Ignorance of the basics of React, which is probably ruining you
- Transfer
Want to get a clear idea of what happens to components when you work with React? Read the translation of the Ohans Emmanuel article published on the freeCodeCamp website under the cut .

It is often impossible to eliminate a certain error because you do not know some basics. For the same reason, it is difficult to master more advanced techniques.
In this article I will try to talk about some of the principles of React, which, in my opinion, you need to understand.
We will not understand these principles from a technical point of view. There are many other articles that deal with such concepts as component properties (
I want to talk about what underlies most of the technical operations that you will perform with React.
Ready?
The first thing everyone learns in React is how to create components. I am sure that you also learned this.
For example:
Most of the components you prescribe return some elements to you.
From the inside, this process looks like this: most components return a tree of elements.
After internal evaluation, components often return an element tree.
In addition, you must remember that components work as functions that return values based on their values
Components are something like functions with props and state parameters.
Therefore, whenever the values of the properties (
If the props or state values change, the element tree is redrawn. As a result, a new element tree appears.
If the component is based on class inheritance, the element tree returns a function
If the component is functional, its return value is given by the element tree.
Why is it important?
Consider a component
Rendering this component returns an element tree.

An element tree returned after redrawing <MyComponent />
What happens when the value
Well, the new element tree is back!
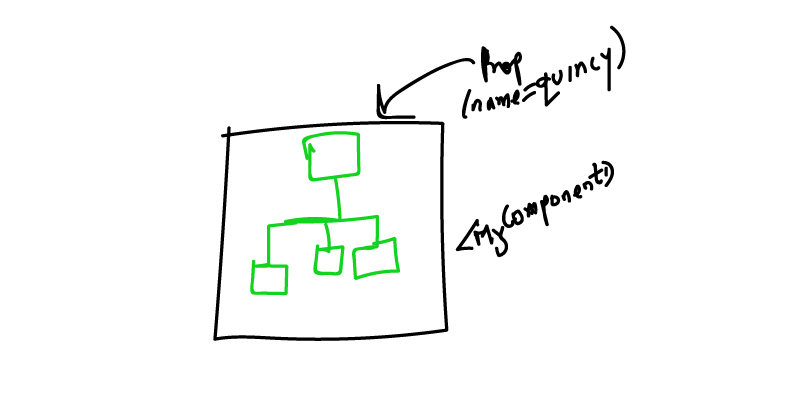
NEW tree of elements returned after redrawing <MyComponent /> with other props
Good.
Now React has two different trees at its disposal - the previous and the current tree of elements.
At this point, React compares both trees to find changes.

Two different trees. What exactly has changed?
The tree has not changed completely, but only partially updated (this happens in most cases).
After comparing, React updates the actual DOM with changes in the new element tree.
It's simple, isn't it?
The comparison of two trees for changes is called “reconciliation.” We were able to disassemble this process, despite the fact that it is quite complicated .
Even before you started working with React, you often had to hear how cool it is, including the fact that it makes only important changes to the updated DOM model.

From React Docs : DOM Inspector Showing Update Details
Is Everything Alright?
It's like that.
However, remember: before proceeding to the DOM update, React builds a tree of elements for the various components and makes the necessary comparison. Simply put, it will find the differences between the previous element tree and the current one.
I repeat this because newcomers to React may not notice the performance degradation of their applications, considering that React updates only the necessary elements in the DOM.
This, of course, is true, but the performance problems of most React applications start even before the DOM update!
Even if the element's element tree is small, its rendering takes some time (at least insignificant). The larger the component element tree, the longer the rendering takes.
This means that redrawing the element trees of your application with React would be superfluous if it is NOT necessary.
Let me show this with a simple example.
Imagine an application with the structure of the components, as in the illustration below.

An application with parent component A and child components B, C, and D
A generic container component

The parent component A receives some properties and passes them on to the child component D.
Now that the value of the property in the component changes


When the parent component gets new features, every child element is redrawn, and returns a new tree
Accordingly, components
This extra redrawing is unnecessary rendering.
In this example, the components
There are many ways to solve this problem, and I described them in my recent article How to Eliminate React Performance Issues (“How to minimize React performance problems”).
Go ahead. Look at the app below.

Cardie in action :)
I called this app Carde .
When I press the button to change the user's profession, I can select the highlighting of updates for the DOM, as shown below.

Activate the visual display of updates (Paint Flashing) using Chrome DevTools
Now I can see what has been updated in the DOM.
This is a visual way of marking the elements that need to be updated in the DOM. Notice the green highlight around the text I am a Librarian (“I'm a Librarian.”)
This is all great, of course, but I'm worried about the original rendering of the element tree of the React components.
I can check it too.
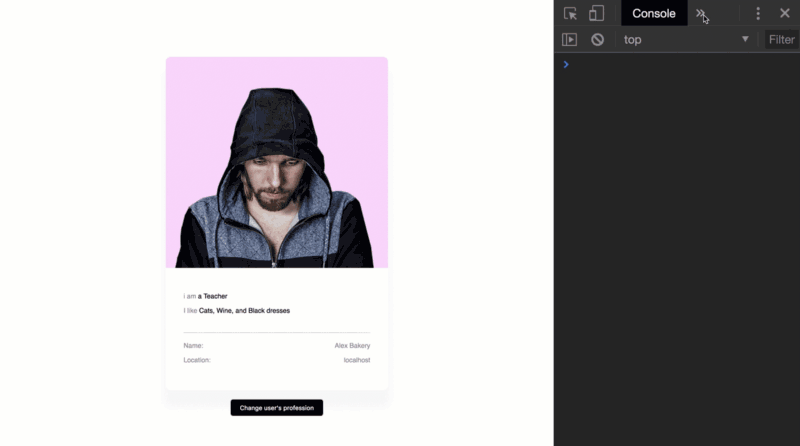
Check the React DevTools checkbox to turn on the highlighting of the updated elements.
Now I can see which components actually redraw when I click this button.

Notice the green highlight around the user's map.
You see how different the visual way is to mark the elements that need to be updated in the DOM, and the rendering updates that React itself conducts?
React redraws the entire user card, and only the short text is updated.
And this is important.
I think that now you have a more visual idea of what is happening with your components in React.
In fact, much more is happening than I have told you here. However, this is a good start.
Forward to creating cool apps!
Learn to work with React / Redux? If yes, I have a great redux book series . Some say it is the best technical literature that they read !

It is often impossible to eliminate a certain error because you do not know some basics. For the same reason, it is difficult to master more advanced techniques.
In this article I will try to talk about some of the principles of React, which, in my opinion, you need to understand.
We will not understand these principles from a technical point of view. There are many other articles that deal with such concepts as component properties (
props
), state ( state
), context ( context
), component state changes ( setState
), and others. I want to talk about what underlies most of the technical operations that you will perform with React.
Ready?
React Hidden Processes
The first thing everyone learns in React is how to create components. I am sure that you also learned this.
For example:
// functional component functionMyComponent() {
return<div> My Functional Component </div>
}
// class based component classMyComponentextendsReact.Component{
render() {
return<div> My Class Component </div>
}
}
Most of the components you prescribe return some elements to you.
functionMyComponent() {
return<span> My Functional Component </span>//span element
}
classMyComponentextendsReact.Component{
render() {
return<div> My Class Component </div>//div element
}
}
From the inside, this process looks like this: most components return a tree of elements.

In addition, you must remember that components work as functions that return values based on their values
props
and state
. 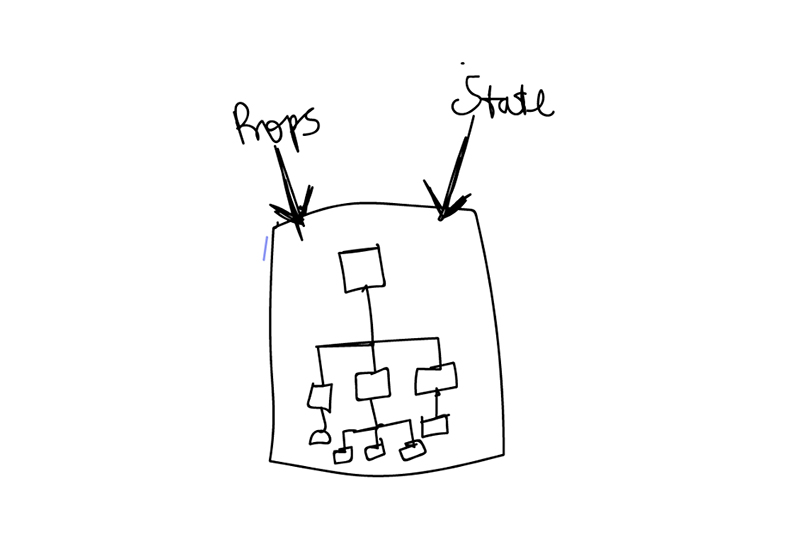
Therefore, whenever the values of the properties (
props
) and states ( state
) of the component change, a new tree of elements is created. 
If the component is based on class inheritance, the element tree returns a function
<code>render</code>.
<source lang="javascript">class MyComponent extends React.Component {
render() {
//this function is invoked to return the tree of elements
}
}
If the component is functional, its return value is given by the element tree.
functionMyComponent() {
// the return value yields the tree of elementsreturn<div></div>
}
Why is it important?
Consider a component
<MyComponent />
that accepts prop
, as shown below.<MyComponent name='Ohans'/>
Rendering this component returns an element tree.

An element tree returned after redrawing <MyComponent />
What happens when the value
name
changes?<MyComponent name='Quincy'/>
Well, the new element tree is back!
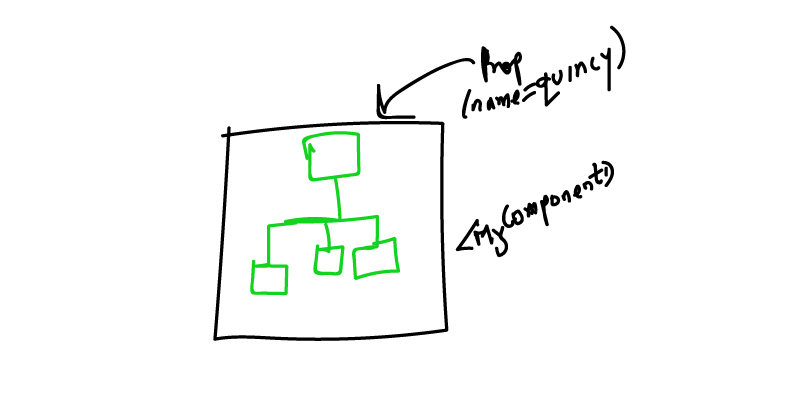
NEW tree of elements returned after redrawing <MyComponent /> with other props
Good.
Now React has two different trees at its disposal - the previous and the current tree of elements.
At this point, React compares both trees to find changes.

Two different trees. What exactly has changed?
The tree has not changed completely, but only partially updated (this happens in most cases).
After comparing, React updates the actual DOM with changes in the new element tree.
It's simple, isn't it?
The comparison of two trees for changes is called “reconciliation.” We were able to disassemble this process, despite the fact that it is quite complicated .
React updates only necessary, right?
Even before you started working with React, you often had to hear how cool it is, including the fact that it makes only important changes to the updated DOM model.

From React Docs : DOM Inspector Showing Update Details
Is Everything Alright?
It's like that.
However, remember: before proceeding to the DOM update, React builds a tree of elements for the various components and makes the necessary comparison. Simply put, it will find the differences between the previous element tree and the current one.
I repeat this because newcomers to React may not notice the performance degradation of their applications, considering that React updates only the necessary elements in the DOM.
This, of course, is true, but the performance problems of most React applications start even before the DOM update!
Unnecessary rendering vs visual updates
Even if the element's element tree is small, its rendering takes some time (at least insignificant). The larger the component element tree, the longer the rendering takes.
This means that redrawing the element trees of your application with React would be superfluous if it is NOT necessary.
Let me show this with a simple example.
Imagine an application with the structure of the components, as in the illustration below.

An application with parent component A and child components B, C, and D
A generic container component
A
gets a specific property. However, this is done only to pass this property to the component D
.
The parent component A receives some properties and passes them on to the child component D.
Now that the value of the property in the component changes
A
, all the child elements are A
redrawn to calculate the new element tree. 

When the parent component gets new features, every child element is redrawn, and returns a new tree
Accordingly, components
B
, and С
also re-rendered, even if they have not changed at all! They got no new properties! This extra redrawing is unnecessary rendering.
In this example, the components
B
and C
redraw is not necessary, but React does not know about it.There are many ways to solve this problem, and I described them in my recent article How to Eliminate React Performance Issues (“How to minimize React performance problems”).
Go ahead. Look at the app below.

Cardie in action :)
I called this app Carde .
When I press the button to change the user's profession, I can select the highlighting of updates for the DOM, as shown below.

Activate the visual display of updates (Paint Flashing) using Chrome DevTools
Now I can see what has been updated in the DOM.
This is a visual way of marking the elements that need to be updated in the DOM. Notice the green highlight around the text I am a Librarian (“I'm a Librarian.”)
This is all great, of course, but I'm worried about the original rendering of the element tree of the React components.
I can check it too.
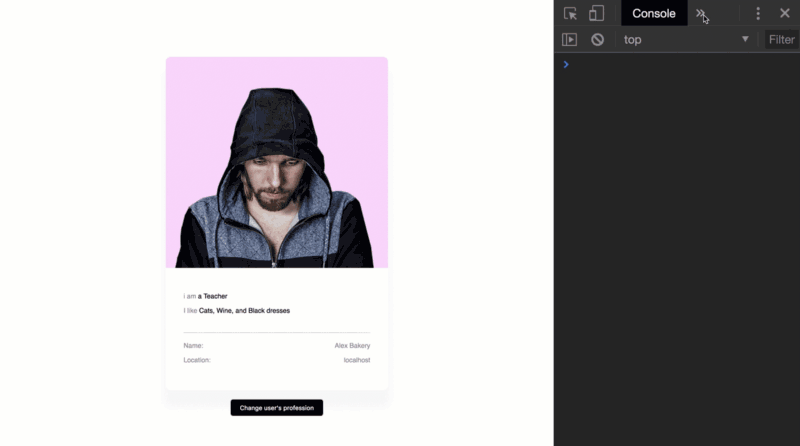
Check the React DevTools checkbox to turn on the highlighting of the updated elements.
Now I can see which components actually redraw when I click this button.

Notice the green highlight around the user's map.
You see how different the visual way is to mark the elements that need to be updated in the DOM, and the rendering updates that React itself conducts?
React redraws the entire user card, and only the short text is updated.
And this is important.
Conclusion
I think that now you have a more visual idea of what is happening with your components in React.
In fact, much more is happening than I have told you here. However, this is a good start.
Forward to creating cool apps!
Learn to work with React / Redux? If yes, I have a great redux book series . Some say it is the best technical literature that they read !