
Accepting bank card payments in applications - PayOnline Payment SDK
- Tutorial
At the end of the summer, PayOnline together with Microsoft announced the release of a new product - the PayOnline Payment SDK, which allows mobile application developers to integrate non-cash payments into the Windows Store and Windows Phone applications. On September 12, we made a presentation at the Windows Camp with the Payment SDK, met with application developers face-to-face, and talked about the most interesting aspects of implementing payment acceptance in applications. A lot has been written about what Internet acquiring is on the Internet, and, in particular, on Habré ( 1 , 2 ), so I don’t feel like repeating ourselves, let's get down to business immediately.
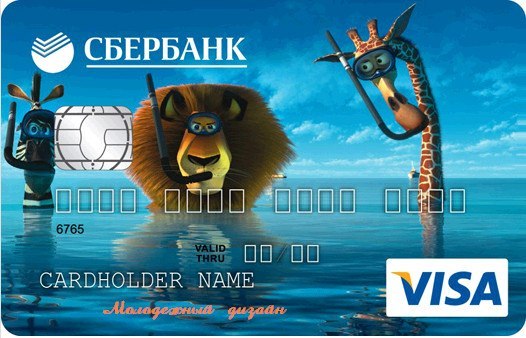
Like any “adult” IPSP (Internet Payment Service Provider), we have our own API that allows us to conduct payment transactions. We pass on the interface to the developers, and from the received “constructor” the application developer on his side builds that part of the payment service that will be responsible for the interaction of the payer and the acquiring bank.
Everything is wonderful and even as long as the development concerns web-sites. Internet acquiring is the reception of money on the Internet, and, accordingly, anti-fraud technologies have been developed taking into account this specificity.
The simplest form of payment acceptance integration in mobile applications is the built-in browser. You need to build in a browser to display the payment page of the acquiring bank or payment gateway - and a page for entering the 3-D Secure verification confirmation code. But what if you do not want to violate the integrity of the design and interface of the application?
In this case, the API that we wrote about above comes to the rescue. But when using the API, a considerable amount of work on application integration with the payment gateway rests with the application developer, especially with regard to the implementation of the 3-D Secure protocol (see sidebar).
And here we come to the most interesting thing - the description of the SDK, which allows you to quickly and painlessly, and "without much hemorrhoids" integrate the acceptance of card payments into the application.
Consider the sample code for the Windows Store application. This is enough to ensure acceptance of payments.
What's going on here.
To make a payment, you must use the Processing object, which accepts an object that must implement the IConfiguration interface.
The implementation is very simple, two fields: your ID and the secret key that is issued upon connection.
The Processing object provides four events:
In the latter case, it is necessary to show the user the page of the issuing bank to enter the code or password, and process the response. You can do everything manually, or you can use the NavigateToAcsUrl method, which takes as a parameter a user control - a browser (it has its own for each platform) and a PayResponse object.
Finally, to call the Pay method, you need to pass it a PayRequest containing the following fields:
A savvy reader, of course, will notice that in our SDK we operate with card numbers and ask how is PCI DSS? Yes, indeed, applications that enter bank card data during payment formally fall under the PCI DSS standard, or rather PCI PA-DSS.
What is PCI DSS we wrote in detail in our post . PCI PA-DSS is a related PCI DSS Payment Card Industry Payment Application Security Standard.
As it is easy to understand from the scope of the standard, if bank card data is entered in the application, it falls within the scope of the standard. And it would seem that owners of applications using both the API and the SDK should think about obtaining a certificate.
However, it is worth noting that, firstly, for mobile applications there are simply no clear requirements (as, for example, for online stores), and secondly, for small businesses, certification is strictly necessary only formally. For example, all online stores that provide customers with the opportunity to pay by credit card must have a PCI DSS Level 4 certificate, even if the payment itself is made on the secure payment page of the service provider. However, it is clear to everyone that an online store with a card turnover of 20,000 rubles a month will not be required to undergo annual certification - the same situation is with PA-DSS certification for mobile applications.
The requirements of the standard apply to applications that enter bank card data. If the application does not enter payment data, but a certain identifier, the application stops processing card data and certification is no longer needed.
In early 2013, we launched the payID project, which allows you to accept payments without entering payment information. The owner of the bank card creates an account in payID, binds a bank card to it, and when making payments on sites accepting payments via PayOnline, he does not enter the full card details, but his payID and CVC \ CVV code. This allows you to remove the responsibility for processing bank card data from the online store or application, and to the payer - to ensure 100% security of your card data and, accordingly, the money in your account.
If you embed payID payments into the application, you can accept card payments without built-in payment forms, passing PA-DSS certification, fear and reproach.
As we wrote above, we launched the SDK for Windows at the end of this summer, the product is still fresh and is under active development. In general, the sphere of accepting card payments in mobile applications is very young in itself. To the extent that security standards are still being formed in it, it is by no means controlled as tightly as standard e-commerce.
But the growth dynamics of the popularity of the mobile Internet in Russia, the massive shift from phones to smartphones, an increase in the number of Russian-language applications - all this speaks of the promise of working in the mobile segment of electronic commerce, its potential and monetization capabilities.
Now we are thinking about how to seamlessly turn the card holder into the owner of the identifier, if it is already in your application.
There are many topics to think about, no less work - we will be grateful to the harazhiteli for constructive criticism, interesting ideas and suggestions for the development of PayOnline Payment SDK.
You can download it from nuget: https://www.nuget.org/packages/PayOnline_PaymentSDK/
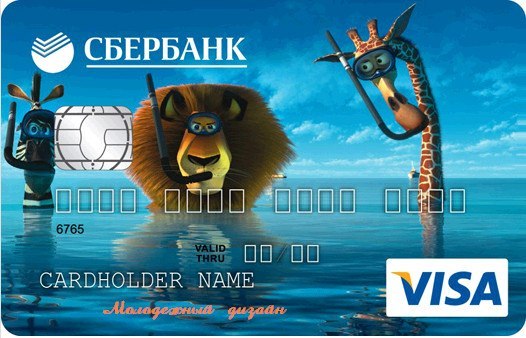
Like any “adult” IPSP (Internet Payment Service Provider), we have our own API that allows us to conduct payment transactions. We pass on the interface to the developers, and from the received “constructor” the application developer on his side builds that part of the payment service that will be responsible for the interaction of the payer and the acquiring bank.
Everything is wonderful and even as long as the development concerns web-sites. Internet acquiring is the reception of money on the Internet, and, accordingly, anti-fraud technologies have been developed taking into account this specificity.
One of the methods of protection is the 3-D Secure protocol, proposed by the VISA world payment system and adopted by the standard by other payment systems.
What is 3-D Secure is known to almost everyone who made purchases on the Internet (now in Russia a very small proportion of banks issuing cards do not support this protocol, and their number is decreasing due to the requirements of the same MPS).
Most often, the 3-D Secure protocol in work looks like this: you make a payment on the website of the online store, enter the card details, then go to the page of the issuing bank, where you enter the code received in SMS. After that, your payment is completed successfully.
The name 3-D comes from 3-Domain (three domains), since organizations on three domains participate in the payment verification under this protocol: the issuer’s domain (payer and issuing bank), the acquirer’s domain (payment processing bank, and online store) ) and the interaction domain (MPS). This is how a simplified payment verification scheme using the 3-D Secure protocol looks like:

The payer enters the card data in the online store, they reach the acquirer bank (1), are sent to the MPS (2), from where they are sent to the issuing bank (3). You can find out which bank issued the card by the first six digits of the card number. The issuing bank reports that the card is subscribed to 3-D Secure, generates a unique code, as well as a link to the code entry page (4). The link is returned to the TSP or IPSP (5), which redirect the card holder to this page in the browser (6,7). At this moment, the issuer sends to the cardholder through another channel (for example, via SMS) a temporary PIN code, which he enters on the page. If the code is entered correctly, the issuing bank reports on the successful completion of the check (8), and the funds are debited from the payer's card (9, 10).
Most often, the 3-D Secure protocol in work looks like this: you make a payment on the website of the online store, enter the card details, then go to the page of the issuing bank, where you enter the code received in SMS. After that, your payment is completed successfully.
The name 3-D comes from 3-Domain (three domains), since organizations on three domains participate in the payment verification under this protocol: the issuer’s domain (payer and issuing bank), the acquirer’s domain (payment processing bank, and online store) ) and the interaction domain (MPS). This is how a simplified payment verification scheme using the 3-D Secure protocol looks like:

The payer enters the card data in the online store, they reach the acquirer bank (1), are sent to the MPS (2), from where they are sent to the issuing bank (3). You can find out which bank issued the card by the first six digits of the card number. The issuing bank reports that the card is subscribed to 3-D Secure, generates a unique code, as well as a link to the code entry page (4). The link is returned to the TSP or IPSP (5), which redirect the card holder to this page in the browser (6,7). At this moment, the issuer sends to the cardholder through another channel (for example, via SMS) a temporary PIN code, which he enters on the page. If the code is entered correctly, the issuing bank reports on the successful completion of the check (8), and the funds are debited from the payer's card (9, 10).
The simplest form of payment acceptance integration in mobile applications is the built-in browser. You need to build in a browser to display the payment page of the acquiring bank or payment gateway - and a page for entering the 3-D Secure verification confirmation code. But what if you do not want to violate the integrity of the design and interface of the application?
In this case, the API that we wrote about above comes to the rescue. But when using the API, a considerable amount of work on application integration with the payment gateway rests with the application developer, especially with regard to the implementation of the 3-D Secure protocol (see sidebar).
And here we come to the most interesting thing - the description of the SDK, which allows you to quickly and painlessly, and "without much hemorrhoids" integrate the acceptance of card payments into the application.
Code example
Consider the sample code for the Windows Store application. This is enough to ensure acceptance of payments.
private void Pay()
{
var conf = new Configuration
{
MerchantId = 1,
Key = "PrivateKey",
};
var request = new PayRequest
{
Amount = 30m,
Currency = Currency.Rub,
OrderId = "335636462808",
CardExpMonth = 1,
CardExpYear = 2018,
CardCvv = 100,
CardHolderName = "CARD HOLDER",
CardNumber = "4111111111111111",
Email = "cardholder@example.com",
};
var po = new Processing(conf);
po.ThreeDs += po_ThreeDs;
po.Success += po_Success;
po.Decline += po_Decline;
po.Error += po_Error;
po.Pay(request);
}
void po_Error(object sender, Exceptions.PaymentSDKException e)
{
}
void po_Decline(object sender, PayResponse e)
{
}
void po_Success(object sender, PayResponse e)
{
}
void po_ThreeDs(object sender, PayResponse e)
{
((Processing)sender).NavigateToAcsUrl(Browser, e);
}
class Configuration : IConfiguration
{
public int MerchantId { get; set; }
public string Key { get; set; }
}
What's going on here.
To make a payment, you must use the Processing object, which accepts an object that must implement the IConfiguration interface.
The implementation is very simple, two fields: your ID and the secret key that is issued upon connection.
class Configuration : IConfiguration
{
public int MerchantId { get; set; }
public string Key { get; set; }
}
The Processing object provides four events:
- Success - arises in case of successful payment.
- Decline - arises in case of refusal to conduct.
- Error - if any errors occurred at the time of payment, for example, the network is unavailable.
- ThreeDs - An additional 3-D Secure check is required.
In the latter case, it is necessary to show the user the page of the issuing bank to enter the code or password, and process the response. You can do everything manually, or you can use the NavigateToAcsUrl method, which takes as a parameter a user control - a browser (it has its own for each platform) and a PayResponse object.
Finally, to call the Pay method, you need to pass it a PayRequest containing the following fields:
- Amount - payment amount.
- Currency - currency (rubles, US dollars and euros are supported).
- OrderId - ID of the order that you generate in your system.
- CardExpMonth - card expiration month.
- CardExpYear - card expiration year.
- CardCvv - CVC2 / CVV2.
- CardHolderName is the name of the card holder.
- CardNumber - card number.
- Email - payer's e-mail.
About PCI PA-DSS
A savvy reader, of course, will notice that in our SDK we operate with card numbers and ask how is PCI DSS? Yes, indeed, applications that enter bank card data during payment formally fall under the PCI DSS standard, or rather PCI PA-DSS.
What is PCI DSS we wrote in detail in our post . PCI PA-DSS is a related PCI DSS Payment Card Industry Payment Application Security Standard.
- Scope: an application that stores, processes or transfers data about cardholders.
- Applicability criterion: storage, processing or transfer of at least one card number (PAN).
- Conformity criterion: 100% compliance.
- Confirmation method: audit of the application by the audit organization.
- Confirmation frequency: annually.
As it is easy to understand from the scope of the standard, if bank card data is entered in the application, it falls within the scope of the standard. And it would seem that owners of applications using both the API and the SDK should think about obtaining a certificate.
However, it is worth noting that, firstly, for mobile applications there are simply no clear requirements (as, for example, for online stores), and secondly, for small businesses, certification is strictly necessary only formally. For example, all online stores that provide customers with the opportunity to pay by credit card must have a PCI DSS Level 4 certificate, even if the payment itself is made on the secure payment page of the service provider. However, it is clear to everyone that an online store with a card turnover of 20,000 rubles a month will not be required to undergo annual certification - the same situation is with PA-DSS certification for mobile applications.
About the possibility of replacing payment data with payID
The requirements of the standard apply to applications that enter bank card data. If the application does not enter payment data, but a certain identifier, the application stops processing card data and certification is no longer needed.
In early 2013, we launched the payID project, which allows you to accept payments without entering payment information. The owner of the bank card creates an account in payID, binds a bank card to it, and when making payments on sites accepting payments via PayOnline, he does not enter the full card details, but his payID and CVC \ CVV code. This allows you to remove the responsibility for processing bank card data from the online store or application, and to the payer - to ensure 100% security of your card data and, accordingly, the money in your account.
If you embed payID payments into the application, you can accept card payments without built-in payment forms, passing PA-DSS certification, fear and reproach.
Pro Future Payment SDK
As we wrote above, we launched the SDK for Windows at the end of this summer, the product is still fresh and is under active development. In general, the sphere of accepting card payments in mobile applications is very young in itself. To the extent that security standards are still being formed in it, it is by no means controlled as tightly as standard e-commerce.
But the growth dynamics of the popularity of the mobile Internet in Russia, the massive shift from phones to smartphones, an increase in the number of Russian-language applications - all this speaks of the promise of working in the mobile segment of electronic commerce, its potential and monetization capabilities.
Therefore, we will continue to develop the Payment SDK, and plan to move in several directions:
- Extension of opportunities for developers: a list of platforms (Android, iOS) and a list of supported programming languages - everything here is clear and without comment.
- Transfer from card to card: we have an implemented service of transfer from card to card, we already wrote about it on Habré. We are thinking about implementing the possibility of integrating this service into applications that work according to the Donation scheme. The application owner will be able to avoid the need to register an IP, create a business card website and conclude an agreement with a payment gateway. He only needs a bank card to receive transfers.
- Convenient payment acceptance via payID in applications: why using payID is convenient and profitable is clear from the part about the PCI PCI-DSS post. This avoids certification costs, relieves responsibility for card data and attracts new customers.
Now we are thinking about how to seamlessly turn the card holder into the owner of the identifier, if it is already in your application.
There are many topics to think about, no less work - we will be grateful to the harazhiteli for constructive criticism, interesting ideas and suggestions for the development of PayOnline Payment SDK.
You can download it from nuget: https://www.nuget.org/packages/PayOnline_PaymentSDK/