
About creating a platform game on Unity. Part 4.1 villainous
- Tutorial
Hello, Habr!
With the last article in this series enough time has passed (and with prior to her articles - even more), so it's time to come back and tell you how to make the most treacherous and villainous part of any computer game - enemies. I will make a reservation in advance: here we will not consider the creation of artificial intelligence for opponents of your character. Let's look at what types of opponents are most common in platform games and how to implement them using Unity.

Caution, under the cut, there are still a lot of gifs!
Disclaimer: all the code shown in the article is not a role model, an example of an ideal code and other fictional and non-existent things. The practices applied in the article may be one of many solutions to a specific problem. And they may not be.
So let's go. I have identified four main types of objects that can somehow prevent the hero of your game from reaching the goal:
1) Static (rotating saws, “deadly” blocks, etc.)

2) Walking on platforms (mushrooms and turtles from Super Mario Bros)
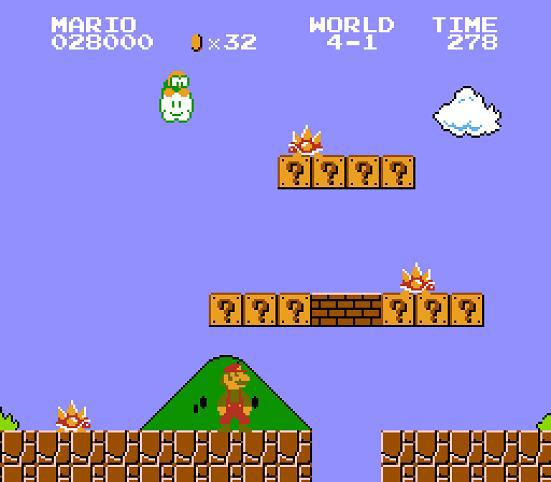
3) Flying ( crows from the second level Ghosts and Goblins)

4) Shooting (Bauzha fromhis castle of the same Mario)

There are still bosses, but they can combine all of the above types, so we will not dwell on them.
1) Static obstacles.
Let's make a rotating saw. To realize such an “enemy” you need just a couple of things - a sprite saw and a script that will rotate it. No sooner said than done.
Drag and drop the sprite onto the scene:

Create a new script (this is, as usual, very simple)

And add a code that looks something like this:
The public variable speed sets the rotation speed. It is important to note that a positive value rotates the saw counterclockwise, and a negative value rotates the saw clockwise.
Now, in order to interact with the saw, add some kind of collider to it (this is described in detail in the most interesting previous parts) and change the object tag to some suitable one in this situation.
Tags are a good thing. We can assign the player the player tag, the enemy the enemy tag, and the level on the walls and floor. After this simple procedure, checking what we, for example, encountered, will be much simpler. And you can find any one (or everything that is on the scene) object with a specific tag. This is done like this:
An array of all objects with a given tag can be obtained using the FindGameObjectsWithTag method - nowhere is easier.
Let's go back to our saws and create a new tag for the enemies in the game.
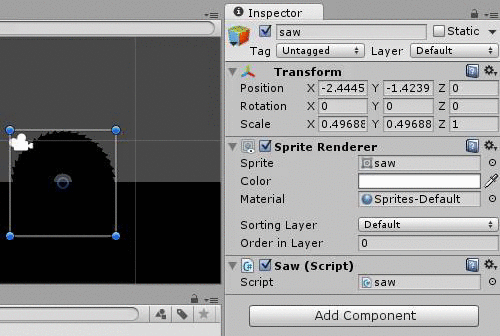
In the character script, add the following check
As you can see, everything is elementary: we check collisions, we check the tag of what we encountered. If everything is bad, restart the level. Or take life. Or something like that. You played platformers, right?
Here's how it all looks in the end:

So, simply and quickly, we created the first enemy - which, of course, is not an enemy, but it’s quite an obstacle. Go ahead.
2) Walking, crawling and other enemies moving on platforms.
It may seem to an unprepared reader that this type of enemy is more difficult to implement than the first. I hasten to reassure - this is not at all true. As in the previous case, we need some kind of sprite, a collider on it, a script and a platform on which all this will move. Only rigidbody2D will be added to this small list so that physics acts on the enemy and you can set his speed.
Unfortunately, I don’t know how to draw and my creative talent was enough only for such a villain:

To move it, use the following script
We set the speed of the enemy and the direction of his movement (-1 - to the left, 1 - to the right), which can be changed in a collision with walls, for example. Then it’s simple - we set the horizontal speed equal to the product of the speed and direction.
“But he looks to the right, but moves to the left!” - the attentive reader will notice. Let's fix this and deploy the sprite according to the direction of movement:
And we will teach you to unfold in a collision with a wall. To do this, make a couple of walls at the level with the wall tag and write collision processing. Here is one:
Now that everything is in place, the final result will look like this.
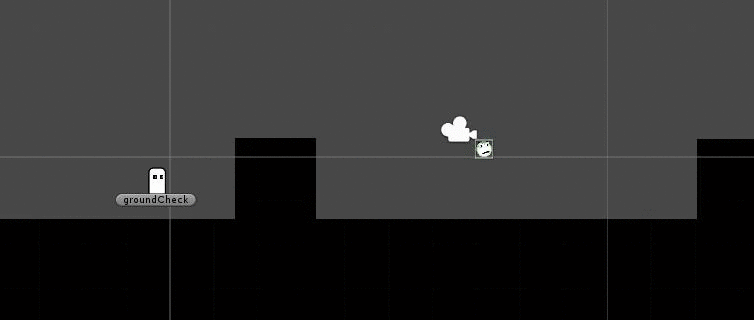
It remains only to add the Enemy tag to the enemy so that he really becomes dangerous for our character.
To summarize the subtotal. We examined how two types of “enemies” are created in 2D platformers: static and moving along the level. As you can see, this is really very simple and the basic implementation takes very little time.
In the next part I will tell you how to create the other two types of enemies - flying and shooting.
Stay tuned - it will be interesting!
Previous Series:
Part One, in which we learn to walk
Part Two, in which we learn to lose
Part Three, in which we have fun with physics
Take Microsoft virtual academy courses on creating games and other technologies.
Introduction to Unity games programming.
Exciting C # programming.
Download Unity.
Download free or trial Visual Studio.
Become a developer of universal Windows applications.
Try Azure for 30 days!
With the last article in this series enough time has passed (and with prior to her articles - even more), so it's time to come back and tell you how to make the most treacherous and villainous part of any computer game - enemies. I will make a reservation in advance: here we will not consider the creation of artificial intelligence for opponents of your character. Let's look at what types of opponents are most common in platform games and how to implement them using Unity.

Caution, under the cut, there are still a lot of gifs!
Disclaimer: all the code shown in the article is not a role model, an example of an ideal code and other fictional and non-existent things. The practices applied in the article may be one of many solutions to a specific problem. And they may not be.
So let's go. I have identified four main types of objects that can somehow prevent the hero of your game from reaching the goal:
1) Static (rotating saws, “deadly” blocks, etc.)

2) Walking on platforms (mushrooms and turtles from Super Mario Bros)
3) Flying ( crows from the second level Ghosts and Goblins)

4) Shooting (Bauzha from

There are still bosses, but they can combine all of the above types, so we will not dwell on them.
1) Static obstacles.
Let's make a rotating saw. To realize such an “enemy” you need just a couple of things - a sprite saw and a script that will rotate it. No sooner said than done.
Drag and drop the sprite onto the scene:

Create a new script (this is, as usual, very simple)

And add a code that looks something like this:
using UnityEngine;
using System.Collections;
public class rotator : MonoBehaviour {
public float speed = 0.04f;
void Update () {
transform.Rotate (new Vector3 (0f, 0f, speed * Time.deltaTime));
}
}
The public variable speed sets the rotation speed. It is important to note that a positive value rotates the saw counterclockwise, and a negative value rotates the saw clockwise.
Now, in order to interact with the saw, add some kind of collider to it (this is described in detail in the most interesting previous parts) and change the object tag to some suitable one in this situation.
Tags are a good thing. We can assign the player the player tag, the enemy the enemy tag, and the level on the walls and floor. After this simple procedure, checking what we, for example, encountered, will be much simpler. And you can find any one (or everything that is on the scene) object with a specific tag. This is done like this:
GameObject someEnemy = GameObject.FindGameObjectWithTag ("Enemy");
An array of all objects with a given tag can be obtained using the FindGameObjectsWithTag method - nowhere is easier.
Let's go back to our saws and create a new tag for the enemies in the game.
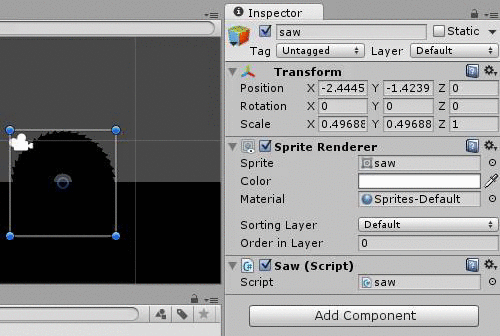
In the character script, add the following check
void OnCollisionEnter2D(Collision2D col){
if (col.gameObject.tag == "Enemy")
Application.LoadLevel (Application.loadedLevel);
}
As you can see, everything is elementary: we check collisions, we check the tag of what we encountered. If everything is bad, restart the level. Or take life. Or something like that. You played platformers, right?
Here's how it all looks in the end:

So, simply and quickly, we created the first enemy - which, of course, is not an enemy, but it’s quite an obstacle. Go ahead.
2) Walking, crawling and other enemies moving on platforms.
It may seem to an unprepared reader that this type of enemy is more difficult to implement than the first. I hasten to reassure - this is not at all true. As in the previous case, we need some kind of sprite, a collider on it, a script and a platform on which all this will move. Only rigidbody2D will be added to this small list so that physics acts on the enemy and you can set his speed.
Unfortunately, I don’t know how to draw and my creative talent was enough only for such a villain:

To move it, use the following script
using UnityEngine;
using System.Collections;
public class walkingEnemy : MonoBehaviour {
public float speed = 7f;
float direction = -1f;
// Use this for initialization
void Start () {
}
// Update is called once per frame
void Update () {
rigidbody2D.velocity = new Vector2 ( speed * direction, rigidbody2D.velocity.y);
}
We set the speed of the enemy and the direction of his movement (-1 - to the left, 1 - to the right), which can be changed in a collision with walls, for example. Then it’s simple - we set the horizontal speed equal to the product of the speed and direction.
Fun fact
Funny fact - if you put a fixedAngle checkbox on rigidbody2D, then the enemy will crawl, and if you remove it, then


“But he looks to the right, but moves to the left!” - the attentive reader will notice. Let's fix this and deploy the sprite according to the direction of movement:
using UnityEngine;
using System.Collections;
public class walkingEnemy : MonoBehaviour {
public float speed = 7f;
float direction = -1f;
// Use this for initialization
void Start () {
}
// Update is called once per frame
void Update () {
rigidbody2D.velocity = new Vector2 ( speed * direction, rigidbody2D.velocity.y);
transform.localScale = new Vector3 (direction, 1, 1);
}
}
And we will teach you to unfold in a collision with a wall. To do this, make a couple of walls at the level with the wall tag and write collision processing. Here is one:
void OnCollisionEnter2D(Collision2D col){
if (col.gameObject.tag == "Wall")
direction *= -1f;
}
Now that everything is in place, the final result will look like this.
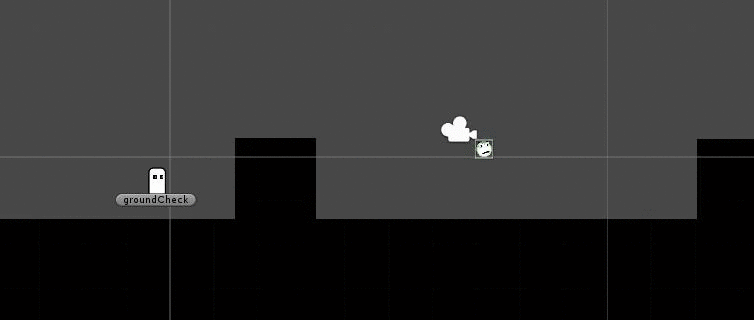
It remains only to add the Enemy tag to the enemy so that he really becomes dangerous for our character.
To summarize the subtotal. We examined how two types of “enemies” are created in 2D platformers: static and moving along the level. As you can see, this is really very simple and the basic implementation takes very little time.
In the next part I will tell you how to create the other two types of enemies - flying and shooting.
Stay tuned - it will be interesting!
Previous Series:
Part One, in which we learn to walk
Part Two, in which we learn to lose
Part Three, in which we have fun with physics
Some more useful links
Take Microsoft virtual academy courses on creating games and other technologies.
Introduction to Unity games programming.
Exciting C # programming.
Download Unity.
Download free or trial Visual Studio.
Become a developer of universal Windows applications.
Try Azure for 30 days!