
Inline editing in Django
Recently, the task was to give users a convenient data editing tool. I wanted users to immediately see the result and not jump between several pages for editing and viewing. A little googling, we found an excellent django-inplaceedit application that allows you to implement visual data editing.
These are the settings we used, there are more of them in the documentation
Why might this be needed? There is an extension for django-inplaceedit , django-inplaceedit-extra-fields adding work with AutoCompleteManyToManyField and AutoCompleteForeingKeyField. The names of the adapters in both applications can match and then we can redefine them. Or you can write your own adapter.
By default, only superuser can edit information using django-inplaceedit. This can be easily fixed by overriding rights. Remember, we described above
Create the perms.py file inside my_app. Here is the file from the documentation
And how it looks before editing:
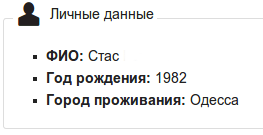
During editing:
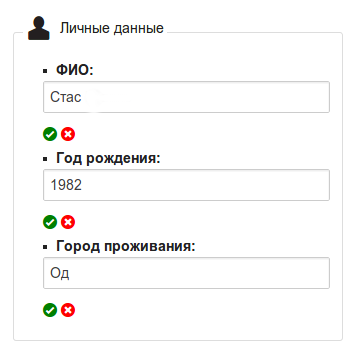
Now a screenshot of the entire page. Pay attention to textarea, there appeared a WYSIWYG editor.
View:
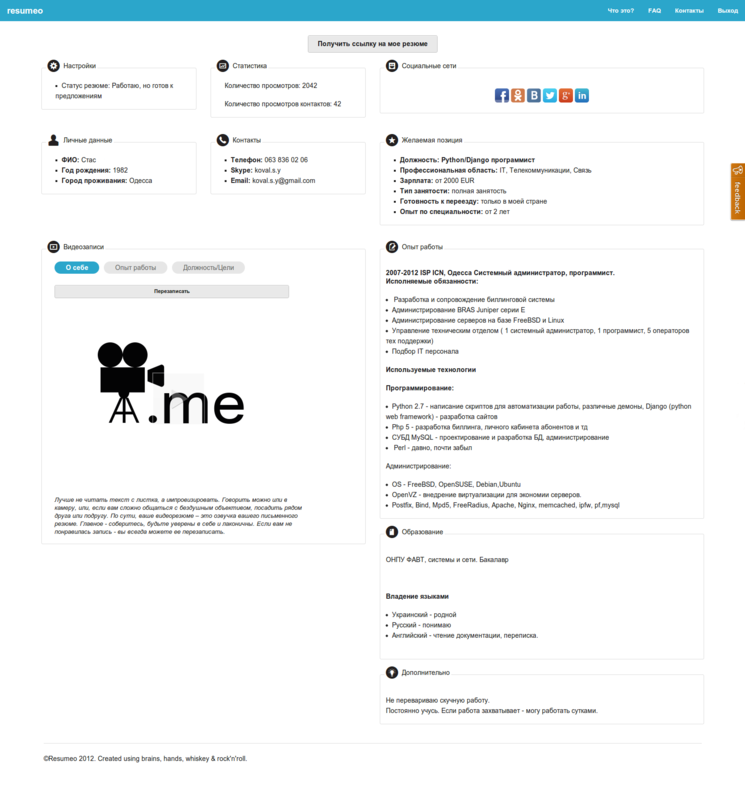
In edit mode:
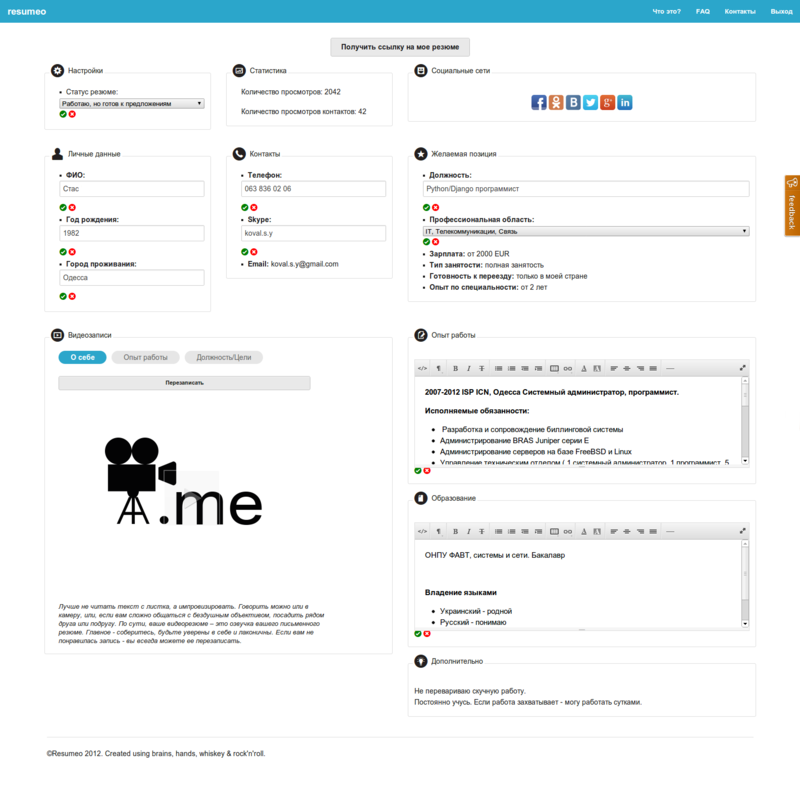
Example of an editor in a template:
And moreover, this is the same page, without a reboot. We checked on users that there were enough tooltips about the possibility of editing so that everyone could figure it out the first time.
In another project, we used the image_thumb adapter to upload and edit pictures. An error occurred while trying to edit the image:
The error occurs due to 'sorl.thumbnail' which is required when installing django-inplaceedit-extra-fields. We just found django-inplaceedit-extra-fields in the source where the first time sorl.thumbnail was called and replaced the call.
Editing the fields.py of the django-inplaceedit-extra-fields package, line 96:
Github links:
django-inplaceedit
django-inplaceedit-extra-fields
Installation from PyPI:
pip install django-inplaceedit
We connect the application to the project in settings.py:
INSTALLED_APPS = (
....
'inplaceeditform',
)
TEMPLATE_CONTEXT_PROCESSORS = (
#...#
'django.core.context_processors.request',
#...#
)
These are the settings we used, there are more of them in the documentation
ADAPTOR_INPLACEEDIT_EDIT = 'my_app.perms.MyAdaptorEditInline' # "подключаем свой perms.py, подробнее - ниже"
INPLACEEDIT_DISABLE_CLICK = False # "разрешаем сохранять изменения нажатием Enter"
THUMBNAIL_DEBUG = True
INPLACEEDIT_EVENT = "click" # "событие для вызова редактирования"
Defining your adapters or overriding default ones.
Why might this be needed? There is an extension for django-inplaceedit , django-inplaceedit-extra-fields adding work with AutoCompleteManyToManyField and AutoCompleteForeingKeyField. The names of the adapters in both applications can match and then we can redefine them. Or you can write your own adapter.
ADAPTOR_INPLACEEDIT = {'auto_fk': 'inplaceeditform_extra_fields.fields.AdaptorAutoCompleteForeingKeyField',
'auto_m2m': 'inplaceeditform_extra_fields.fields.AdaptorAutoCompleteManyToManyField',
'image_thumb': 'inplaceeditform_extra_fields.fields.AdaptorImageThumbnailField',
'tiny': 'inplaceeditform_extra_fields.fields.AdaptorTinyMCEField',}
Connection in base.html
{% load inplace_edit %}
{% inplace_toolbar %}
or
{% inplace_static %}
Connection in urls.py
urlpatterns = patterns('',
#...#
(r'^inplaceeditform/', include('inplaceeditform.urls')),
#...#
)
Editing Rights Definitions
By default, only superuser can edit information using django-inplaceedit. This can be easily fixed by overriding rights. Remember, we described above
ADAPTOR_INPLACEEDIT_EDIT = 'my_app.perms.MyAdaptorEditInline'
now in more detail. Create the perms.py file inside my_app. Here is the file from the documentation
class MyAdaptorEditInline(object):
@classmethod
def can_edit(cls, adaptor_field):
user = adaptor_field.request.user
obj = adaptor_field.obj
can_edit = False
if user.is_anonymous():
pass
elif user.is_superuser:
can_edit = True
else:
can_edit = has_permission(obj, user, 'edit')
return can_edit
I made all the checks before downloading the template with inlineedit, so my file is simpler
class MyAdaptorEditInline(object):
@classmethod
def can_edit(cls, adaptor_field):
can_edit = True
return can_edit
Use in edit template
{% extends "base.html" %}
{% load i18n inplace_edit %}
...
And how it looks before editing:
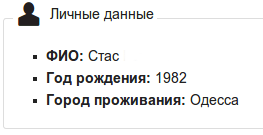
During editing:
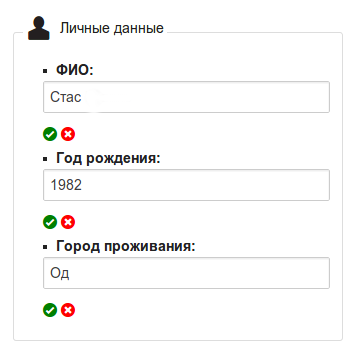
Now a screenshot of the entire page. Pay attention to textarea, there appeared a WYSIWYG editor.
View:
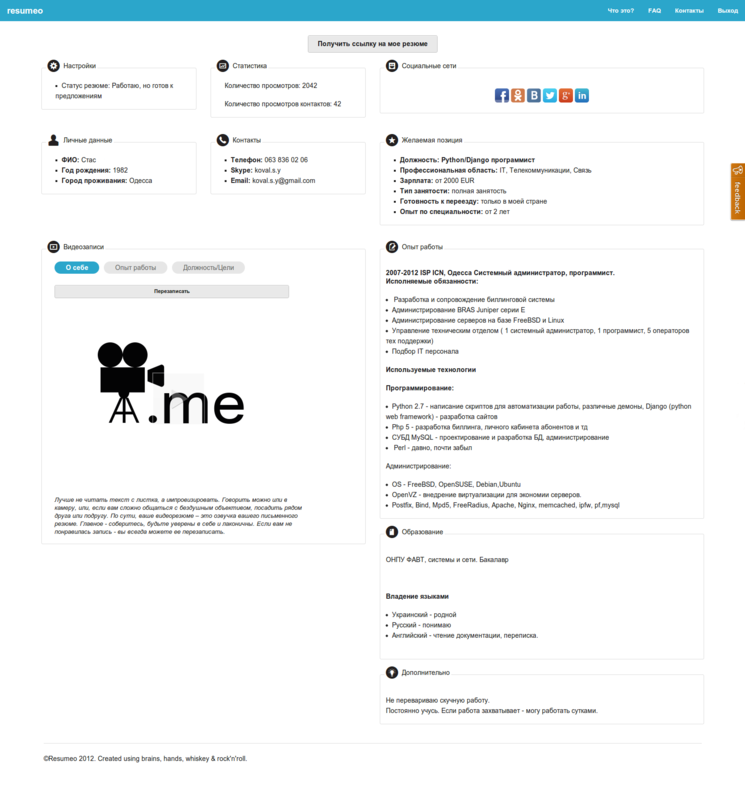
In edit mode:
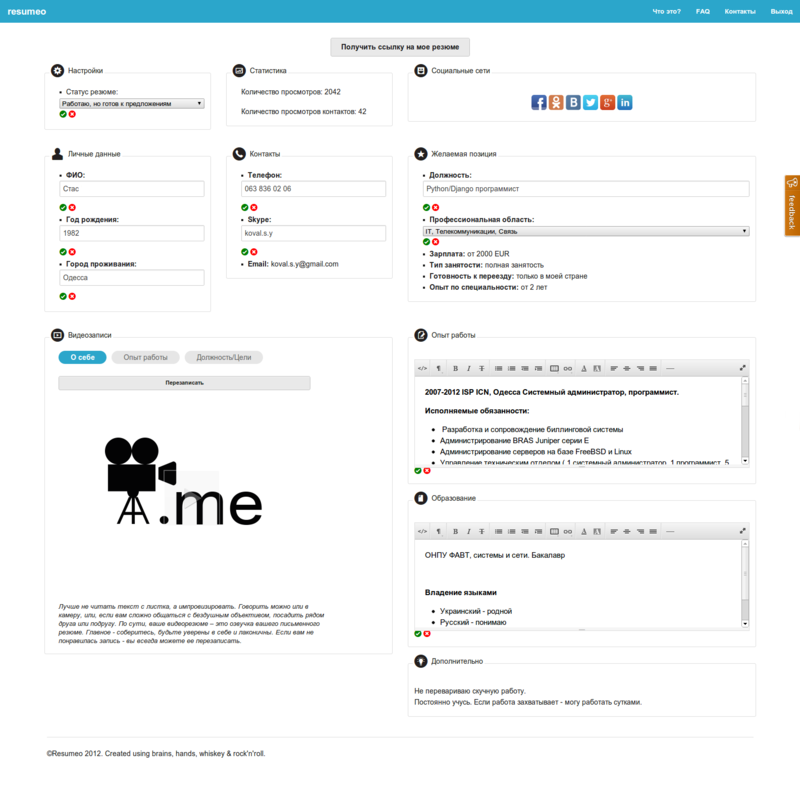
Example of an editor in a template:
{% inplace_edit "seeker.resume_workexp|safe" filters_to_show="safe", adaptor="tiny", tag_name_cover="div", auto_height=1, auto_width=1, edit_empty_value="Кликните для редактирования" %}
And moreover, this is the same page, without a reboot. We checked on users that there were enough tooltips about the possibility of editing so that everyone could figure it out the first time.
Possible problems
In another project, we used the image_thumb adapter to upload and edit pictures. An error occurred while trying to edit the image:
TemplateSyntaxError at /adverts/cabinet/
Invalid block tag: ‘endthumbnail’, expected ‘elif’,'else’ или ‘endif’
Decision
The error occurs due to 'sorl.thumbnail' which is required when installing django-inplaceedit-extra-fields. We just found django-inplaceedit-extra-fields in the source where the first time sorl.thumbnail was called and replaced the call.
Editing the fields.py of the django-inplaceedit-extra-fields package, line 96:
from sorl import thumbnail # меняем на
from easy_thumbnails import thumbnail # не забудьте установить easy_thumbnails
Github links:
django-inplaceedit
django-inplaceedit-extra-fields