
The framework of your next web application
Each time there is a task of choosing a framework for writing a new project, we (web developers) most likely choose what we already know well and constantly use. The world of PHP frameworks is quite rich and the largest players are: CodeIgniter , Kohana , Symfony , Yii , Zend Framework . But I want to draw your attention to a fundamentally new, or rather a fundamentally new branch of the best framework with which I had to deal. Meet Laravel 4!
First, let's find out what the framework really is. According to Wikipedia, the framework is:
Laravel is a set of best practices from the world of PHP development. From the framework, the simplicity and professionalism that its developers have at the same time blows.
Simplicity begins during the installation phase. After all, it’s enough to drive just one line on the command line and wait until everything is installed:
And professionalism lies in the fact that the framework carries and preaches the use of such patterns as:
And Laravel gives you the ability to use them extremely efficiently. For example, to implement a DI of a class, it is enough to write:
Laravel will create an instance of the UserRepository class. And if the `UserRepository` also requires DI of some classes, Laravel will also instantiate them. (note how good and useful DI is and what other ways to implement it will not be discussed in this post).
It is also worth mentioning that Laravel is easily extensible, that is, if you use a queue server, and Laravel does not know how to work with it, then you just need to write a class (driver) to work with your queue server - inheriting from the Queue class and implementing the interface QueueInterface , and then register in the appropriate config that such and such a class should be used for queues.
One of the main advantages of Laravel is the brevity and readability of its code. For example, to queue a task it is enough to write:
or to trigger an event:
Concise, concise and elegant, isn't it?
It is also worth mentioning the ORM in Laravel (what kind of framework if it does not have ORM?), Which is called Eloquent (note that the translation means - eloquent, expressive). And he really lives up to his name. To select the first entry from the `users` table, for which we have the` User` model, we need to write:
or to search for users who are active and sort them by registration date, just write the following:
The code is very easy to read and enjoyable.
Suppose we have a `roles` table and a table that binds user roles and the roles themselves - user_roles. To add a connection in the `User` class, you just need to write 3 lines:
and if you want to select users along with their roles, the code will look like this:
And again the wow effect works :)
In order to get acquainted with all the features and delights of Eloquent ORM, I recommend that you look into the official documentation and discover a bunch of interesting features (http://laravel.com/docs/eloquent).
Laravel is incredibly convenient and cool for creating a RESTful API, because in the controller method it’s enough to write:
and Laravel will convert this model to JSON. Very comfortably. There are also other options for creating a RESTful API, which should be explored in the official documentation.
I will not fully state all the possibilities that Laravel gives the web developer, because it is impossible to take them all and paint them in one post. Every day, when I come across Laravel development, I discover new ways to write this or that functional.
Not a single framework has been documented as concisely and clearly as Laravel yet. Do you need to know how to work with queues? Please go to the Queues section and read how to work with them. Moreover, it turns out that there are several options for working with them - choose your favorite or suitable for your task and go! Want to learn how to expand the kernel? Please, and there are answers with examples.
Above, I wrote how easy it is to use the capabilities of the framework. And this is not only a wonderful ORM, convenient data output, but also incredibly beautiful and flexible routing, testability of everything and everything, the Blade template engine, Schema Builder, etc. Speaking of tests, you no longer have to worry about the fact that when you use Mock objects in your tests, the framework may work in an unexpected way or not how you planned. Laravel already contains tests for all its functionality. You do not need to test the framework. Test your code. The developers reacted with great responsibility to the tests and the operability of the framework.
You should no longer be surprised that magic happens somewhere and that something does not function as it should. Everything here works exactly as it is written in the documentation.
Taylor Otwell

The main developer of the framework. It is he who thinks about what the framework and its API should look like, and decides whether or not to introduce this or that functionality.
Phill Sparks

Dayle Rees
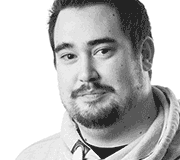
Shawn McCool
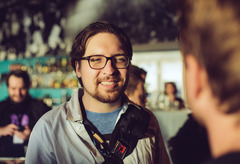
All these guys have full access to the repository and deal directly with the kernel.
In conclusion, I want to say that Laravel is gaining momentum very quickly. In trending on github, it has been in the first place for a long time, and among 2nd PHP frameworks in the 2nd. Communities are emerging, with which they are quite responsive. There is also a community in Russia. We are few, but we are in vests :) Enter , we will be very happy.
Laravel returns those feelings when you first sat down for programming and it starts to work out for you, those feelings that gave you pleasure from programming in PHP, and did not turn development into some kind of routine work.
What is a framework?
First, let's find out what the framework really is. According to Wikipedia, the framework is:
Software system structure; software that facilitates the development and integration of various components of a large software project. The word "wireframe" is also used, and some authors use it as the main one, including without basing it on the English-language analogue at all.
The framework differs from the concept of a library in that the library can be used in a software product simply as a set of routines of close functionality, without affecting the architecture of the software product and without imposing any restrictions on it. While the framework dictates the rules for building the application architecture, setting the default behavior at the initial stage of development, the framework that will need to be expanded and modified according to the specified requirements.
What is Laravel
Laravel is a set of best practices from the world of PHP development. From the framework, the simplicity and professionalism that its developers have at the same time blows.
Simplicity begins during the installation phase. After all, it’s enough to drive just one line on the command line and wait until everything is installed:
composer create-project laravel/laravel project_name --prefer-dist
And professionalism lies in the fact that the framework carries and preaches the use of such patterns as:
- DI (Dependency Injection)
- Repository
- Observable
- Singleton
- Facade
- Factory
- Iterator
- etc ...
And Laravel gives you the ability to use them extremely efficiently. For example, to implement a DI of a class, it is enough to write:
class UserController extends Controller {
private $users;
public function __construct(UserRepository $users) {
$this->users = $users;
}
}
Laravel will create an instance of the UserRepository class. And if the `UserRepository` also requires DI of some classes, Laravel will also instantiate them. (note how good and useful DI is and what other ways to implement it will not be discussed in this post).
It is also worth mentioning that Laravel is easily extensible, that is, if you use a queue server, and Laravel does not know how to work with it, then you just need to write a class (driver) to work with your queue server - inheriting from the Queue class and implementing the interface QueueInterface , and then register in the appropriate config that such and such a class should be used for queues.
One of the main advantages of Laravel is the brevity and readability of its code. For example, to queue a task it is enough to write:
Queue::push('SendEmail@send', array('message' => $message));
or to trigger an event:
Event::fire('user.login', array($user));
Concise, concise and elegant, isn't it?
It is also worth mentioning the ORM in Laravel (what kind of framework if it does not have ORM?), Which is called Eloquent (note that the translation means - eloquent, expressive). And he really lives up to his name. To select the first entry from the `users` table, for which we have the` User` model, we need to write:
User::first();
or to search for users who are active and sort them by registration date, just write the following:
$users = User::where('is_active', 1)->orderBy('created_at', 'DESC')->get();
The code is very easy to read and enjoyable.
Suppose we have a `roles` table and a table that binds user roles and the roles themselves - user_roles. To add a connection in the `User` class, you just need to write 3 lines:
public function roles() {
return $this->belongsToMany(Role::class, 'user_roles');
}
and if you want to select users along with their roles, the code will look like this:
$users = User::with('roles')->get();
And again the wow effect works :)
In order to get acquainted with all the features and delights of Eloquent ORM, I recommend that you look into the official documentation and discover a bunch of interesting features (http://laravel.com/docs/eloquent).
Laravel is incredibly convenient and cool for creating a RESTful API, because in the controller method it’s enough to write:
$user = User::find(1);
return $user;
and Laravel will convert this model to JSON. Very comfortably. There are also other options for creating a RESTful API, which should be explored in the official documentation.
I will not fully state all the possibilities that Laravel gives the web developer, because it is impossible to take them all and paint them in one post. Every day, when I come across Laravel development, I discover new ways to write this or that functional.
Documentation
Not a single framework has been documented as concisely and clearly as Laravel yet. Do you need to know how to work with queues? Please go to the Queues section and read how to work with them. Moreover, it turns out that there are several options for working with them - choose your favorite or suitable for your task and go! Want to learn how to expand the kernel? Please, and there are answers with examples.
Everything has already been taken care of
Above, I wrote how easy it is to use the capabilities of the framework. And this is not only a wonderful ORM, convenient data output, but also incredibly beautiful and flexible routing, testability of everything and everything, the Blade template engine, Schema Builder, etc. Speaking of tests, you no longer have to worry about the fact that when you use Mock objects in your tests, the framework may work in an unexpected way or not how you planned. Laravel already contains tests for all its functionality. You do not need to test the framework. Test your code. The developers reacted with great responsibility to the tests and the operability of the framework.
You should no longer be surprised that magic happens somewhere and that something does not function as it should. Everything here works exactly as it is written in the documentation.
Who is behind the curtains?
Taylor Otwell

The main developer of the framework. It is he who thinks about what the framework and its API should look like, and decides whether or not to introduce this or that functionality.
Phill Sparks

Dayle Rees
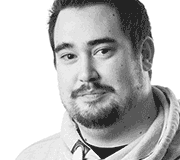
Shawn McCool
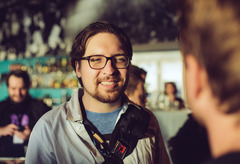
All these guys have full access to the repository and deal directly with the kernel.
?>
In conclusion, I want to say that Laravel is gaining momentum very quickly. In trending on github, it has been in the first place for a long time, and among 2nd PHP frameworks in the 2nd. Communities are emerging, with which they are quite responsive. There is also a community in Russia. We are few, but we are in vests :) Enter , we will be very happy.
Laravel returns those feelings when you first sat down for programming and it starts to work out for you, those feelings that gave you pleasure from programming in PHP, and did not turn development into some kind of routine work.