Do I need to read the code of the libraries used?
What a wonderful time we live in! You are writing a program and you need a library for something - you will definitely find it! Many libraries are located in opensource and are even distributed under pleasant licenses like LGPL, I took it and solved the problem. Something: the connection method is described in readme, the library provides beautiful interfaces, there is a demo (it even compiles and works). In general, OOP with all its ideas of abstractions, interfaces, encapsulation of internal data is a powerful thing ( here without irony ).
But let's think about where and when “abstraction” begins. For example, I have a car in which I go to the supermarket once a week. In this case, for me it’s quite an abstraction of the level “steering wheel, three pedals and a pair of levers”, which provides the functionality I need. And I can use it for many years, without really thinking about what's under the hood - an engine or magic gnomes, for some reason drinking gasoline and spinning wheels. But if I work as a car mechanic, car designer, or simply as a professional driver, I can no longer afford to look at the car so “down”, to know its internal structure is my job. A milk buyer in a store may never see a living cow at all, but the farmer who grows this cow is simply obliged to know everything about her health \ maintenance \ nutrition \ care \ milking. Well, etc. There is a clear division into "users" of the product and its "creators". The former may allow themselves not to think about the details; the latter may not.

If you read up to this place, with a high degree of probability you are a programmer. Once again, you are a person who is professionally engaged in the creation of computer programs. Which, probably, is responsible for his work and, perhaps, considers himself a good specialist. And you consider it possible to take someone else's library and, having familiarized yourself with it at the level of its interface, use it in your product?
Let's imagine the interface of such an abstract library in an unidentified language:
Everything is clear, right? We call Init (), then we transfer something to Calculate (), we receive the answer, we call Release ().
Elementary? Do not hurry. Answer what happens if:
well, etc.
You say that these things should be described in the documentation? Yes, they should be. But, firstly, this is not always the case. And secondly, the documentation is a set of letters that are not parsed by the compiler, are not executed on runtime, are not automatically verified by anyone. There may be truth. Or maybe the result of knocking on a typewriter flock of monkeys. Or the weather on Mars. Developers do not like to write documentation and even less like to update it. Deal with it. Well, or read, finally, the library code.
So, you found in the library a function that does "seem to be what I need." Do not hurry. You do not know why and how its creator wrote it. No one guarantees that it will work on all the set of input data you need, on all the platforms you need, with the performance you need, without side effects. At the moment, all that you have is the name of the function and a few words from a person whom you yourself have never seen, with the promise that everything will be fine.
There is immediately a whole set of nuances. If the library contains tests, it says a lot, they can be read, run, it will significantly add understanding of how this library can / should be used. For example, I have often seen in the tests the lines “TODO: ...”, “possible bug”, commented pieces of tests marked “uncomment after implementing the functionality”, etc. Even the most elementary test may suddenly discover, for example, that the author expects a temperature in Kelvin at the input of the function, although you were 100% sure that it should be in Celsius.
If there are no tests, this also says a lot (first of all, that it would be better to look for another library). Perhaps you should write them yourself. Or perhaps the library is written in such a way that it is very difficult to write them - then there can be problems using the library.

There are exactly two options. With a high degree of probability the creator of the library was more qualified than you in the field of tasks solved by the library. Well, it would not have been otherwise he would have undertaken to write a library. This is quite an important reason for reading his code - perhaps you will learn something, maybe the code shows some "pitfalls" of the problem being solved, which you would be useful to know or even offer better solutions than you expected. In any case, reading the code of a good specialist is always useful.
The second (less common) case is when the creator of the library has a lower qualification than you. Firstly, it would be nice to understand this (and how to do it without reading the code?). Secondly, here is it like with a chain - the quality of your product will not be higher than the quality of its worst component, and here you consciously want to create a problem for yourself out of the blue?
You do not know how much the person who wrote the library wanted to make it quality. Perhaps he spent an extra hundred hours "polishing" the code, or maybe this library was his last product at his last place of work, written during the "last two weeks" before leaving the hated employer. Can you imagine how different the quality of the code can be in the first and second cases?

We all know the story with the recent NSA bookmark in a random number generator. Well, about the rumors (or not rumors) about $ 10 million for this case, too, in the know. People are very skeptical and suspicious in everyday life, but in terms of trust in software (and even if suddenly free!) They take on completely opposite positions.
The same is true for the relationship of a product with its libraries. If there is a bug in the library, it will be in your product. Memory leak in the library? No, now this is a memory leak in your product. Lock? Performance issues? Undefined behavior? Receive, sign.
Abstraction is not when you do not understand the internal structure of something, it is when you are so confident in it that you can afford not to think about it.
But let's think about where and when “abstraction” begins. For example, I have a car in which I go to the supermarket once a week. In this case, for me it’s quite an abstraction of the level “steering wheel, three pedals and a pair of levers”, which provides the functionality I need. And I can use it for many years, without really thinking about what's under the hood - an engine or magic gnomes, for some reason drinking gasoline and spinning wheels. But if I work as a car mechanic, car designer, or simply as a professional driver, I can no longer afford to look at the car so “down”, to know its internal structure is my job. A milk buyer in a store may never see a living cow at all, but the farmer who grows this cow is simply obliged to know everything about her health \ maintenance \ nutrition \ care \ milking. Well, etc. There is a clear division into "users" of the product and its "creators". The former may allow themselves not to think about the details; the latter may not.

If you read up to this place, with a high degree of probability you are a programmer. Once again, you are a person who is professionally engaged in the creation of computer programs. Which, probably, is responsible for his work and, perhaps, considers himself a good specialist. And you consider it possible to take someone else's library and, having familiarized yourself with it at the level of its interface, use it in your product?
Ambiguity of behavior
Let's imagine the interface of such an abstract library in an unidentified language:
interface IInterface
{
bool Init();
int Calculate(int val);
void Release();
}
Everything is clear, right? We call Init (), then we transfer something to Calculate (), we receive the answer, we call Release ().
Elementary? Do not hurry. Answer what happens if:
- Call Init () twice? And 1000 times?
- Call Calculate () without calling Init ()?
- Do not call Release ()? And if you call twice? And if 1000 times? But what if before calling Init ()?
- Call Init (), Calculate (), Release () from different threads?
- What is the range of the val argument in general? Whole int? What exactly? And 0 too? What about MIN_INT and MAX_INT?
- And then some exceptions are thrown?
- Are any logs written here? Does it somehow configure?
well, etc.
You say that these things should be described in the documentation? Yes, they should be. But, firstly, this is not always the case. And secondly, the documentation is a set of letters that are not parsed by the compiler, are not executed on runtime, are not automatically verified by anyone. There may be truth. Or maybe the result of knocking on a typewriter flock of monkeys. Or the weather on Mars. Developers do not like to write documentation and even less like to update it. Deal with it. Well, or read, finally, the library code.
Library purpose
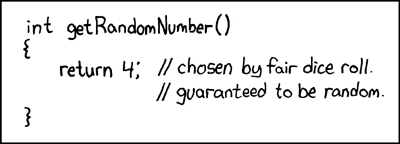
Tests
There is immediately a whole set of nuances. If the library contains tests, it says a lot, they can be read, run, it will significantly add understanding of how this library can / should be used. For example, I have often seen in the tests the lines “TODO: ...”, “possible bug”, commented pieces of tests marked “uncomment after implementing the functionality”, etc. Even the most elementary test may suddenly discover, for example, that the author expects a temperature in Kelvin at the input of the function, although you were 100% sure that it should be in Celsius.
If there are no tests, this also says a lot (first of all, that it would be better to look for another library). Perhaps you should write them yourself. Or perhaps the library is written in such a way that it is very difficult to write them - then there can be problems using the library.
Library Creator Qualification

There are exactly two options. With a high degree of probability the creator of the library was more qualified than you in the field of tasks solved by the library. Well, it would not have been otherwise he would have undertaken to write a library. This is quite an important reason for reading his code - perhaps you will learn something, maybe the code shows some "pitfalls" of the problem being solved, which you would be useful to know or even offer better solutions than you expected. In any case, reading the code of a good specialist is always useful.
The second (less common) case is when the creator of the library has a lower qualification than you. Firstly, it would be nice to understand this (and how to do it without reading the code?). Secondly, here is it like with a chain - the quality of your product will not be higher than the quality of its worst component, and here you consciously want to create a problem for yourself out of the blue?
Negligence
You do not know how much the person who wrote the library wanted to make it quality. Perhaps he spent an extra hundred hours "polishing" the code, or maybe this library was his last product at his last place of work, written during the "last two weeks" before leaving the hated employer. Can you imagine how different the quality of the code can be in the first and second cases?
Evil intent

We all know the story with the recent NSA bookmark in a random number generator. Well, about the rumors (or not rumors) about $ 10 million for this case, too, in the know. People are very skeptical and suspicious in everyday life, but in terms of trust in software (and even if suddenly free!) They take on completely opposite positions.
Tell me who your friend is and I will tell you who you are
The same is true for the relationship of a product with its libraries. If there is a bug in the library, it will be in your product. Memory leak in the library? No, now this is a memory leak in your product. Lock? Performance issues? Undefined behavior? Receive, sign.
Conclusion
Abstraction is not when you do not understand the internal structure of something, it is when you are so confident in it that you can afford not to think about it.