
Unity3d. Lessons from Unity 3D Student (B25-B28)
- Tutorial
Hello. This is the final series of basic Unity 3D tutorials from Unity3DStudent . Then there will be two more intermediate lessons. Upd: the plans have changed, these lessons will not be, since the second lesson (in animation) is already quite outdated, and I don’t see the point of spreading the translation of only one lesson. Let him stay on his own study =)
Links to previous lessons:
The lesson talks about creating a simple button using the GUI Texture and mouse events.
If you want to create a simple user interface, you can use the GUI Texture object . Consider this object using the button creation example. First of all, we need two textures: for the button in the normal state and for the button in the state when the mouse cursor is over it. We will prepare the appropriate files and add them to the project.
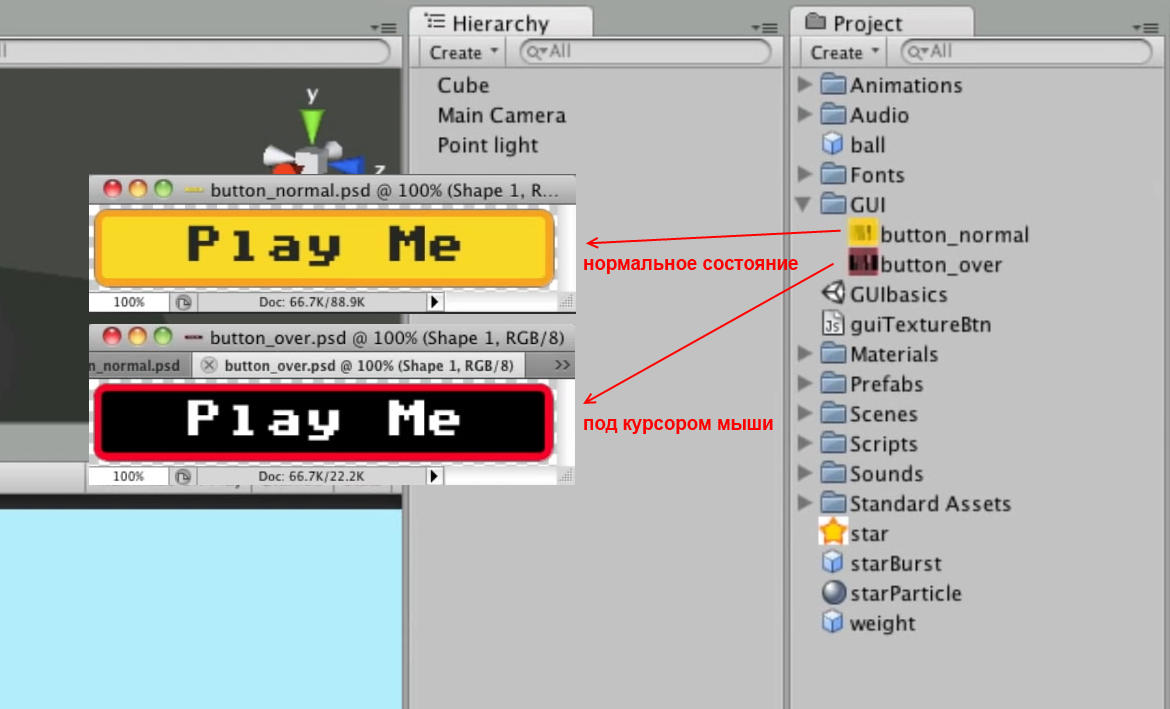
Now click on the button_normal file , and then create a GUI Texture from the menu GameObject - Create Other - GUI Texture . A new object will appear on the scene, on which the corresponding texture of the normal state of the button will be immediately applied.
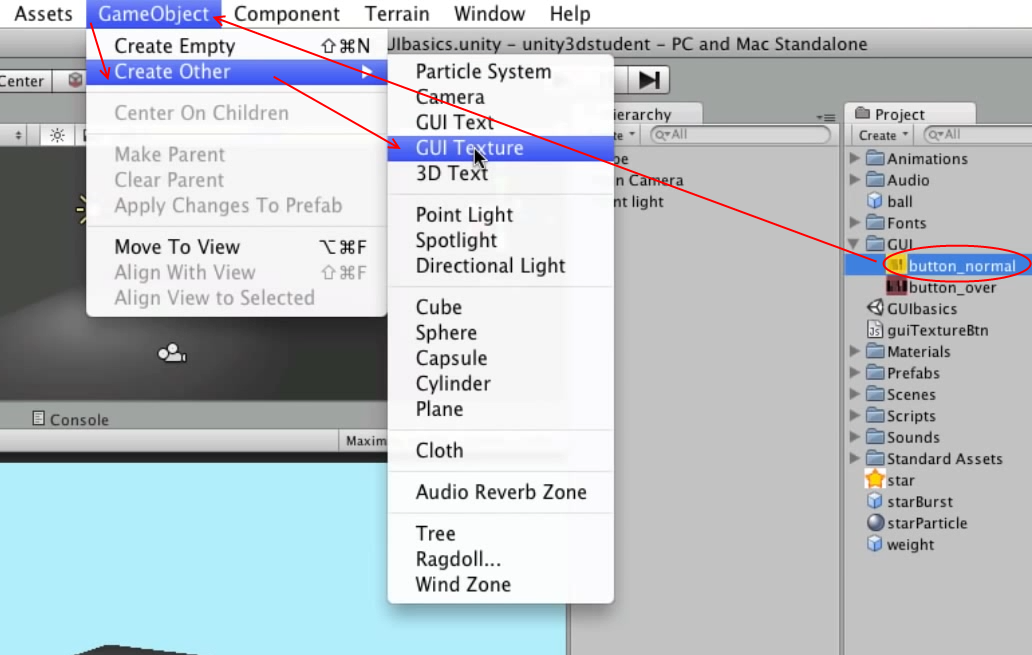

The created object consists of two components: Transform and GUITexture . Please note that changing the parameters of the Transform component works a little differently compared to other objects in the scene. In the Position and Scale parameters , only changes in the X and Y axes are processed , changes in the Z axis are ignored. Changes to the Rotation parameter are completely ignored. In addition, in the Position parametervalues are indicated not in world, but in screen coordinates, where 0,0 is the lower left corner of the screen, and 1,1 is the upper right corner of the screen. The default values are 0.5.0.5, i.e. center of the screen. In the GUITexture component, you can set the texture, color, additional offset from the edges of the screen and the border. Since we selected a specific texture before creating the object, Unity set these values ourselves. If desired, they can be changed.
So, we have a button in the center of the screen that does nothing yet. Let's animate it with the following script:
JavaScript code:
C # Code:
We set the variables normalTex and hoverTex to store, respectively, the texture of the normal state of the button and the texture of the button under the mouse cursor. Then we process three methods: OnMouseEnter (hovering the mouse over an object), OnMouseExit (exiting the mouse from the object) and OnMouseDown (clicking on the object). These methods are called after the corresponding mouse events if the object to which the script is attached is inherited from the GUIElement or Collider class . In the input / output methods of the mouse cursor, we set the corresponding texture to the object; in the click method, we simply write a message to the console.
We attach the script to our button, enter the texture files in the appropriate fields:
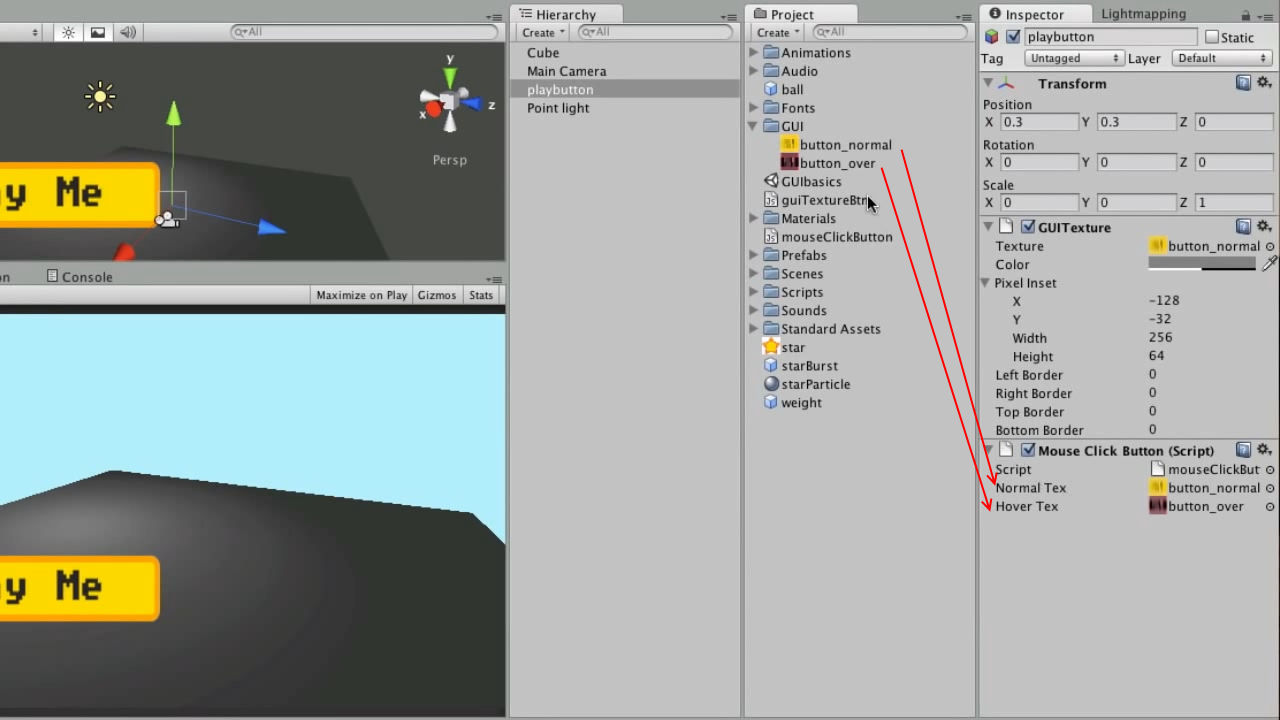
Run the scene and test the button. The button changes the texture when you hover over the mouse, when clicked, displays the message “clicked” in the console and changes the texture back when the cursor is displayed.
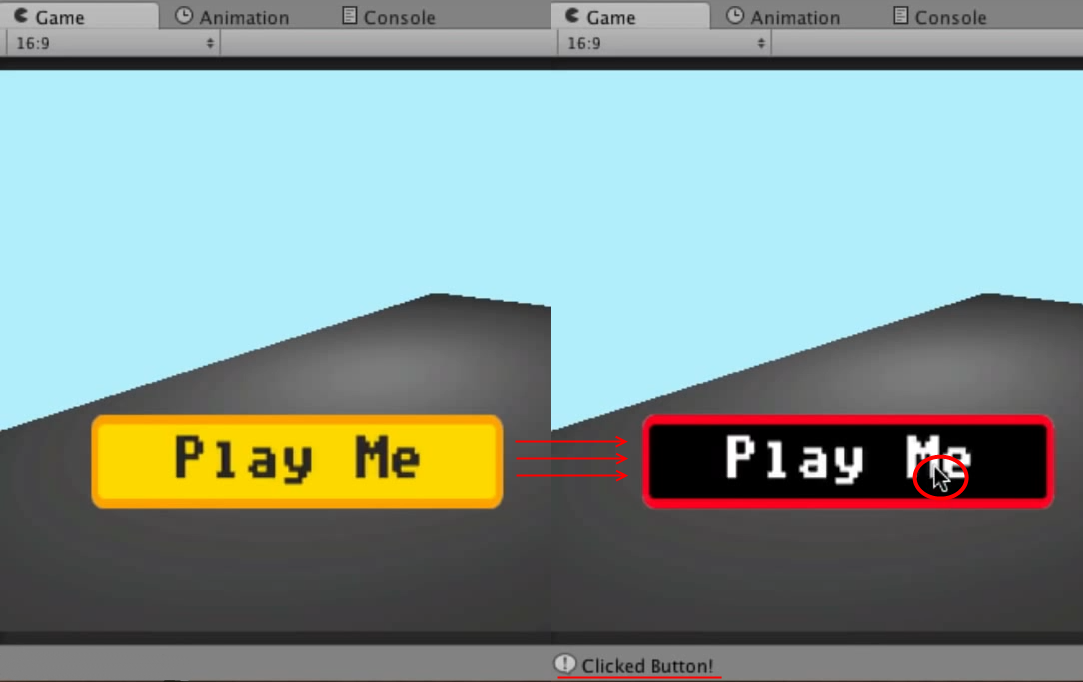
Link to the original lesson
A link to the documentation of the GUI Texture component
A link to the documentation of the OnMouseEnter method
A link to the documentation of the OnMouseExit method
A link to the documentation of the OnMouseDown method
The lesson shows how to limit values in a certain range using the Mathf.Clamp method.
When developing game mechanics, sometimes you need to limit a certain value within a given range of values. For example, you write some simple space shooter and you want the player-controlled ship not to fly outside the borders of the screen. The Clamp method of the Mathf class will help us with this .
Consider using this method on a stage where we have a camera, a light source and a simple Cube .

We realize the possibility of moving the cube along the X axis , and it will be possible to move only in the range from X = -10 to X= 10. To do this, write the following script and add it to the cube:
JavaScript code:
C # Code:
We move the cube using the value of the virtual X axis and the Transform.Translate method , and then limit the position of the cube along the X axis by calling the Mathf.Clamp method . The method accepts three parameters: a limited value, a minimum range boundary and a maximum range boundary.
We start the scene, press A / D on the keyboard or left-right arrows. The cube moves, and it cannot move beyond the borders of the range X = -10..10 (to be sure of this, you can add the position values along the X axis to the console at the end of the Update method - Debug.Log (transform.position.x); - comment of a translator) .
Link to the original lesson
Link to the documentation of the Mathf.Clamp method
The lesson discusses the use of the Time.timeScale property to pause / resume a game.
When developing a game, you will probably want to give players the opportunity to pause it and then resume it. To do this, just work a little with the static timeScale property of the Time class .
For example, we have a scene where a small ball jumps across the floor and we want to pause / resume its movement. First, we need an object on which we will “hang” a pause implementation script. To do this, you can create an empty game object ( GameObject menu - Create Empty ), place it in any convenient place and name it, for example, Manager .
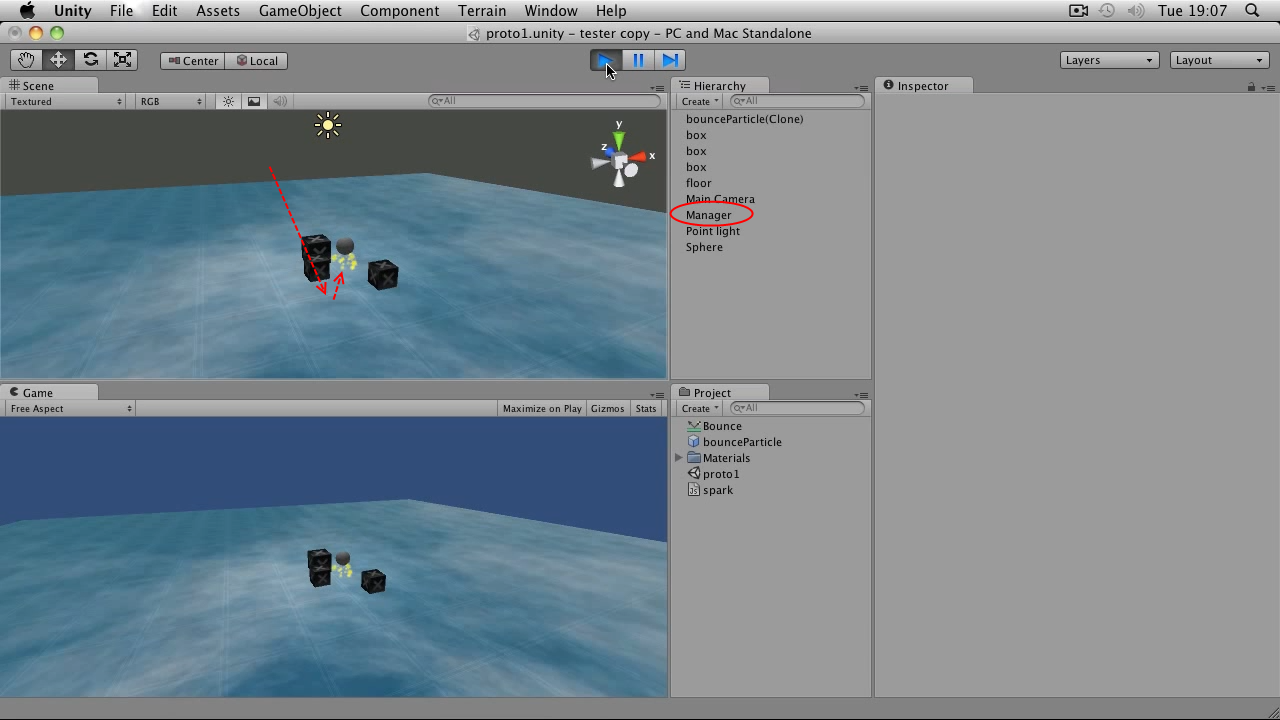
Now create a new script and write the following in it:
JavaScript Code:
C # Code:
In the script we made variable paused for control, pause or not. We pause on / off in the Update method by pressing the “Jump” button - with standard input settings, this is the space bar. Next, we check if the pause is off? If so, set the Time.timeScale property to 0 , which will stop the flow of time in the game; and we mean that the pause is on - paused = true . Otherwise, set Time.timeScale to 1 and turn off the pause. The Time.timeScale property can also be set between 0 and 1 and greater than 1, which will, accordingly, slow down and speed up the passage of time if you need it.
Add a script to the Manager object , run the scene. At any time, click on the space bar - the ball stops. Repeated pressing returns the paused movement of the ball.
Link to the original lesson
Link to the documentation for the Time.timeScale property
The lesson describes how to use the SendMessage method of the GameObject class to call the script method of another game object.
There are different ways to connect from the script of one game object to the script of another game object. Earlier, we examined the GetComponent method , with which you can get the components of an object, including its scripts. An alternative to this would be to use the SendMessage method .
Consider a scene with a blue Ball falling onto a red Switch box . Next to them hangs a black Block in the air . Let's make the block change the texture when the ball falls on the box and also falls down.
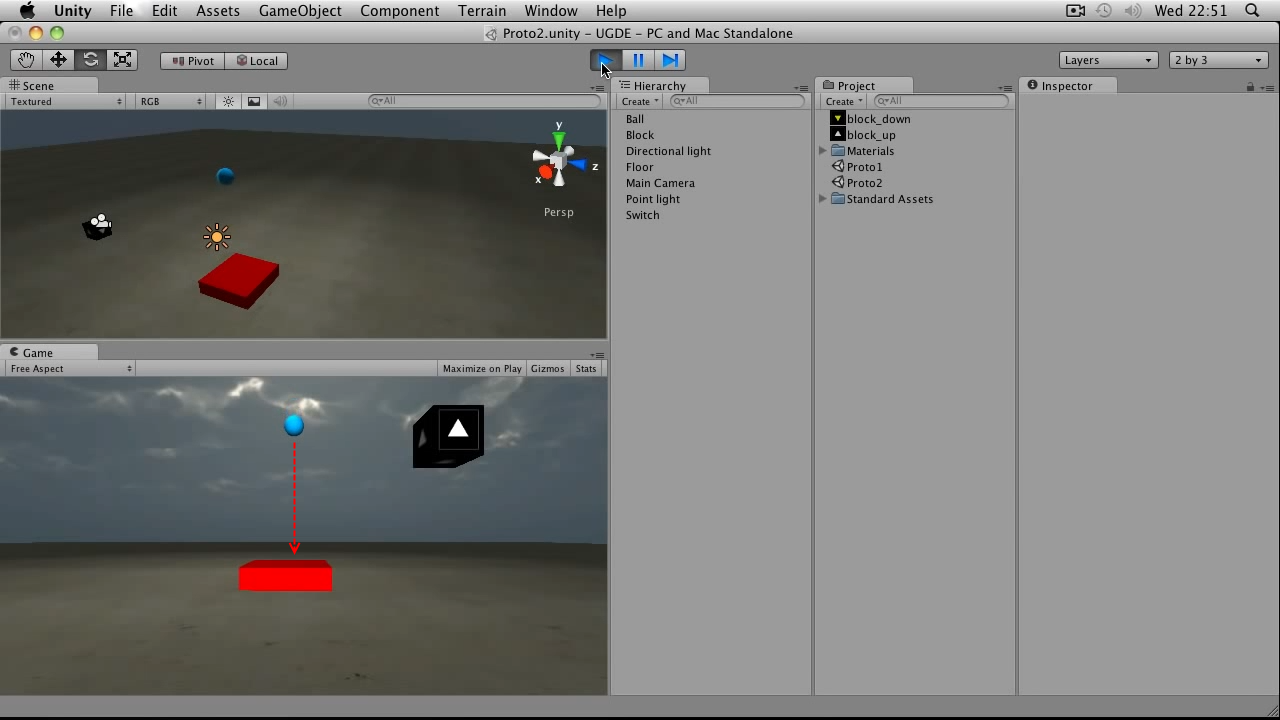
First we need to write a script for the block. Create a new script, call it Reactor and write the following:
JavaScript code:
C # Code:
We declared the variable downTexture for texture storage and implemented the React method , in which we change the texture of the object, make a short delay of 1 second and add the Rigidbody component of the solid body so that the object begins to be affected by gravity. We immediately add this script to the Block object and drag any suitable picture into the Down Texture script field . In the lesson, this is an image of a yellow arrow pointing down on a black background.

Take your time to run the scene, nothing will change on it for now. Next we need to make a script for the Ball . Call it Switcher and write it inside:
JavaScript code:
C # Code:
We implemented the OnCollisionEnter method , which will be called when the collider of the ball collides with the collider of another object. We are interested in a collision with the Switch box , so we first do the corresponding check. If so, we look for the Block object using the GameObject.Find method , specifying the name of the block. For the found object, the SendMessage method is called , in which the name of the method we want to call ( React ) is passed , after which, accordingly, this method executes.
Add a script to the ball, run the scene. Now, when a ball falls on a box, the block changes its texture and after a second also falls down.

Note from the translator:One of the downsides of using SendMessage is its potential performance degradation compared to calling methods directly. Here on this page dedicated to performance issues, we talk about the difference of 100 times in favor of a direct call. Therefore, this method should be used with caution (like the GetComponent , Find , etc. methods ), and if its use really becomes the culprit of a performance drop, use faster solutions.
Link to the original lesson
Link to documentation of the GameObject.SendMessage method
Links to previous lessons:
Base Lesson 25 - Texture GUI Object and Mouse Events
The lesson talks about creating a simple button using the GUI Texture and mouse events.
If you want to create a simple user interface, you can use the GUI Texture object . Consider this object using the button creation example. First of all, we need two textures: for the button in the normal state and for the button in the state when the mouse cursor is over it. We will prepare the appropriate files and add them to the project.
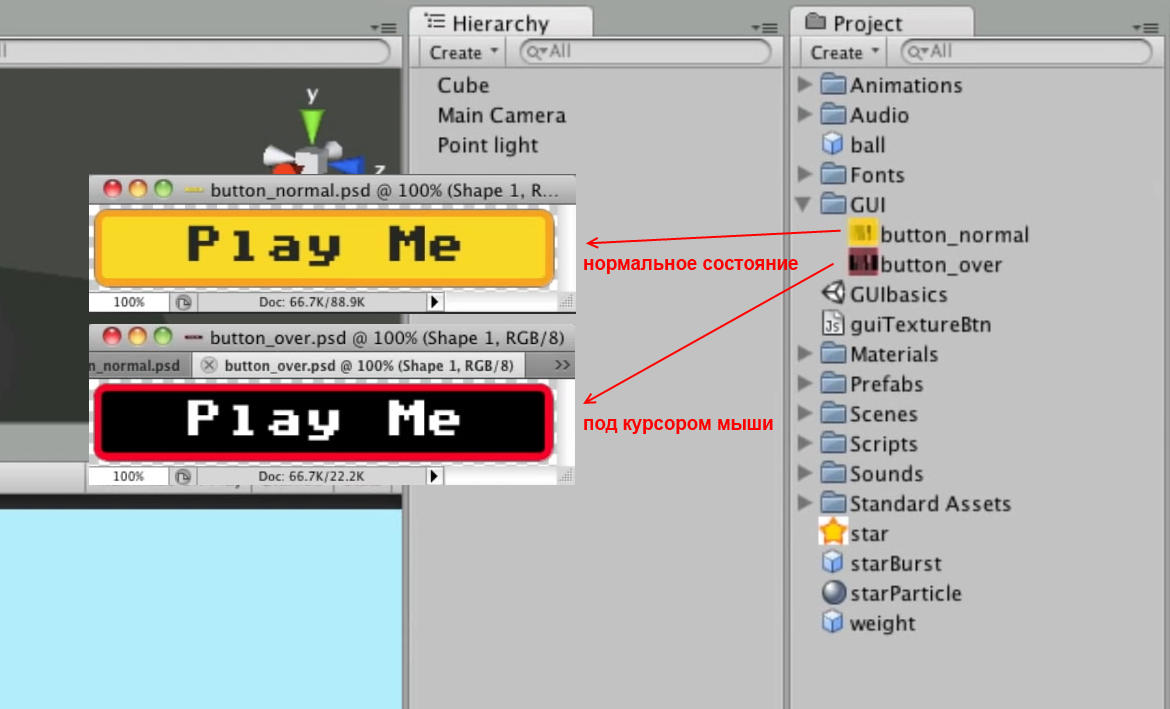
Now click on the button_normal file , and then create a GUI Texture from the menu GameObject - Create Other - GUI Texture . A new object will appear on the scene, on which the corresponding texture of the normal state of the button will be immediately applied.
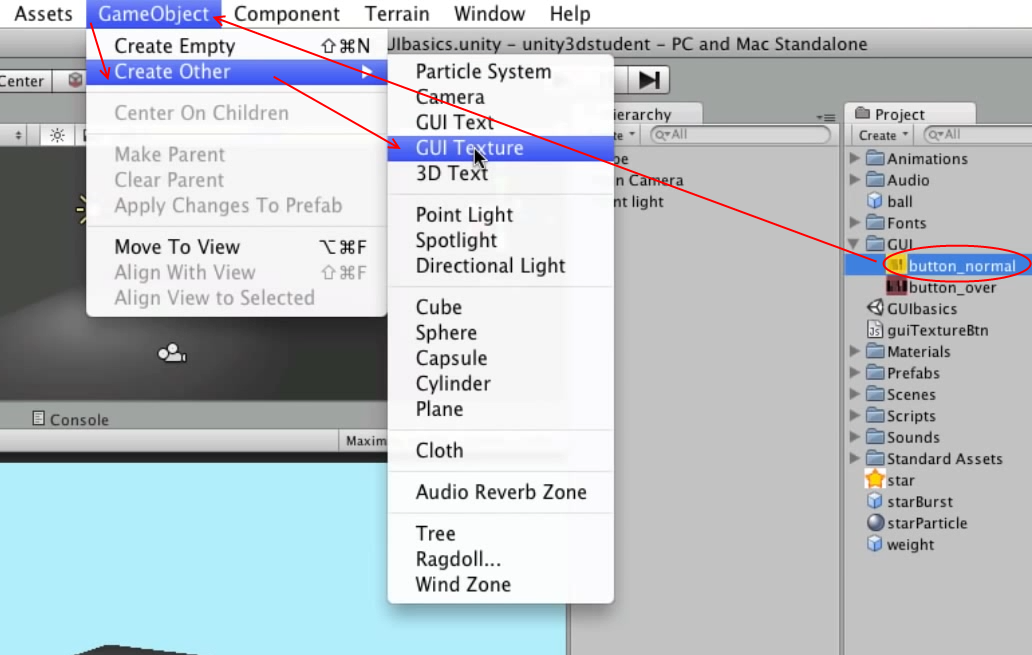

The created object consists of two components: Transform and GUITexture . Please note that changing the parameters of the Transform component works a little differently compared to other objects in the scene. In the Position and Scale parameters , only changes in the X and Y axes are processed , changes in the Z axis are ignored. Changes to the Rotation parameter are completely ignored. In addition, in the Position parametervalues are indicated not in world, but in screen coordinates, where 0,0 is the lower left corner of the screen, and 1,1 is the upper right corner of the screen. The default values are 0.5.0.5, i.e. center of the screen. In the GUITexture component, you can set the texture, color, additional offset from the edges of the screen and the border. Since we selected a specific texture before creating the object, Unity set these values ourselves. If desired, they can be changed.
So, we have a button in the center of the screen that does nothing yet. Let's animate it with the following script:
JavaScript code:
var normalTex : Texture2D;
var hoverTex : Texture2D;
function OnMouseEnter () {
guiTexture.texture = hoverTex;
}
function OnMouseExit(){
guiTexture.texture = normalTex;
}
function OnMouseDown(){
Debug.Log("clicked");
}
C # Code:
public Texture2D normalTex;
public Texture2D hoverTex;
private void OnMouseEnter()
{
guiTexture.texture = hoverTex;
}
private void OnMouseExit()
{
guiTexture.texture = normalTex;
}
private void OnMouseDown()
{
Debug.Log("clicked");
}
We set the variables normalTex and hoverTex to store, respectively, the texture of the normal state of the button and the texture of the button under the mouse cursor. Then we process three methods: OnMouseEnter (hovering the mouse over an object), OnMouseExit (exiting the mouse from the object) and OnMouseDown (clicking on the object). These methods are called after the corresponding mouse events if the object to which the script is attached is inherited from the GUIElement or Collider class . In the input / output methods of the mouse cursor, we set the corresponding texture to the object; in the click method, we simply write a message to the console.
We attach the script to our button, enter the texture files in the appropriate fields:
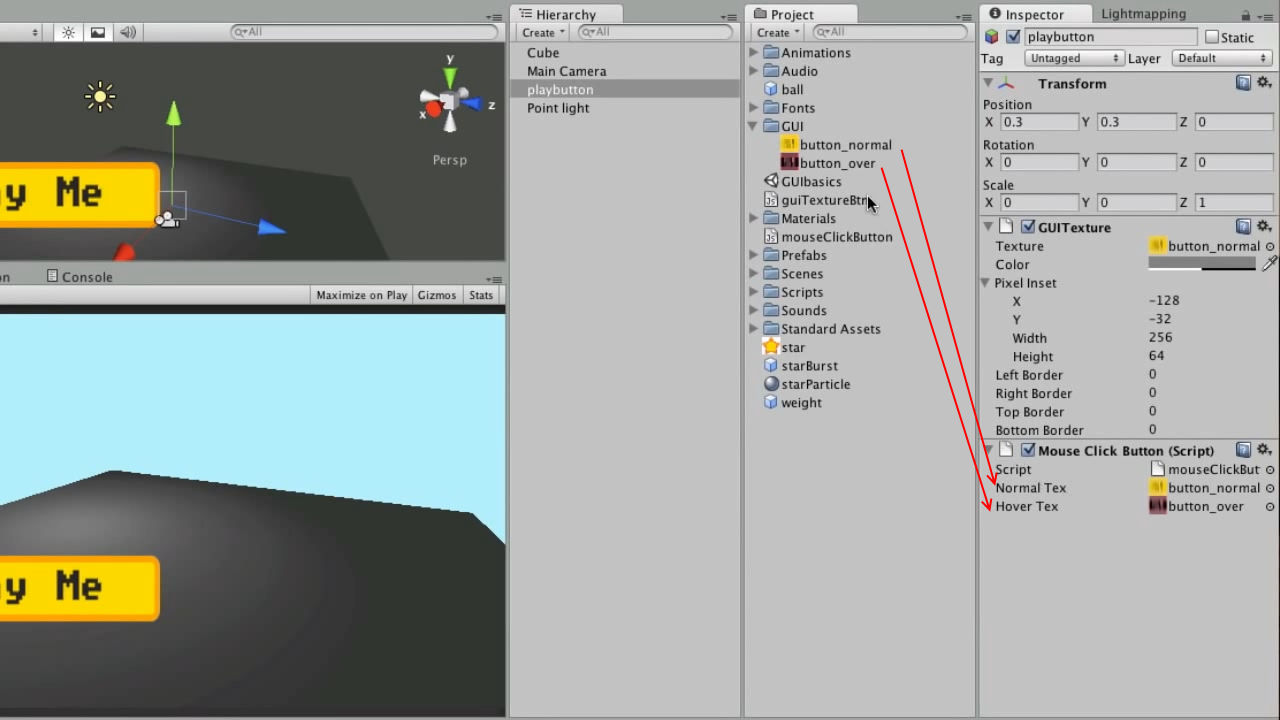
Run the scene and test the button. The button changes the texture when you hover over the mouse, when clicked, displays the message “clicked” in the console and changes the texture back when the cursor is displayed.
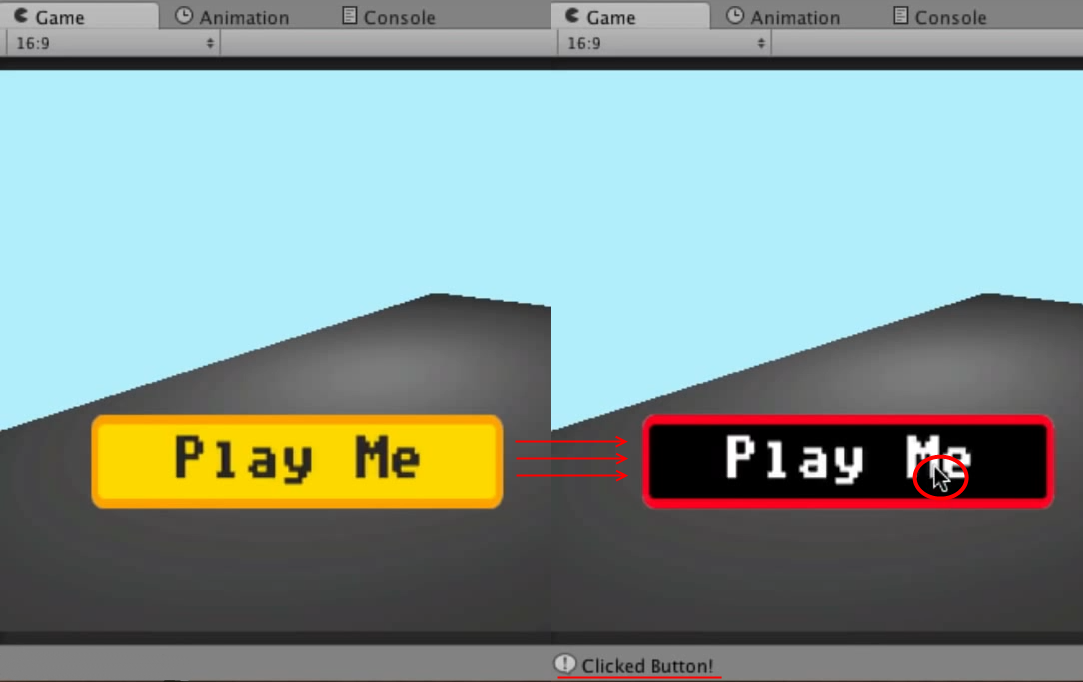
Link to the original lesson
Additional materials:
A link to the documentation of the GUI Texture component
A link to the documentation of the OnMouseEnter method
A link to the documentation of the OnMouseExit method
A link to the documentation of the OnMouseDown method
Base Lesson 26 - Using the Mathf.Clamp Method to Limit Values
The lesson shows how to limit values in a certain range using the Mathf.Clamp method.
When developing game mechanics, sometimes you need to limit a certain value within a given range of values. For example, you write some simple space shooter and you want the player-controlled ship not to fly outside the borders of the screen. The Clamp method of the Mathf class will help us with this .
Consider using this method on a stage where we have a camera, a light source and a simple Cube .

We realize the possibility of moving the cube along the X axis , and it will be possible to move only in the range from X = -10 to X= 10. To do this, write the following script and add it to the cube:
JavaScript code:
function Update () {
var xMove : float = Input.GetAxis("Horizontal") * Time.deltaTime * 20;
transform.Translate(Vector3(xMove,0,0));
transform.position.x = Mathf.Clamp(transform.position.x, -10, 10);
}
C # Code:
void Update()
{
float xMove = Input.GetAxis("Horizontal") * Time.deltaTime * 20;
transform.Translate(new Vector3(xMove,0,0));
transform.position =
new Vector3(Mathf.Clamp(transform.position.x, -10, 10), transform.position.y, transform.position.z);
}
We move the cube using the value of the virtual X axis and the Transform.Translate method , and then limit the position of the cube along the X axis by calling the Mathf.Clamp method . The method accepts three parameters: a limited value, a minimum range boundary and a maximum range boundary.
We start the scene, press A / D on the keyboard or left-right arrows. The cube moves, and it cannot move beyond the borders of the range X = -10..10 (to be sure of this, you can add the position values along the X axis to the console at the end of the Update method - Debug.Log (transform.position.x); - comment of a translator) .
Link to the original lesson
Additional materials:
Link to the documentation of the Mathf.Clamp method
Base lesson 27 - Implementing a suspension (pause) in a game using Time.timeScale
The lesson discusses the use of the Time.timeScale property to pause / resume a game.
When developing a game, you will probably want to give players the opportunity to pause it and then resume it. To do this, just work a little with the static timeScale property of the Time class .
For example, we have a scene where a small ball jumps across the floor and we want to pause / resume its movement. First, we need an object on which we will “hang” a pause implementation script. To do this, you can create an empty game object ( GameObject menu - Create Empty ), place it in any convenient place and name it, for example, Manager .
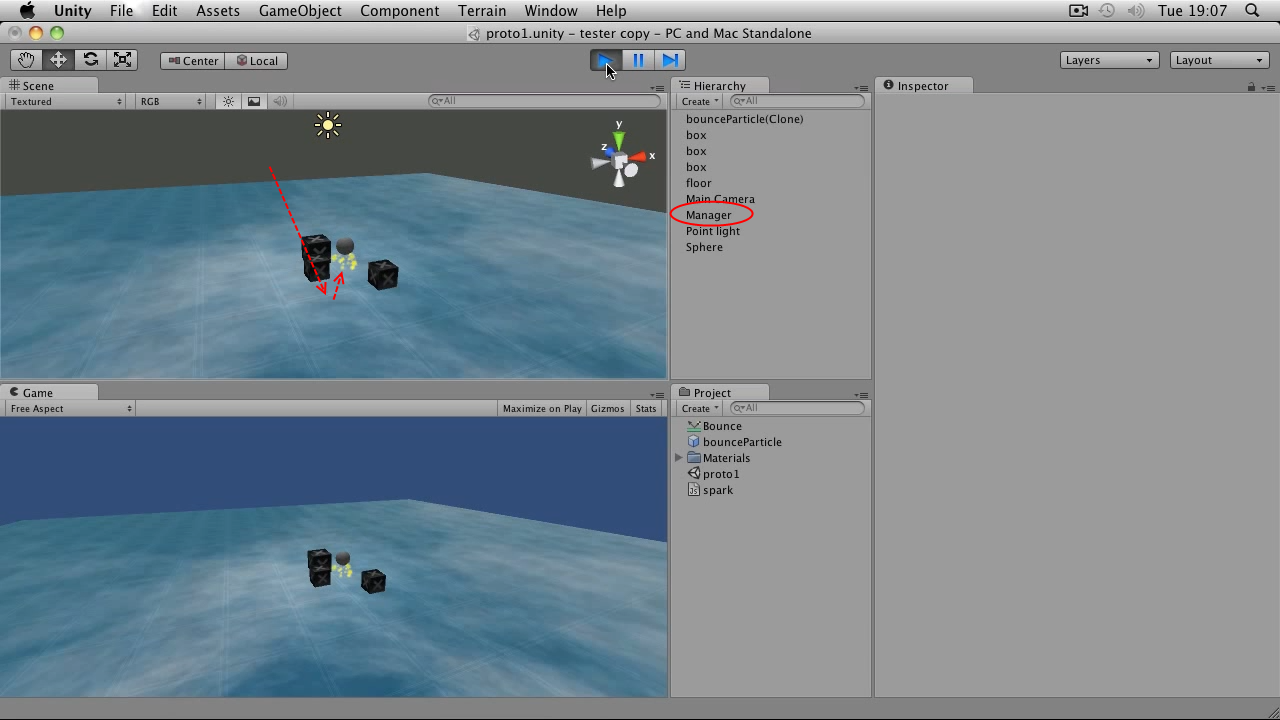
Now create a new script and write the following in it:
JavaScript Code:
var paused : boolean = false;
function Update () {
if(Input.GetButtonUp("Jump")){
if(!paused){
Time.timeScale = 0;
paused=true;
}else{
Time.timeScale = 1;
paused=false;
}
}
}
C # Code:
bool paused = false;
private void Update()
{
if(Input.GetButtonUp("Jump"))
{
if(!paused)
{
Time.timeScale = 0;
paused=true;
}
else
{
Time.timeScale = 1;
paused=false;
}
}
}
In the script we made variable paused for control, pause or not. We pause on / off in the Update method by pressing the “Jump” button - with standard input settings, this is the space bar. Next, we check if the pause is off? If so, set the Time.timeScale property to 0 , which will stop the flow of time in the game; and we mean that the pause is on - paused = true . Otherwise, set Time.timeScale to 1 and turn off the pause. The Time.timeScale property can also be set between 0 and 1 and greater than 1, which will, accordingly, slow down and speed up the passage of time if you need it.
Add a script to the Manager object , run the scene. At any time, click on the space bar - the ball stops. Repeated pressing returns the paused movement of the ball.
Link to the original lesson
Additional materials:
Link to the documentation for the Time.timeScale property
Base Lesson 28 - Using the SendMessage Method to Call External Methods
The lesson describes how to use the SendMessage method of the GameObject class to call the script method of another game object.
There are different ways to connect from the script of one game object to the script of another game object. Earlier, we examined the GetComponent method , with which you can get the components of an object, including its scripts. An alternative to this would be to use the SendMessage method .
Consider a scene with a blue Ball falling onto a red Switch box . Next to them hangs a black Block in the air . Let's make the block change the texture when the ball falls on the box and also falls down.
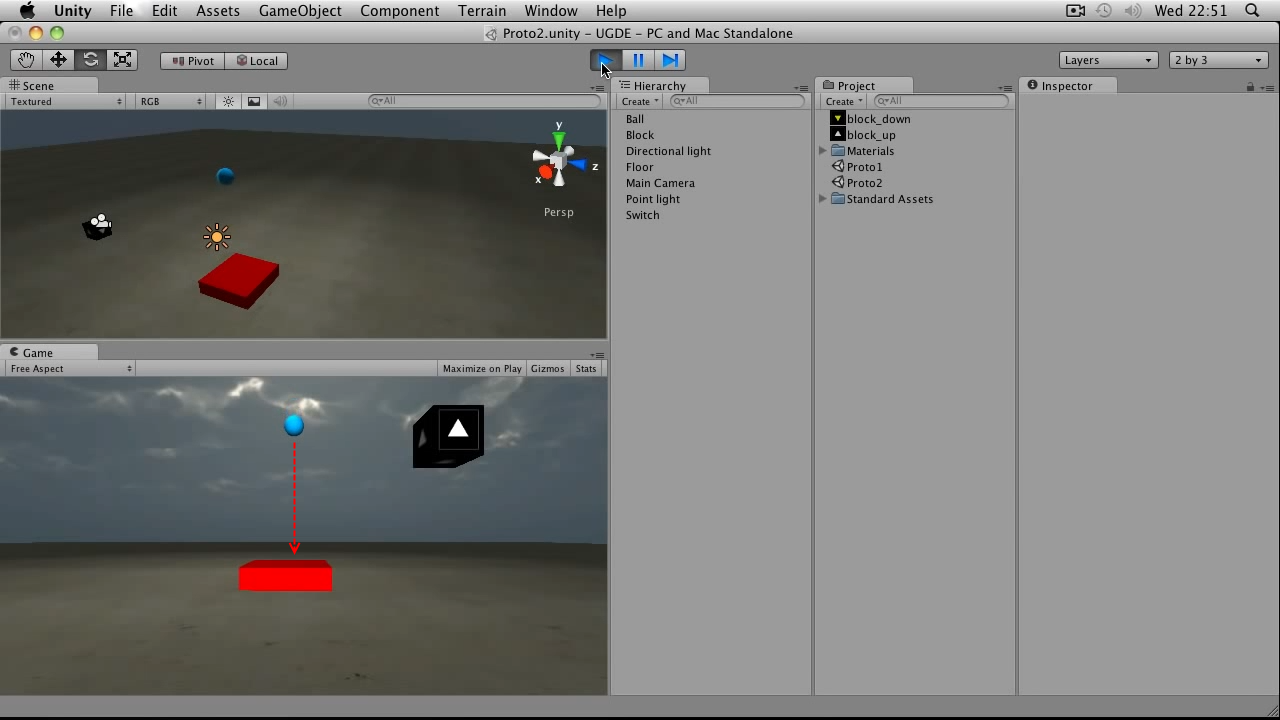
First we need to write a script for the block. Create a new script, call it Reactor and write the following:
JavaScript code:
var downTexture : Texture2D;
function React () {
renderer.material.mainTexture = downTexture;
yield WaitForSeconds(1);
gameObject.AddComponent(Rigidbody);
}
C # Code:
public Texture2D downTexture;
private IEnumerator React()
{
renderer.material.mainTexture = downTexture;
yield return new WaitForSeconds(1);
gameObject.AddComponent(typeof(Rigidbody));
}
We declared the variable downTexture for texture storage and implemented the React method , in which we change the texture of the object, make a short delay of 1 second and add the Rigidbody component of the solid body so that the object begins to be affected by gravity. We immediately add this script to the Block object and drag any suitable picture into the Down Texture script field . In the lesson, this is an image of a yellow arrow pointing down on a black background.

Take your time to run the scene, nothing will change on it for now. Next we need to make a script for the Ball . Call it Switcher and write it inside:
JavaScript code:
function OnCollisionEnter(col : Collision) {
if(col.gameObject.name == "Switch"){
gameObject.Find("Block").SendMessage("React");
}
}
C # Code:
private void OnCollisionEnter(Collision col)
{
if (col.gameObject.name == "Switch")
GameObject.Find("Block").SendMessage("React");
}
We implemented the OnCollisionEnter method , which will be called when the collider of the ball collides with the collider of another object. We are interested in a collision with the Switch box , so we first do the corresponding check. If so, we look for the Block object using the GameObject.Find method , specifying the name of the block. For the found object, the SendMessage method is called , in which the name of the method we want to call ( React ) is passed , after which, accordingly, this method executes.
Add a script to the ball, run the scene. Now, when a ball falls on a box, the block changes its texture and after a second also falls down.

Note from the translator:One of the downsides of using SendMessage is its potential performance degradation compared to calling methods directly. Here on this page dedicated to performance issues, we talk about the difference of 100 times in favor of a direct call. Therefore, this method should be used with caution (like the GetComponent , Find , etc. methods ), and if its use really becomes the culprit of a performance drop, use faster solutions.
Link to the original lesson
Additional materials:
Link to documentation of the GameObject.SendMessage method