
How to make a table cell movable to display additional options in iOS applications
- Transfer
- Tutorial
As a translator, I apologize in advance for possible errors in the translation. I would be grateful for error messages to correct them as soon as possible.
When iOS 7 was first announced, one of the many visual innovations that particularly interested me was the swipe-to-delete gesture (swipe to delete) in the Mail app. You have long been familiar with this feature of the application. After swiping the table cell, you see the basket buttons, additional options (“More”) and flagging the letter. When you click on the "More" button, the action selection panel becomes active, on which you can select one of the action options with a letter - Reply, Flag with a flag, etc.
I think this is a great tool for manipulating table entries. However, as you well know that Apple does not provide this feature for developers in iOS 7. You can only add a gesture for the function to delete a record from the table. Other options for working with the table record are limited to the standard Mail application. I have no idea why Apple limits the use of such a great tool only to its applications. Fortunately, some developers created solutions (such as UITableView-Swipe-for-Options , MCSwipeTableViewCell ) and made them completely free.
In this tutorial I will use the SWTableViewCell library. Let's see how it helps us implement the swipe-to-show-options function (swipe to see options) in your application. SWTableViewCell is fairly easy to use and if you understand how UITableView works, you should not have problems with SWTableViewCell. In addition, this component supports the display of option buttons when swiping in both directions. You will quickly understand what this means.
So, let's get started and create our demo application.
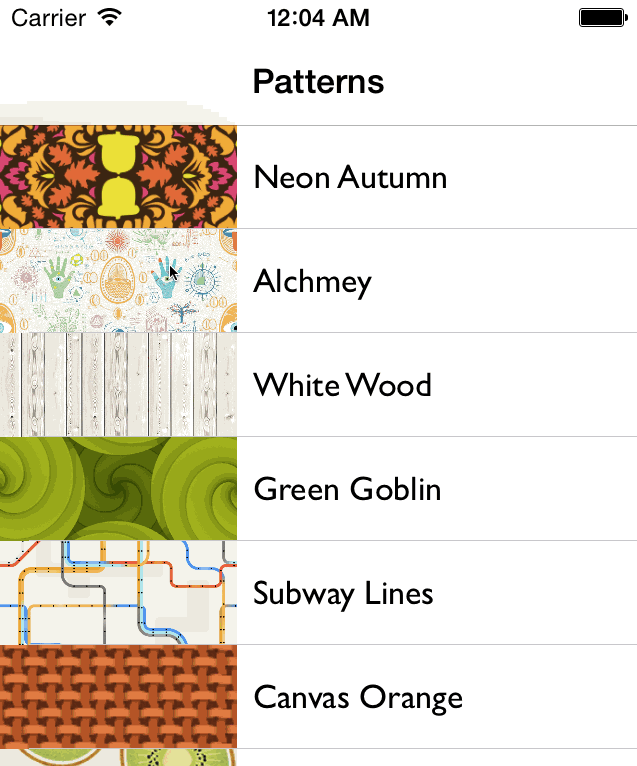
I highly recommend you create a demo application project from scratch. However, in order to focus exclusively on using the SWTableViewCell library, I have prepared a project template for you. You can download it here . If you know how to create a custom table view, you need to understand how this template works. After you download it, you can try to compile and execute the project. The application should display a list of pattern images (kindly provided by thepatternlibrary.com ).
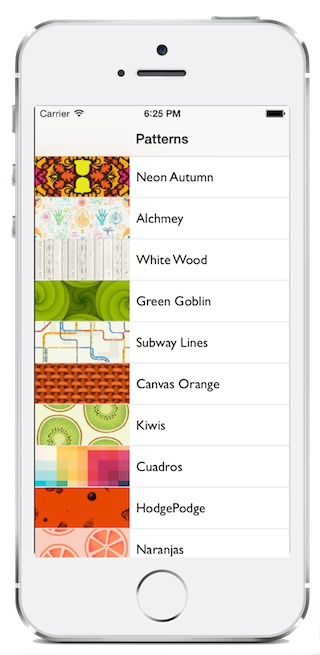
SWTableViewCell is developed by Chris Wendel and is available absolutely free on GitHub. To use it, first download it from GitHub, unzip the archive and add the unpacked files to the SWTableViewCell or PodFiles group in your project.
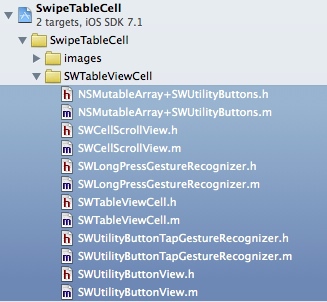
The SWTableViewCell library is very easy to use. If you are familiar with UITableView and UITableViewCell, you should not have difficulty using it. Here is what you should do:
1. Choose as a class that will serve the cells of our table SWTableViewCell instead of UITableViewCell. SWTableViewCell is actually inherited from UITableViewCell, providing additional tools for displaying option buttons when swiping over a table cell.
2. Modify the cellForRowAtIndexPath method : to create new buttons - SWTableViewCell provides two properties with the names leftUtilityButtons and rightUtilityButtons. These two properties provide a set of option buttons that are shown when swiping left and right, respectively. You must first create arrays of option buttons and assign them all the necessary properties. It is not necessary to assign button sets to both leftUtilityButtons and rightUtilityButtons properties at once .
3. Implement the SWTableViewCellDelegate protocol. The methods of this protocol allow developers to control the actions of additional buttons. When the user swipe through the table cell, this cell will show a set of option buttons. Depending on the direction of the swipe, when you click on the option button, one of the didTriggerRightUtilityButtonWithIndex: or didTriggerLeftUtilityButtonWithIndex methods will be called :. You must implement these methods to respond to button presses.
Well, let's change the application template to use SWTableViewCell.
To get started, open the CustomTableViewCell.h file and change the parent class to SWTableViewCell. Your code should look something like this after making the changes:
Now we will change the cellForRowAtIndexPath: method in the SwipeTableViewController.m file to create the cell option buttons. But before we do this, add the import statement to the library and specify implement the SWTableViewCellDelegate protocol in the SwipeTableViewController.h file. We will talk about protocol implementation a bit later, in the next section, first, let's make changes to the code. After making the changes, it should look something like this:
Open the SwipeTableViewController.m file and make changes to the cellForRowAtIndexPath method :
In this example, we created two sets of option buttons. One of them will be shown when swiping to the left, the other when swiping to the right. We created a set of buttons for sharinga and placed it in the variable leftUtilityButtons, and we also created buttons “More” (Advanced) and “Delete” and placed them in the variable rightUtilityButtons. As you can see from the code above, you can simply create an array of NSMutableArray and add the necessary buttons to it using the sw_addUtilityButtonWithColor: method .
Now you are ready to compile and run the test application. After starting, try navigating through any cell in the table and you should see the cell options buttons. However, the buttons do not work and we know why. We did not implement some methods of the SWTableViewCellDelegate protocol. Well, let's move on.
The SWTableViewCellDelegate protocol provides methods for controlling actions on clicking option buttons. An attentive reader, of course, noticed that the SwipeTableViewController is assigned as the delegate of SWTableViewCell in the code above. Well, we implement the following two methods in SwipeTableViewController.m:
1. didTriggerLeftUtilityButtonWithIndex method : - works when any of the option buttons on the left side of the cell is pressed
2. didTriggerRightUtilityButtonWithIndex method : - works when any of the option buttons is pressed on the right of the cell
Add the following code in SwipeTableViewController.m:
To keep our example simple, we simply display an alert when any of the sharing buttons is pressed. In a real application, you can implement real sharing on Facebook and Twitter, for example.
For the option buttons located on the right side of the cell, we implemented the didTriggerRightUtilityButtonWithIndex: method with the following code:
And again, the code is simple and straightforward. When the More button is clicked, the application displays an action sheet containing a list of social networks for sharing. For the “delete” option, we just need to remove the item from the list of patterns and the patternImages array, and then call the deleteRowsAtIndexPaths: method to remove the record from the displayed table.
That's all! Now you can compile and run your application. The option buttons should work something like this:

SWTableViewCell makes it very easy to implement the swipe-for-options function for tables. When developing your next application, consider implementing this feature. I think this will raise the level of your application for the user.
And although we can implement this functionality using many third-party libraries or implement it on our own , I hope that Apple will consider providing this tool to developers in future versions of iOS.
You can download the finished project if you suddenly need it.
When iOS 7 was first announced, one of the many visual innovations that particularly interested me was the swipe-to-delete gesture (swipe to delete) in the Mail app. You have long been familiar with this feature of the application. After swiping the table cell, you see the basket buttons, additional options (“More”) and flagging the letter. When you click on the "More" button, the action selection panel becomes active, on which you can select one of the action options with a letter - Reply, Flag with a flag, etc.
I think this is a great tool for manipulating table entries. However, as you well know that Apple does not provide this feature for developers in iOS 7. You can only add a gesture for the function to delete a record from the table. Other options for working with the table record are limited to the standard Mail application. I have no idea why Apple limits the use of such a great tool only to its applications. Fortunately, some developers created solutions (such as UITableView-Swipe-for-Options , MCSwipeTableViewCell ) and made them completely free.
In this tutorial I will use the SWTableViewCell library. Let's see how it helps us implement the swipe-to-show-options function (swipe to see options) in your application. SWTableViewCell is fairly easy to use and if you understand how UITableView works, you should not have problems with SWTableViewCell. In addition, this component supports the display of option buttons when swiping in both directions. You will quickly understand what this means.
So, let's get started and create our demo application.
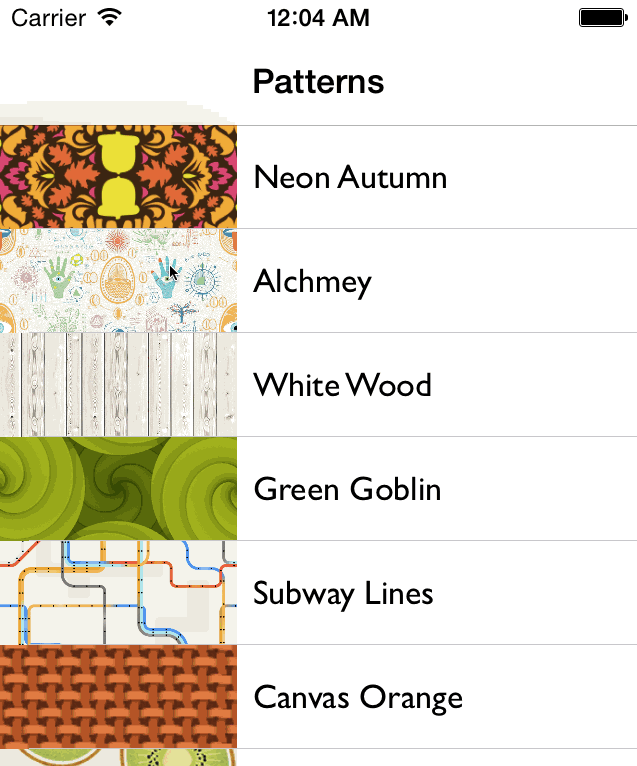
Xcode Project Template for Demo Application
I highly recommend you create a demo application project from scratch. However, in order to focus exclusively on using the SWTableViewCell library, I have prepared a project template for you. You can download it here . If you know how to create a custom table view, you need to understand how this template works. After you download it, you can try to compile and execute the project. The application should display a list of pattern images (kindly provided by thepatternlibrary.com ).
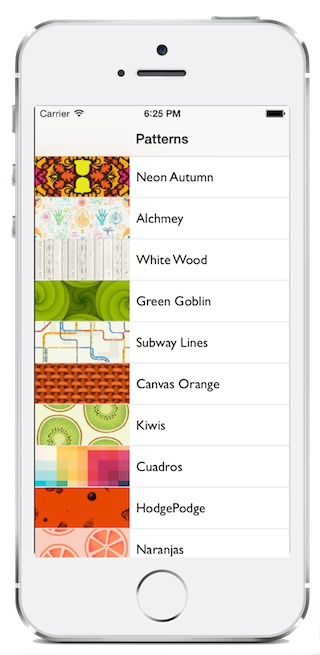
Adding the SWTableViewCell library to our project
SWTableViewCell is developed by Chris Wendel and is available absolutely free on GitHub. To use it, first download it from GitHub, unzip the archive and add the unpacked files to the SWTableViewCell or PodFiles group in your project.
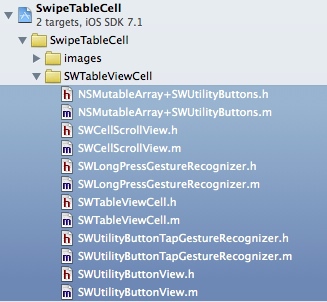
Using SWTableViewCell
The SWTableViewCell library is very easy to use. If you are familiar with UITableView and UITableViewCell, you should not have difficulty using it. Here is what you should do:
1. Choose as a class that will serve the cells of our table SWTableViewCell instead of UITableViewCell. SWTableViewCell is actually inherited from UITableViewCell, providing additional tools for displaying option buttons when swiping over a table cell.
2. Modify the cellForRowAtIndexPath method : to create new buttons - SWTableViewCell provides two properties with the names leftUtilityButtons and rightUtilityButtons. These two properties provide a set of option buttons that are shown when swiping left and right, respectively. You must first create arrays of option buttons and assign them all the necessary properties. It is not necessary to assign button sets to both leftUtilityButtons and rightUtilityButtons properties at once .
3. Implement the SWTableViewCellDelegate protocol. The methods of this protocol allow developers to control the actions of additional buttons. When the user swipe through the table cell, this cell will show a set of option buttons. Depending on the direction of the swipe, when you click on the option button, one of the didTriggerRightUtilityButtonWithIndex: or didTriggerLeftUtilityButtonWithIndex methods will be called :. You must implement these methods to respond to button presses.
Well, let's change the application template to use SWTableViewCell.
Changing the Serving Table View Cell class to SWTableViewCell
To get started, open the CustomTableViewCell.h file and change the parent class to SWTableViewCell. Your code should look something like this after making the changes:
#import
#import "SWTableViewCell.h"
@interface CustomTableViewCell : SWTableViewCell
@property (weak, nonatomic) IBOutlet UIImageView *patternImageView;
@property (weak, nonatomic) IBOutlet UILabel *patternLabel;
@end
Creating Option Buttons
Now we will change the cellForRowAtIndexPath: method in the SwipeTableViewController.m file to create the cell option buttons. But before we do this, add the import statement to the library and specify implement the SWTableViewCellDelegate protocol in the SwipeTableViewController.h file. We will talk about protocol implementation a bit later, in the next section, first, let's make changes to the code. After making the changes, it should look something like this:
#import
#import "SWTableViewCell.h"
@interface SwipeTableViewController : UITableViewController
@end
Open the SwipeTableViewController.m file and make changes to the cellForRowAtIndexPath method :
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *cellIdentifier = @"Cell";
CustomTableViewCell *cell = (CustomTableViewCell *)[tableView dequeueReusableCellWithIdentifier:cellIdentifier forIndexPath:indexPath];
// Add utility buttons
NSMutableArray *leftUtilityButtons = [NSMutableArray new];
NSMutableArray *rightUtilityButtons = [NSMutableArray new];
[leftUtilityButtons sw_addUtilityButtonWithColor:
[UIColor colorWithRed:1.0f green:1.0f blue:0.35f alpha:0.7]
icon:[UIImage imageNamed:@"like.png";
[leftUtilityButtons sw_addUtilityButtonWithColor:
[UIColor colorWithRed:1.0f green:1.0f blue:0.35f alpha:0.7]
icon:[UIImage imageNamed:@"message.png";
[leftUtilityButtons sw_addUtilityButtonWithColor:
[UIColor colorWithRed:1.0f green:1.0f blue:0.35f alpha:0.7]
icon:[UIImage imageNamed:@"facebook.png";
[leftUtilityButtons sw_addUtilityButtonWithColor:
[UIColor colorWithRed:1.0f green:1.0f blue:0.35f alpha:0.7]
icon:[UIImage imageNamed:@"twitter.png";
[rightUtilityButtons sw_addUtilityButtonWithColor:
[UIColor colorWithRed:0.78f green:0.78f blue:0.8f alpha:1.0]
title:@"More"];
[rightUtilityButtons sw_addUtilityButtonWithColor:
[UIColor colorWithRed:1.0f green:0.231f blue:0.188 alpha:1.0f]
title:@"Delete"];
cell.leftUtilityButtons = leftUtilityButtons;
cell.rightUtilityButtons = rightUtilityButtons;
cell.delegate = self;
// Configure the cell...
cell.patternLabel.text = [patterns objectAtIndex:indexPath.row];
cell.patternImageView.image = [UIImage imageNamed:[patternImages objectAtIndex:indexPath.row;
return cell;
}
In this example, we created two sets of option buttons. One of them will be shown when swiping to the left, the other when swiping to the right. We created a set of buttons for sharinga and placed it in the variable leftUtilityButtons, and we also created buttons “More” (Advanced) and “Delete” and placed them in the variable rightUtilityButtons. As you can see from the code above, you can simply create an array of NSMutableArray and add the necessary buttons to it using the sw_addUtilityButtonWithColor: method .
Now you are ready to compile and run the test application. After starting, try navigating through any cell in the table and you should see the cell options buttons. However, the buttons do not work and we know why. We did not implement some methods of the SWTableViewCellDelegate protocol. Well, let's move on.
Implementing the SWTableViewCellDelegate Protocol
The SWTableViewCellDelegate protocol provides methods for controlling actions on clicking option buttons. An attentive reader, of course, noticed that the SwipeTableViewController is assigned as the delegate of SWTableViewCell in the code above. Well, we implement the following two methods in SwipeTableViewController.m:
1. didTriggerLeftUtilityButtonWithIndex method : - works when any of the option buttons on the left side of the cell is pressed
2. didTriggerRightUtilityButtonWithIndex method : - works when any of the option buttons is pressed on the right of the cell
Add the following code in SwipeTableViewController.m:
- (void)swipeableTableViewCell:(SWTableViewCell *)cell didTriggerLeftUtilityButtonWithIndex:(NSInteger)index {
switch (index) {
case 0:
{
UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Bookmark" message:@"Save to favorites successfully" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alertView show];
break;
}
case 1:
{
UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Email sent" message:@"Just sent the image to your INBOX" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alertView show];
break;
}
case 2:
{
UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Facebook Sharing" message:@"Just shared the pattern image on Facebook" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alertView show];
break;
}
case 3:
{
UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Twitter Sharing" message:@"Just shared the pattern image on Twitter" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles: nil];
[alertView show];
}
default:
break;
}
}
To keep our example simple, we simply display an alert when any of the sharing buttons is pressed. In a real application, you can implement real sharing on Facebook and Twitter, for example.
For the option buttons located on the right side of the cell, we implemented the didTriggerRightUtilityButtonWithIndex: method with the following code:
- (void)swipeableTableViewCell:(SWTableViewCell *)cell didTriggerRightUtilityButtonWithIndex:(NSInteger)index {
switch (index) {
case 0:
{
// More button is pressed
UIActionSheet *shareActionSheet = [[UIActionSheet alloc] initWithTitle:@"Share" delegate:nil cancelButtonTitle:@"Cancel" destructiveButtonTitle:nil otherButtonTitles:@"Share on Facebook", @"Share on Twitter", nil];
[shareActionSheet showInView:self.view];
[cell hideUtilityButtonsAnimated:YES];
break;
}
case 1:
{
// Delete button is pressed
NSIndexPath *cellIndexPath = [self.tableView indexPathForCell:cell];
[patterns removeObjectAtIndex:cellIndexPath.row];
[patternImages removeObjectAtIndex:cellIndexPath.row];
[self.tableView deleteRowsAtIndexPaths:@[cellIndexPath] withRowAnimation:UITableViewRowAnimationLeft];
break;
}
default:
break;
}
}
And again, the code is simple and straightforward. When the More button is clicked, the application displays an action sheet containing a list of social networks for sharing. For the “delete” option, we just need to remove the item from the list of patterns and the patternImages array, and then call the deleteRowsAtIndexPaths: method to remove the record from the displayed table.
That's all! Now you can compile and run your application. The option buttons should work something like this:

To summarize
SWTableViewCell makes it very easy to implement the swipe-for-options function for tables. When developing your next application, consider implementing this feature. I think this will raise the level of your application for the user.
And although we can implement this functionality using many third-party libraries or implement it on our own , I hope that Apple will consider providing this tool to developers in future versions of iOS.
You can download the finished project if you suddenly need it.