
SDK and YotaPhone Architecture Features
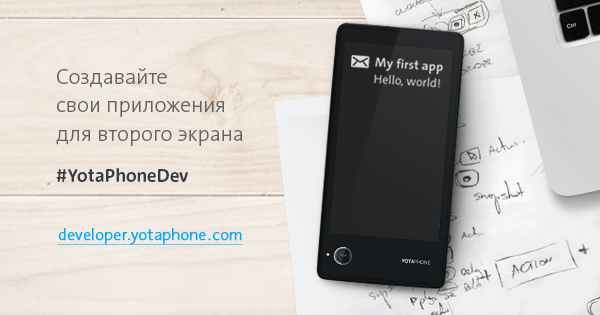
Hi, Habr. It so happened that basically the posts on our blog were written for potential users and just interested in the development of YotaPhone. And for software developers, we published much less information, which we want to fix: today we will tell you about the features of developing applications for YotaPhone and the tools we created for this.
Developer Tools
First, a few words about the development tools for YotaPhone. You will need to install the Android SDK , on top of which to put the YotaPhone SDK. Detailed installation and configuration instructions for different operating systems can be found on our website for developers .
We tried to make the YotaPhone SDK as clear and convenient as possible for any Android developer, so that the terminology and internal logic are closest to those in the Android SDK.
The second screen is made using electronic ink technology and has a number of features that must be considered when developing applications:
• The color space consists of 16 colors (shades of gray)
• A long image update time
• Ghosting effect, originally inherent in this technology
• Lack of matrix backlight
Application architecture
Before you start developing your first application for YotaPhone, familiarize yourself with the accepted software architecture. In general, it is similar to the traditional Android architecture:

Interaction scheme between architectural components:

A few words about each of the components of the presented scheme.
• Application Layer. This is the most important component for third-party developers, since the installation of their products is possible only within this level. It works on the same level with Google applications and services, and contains various additional applications, such as Organizer, Notepad, Wallpaper, etc. Interaction with the SDK occurs in both directions.
• SDK. This level is intended for use by applications specifically for YotaPhone. The SDK supports a number of high-level services for front-end applications in the form of Java classes. These services are also available when developing applications for the second display. The SDK contains two-way interfaces between services and additional applications.
• YotaPhone Manager. This is an important part of the architecture. This component controls the interaction between applications and low-level methods. Any third-party applications interact with the SDK through the YotaPhone Manager.
• YotaPhone Daemon. This is a low-level software component responsible for transferring commands from applications / services through YotaPhone Manager to the E-ink controller driver.
• E-ink controller driver. It was developed by the manufacturer and is responsible for the execution of commands received from applications, services or the OS.
• Android Framework. YotaPhone uses the standard Android framework, with minimal modifications.
The scheme of work with the second screen
The BSActivity (Back Screen Activity) class, based on the standard Android service, is responsible for displaying information on the second display of YotaPhone . When developing an application for the second display, you need to create your own class inherited from BSActivity and describe it in the AndroidManifest.xml file:
The life cycle of BSActivity itself can be represented as follows:

Launching the application on the second display is the same as launching the standard Android service:
mContext.startService(BSActivityIntent);
Let us describe the life cycle presented in more detail:
During startup, BSActivity receives a callback onBSCreate () and registers itself in YotaPhone Manager as an application for the second display, which receives the icon, title and becomes visible in the Task Manager. It appears with a long press on the touch zone of the second display.
When an application for the second display is launched or the user opens the task manager, YotaPhone Manager stops the current BSActivity by sending a call back onBSPause (), onBSStop (). After that, the application receives a call back onBSDestroy () (similar to onDestroy () in the standard Android service) and terminates.
In the call back onBSSaveInstanceState (Bundle savedInstanceState), which is called after onBSPause (), you can save any data. When the application on the second display receives a callback onBSPause (), it becomes inactive (cannot display images on the second display and receive information about control gestures from the touch zone of the second display). At the same time, at any time, the application can receive a call back onBSStop (). If you want to transfer information to BSActivity, then to access it you need to redefine the onHandleIntent (Intent intent) method at startup.
Features of displaying images on the second screen
As you remember, the screen on electronic ink has a number of features that must be considered when developing. A typical method for displaying an image on a second screen is as follows:
getBSDrawer().drawBitmap(bitmap, Waveform.WAVEFORM_GC_FULL);
The second argument here is waveform. This is a set of instructions for the display controller on how to draw the image. There are four main types of waveform in the YotaPhone SDK that can be combined with each other.
• GC_FULL. Used to completely refresh the display when the current image is replaced with a new one. Provides high image quality.
o 4 bits, 16 shades of gray
o ~ 600 ms
o The drawing process is accompanied by a single flickering of the display
o Low level of ghosting effect
• GC_PARTIAL. It is used to partially update the image. Only that part of the screen where the image has changed is redrawn.
o 4 bits, 16 shades of gray
o ~ 600 ms
o No flicker
o There is a ghosting effect
• DU (Direct update). A quick update method that supports only two colors: black and white. No shades are supported. This waveform is most conveniently used for menu selection indicators or for displaying text.
o 1 bit (black or white)
o ~ 250 ms
o No
flicker , only part of the image is updated o There is a ghosting effect
• A2. An even faster method, which is designed to quickly update the page or create a simple black and white animation. It supports only two colors - black and white.
o 1 bit black or white
o ~ 120 ms
o No
flicker , only part of the image is updated o There is a ghosting effect
Image Optimization on the Second Display
EPDs are very different from LCDs, and so images that look good on the main display can often look mediocre on the second. However, the YotaPhone SDK contains several methods to improve the quality of the display on the second display. In particular, you can adjust dithering (color mixing), sharpness, brightness and contrast.
Smoothing . Since electronic ink displays 16 colors (shades of gray), and LCD - 16,777,216 colors, mixing colors is the most logical step. The YotaPhone SDK supports two algorithms: Atkinson (used in most cases) and Floyd-Steinberg (for images with a lot of gradients).
You can apply two methods of applying color mixing:
•
bitmap = EinkUtils.ditherBitmap(bitmap, ATKINSON_DITHERING);
•
getBSDrawer().drawBitmap(0, 0, bitmap, BSDrawer.Waveform.WAVEFORM_GC_PARTIAL, EinkUtils.ATKINSON_DITHERING);
Sharpness . Electronic ink blurs the image somewhat, and sharpening helps combat this effect. In our algorithm, the twist matrix is used:

Example sharpening code for k = 0.15:
bitmap = BitmapUtils.sharpenBitmap(context, bitmap, 0.15f);
Contrast and brightness. Enhancing contrast also helps reduce the blur effect of the image on the second display. But it also affects the brightness, which must be lowered in this case.
Sample code to increase contrast with a factor of 1.2 and reduce brightness by 30:
bitmap = BitmapUtils.changeBitmapContrastBrightness(bitmap, 1.2f, -30)
An example of a standard image processing code for output to the second display:
1) Increase sharpness at k = 0.15
2) Increase contrast with a factor of 1.2 and decrease brightness by 30
3) Use the Atkinson blending algorithm to convert to a 16-color palette:
bitmap = BitmapUtils.prepareImageForBS(context, bitmap);
getBSDrawer().drawBitmap(0, 0, bitmap, BSDrawer.Waveform.WAVEFORM_GC_PARTIAL, EinkUtils.ATKINSON_DITHERING);
Notifications
One of the main advantages of the second display in YotaPhone is the ability to display custom notifications. There are three types of them, and the type of specific notification, its position on the screen and display time are determined by the parent application.
• Full screen notification. Best for displaying pictures or a comparable amount of text.

• Notification half screen. Always located at the bottom, mainly used for short text messages.

• Panel notifications. The smallest view may contain icons and text.

Full-screen and half-notice after 60 seconds are reduced to the size of the panel. After another 60 seconds, notifications are displayed only in the counter of unread notifications.
Rotation algorithm
Since YotaPhone has two touch surfaces, a very neat unlocking mechanism is required to prevent accidental actions by the user. To do this, we have developed a “switching algorithm” that uses information from the device’s sensors to determine its position in space and unlock the corresponding display. Thanks to this, the user has the impression of a single workspace on two displays, rather than two disparate devices.
Algorithm 1:
• The user performs some action with the smartphone -> the gyroscope and accelerometer
turn on • If the user does not turn the smartphone over for 4 seconds, the sensors are turned off
• If you do not turn over for 4 seconds -> the main display is locked and the second is unlocked (if this action is not blocked by software). The sensors continue to work for another 4 seconds
• If during these second 4 seconds the user flips the smartphone back -> the second display is locked, the main one is unlocked, the sensors turn off
• If you do not turn the smartphone over for the second 4 seconds -> the sensors are blocked, the main display turns off, the user continues to use the second display.
Algorithm 2:
• The user performs some action with the second display -> the gyroscope and accelerometer are turned on
• If the user does not turn the smartphone over for 4 seconds -> the sensors turn off, the state of the main display does not change, the user continues to use the second display
• If the user turns the phone over for 4 seconds -> the second display is locked, the main one turns on and unlocks, the sensors turn off.
Below is an example of a code that starts the switching algorithm after pressing a button on the main display, as a result of which the user expects to switch to the second display:
mButtonP2B.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
RotationAlgorithm.getInstance(getApplicationContext()
).issueStandardToastAndVibration(); //To let the user know that he is expected to rotate the phone now
RotationAlgorithm.getInstance(getApplicationContext()
).turnScreenOffIfRotated(); //Perform drawing on back screen here
}
});
Afterword
As you already noticed, we tried to make the work of application developers for YotaPhone as easy as possible. If you already have experience developing for Android, then mastering our SDK will not cause any difficulties.
Separately, we want to draw your attention to the development of applications for the second display. Features of electronic ink technology and the fact of having two displays on a smartphone require a careful approach to image adaptation. For a deeper study of this issue, we recommend that you refer to the appropriate section on our site for developers.
We are currently working on the SDK architecture for the second generation YotaPhone, which we have already substantially redesigned and improved.