
If you use the sfml-audio module (regardless of whether it is static or dynamic), you must also copy the external dll library OpenAL32.dll. This file can also be found in the <path to installation of SFML> / bin directory .
Next article: SFML and Code :: Blocks (MinGW) .