
Mobile web development: gestures, frameworks, numbers
Continuing the topic of web development of mobile sites and applications for mobile devices, one cannot help but touch upon a topic such as Framework for manipulating the DOM and sending asynchronous requests.
The standard on the desktop has long been jQuery, but it is no good for development on a mobile platform. Let's see how jQuery makes friends with mobile devices.
The first jQuery problem is tachi. Processing clicks with the click event is not a good idea. Due to the fact that mobile browsers have to support the click event, we can do it, but at a high price. On mobile devices, click the event is not called until after 300ms after the user touched the screen, to determine whether it is scrolling or double tap or something else and the click event processing will work, and this may seem insignificant, but the reaction to another action through other 300ms will cause a noticeable delay.
To avoid this, you can use tap events that are supported by the jQuery satellite - jQuery Mobile. But jQuery Mobile is also not the best solution. Firstly, it is very large in size, like jQuery and even services that allow you to cut parts of jQuery do not help to reduce its size sufficiently. The second major flaw is the lack of gesture support.
The mobile version of WebKit (iOS, Android) supports the following events:
So, in order to swipe svayp we need the following code:
A working example:
http://jsfiddle.net/sagens/zNvtL/2/

Due to the complexity of gesture processing, you can refer to jQuery alternatives that support gestures
In search of alternatives, the following requirements can be put forward:
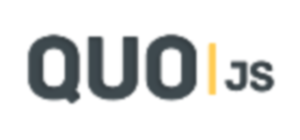
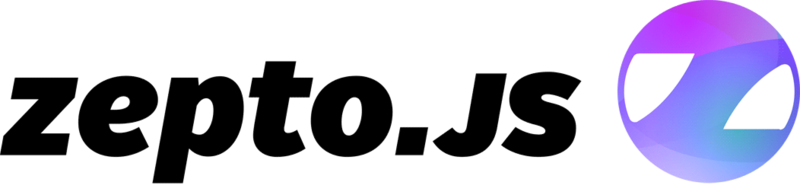
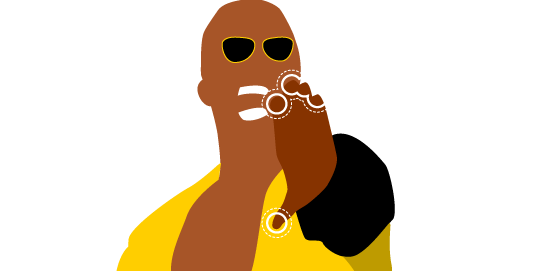
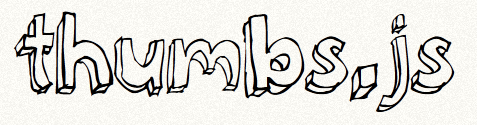
The standard on the desktop has long been jQuery, but it is no good for development on a mobile platform. Let's see how jQuery makes friends with mobile devices.
jQuery and mobile devices.
The first jQuery problem is tachi. Processing clicks with the click event is not a good idea. Due to the fact that mobile browsers have to support the click event, we can do it, but at a high price. On mobile devices, click the event is not called until after 300ms after the user touched the screen, to determine whether it is scrolling or double tap or something else and the click event processing will work, and this may seem insignificant, but the reaction to another action through other 300ms will cause a noticeable delay.
To avoid this, you can use tap events that are supported by the jQuery satellite - jQuery Mobile. But jQuery Mobile is also not the best solution. Firstly, it is very large in size, like jQuery and even services that allow you to cut parts of jQuery do not help to reduce its size sufficiently. The second major flaw is the lack of gesture support.
Gestures in theory.
The mobile version of WebKit (iOS, Android) supports the following events:
- touchstart - triggered when touching. Analog - mouseDown
- touchmove - Triggered when the touch moves. Analog - mouseMove
- touchend - Triggered when tapping ends. Analog - mouseUp
- touchcancel - Initiated when the touch is interrupted (User locked the screen, pressed the home button, changed the screen orientation)
So, in order to swipe svayp we need the following code:
var touchstartX = 0;
var touchstartY = 0;
var touchendX = 0;
var touchendY = 0;
var gesuredZone = document.getElementById('gesuredZone');
gesuredZone.addEventListener('touchstart', function(event) {
touchstartX = event.screenX;
touchstartY = event.screenY;
}, false);
gesuredZone.addEventListener('touchend', function(event) {
touchendX = event.screenX;
touchendY = event.screenY;
handleGesure();
}, false);
function handleGesure() {
var swiped = 'swiped: ';
if (touchendX < touchstartX) {
alert(swiped + 'left!');
}
if (touchendX > touchstartX) {
alert(swiped + 'right!');
}
if (touchendY < touchstartY) {
alert(swiped + 'down!');
}
if (touchendY > touchstartY) {
alert(swiped + 'left!');
}
if (touchendY == touchstartY) {
alert('tap!');
}
}
A working example:
http://jsfiddle.net/sagens/zNvtL/2/

Due to the complexity of gesture processing, you can refer to jQuery alternatives that support gestures
JQuery alternatives
In search of alternatives, the following requirements can be put forward:
- DOM manipulation
- Gesture handling
- Asynchronous requests
- Small size
Frameworks
Quo.JS.
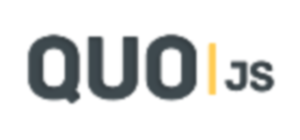
- Link to the Framework: http://quojs.tapquo.com/
- Supported Events: Tap, Single Tap, Double Tab, Hold, 2xFingers Tap, 2xFingers Double Tap, Swipe Up, Swipe Right, Swipe Down, Swipe Down, Swipe Left, Swipe, Drag, Rotate Left, Rotate Right, Rotate, Pinch Out, Pinch, Fingers
- Framework Size: 18KB minimized.
- Pros:
- In addition to events, it supports CSS selectors, work with attributes, work with DOM and AJAX requests very similar to analogues in jQuery
- Requires nothing extra to work
- Small size of the framework
- Migrating with jQuery is pretty simple, except for using $$ instead of $ to avoid conflicts, but this is easily treated
- Minuses:
- No documentation available
Zepto.JS
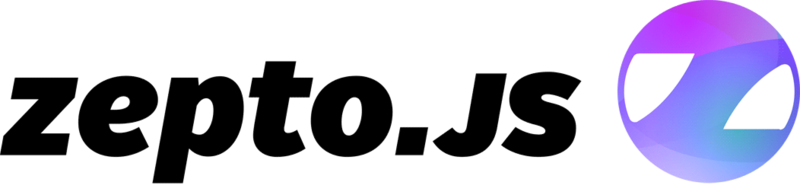
- Link to the Framework: http://zeptojs.com
- Supported Events: Tap, Single Tap, Double Tap, Long Tap, Swipe, SwipeLeft, SwipeRight, SwipeUp, SwipeDown
- Framework Size: 11KB minimized.
- Pros:
- A framework focused on mobile development. Also has a jQuery-like style and the code is almost compatible with jQuery
- Only supports modern browsers
- Minuses:
- Does not support Drag and Multitouch *
Libraries
Hammer.js
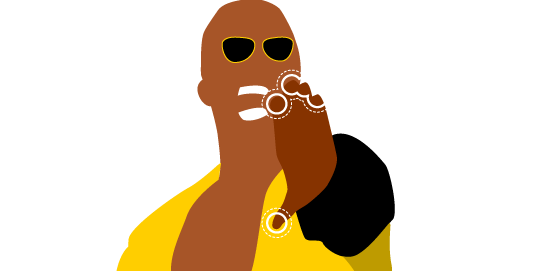
- Link to the library: http://eightmedia.github.io/hammer.js
- Supported Events: Hold, Tap, Doubletap, Drag, Dragstart, Dragend, Dragup, Dragdown, Dragleft, Dragright, Swipe, Swipeup, Swipedown, Swipeleft, Swiperight, Transform, Transformstart, Transformend, Rotate, Pinch, Pinchin, Pinchout, Touch, Release
- Library size: 13KB minimized.
- Pros:
- A large number of supported gestures including Touch and Release for tracking complex gestures
- There is a plugin for jQuery
- The plugin is fully compatible with Zepto.JS
- Minuses:
- The size. 13 KB - too much for gestures only
Jester
- Link to the library: https://github.com/plainview/Jester
- Supported Events: Tap, Double tap, Swipe, Flick, Pinch, Pinch arrow, Pinch widen, Pinch end
- Library size: 17KB minimized.
- Pros:
- Standalone Library
- Minuses:
- Missing Multitouch * and Drag
Thumbs.js
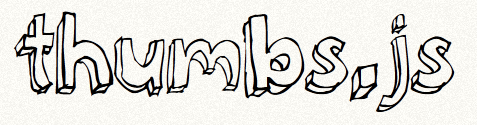
- Link to the library: http://mwbrooks.github.io/thumbs.js/
- Supported Events: touchstart, touchend, touchmove
- Library size: 612B minimized.
- Pros:
- Standalone Library
- Small size
- Minuses:
- It simply adds support for standard events touchstart, touchend, touchmove. Gestures need to be processed by ourselves.
*On a note:
The standard Android browser does not support Multitouch up to version 3.2. This means that Multitouch will not work on any application running in PhoneGap on Android versions up to 3.2.Counting parrots
When selecting elements by class, the situation is as follows:
How the raw class was implemented Robert Nyman http://code.google.com/p/getelementsbyclassname/
More results: http://jsperf.com/zepto-vs-jquery-selectors/12Total
As you can see from the review of leaders there is no, and for each case you need to use something of your own. Since my application uses gestures very actively and I needed to manage the DOM and send cross-domain requests, I settled on the Zepto.JS + Hammer.JS bundle. There were no compatibility issues