Google Maps API: Directions, Animation, and Styling
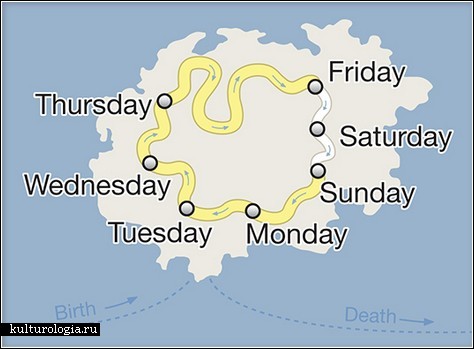
Many of us often insert maps into our sites. Usually, this is a map on the contact page with the only marker that marks the office location. But sometimes at the customer’s imagination turns on and “creativity” begins. At one time, I spent a lot of time to understand the ever-changing Google Maps API and I want to share with you my best practices for solving common problems with maps that go beyond a single marker.
Everything described below can be found out carefully by reading the GMaps API documentation. It is assumed that the reader is already able to implement the map, connect the API and set a marker. The article does not claim to be complete, but may be useful to novice users of the Google Maps API.
Driving directions
Quite often, on the map it is necessary to mark not only a place, but also a way to get to it. There are several ways to do this. For all of them, polylines are used.
Option 1: Drive by Car
This option involves laying the route by Google itself. To do this, specify where to go, where and on what.

directionsDisplay = new google.maps.DirectionsRenderer();
var request = {
origin: new google.maps.LatLng(60.023539414725356,30.283663272857666), //точка старта
destination: new google.maps.LatLng(59.79530896374892,30.410317182540894), //точка финиша
travelMode: google.maps.DirectionsTravelMode.DRIVING //режим прокладки маршрута
};
directionsService.route(request, function(response, status){
if (status == google.maps.DirectionsStatus.OK) {
directionsDisplay.setDirections(response);
}
});
directionsDisplay.setMap(map);
Google is laying the route in the most convenient way, in his opinion. An important point: in large (and not so) cities there is a big difference between the directions of movement. Both for convenience and due to traffic restrictions.
Google has four routing modes:
• google.maps.TravelMode.DRIVING - Car
• google.maps.TravelMode.BICYCLING - Bicycle
• google.maps.TravelMode.TRANSIT - Public transport (does not work everywhere)
• google.maps.TravelMode .WALKING - Pedestrian
And if far?
Option 2: fly by plane
The API does not have the ability to calculate sea and air routes. Apparently because of the lack of demand. But sometimes you still need to display some sort of air route on the map. For example, for a perishable goods supply card. The initial data are the same, the point of departure and the point of arrival. To create this route you just need to draw a polyline on the map:
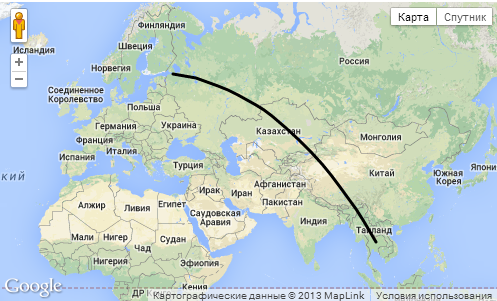
route[route.length] = new google.maps.Polyline({
path: [new google.maps.LatLng(16.003575733881323, 101.689453125), new google.maps.LatLng(59.934288, 30.3350336)], //указываем точки старта и финиша
geodesic: true, //устанавливаем флаг геодезической кривой. Так и выглядит лучше и точнее передает кратчайший путь между двумя точкамиmap: map//устанавливаем на карту
});
Map animation
If we have a line on the map, for example, an air supply route, then it would be nice to screw an animation there that shows where this plane is flying from.
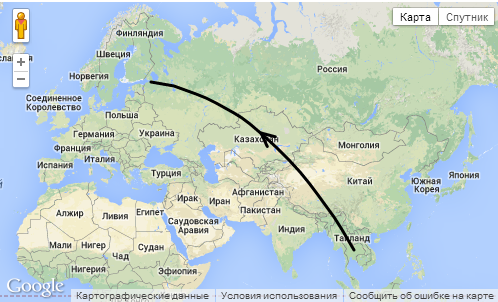
functionanimate(route) { //функция анимирует каждый первый символ каждой полилинииvar count = 0;
icons = null;
var lnght=route.length;
offsetId = window.setInterval(function() {
count = (count + 1) % 200;
for(var i= 0; i < lnght; i++){
icons = route[i].get('icons');
icons[0].offset = (count / 2) + '%';
route[i].set('icons', icons);
}
}, 40);
}
functioninitialize() {
... //настройка карты
route[route.length] = new google.maps.Polyline({
path: [new google.maps.LatLng(16.003575733881323, 101.689453125), new google.maps.LatLng(59.934288, 30.3350336)],
geodesic: true,
icons: [{
icon: {path: google.maps.SymbolPath.FORWARD_OPEN_ARROW},
offset: '100%'}],
map: map
});
animateCircle(route);
}
How to paint over the country?
Suppose that for some purpose you need to paint over a country on the map. For example, to show the countries in which you have been. For these purposes, it is convenient to use KML format files. The files with the contours of the countries themselves can be taken here or here . Edit (color, etc. can be in the program Google Earth).
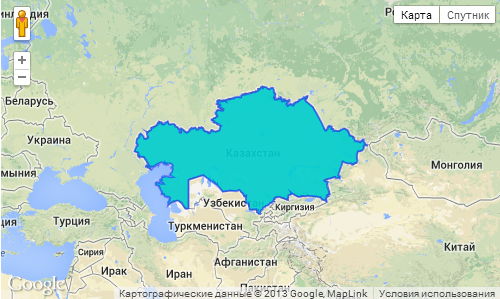
var KmlLayer = new google.maps.KmlLayer({
url: 'http://yoursite.com/kml/Kazakhstan.kmz',
map: map
});
The code here is very simple, it makes no sense to describe it. The only thing worth noting is that the path to the file must be complete, since Google servers are involved in its processing.
Colorize the map
Sometimes it is necessary to stylize a map for the appearance of the site. Although Google’s specialists made a map that suits most, there’s a way to change everything. For example, so that the selected country is better visible. For these purposes, it is most convenient to use the card styling wizard , which provides Google for these purposes. The wizard outputs the code in JSON, which can be immediately put into the script.

var styles = [
{
"featureType": "landscape",
"stylers": [
{ "color": "#ffffff" }
]
},{
"featureType": "road",
"stylers": [
{ "lightness": 80 }
]
},{
"featureType": "administrative.locality",
"stylers": [
{ "visibility": "off" }
]
},{
"elementType": "geometry.stroke",
"stylers": [
{ "color": "#000000" },
{ "weight": 0.5 },
{ "lightness": 11 }
]
},{
"featureType": "poi",
"stylers": [
{ "visibility": "off" }
]
}
];
map.setOptions({styles: styles});
useful links
Service for creating KML . It also makes it easy to get an array of points to create a polyline or just determine the coordinates.
Another generator of polylines, with the ability to cancel.