
Quick Help for your code in Xcode 5
- Tutorial
Quick Help learned to take documentation from comments:
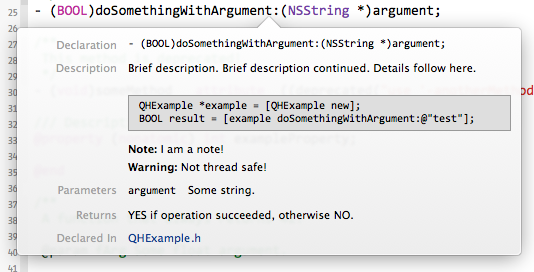
Previously, you had to install appledoc (or an analogue), compile and install it
There is no official documentation on the supported syntax yet (at least I have not found), but something is used between HeaderDoc and Doxygen .
The first paragraph of the comment is used in autocompletion, in Quick Help everyone is visible:

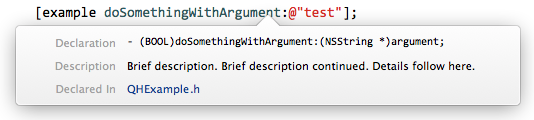
Of course, supported by the standard tags
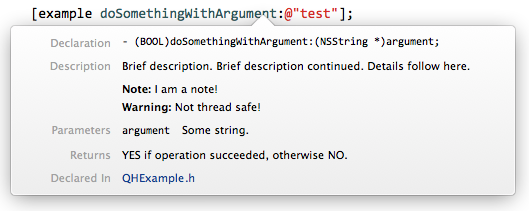
You can document not only individual methods, but the whole class as a whole:
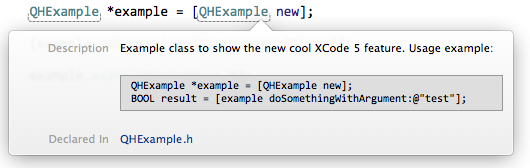
Documenting properties:
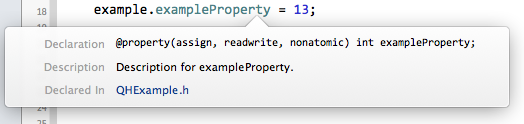
Deprecated methods are automatically determined:
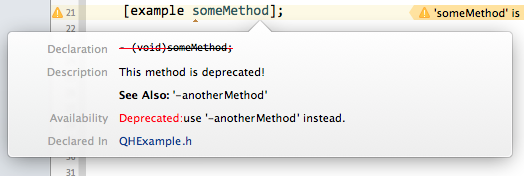
Functions are also supported:
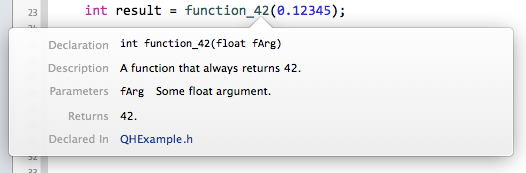
But macros, unfortunately, are not.
There is support for
But there is no support for recommended
Those. if you write:
then for constants descriptions in Quick Help and in autocompletion will be available, but for the type itself
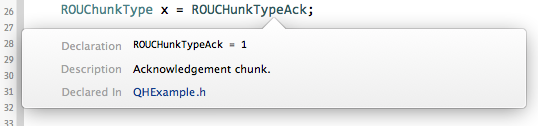
For structure types, the situation is better: for the type itself, there is no description in the auto-completion, but in Quick Help there is, for fields there is both.
But the documentation for block types works well:
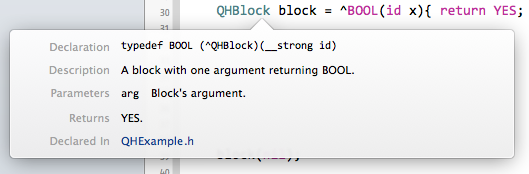
In addition, comments are supported not only in the interface, but also in the implementation, including for variables:

In total, it became one more reason to write documentary comments. Moreover, this is not at all laborious if you use code snippets . For example, to document a method, you can create a snippet:
and hang it on a shortcut
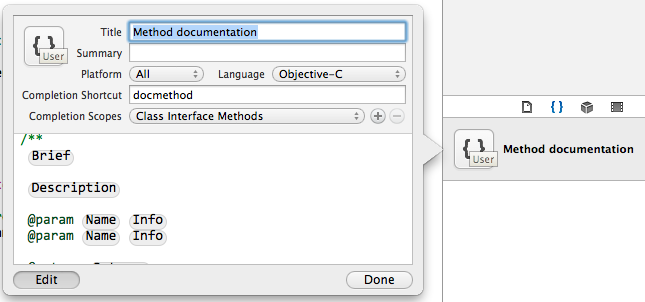
Then, when typing,
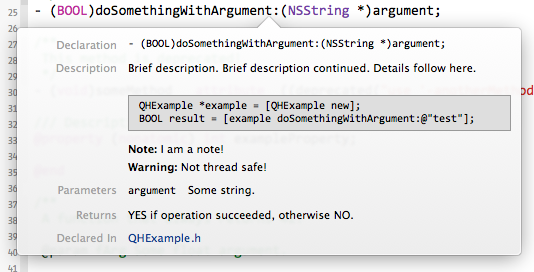
Previously, you had to install appledoc (or an analogue), compile and install it
.docset
; Now just write a documentary comment and Quick Help will immediately see it. There is no official documentation on the supported syntax yet (at least I have not found), but something is used between HeaderDoc and Doxygen .
1)
The first paragraph of the comment is used in autocompletion, in Quick Help everyone is visible:
/**
Brief description.
Brief description continued.
Details follow here.
*/
- (BOOL)doSomethingWithArgument:(NSString *)argument;

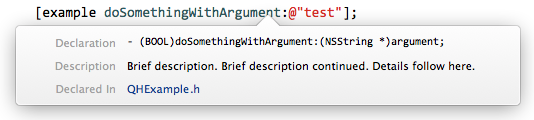
2)
Of course, supported by the standard tags
@param
, @return
as well as some others, such as @note
, @warning
, @see
, @code
:/**
Brief description.
Brief description continued.
Details follow here.
@note I am a note!
@warning Not thread safe!
@param argument Some string.
@return YES if operation succeeded, otherwise NO.
*/
- (BOOL)doSomethingWithArgument:(NSString *)argument;
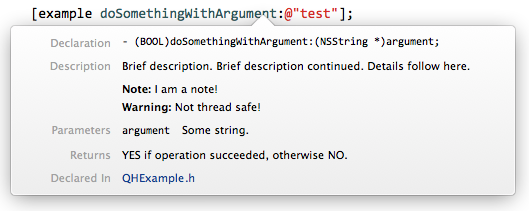
3)
You can document not only individual methods, but the whole class as a whole:
/**
Example class to show the new cool XCode 5 feature.
Usage example:
@code
QHExample *example = [QHExample new];
BOOL result = [example doSomethingWithArgument:@"test"];
@endcode
*/
@interface QHExample : NSObject
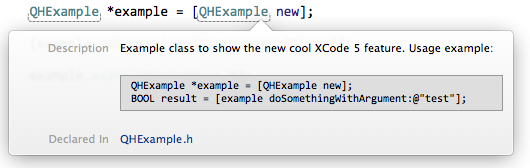
4)
Documenting properties:
/// Description for exampleProperty.
@property (nonatomic) int exampleProperty;
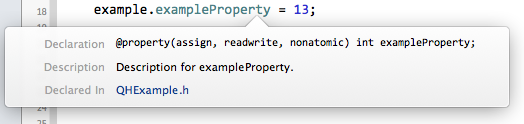
5)
Deprecated methods are automatically determined:
/**
This method is deprecated!
@see '-anotherMethod'
*/
- (void)someMethod __attribute__((deprecated("use '-anotherMethod' instead.")));
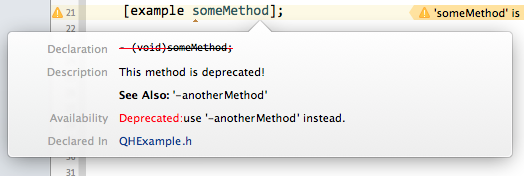
6)
Functions are also supported:
/**
A function that always returns 42.
@param fArg Some float argument.
@return 42.
*/
int function_42(float fArg);
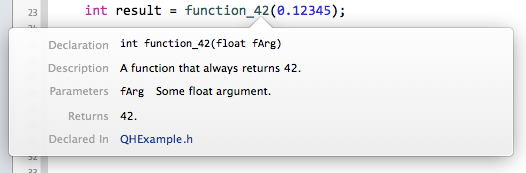
But macros, unfortunately, are not.
7)
There is support for
enum
:/**
ROUChunkType description.
*/
enum ROUChunkType {
/// Data chunk.
ROUChunkTypeData = 0,
/// Acknowledgement chunk.
ROUCHunkTypeAck = 1
};
But there is no support for recommended
NS_ENUM
and NS_OPTIONS
. Those. if you write:
/**
ROUChunkType description.
*/
typedef NS_ENUM(uint8_t, ROUChunkType){
/// Data chunk.
ROUChunkTypeData = 0,
/// Acknowledgement chunk.
ROUCHunkTypeAck = 1
};
then for constants descriptions in Quick Help and in autocompletion will be available, but for the type itself
ROUChunkType
- not.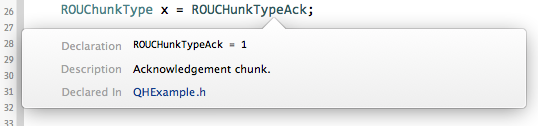
8)
For structure types, the situation is better: for the type itself, there is no description in the auto-completion, but in Quick Help there is, for fields there is both.
/**
Chunk header description.
*/
typedef struct {
/// Chunk type.
enum ROUChunkType type;
/// Chunk length.
uint16_t length;
} ROUChunkHeader;
9)
But the documentation for block types works well:
/**
A block with one argument returning BOOL.
@param arg Block's argument.
@return YES.
*/
typedef BOOL (^QHBlock)(id arg);
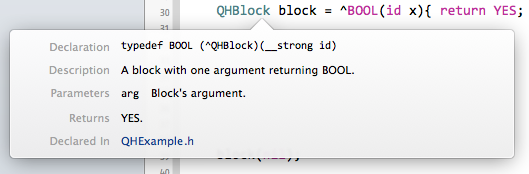
10)
In addition, comments are supported not only in the interface, but also in the implementation, including for variables:
- (double)perimeter{
/// The number π, the ratio of a circle's circumference to its diameter.
double pi = M_PI;
return 2 * pi * self.r;
}

Conclusion
In total, it became one more reason to write documentary comments. Moreover, this is not at all laborious if you use code snippets . For example, to document a method, you can create a snippet:
/**
<#Brief#>
<#Description#>
@param <#Name#> <#Info#>
@param <#Name#> <#Info#>
@return <#Returns#>
*/
and hang it on a shortcut
docmethod
: 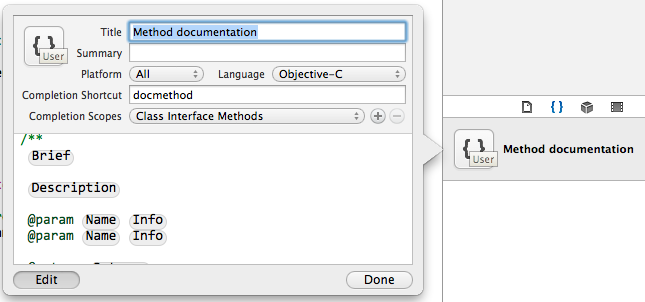
Then, when typing,
docmethod
auto-completion will automatically offer the appropriate template.