Localization of mobile applications on the example of iOS. Implementation, support and development in future versions
- Tutorial
My colleague, Vyacheslav Budnikov , a leading developer of mobile applications for iOS, decided to share the experience of restoring order in the localization of projects.
Localization of a mobile application involves translating the interface and adapting the software for use in another language. Tinkoff Bank’s mobile application is used only by Russian-speaking customers, so we didn’t have such a task. But the principle of localization was applied to restore order in the texts, so that it was easier to read, check for errors and edit.
Consider the localization of text elements on the example of iOS. To do this, use the Localizable.strings list which describes all phrases in the format "key" = "localized value". Each localization language has a different key value.
Important:
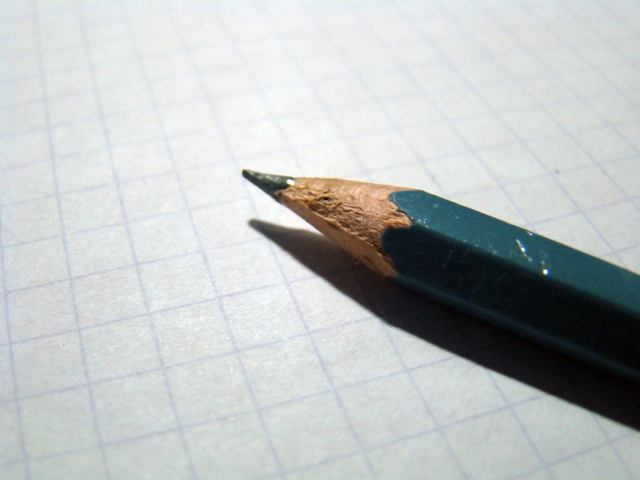
Localization was implemented during the development of MB 2.0 - the second version of the Tinkoff mobile bank. Initially, they took out localized strings in Localizable.strings, filled in the missing strings before testing, changed and added new strings during testing. Then we made a new assembly and checked everything. And so for each platform.
Naturally, errors arose. Therefore, we turned on localization in TK and began to take all the values from the documentation, which allowed us to synchronize localization between platforms and get rid of different localized names.
The method did not solve all the problems. There were situations when it was required to change the phrase on only one screen or only for one scenario. It was necessary to carefully look at the search results wherever the old key was, change it to a new one, observing the new logic. Errors often occurred here, which we saw only at the testing stage.
The next step was to use not localized strings in the code, but pointers to localized string constants. This eliminated the magic lines and typos.
How to do it
An example of a functional description in TK:
The TK text is written in a wiki and stored in html, in the source code it looks like this:
To search for such phrases, we use a script that finds these phrases and creates a list of "key" = "value" of the form: "main_continue" = "Next". This is the main list of localized phrases that we will use in the application.
Further, on this list, create 2 more lists:
And save them in 3 files:
We replace these files in our project and collect it. Now you can use the new localization keys as follows:
NSLocalizedString (LocKey_common_error, @ "");
If the project is not collected during the next localization update, then the necessary constants have been deleted or renamed. Therefore, we either change them in the code, or return to the TK.
Thus, with each change of the ToR, new files Localizable.strings LocalizableKeys.h LocalizableKeys.m are generated and the project is assembled. As a result, we obtain full compliance with technical requirements and their implementation.
Advantages of our approach:
This approach will help clean up the TK, even if you don’t have a task to translate the mobile application into other languages.
When writing autotests to identify objects on the screen, you need unique keys for all interface elements - a text input field, a button, and so on.
Just as in localization, we will assign accessibilityLabel objects constants from the TK and add a style to style phrases for autotests.
An example of a functional description in TK:
We write the TK text in a wiki and store it in html, in the source code it will look like this:
As a result, we get:
In code, it looks like this:
Now for writing autotests you need an application and TK. For multi-platform applications, you can use the same autotest scripts for all platforms.
Introduction
Localization of a mobile application involves translating the interface and adapting the software for use in another language. Tinkoff Bank’s mobile application is used only by Russian-speaking customers, so we didn’t have such a task. But the principle of localization was applied to restore order in the texts, so that it was easier to read, check for errors and edit.
Consider the localization of text elements on the example of iOS. To do this, use the Localizable.strings list which describes all phrases in the format "key" = "localized value". Each localization language has a different key value.
Important:
- monitor the writing of string keys in the code, otherwise instead of a localized phrase we get a “technical” phrase (name of a constant);
- make sure that each key has localized values;
- ensure that the localization on all application platforms is identical. If a new version of the application comes out, you need to check existing phrases.
Where to begin
Localization was implemented during the development of MB 2.0 - the second version of the Tinkoff mobile bank. Initially, they took out localized strings in Localizable.strings, filled in the missing strings before testing, changed and added new strings during testing. Then we made a new assembly and checked everything. And so for each platform.
Naturally, errors arose. Therefore, we turned on localization in TK and began to take all the values from the documentation, which allowed us to synchronize localization between platforms and get rid of different localized names.
The method did not solve all the problems. There were situations when it was required to change the phrase on only one screen or only for one scenario. It was necessary to carefully look at the search results wherever the old key was, change it to a new one, observing the new logic. Errors often occurred here, which we saw only at the testing stage.
The next step was to use not localized strings in the code, but pointers to localized string constants. This eliminated the magic lines and typos.
How to do it
- Add Localizable.strings file;
- Add 2 files for string constants:
LocalizableKeys.h
LocalizableKeys.m; - For TK, select a style for localized phrases.
An example of a functional description in TK:
If you clicked "Search" (main_continue) on screen 1.1 then ... "
The TK text is written in a wiki and stored in html, in the source code it looks like this:
Если нажата кнопка "Искать" (main_continue) на экране 1.1 то
Implementation
To search for such phrases, we use a script that finds these phrases and creates a list of "key" = "value" of the form: "main_continue" = "Next". This is the main list of localized phrases that we will use in the application.
Further, on this list, create 2 more lists:
- For the header file: extern NSString * const LocKey_main_continue;
- Implementation: NSString * const LocKey_main_continue = @ "main_continue";
And save them in 3 files:
- Localizable.strings - the file in which the key-value localization for each localization language lies
"main_continue" = "Далее"; "common_error" = "Ошибка";
- LocalizableKeys.h
import
extern NSString *const LocKey_main_continue; extern NSString *const LocKey_common_error; - 3.LocalizableKeys.m
#import "LocalizableKeys.h" NSString *const LocKey_main_continue = @"main_continue"; NSString *const LocKey_common_error = @"common_error";
We replace these files in our project and collect it. Now you can use the new localization keys as follows:
NSLocalizedString (LocKey_common_error, @ "");
If the project is not collected during the next localization update, then the necessary constants have been deleted or renamed. Therefore, we either change them in the code, or return to the TK.
Thus, with each change of the ToR, new files Localizable.strings LocalizableKeys.h LocalizableKeys.m are generated and the project is assembled. As a result, we obtain full compliance with technical requirements and their implementation.
Advantages of our approach:
- the integrity of all keys is checked by the compiler. If such a constant was removed from localization, then the compiler will tell us about it during assembly;
- the name and meaning of the keys governs TK;
- when writing code, you can use the context help, which significantly increases the speed of writing code;
- Another person who monitors localization is connected to reading TK.
This approach will help clean up the TK, even if you don’t have a task to translate the mobile application into other languages.
An example of using the localization approach for autotests
When writing autotests to identify objects on the screen, you need unique keys for all interface elements - a text input field, a button, and so on.
Just as in localization, we will assign accessibilityLabel objects constants from the TK and add a style to style phrases for autotests.
An example of a functional description in TK:
“If the Continue button (main_continue btn_continue) is pressed on screen 1.1 then ...”
We write the TK text in a wiki and store it in html, in the source code it will look like this:
«Если нажата кнопка "Продолжить" (main_continuebtn_continue) на экране 1.1 то»
As a result, we get:
- AccessibilityKeys.h
#import
extern NSString *const AccKey_btn_continue - AccessibilityKeys.m
#import "AccessibilityKeys.h" NSString *const AccKey_btn_continue = @"bnt_continue"
In code, it looks like this:
UIButton *buttonRegistration;
[buttonRegistration setAccessibilityLabel: AccKey_btn_continue];
[buttonRegistration setTitle: NSLocalizedString(LocKey_main_continue, @"") forState:UIControlStateNormal]
Now for writing autotests you need an application and TK. For multi-platform applications, you can use the same autotest scripts for all platforms.