
AngularJS for those used to jQuery
- Transfer
- Tutorial
AngularJS is a great framework for building web applications. He has great documentation with examples. In the training "trial" applications (like TodoMVC Project ), he very worthily shows himself among the rest of the other frameworks. There are great presentations and screencasts on it.
However, if a developer has never before come across frameworks like Angular and used libraries like jQuery to work, then it can be difficult for him to change his mindset. At least that was the case with me, and I would like to share some notes on this subject. Maybe it will be useful to someone.
This difference is easier to understand if you think about what happens during execution. What does jQuery do in runtime? Almost nothing. JQuery code is called only in response to something that happened in your code - when a trigger of one of the functions triggered by a DOM event fires.
Angular, at the loading stage, turns your DOM tree and code into an angular application. HTML markup of a page with angular directives and filters in it is compiled into a template tree, the corresponding scope and controllers are attached to them in the right places, the application’s internal loop ensures the correct data binding between the view and the model. This is a real working circuit in full compliance with the principles of MVC, providing a very clean separation between the view, the controller and the model. If we talk about the general cycle of events, page rendering and data binding, then you can assume that it runs continuously all the time, while calling code from your controllers only when necessary.
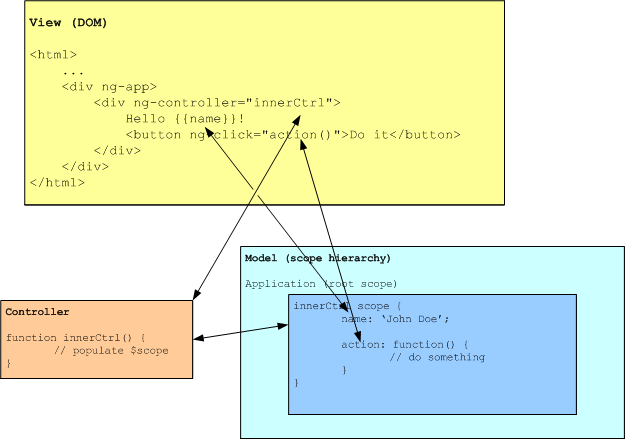
Each time the model is updated (no matter through an asynchronous AJAX request or directly through changing data from the controller), Angular restarts the cycle of the special procedure $ digest, which updates the data bindings and keeps the entire system up to date.
Suppose we want to show and hide some element based on the state of the checkbox. In jQuery, we would do something like this:
Please note that the javascript code here perceives the DOM from the point of view of the imperative approach: take this element and its attribute, look at its value, do so-and-so.
Now let's look at the same thing in terms of Angular:
And that’s it! There is no code at all, just a pure declarative style for defining bindings and rules. Here is a demonstration of this code in jsFiddle: jsfiddle.net/Y2M3r
Direct manipulation of the DOM does not just stop being mandatory; even more so, their use in the Angular approach is highly discouraged. The DOM tree must be fully defined in the templates, data in the models and scopes, functionality in the controllers, any non-standard transformations in its own filters and directives.
This clear division of tasks at first glance seems difficult to digest, but as the project grows, it will pay off handsomely: the code is easy to maintain, it is easy to divide into modules, it is convenient to test and research.
Demo: jsfiddle.net/6UnVA/1
Suppose you have some source of JSON data wrapped in a special $ resource service on the Angular side.
Any controller function that needs this data can include a DataSource in its list of parameters. This is all that is required of her. This is such a little street magic that never ceases to amaze me every day when working with AngularJS. Do you need to make asynchronous HTTP requests from the controller? Add $ http to the options. Need to write to the console log? Add $ log as an argument to your function.
Inside at this point, the following happens: Angular parses the source code of the function, finds a list of arguments, and concludes what services your code requires.
Angular has good presets for the usual operations of fetching, deleting, retrieving, and modifying data. And the special parameters in the URL give you the ability to customize access according to your needs.
Other important points that have been ignored here are, for example, form validation, unit testing ( I personally consider unit testing to be even more important than much of this article - approx. Transl. ) And the angular-ui library. Perhaps in future posts.
However, if a developer has never before come across frameworks like Angular and used libraries like jQuery to work, then it can be difficult for him to change his mindset. At least that was the case with me, and I would like to share some notes on this subject. Maybe it will be useful to someone.
Not a library
The first thing to understand about Angular: this is a completely different tool. jQuery is a library. AngularJS is a framework. When your code works with the library, it decides when to call this or that function. In the case of the framework, you implement event handlers, and the framework already decides at what point to call them.This difference is easier to understand if you think about what happens during execution. What does jQuery do in runtime? Almost nothing. JQuery code is called only in response to something that happened in your code - when a trigger of one of the functions triggered by a DOM event fires.
Angular, at the loading stage, turns your DOM tree and code into an angular application. HTML markup of a page with angular directives and filters in it is compiled into a template tree, the corresponding scope and controllers are attached to them in the right places, the application’s internal loop ensures the correct data binding between the view and the model. This is a real working circuit in full compliance with the principles of MVC, providing a very clean separation between the view, the controller and the model. If we talk about the general cycle of events, page rendering and data binding, then you can assume that it runs continuously all the time, while calling code from your controllers only when necessary.
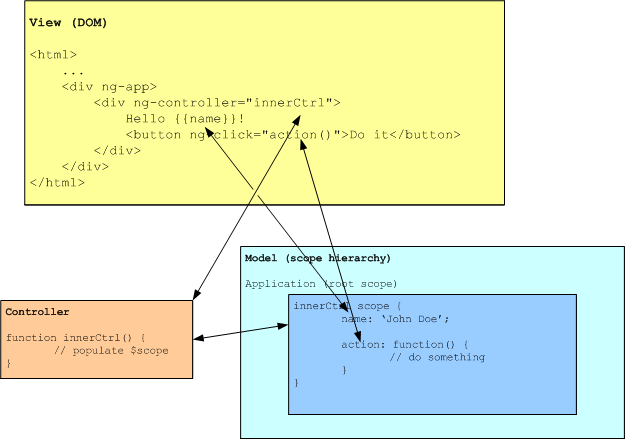
Each time the model is updated (no matter through an asynchronous AJAX request or directly through changing data from the controller), Angular restarts the cycle of the special procedure $ digest, which updates the data bindings and keeps the entire system up to date.
A declarative approach instead of an imperative
Unlike some other libraries and frameworks, Angular does not regard HTML as a problem that needs to be solved (I won’t point it with a finger). Instead, he expands them in such a natural way that inevitably you will think about why he had not thought of this before. This is easier to show than to explain.Suppose we want to show and hide some element based on the state of the checkbox. In jQuery, we would do something like this:
Этот элемент будет пропадать и возвращаться, когда вы нажимаете на чекбокс
$(function() {
function toggle() {
var isChecked = $('#toggleShowHide).is(':checked');
var specialParagraph = $('#specialParagraph');
if (isChecked) {
specialParagraph.show();
} else {
specialParagraph.hide();
}
}
$('#toggleShowHide).change(function() {
toggle();
});
toggle();
});
Please note that the javascript code here perceives the DOM from the point of view of the imperative approach: take this element and its attribute, look at its value, do so-and-so.
Now let's look at the same thing in terms of Angular:
Этот элемент будет пропадать и возвращаться, когда вы нажимаете на чекбокс
And that’s it! There is no code at all, just a pure declarative style for defining bindings and rules. Here is a demonstration of this code in jsFiddle: jsfiddle.net/Y2M3r
Direct manipulation of the DOM does not just stop being mandatory; even more so, their use in the Angular approach is highly discouraged. The DOM tree must be fully defined in the templates, data in the models and scopes, functionality in the controllers, any non-standard transformations in its own filters and directives.
This clear division of tasks at first glance seems difficult to digest, but as the project grows, it will pay off handsomely: the code is easy to maintain, it is easy to divide into modules, it is convenient to test and research.
Two-way data binding
The process of binding values from the DOM to model data through the controller and its scope creates the most “binding” in the full sense of the word. Most likely, you already know this from the documentation and examples, but I just can't help but say this. This is one of the strongest first impressions of using Angular.Hello {{yourName}}!
Demo: jsfiddle.net/6UnVA/1
Dependency Injection
I will express myself somewhat confidently, but Angular has the most elegant way to track dependencies in the world.Suppose you have some source of JSON data wrapped in a special $ resource service on the Angular side.
DataSource = $resource(url, default_params, method_details)
(refer to the documentation for details) Any controller function that needs this data can include a DataSource in its list of parameters. This is all that is required of her. This is such a little street magic that never ceases to amaze me every day when working with AngularJS. Do you need to make asynchronous HTTP requests from the controller? Add $ http to the options. Need to write to the console log? Add $ log as an argument to your function.
Inside at this point, the following happens: Angular parses the source code of the function, finds a list of arguments, and concludes what services your code requires.
Data access
Besides the fact that Angular gives you complete freedom of choice in how to organize the data in the model (you can use simple variables, objects and arrays in any combination), it also provides a convenient way to communicate with the REST API on the server. For example, we want to receive and save user records. Here is how access to them can be arranged:var User = $resource('/user/:userId', {userId:'@id'});
var user = User.get({userId:123}, function() {
user.abc = true;
user.$save();
});
Angular has good presets for the usual operations of fetching, deleting, retrieving, and modifying data. And the special parameters in the URL give you the ability to customize access according to your needs.
Other important points that have been ignored here are, for example, form validation, unit testing ( I personally consider unit testing to be even more important than much of this article - approx. Transl. ) And the angular-ui library. Perhaps in future posts.