Develop React applications using ReasonReact
- Transfer
Do you use React to create user interfaces? The author of the material, the translation of which we publish, says that he also works with React. Here he wants to talk about why ReasonML should be used to write React applications .
React is a very good tool for developing interfaces. Can you make it even better? In order to improve your work with React, you first need to understand its main problems. In particular, the problem, the source of which is the fact that React is a JavaScript library.

If you look at React, you can see that some of the principles underlying this library are foreign to JavaScript. In particular, we are talking about immunity, the principles of functional programming and the type system.
Immunity is one of the basic principles of React. Mutations of the properties of the components or the state of the application are highly undesirable, since this can lead to unpredictable consequences. There are no standard mechanisms in JavaScript to ensure immunity. Data structures make them immutable either by adhering to certain conventions or by using libraries like immutable-js .
The React library is based on the principles of functional programming, since React applications are combinations of functions. Although JavaScript has some functional programming features, such as first-class functions, it is not a functional programming language. If you need to write good declarative code in JavaScript, you have to resort to third-party libraries like Lodash / fp or Ramda .
What's wrong with the type system? React has the concept of PropTypes. It is used to simulate types in JavaScript, since this language, by itself, is not statically typed. In order to use the benefits of static typing in JS, again, you have to resort to third-party tools, such as Flow and TypeScript .
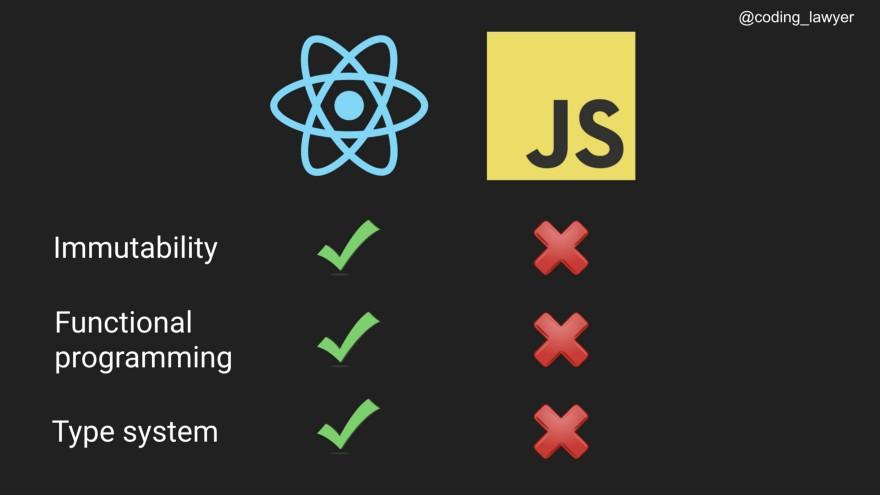
Comparing React and JavaScript
As you can see, JavaScript is not compatible with the basic principles of React.
Is there a programming language that better javascript is consistent with React?
This question can be answered positively. This language is ReasonML .
Immunity is implemented in Reason. Since it is based on OCaml , a functional programming language, the corresponding capabilities are also embedded in Reason. This language also has its own type system, suitable for React.

Comparing React, JavaScript and Reason
It turns out that Reason is compatible with the basic principles of React.
Reason is not a new language. It is an alternative, JavaScript-like syntax and set of tools for OCaml, a functional programming language that has been around for over 20 years. Reason was created by Facebook developers who have already used OCaml in their projects ( Flow , Infer ).

OCaml
C-like syntax Reason makes OCaml accessible to programmers who are familiar with common languages like JavaScript or Java. Reason gives the developer a higher-quality, in comparison with OCaml, documentation, an ever-growing community of enthusiastshas developed around him. In addition, what is written in Reason is easy to integrate with existing JS projects.

Reason
Reason is based on OCaml. Reason has the same semantics as OCaml, only syntax is different. This means that Reason makes it possible to write OCaml code using JavaScript-like syntax. As a result, the programmer has at his disposal such remarkable features of OCaml as a strict type system and pattern matching mechanism.
Take a look at the Reason code snippet in order to familiarize yourself with its syntax.
Although this snippet uses a pattern matching mechanism, it remains very similar to JavaScript.
The only language that works in browsers is JavaScript, therefore, in order to write for browsers in any language, we need to compile it in JavaScript.
One of the most interesting features of Reason is the BuckleScript compiler, which takes the code written in Reason and converts it into readable and productive JS code, moreover, cleansing it well from unused constructs.

BuckleScript The readability of
BuckleScript work results is useful if you work in a team in which not everyone is familiar with Reason. These people, at least, will be able to read the resulting JS code.
Reason code is sometimes so similar to JS code that the compiler does not need to convert it at all. Thanks to this state of affairs, you can enjoy the benefits of Reason's static typing and write code that looks like it is written in JavaScript.
Here is an example of code that will work in both Reason and JavaScript:
BuckleScript comes with four libraries. This is a standard library called Belt ( the OCaml standard library is not enough here), and bindings for JavaScript, Node.js, and for the DOM API.
Since BuckleScript is based on the OCaml compiler, compilation is very fast — much faster than Babel and several times faster than TypeScript.
Compile with BuckleScript the above Reason code fragment containing the function
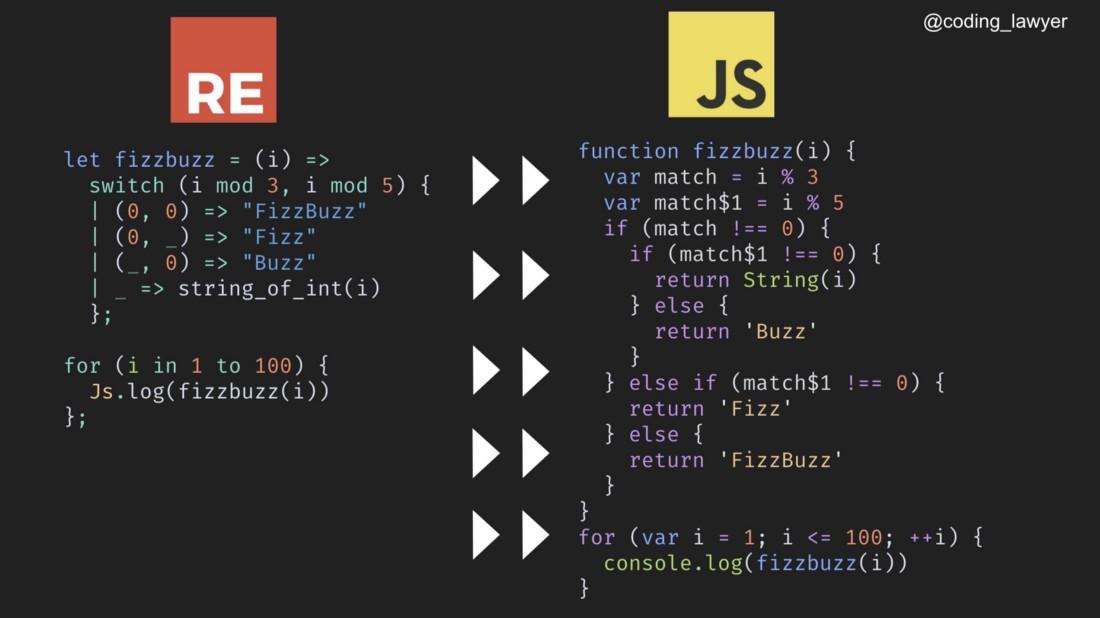
Compiling Reason code in JavaScript using BuckleScript
As you can see, the JS code was quite readable. It looks like it was written by man.
Programs written in Reason are compiled not only in JavaScript, but also in native code and byte code. As a result, for example, you can write an application on Reason and run it in a browser, on MacOS, on smartphones running Android and iOS. There is a game Gravitron , written by Jared Forsythe on Reason. It can be run on all the above platforms.
BuckleScript makes it possible to organize interaction between Reason and JavaScript. This means not only the ability to use working JS code in the Reason code base, but also the ability of code written in Reason to interact with this JavaScript code. As a result, code written in Reason can be easily integrated into existing JS projects. Moreover, in Reason-code, you can use JavaScript packages from NPM. For example, you can create a project in which Flow, TypeScript and Reason are shared.
However, everything is not so simple. In order to use JavaScript code or libraries in Reason, they must first be ported using Reason bindings. In other words, we need types for regular JavaScript code in order to use the strict Reason type system.
If you need to use any JavaScript library in Reason code, you should first go to the Reason Package Index ( Redex ) and find out if this library has already been ported to Reason. The Redex project is a directory of libraries and tools written in Reason and JavaScript libraries with Reason bindings. If you managed to find the required library in this directory, you can set it as a dependency and use it in a Reason application.
If you failed to find the necessary library, you will have to write your own bindings. If you are just starting to get acquainted with Reason, keep in mind that writing binders is not a newbie task. This is one of the most difficult tasks that those who program at Reason have to solve. In fact, this is a topic for a separate article.
If you only need some limited functionality of any JavaScript library, you do not need to write bindings for the entire such library. This can be done only for the necessary functions or components.
At the beginning of the material we talked about the fact that it is dedicated to the development of React-applications using Reason. You can do this thanks to the ReasonReact library .
Perhaps now you are thinking: “It is still incomprehensible to me - why do I need to write React-applications in Reason”. However, we have already discussed the main reason for using the React and Reason bundles, which is that React is better compatible with Reason than with JavaScript. Why is this so? The fact is that React was created in the calculation for Reason, or, more precisely, in the calculation for OCaml.

The first React prototype was developed by Facebook and was written on Standard Meta Language ( StandardML ), in a language that is a relative of OCaml. Then React was transferred to OCaml, in addition, React was transferred to JavaScript. This was done due to the fact that the entire web used JavaScript and it would probably be unwise to make statements like: “And now we will write a UI on OCaml”. The translation of React to JavaScript justified itself and led to the wide distribution of this library.
As a result, everyone is accustomed to perceive React as a JS library. React, as well as other libraries and languages, such as Elm , Redux , Recompose , Ramda , and PureScript, contributed to the popularization of functional programming style in javascript. And thanks to the proliferation of Flow and TypeScript in JavaScript, static typing has become popular. As a result, the functional programming paradigm using static types has become dominant in the world of frontend development.
In 2006, Bloomberg created and translated into the category of open source projects the BuckleScript compiler, which converts OCaml to JavaScript. This allowed them to write better and safer front-end code using the strict OCaml type system. They took an optimized and very fast OCaml compiler and forced it to generate JavaScript code.
The popularity of functional programming and the release of BuckleScript created the ideal climate that allowed Facebook to return to the original idea of React - a library that was originally written in StandardML.
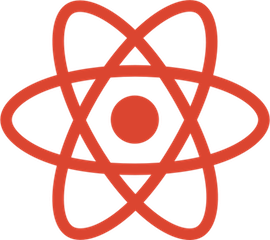
ReasonReact
They mixed OCaml semantics with JavaScript syntax and created Reason. In addition, they created a Reason wrapper for React, presented as a Reason React library, which has additional features, such as encapsulating Redux principles in stateful components. By doing this, they brought the React back to its roots .
When the React library was translated into JavaScript, the language capabilities were customized to the React needs by creating various libraries and tools. Such an approach, in particular, means the need for a large number of dependencies for projects. Let's not talk about the fact that such libraries are constantly evolving, and they regularly undergo changes that make their new versions incompatible with the old ones. As a result, the developer has to be very serious and cautious about the maintenance of the libraries on which his projects depend.
This adds an extra level of complexity to JavaScript development. For example, a typical React application typically contains, at a minimum, dependencies, which can be seen in the following figure.

Dependencies of a typical React application
These are the tasks that these dependencies solve:
Now we will use, instead of React for JavaScript, the ReasonReact library. Do we need, with this approach, all these dependencies?

Switching to ReasonReact
After analyzing the same list of tasks that were previously solved with the help of additional tools, we find out that all of them can be solved using the built-in ReasonReact tools. Details about them you can read here .
In the application prepared by ReasonReact, all these and many other dependencies are not needed. The fact is that many of the most important features that simplify development are already included in the language. As a result, work with dependencies is simplified, and as the application grows and develops, this work is not complicated.
All this is possible thanks to the use of the OCaml language, which is already more than 20 years old. It is a mature language, the basic principles and mechanisms of which are time-tested and stable.

If you come from the world of JavaScript, it will be easy for you to start working with Reason due to the fact that the syntax of this language is similar to JavaScript. If you have previously written React applications, it will be even easier for you to switch to Reason, since you can use all your knowledge in the React area when working with Reason React. ReasonReact is based on the same thinking model as React is based on, and the process of working with them is also very similar. This means that when you switch to Reason, you do not have to start from scratch. You will deal with Reason in the process.
The best way to start using Reason in your projects is to gradually introduce fragments written in Reason. As already mentioned, Reason code can be used in JS projects, as well as JS code in Reason projects. This approach is applicable when using ReasonReact. You can take a ReasonReact component and use it in a traditional React-application written in JavaScript.
It was such an incremental approach that was chosen by Facebook developers, who widely used Reason when developing the Facebook messenger .
If you want to write a React application using Reason and learn the basics of this language in practice, take a look at this material, where the design of Tic-tac-toe is developed step by step.
The creators of Reason had two options. The first was to take javascript and somehow improve it. If they chose this path - they would have to deal with the historical shortcomings of JS.
They, however, chose the second path associated with OCaml. They took OCaml, a mature language with excellent performance, and modified it to make it look like JavaScript.
React is also based on OCaml principles. That is why writing React-applications is much easier and more enjoyable using Reason. Working with React in Reason offers a more stable and secure approach to creating React components, since the strict type system insures the developer and does not have to deal with most of the historical problems of JavaScript.
Dear readers! Have you tried ReasonReact?


React and JavaScript
If you look at React, you can see that some of the principles underlying this library are foreign to JavaScript. In particular, we are talking about immunity, the principles of functional programming and the type system.
Immunity is one of the basic principles of React. Mutations of the properties of the components or the state of the application are highly undesirable, since this can lead to unpredictable consequences. There are no standard mechanisms in JavaScript to ensure immunity. Data structures make them immutable either by adhering to certain conventions or by using libraries like immutable-js .
The React library is based on the principles of functional programming, since React applications are combinations of functions. Although JavaScript has some functional programming features, such as first-class functions, it is not a functional programming language. If you need to write good declarative code in JavaScript, you have to resort to third-party libraries like Lodash / fp or Ramda .
What's wrong with the type system? React has the concept of PropTypes. It is used to simulate types in JavaScript, since this language, by itself, is not statically typed. In order to use the benefits of static typing in JS, again, you have to resort to third-party tools, such as Flow and TypeScript .
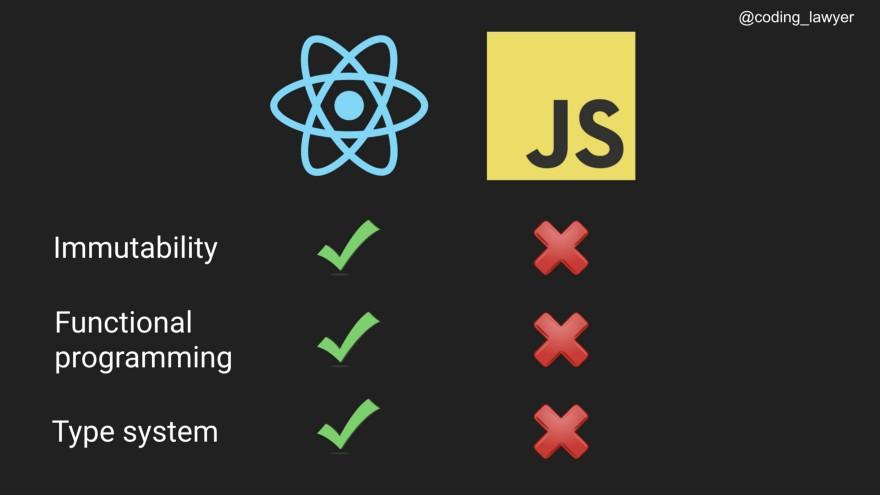
Comparing React and JavaScript
As you can see, JavaScript is not compatible with the basic principles of React.
Is there a programming language that better javascript is consistent with React?
This question can be answered positively. This language is ReasonML .
Immunity is implemented in Reason. Since it is based on OCaml , a functional programming language, the corresponding capabilities are also embedded in Reason. This language also has its own type system, suitable for React.

Comparing React, JavaScript and Reason
It turns out that Reason is compatible with the basic principles of React.
Reason
Reason is not a new language. It is an alternative, JavaScript-like syntax and set of tools for OCaml, a functional programming language that has been around for over 20 years. Reason was created by Facebook developers who have already used OCaml in their projects ( Flow , Infer ).

OCaml
C-like syntax Reason makes OCaml accessible to programmers who are familiar with common languages like JavaScript or Java. Reason gives the developer a higher-quality, in comparison with OCaml, documentation, an ever-growing community of enthusiastshas developed around him. In addition, what is written in Reason is easy to integrate with existing JS projects.

Reason
Reason is based on OCaml. Reason has the same semantics as OCaml, only syntax is different. This means that Reason makes it possible to write OCaml code using JavaScript-like syntax. As a result, the programmer has at his disposal such remarkable features of OCaml as a strict type system and pattern matching mechanism.
Take a look at the Reason code snippet in order to familiarize yourself with its syntax.
let fizzbuzz = (i) =>
switch (i mod 3, i mod 5) {
| (0, 0) =>"FizzBuzz"
| (0, _) =>"Fizz"
| (_, 0) =>"Buzz"
| _ => string_of_int(i)
};
for (i in1 to 100) {
Js.log(fizzbuzz(i))
};
Although this snippet uses a pattern matching mechanism, it remains very similar to JavaScript.
The only language that works in browsers is JavaScript, therefore, in order to write for browsers in any language, we need to compile it in JavaScript.
BuckleScript
One of the most interesting features of Reason is the BuckleScript compiler, which takes the code written in Reason and converts it into readable and productive JS code, moreover, cleansing it well from unused constructs.

BuckleScript The readability of
BuckleScript work results is useful if you work in a team in which not everyone is familiar with Reason. These people, at least, will be able to read the resulting JS code.
Reason code is sometimes so similar to JS code that the compiler does not need to convert it at all. Thanks to this state of affairs, you can enjoy the benefits of Reason's static typing and write code that looks like it is written in JavaScript.
Here is an example of code that will work in both Reason and JavaScript:
letadd = (a, b) => a + b;
add(6, 9);
BuckleScript comes with four libraries. This is a standard library called Belt ( the OCaml standard library is not enough here), and bindings for JavaScript, Node.js, and for the DOM API.
Since BuckleScript is based on the OCaml compiler, compilation is very fast — much faster than Babel and several times faster than TypeScript.
Compile with BuckleScript the above Reason code fragment containing the function
fizzbuzz()
in JavaScript.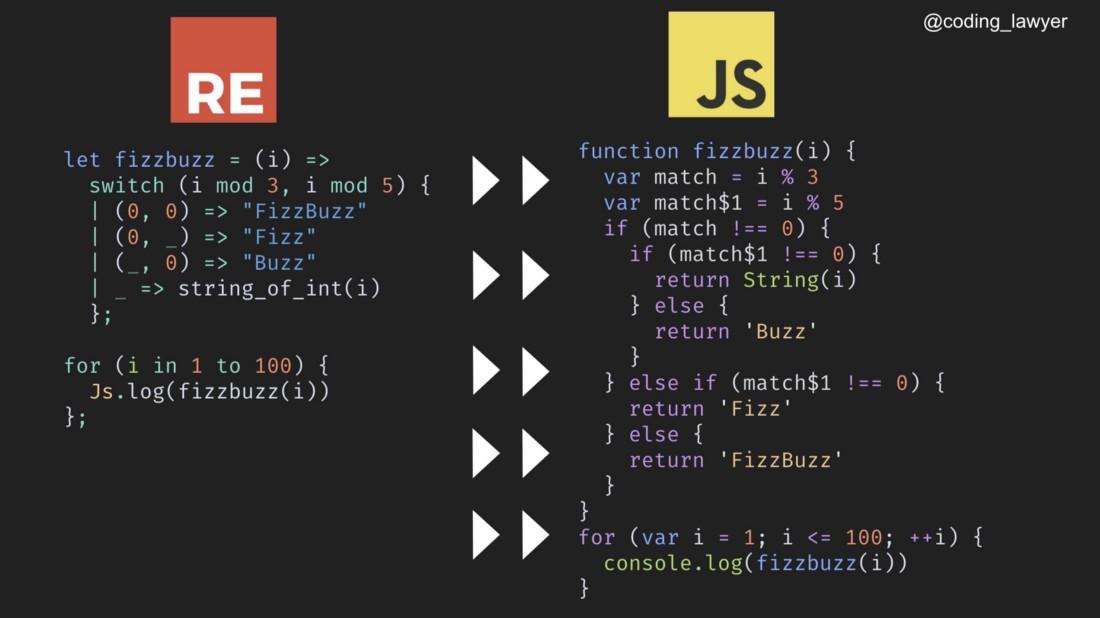
Compiling Reason code in JavaScript using BuckleScript
As you can see, the JS code was quite readable. It looks like it was written by man.
Programs written in Reason are compiled not only in JavaScript, but also in native code and byte code. As a result, for example, you can write an application on Reason and run it in a browser, on MacOS, on smartphones running Android and iOS. There is a game Gravitron , written by Jared Forsythe on Reason. It can be run on all the above platforms.
Organization of interaction with JavaScript
BuckleScript makes it possible to organize interaction between Reason and JavaScript. This means not only the ability to use working JS code in the Reason code base, but also the ability of code written in Reason to interact with this JavaScript code. As a result, code written in Reason can be easily integrated into existing JS projects. Moreover, in Reason-code, you can use JavaScript packages from NPM. For example, you can create a project in which Flow, TypeScript and Reason are shared.
However, everything is not so simple. In order to use JavaScript code or libraries in Reason, they must first be ported using Reason bindings. In other words, we need types for regular JavaScript code in order to use the strict Reason type system.
If you need to use any JavaScript library in Reason code, you should first go to the Reason Package Index ( Redex ) and find out if this library has already been ported to Reason. The Redex project is a directory of libraries and tools written in Reason and JavaScript libraries with Reason bindings. If you managed to find the required library in this directory, you can set it as a dependency and use it in a Reason application.
If you failed to find the necessary library, you will have to write your own bindings. If you are just starting to get acquainted with Reason, keep in mind that writing binders is not a newbie task. This is one of the most difficult tasks that those who program at Reason have to solve. In fact, this is a topic for a separate article.
If you only need some limited functionality of any JavaScript library, you do not need to write bindings for the entire such library. This can be done only for the necessary functions or components.
ReasonReact
At the beginning of the material we talked about the fact that it is dedicated to the development of React-applications using Reason. You can do this thanks to the ReasonReact library .
Perhaps now you are thinking: “It is still incomprehensible to me - why do I need to write React-applications in Reason”. However, we have already discussed the main reason for using the React and Reason bundles, which is that React is better compatible with Reason than with JavaScript. Why is this so? The fact is that React was created in the calculation for Reason, or, more precisely, in the calculation for OCaml.
Path to ReasonReact

The first React prototype was developed by Facebook and was written on Standard Meta Language ( StandardML ), in a language that is a relative of OCaml. Then React was transferred to OCaml, in addition, React was transferred to JavaScript. This was done due to the fact that the entire web used JavaScript and it would probably be unwise to make statements like: “And now we will write a UI on OCaml”. The translation of React to JavaScript justified itself and led to the wide distribution of this library.
As a result, everyone is accustomed to perceive React as a JS library. React, as well as other libraries and languages, such as Elm , Redux , Recompose , Ramda , and PureScript, contributed to the popularization of functional programming style in javascript. And thanks to the proliferation of Flow and TypeScript in JavaScript, static typing has become popular. As a result, the functional programming paradigm using static types has become dominant in the world of frontend development.
In 2006, Bloomberg created and translated into the category of open source projects the BuckleScript compiler, which converts OCaml to JavaScript. This allowed them to write better and safer front-end code using the strict OCaml type system. They took an optimized and very fast OCaml compiler and forced it to generate JavaScript code.
The popularity of functional programming and the release of BuckleScript created the ideal climate that allowed Facebook to return to the original idea of React - a library that was originally written in StandardML.
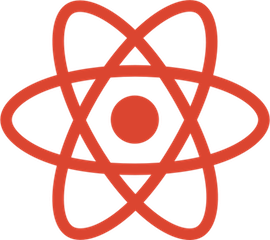
ReasonReact
They mixed OCaml semantics with JavaScript syntax and created Reason. In addition, they created a Reason wrapper for React, presented as a Reason React library, which has additional features, such as encapsulating Redux principles in stateful components. By doing this, they brought the React back to its roots .
React React Features
When the React library was translated into JavaScript, the language capabilities were customized to the React needs by creating various libraries and tools. Such an approach, in particular, means the need for a large number of dependencies for projects. Let's not talk about the fact that such libraries are constantly evolving, and they regularly undergo changes that make their new versions incompatible with the old ones. As a result, the developer has to be very serious and cautious about the maintenance of the libraries on which his projects depend.
This adds an extra level of complexity to JavaScript development. For example, a typical React application typically contains, at a minimum, dependencies, which can be seen in the following figure.

Dependencies of a typical React application
These are the tasks that these dependencies solve:
- Static typing - Flow / TypeScript.
- Immunity - ImmutableJS.
- Routing - ReactRouter.
- Code Formatting - Prettier.
- Lintting - ESLint.
- Auxiliary functions - Ramda / Lodash.
Now we will use, instead of React for JavaScript, the ReasonReact library. Do we need, with this approach, all these dependencies?

Switching to ReasonReact
After analyzing the same list of tasks that were previously solved with the help of additional tools, we find out that all of them can be solved using the built-in ReasonReact tools. Details about them you can read here .
In the application prepared by ReasonReact, all these and many other dependencies are not needed. The fact is that many of the most important features that simplify development are already included in the language. As a result, work with dependencies is simplified, and as the application grows and develops, this work is not complicated.
All this is possible thanks to the use of the OCaml language, which is already more than 20 years old. It is a mature language, the basic principles and mechanisms of which are time-tested and stable.
What's next?

If you come from the world of JavaScript, it will be easy for you to start working with Reason due to the fact that the syntax of this language is similar to JavaScript. If you have previously written React applications, it will be even easier for you to switch to Reason, since you can use all your knowledge in the React area when working with Reason React. ReasonReact is based on the same thinking model as React is based on, and the process of working with them is also very similar. This means that when you switch to Reason, you do not have to start from scratch. You will deal with Reason in the process.
The best way to start using Reason in your projects is to gradually introduce fragments written in Reason. As already mentioned, Reason code can be used in JS projects, as well as JS code in Reason projects. This approach is applicable when using ReasonReact. You can take a ReasonReact component and use it in a traditional React-application written in JavaScript.
It was such an incremental approach that was chosen by Facebook developers, who widely used Reason when developing the Facebook messenger .
If you want to write a React application using Reason and learn the basics of this language in practice, take a look at this material, where the design of Tic-tac-toe is developed step by step.
Results
The creators of Reason had two options. The first was to take javascript and somehow improve it. If they chose this path - they would have to deal with the historical shortcomings of JS.
They, however, chose the second path associated with OCaml. They took OCaml, a mature language with excellent performance, and modified it to make it look like JavaScript.
React is also based on OCaml principles. That is why writing React-applications is much easier and more enjoyable using Reason. Working with React in Reason offers a more stable and secure approach to creating React components, since the strict type system insures the developer and does not have to deal with most of the historical problems of JavaScript.
Dear readers! Have you tried ReasonReact?
