
Creating your own control based on TabControl in C #
- Tutorial
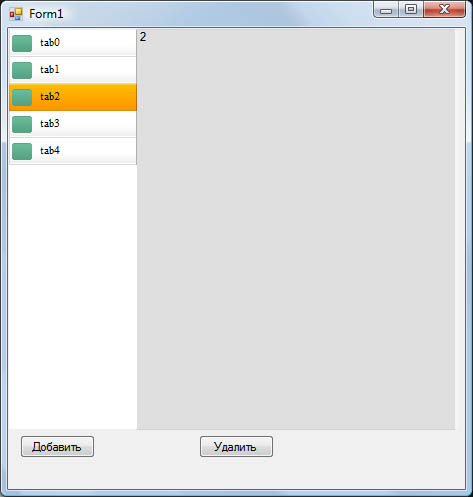
At one point, the task arose to make TabControl according to the rendered design, with tabs on the left side. The difficulty was that the project was already written using winform, and I did not want to redo it entirely. I tried to implement this task using the classic TabControl, but met with many problems associated with this.
The first problem was that if we position the tabs on the left side, we get the following picture:
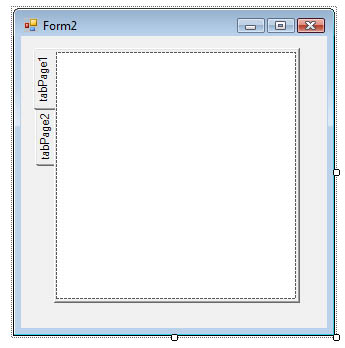
But I needed the inscriptions to go horizontally. Having studied this control a little deeper, I decided to use the parameter DrawMode = OwnerDrawFixed. All inscriptions were erased, and it became possible to write and draw on the button. But there remained the background of the button itself, which could not be completely painted over.
The next step I changed Appearance from Normal to Buttons, there was also the FlatButtons option, but I couldn’t install it through the constructor, and setting the code did not affect anything.
In Buttons mode, such
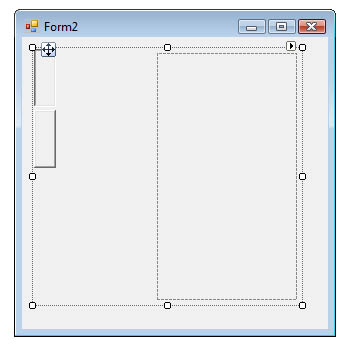
crap came out: The picture shows that there is a distance between the buttons and the TabPages set. It came from there and what parameter is regulated, I could not find out.
For some time, I studied the existing paid and free control libraries for the possibility of changing TabControl tabs for myself, but they either suggested using pre-created styles, or letting the color change as much as possible.
As a result, having tormented myself with it, I decided to write my own control, taking the standard as the basis. The goal was to hide the standard tabs and replace them with their own, tying them to control.
I will try to describe in detail everything that had to be done for this.
Step 1
First you need to create a new control in the project. To do this, in the Solution Explorer panel, right-click on the project, then Add-> Component, in the panel that opens, enter the name of the new control (I have NewTabControl)
Step 2
After creating, open the code for the created control. In the code we make the following edits:
add
using System.Windows.Forms;
using System.Drawing;
We create three classes, inheriting them from the classes of standard controls.
Class New TabControl
public partial class NewTabControl: System.Windows.Forms.TabControl
New control
class public class NewTabPanel: System.Windows.Forms.Panel
Single tab
class public class PanelTP: System.Windows.Forms.Panel
Now we need to reload the following method in the NewTabControl class:
protected override void WndProc(ref Message m)
{
if (m.Msg == 0x1328 && !DesignMode) m.Result = (IntPtr)1;
else base.WndProc(ref m);
}
This action will allow us to hide the standard tabs.
Now we need to connect all the classes together. I will not describe all the code; I will attach it to this article. I will describe only the most interesting points.
Step 3
Compose all the elements in the NewTabPanel class:
private void InitializeComponent()
{
this.panel2 = new System.Windows.Forms.Panel(); //Панель с вкладками
this.tabControl = new NewTabControl();
this.Controls.Add(this.tabControl);
this.Controls.Add(this.panel2);
this.Size = new System.Drawing.Size(311, 361);
this.panel2.Dock = System.Windows.Forms.DockStyle.Left;
this.tabControl.Dock = System.Windows.Forms.DockStyle.Fill;
tabControl.ControlAdded += new ControlEventHandler(tc_ControlAdded); //Событие на создание новой вкладки
tabControl.ControlRemoved += new ControlEventHandler(tc_ControlRemoved); //Событие удаления вкладки
tabControl.Selected += new TabControlEventHandler(tc_Selected); //Событие выделения вкладки
}
Step 4
Now you can set the format of how the tab itself will look.
At this stage, you can arrange the text, image or any other element on a future tab. And also set the size and background of the tab itself.
At home, I display the icon and the name of the tab.
In the PanelTP class, create a method:
private void InitializeComponent()
{
this.Height = 27;
this.Width = 128;
this.BackgroundImage = Tabpanel.Properties.Resources.tab_c_74;
this.Click += new EventHandler(Select_Item);
PictureBox Icon;
Icon = new PictureBox();
Icon.Width = 25;
Icon.Height = 26;
Icon.Left = 3;
Icon.Top = 5;
Icon.Image = Tabpanel.Properties.Resources.green_dialbut_611;
this.Controls.Add(Icon);
Label lname;
lname = new Label();
lname.Width = 95;
lname.Height = 25;
lname.Left = 28;
lname.Top = 5;
lname.Font = new System.Drawing.Font("Times New Roman", 8f, FontStyle.Regular);
lname.Text = this.name;
lname.Click += new EventHandler(Select_Item);
this.Controls.Add(lname);
}
Step 5
I will not describe the methods that handle events; they are described in detail in the attached project. I will pass to application.
After we have saved everything, new components will appear on the Toolbox panel.
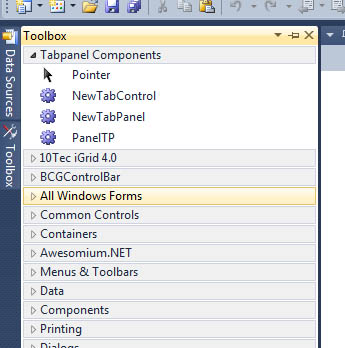
Now we can place it in our form as we want.
To add a tab, use:
newTabPanel1.TabPages.Add("TabName");
To delete:
newTabPanel1.TabPages.Remove(newTabPanel1.TabPages[id])
Where id is the vkadka number.
With such an implementation of TabControl, you can always configure sorting between tabs or hide the selected tab, make a tab of any size and design, make the tabbed panel sable and expandable.
By the same principle, you can create any of your controls by linking and programming it from the existing ones.
Perhaps for someone I described the obvious things, but I hope there are those to whom this article will be useful.
Project sources can be downloaded here .
The binary is here .
You may also be interested in my article How to connect a third-party browser in an application in C #