Automate work with Xcode projects using Ruby
- From the sandbox
- Tutorial
Hi, Habr. There are a number of tasks for which there is no need to use the Xcode GUI. The execution time of such tasks can be reduced at least by the start time of Xcode. Saving time may seem insignificant when working with one project, but if there are many projects, the process of editing them can be very tedious. But most importantly, this approach opens up opportunities for the automation of work with projects.
I want to talk about a new tool whose purpose is to solve the described problem. XcodeProject is a Ruby language library that can be used to read and modify Xcode project data, as well as to build projects, archive and perform a number of tasks to automate working with them.
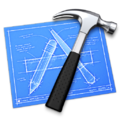
The library is a gem; you can install it from the terminal with the following command:
The simplest example of using a library that displays all the goals of a project:
First you need to create an object of type XcodeProject :: Project:
Or you can find all Xcode projects in a given directory:
Or according to the given pattern:
After the project object is created, you can read the data:
Or change the data:
We display all the groups of the highest level:
We display only the files from the given group:
You can get GroupPath groups at any time. GroupPath is the path to a group relative to the root of the project (the root of the project is the Main Group, a hidden group that is the parent of all project groups) or the current group.
Directories are groups that are represented on your file system. For them, you can get FilePath - the real path in the file system to the directory with which the object is associated.
You can add a group to the project by specifying GroupPath to it, relative to the root of the project:
Or relative to the current group:
To add a directory to the project, you must specify FilePath:
You can add a file to the project in the same way:
You can also delete files and groups from the project:
Getting the target object is simple:
After the file is added to the project, you can use it to build the target:
You can exclude a file from the assembly as follows:
XcodeProject uses XcodeBuilder to build projects.
To get started, you need to create a rakefile. In a simple case, it might look like this:
A number of tasks are now available, including building a project. A complete list of tasks can be obtained by running rake -T.
Change build parameters:
For more information on XcodeBuilder, click here .
In conclusion, a few words on the XcodeProject project. The project is under development and has only a little functionality presented in Xcode. The emergence of new opportunities will be associated with real portability in them. The project is available under the MIT license, you can use the project code as you wish. Any help in developing the project is appreciated.
Project address on github .
I want to talk about a new tool whose purpose is to solve the described problem. XcodeProject is a Ruby language library that can be used to read and modify Xcode project data, as well as to build projects, archive and perform a number of tasks to automate working with them.
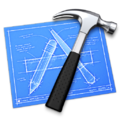
Installation
The library is a gem; you can install it from the terminal with the following command:
gem install xcodeproject
Beginning of work
The simplest example of using a library that displays all the goals of a project:
require 'rubygems'
require 'xcodeproject'
proj = XcodeProject::Project.new('path/to/example.xcodeproj')
proj.read.targets.each do |target|
puts target.name
end
First you need to create an object of type XcodeProject :: Project:
proj = XcodeProject::Project.new('path/to/example.xcodeproj')
Or you can find all Xcode projects in a given directory:
projs = XcodeProject::Project.find('path/to/dir')
Or according to the given pattern:
projs = XcodeProject::Project.find('*/**')
After the project object is created, you can read the data:
data = proj.read
p data.target('example').config('Release').build_settings
Or change the data:
proj.change do |data|
data.target('example').config('Release').build_settings['GCC_VERSION'] = 'com.apple.compilers.llvmgcc42'
end
Files, Groups, and Directories
We display all the groups of the highest level:
data.main_group.children.each do |child|
p child.name
end
We display only the files from the given group:
group = data.group('path/from/main_group')
group.files.each do |file|
p file.name
end
You can get GroupPath groups at any time. GroupPath is the path to a group relative to the root of the project (the root of the project is the Main Group, a hidden group that is the parent of all project groups) or the current group.
group.group_path
Directories are groups that are represented on your file system. For them, you can get FilePath - the real path in the file system to the directory with which the object is associated.
group.total_path
You can add a group to the project by specifying GroupPath to it, relative to the root of the project:
data.add_group('path/from/main_group')
Or relative to the current group:
group.add_group('path/from/current_group')
To add a directory to the project, you must specify FilePath:
data.add_dir('group_path/to/parent', '/file_path/to/dir')
group.add_dir('/file_path/to/dir')
You can add a file to the project in the same way:
data.add_file('group_path/to/parent', '/file_path/to/file')
group.add_file('/file_path/to/file')
You can also delete files and groups from the project:
data.remove_file('path/from/main_group')
data.remove_group('path/from/main_group')
group.remove_file('path/from/current_group')
group.remove_group('path/from/current_group')
Goals
Getting the target object is simple:
target = data.target('example')
After the file is added to the project, you can use it to build the target:
file = main_group.add_file('/file_path/to/file')
target.add_source(file)
You can exclude a file from the assembly as follows:
target.remove_source(file)
Proct assembly
XcodeProject uses XcodeBuilder to build projects.
To get started, you need to create a rakefile. In a simple case, it might look like this:
require 'rubygems'
require 'xcodeproject'
proj = XcodeProject::Project.new('path/to/example.xcodeproj')
XcodeProject::Tasks::BuildTask.new(proj)
A number of tasks are now available, including building a project. A complete list of tasks can be obtained by running rake -T.
$ rake -T
rake example:archive # Creates an archive build of the specified target(s).
rake example:build # Builds the specified target(s).
rake example:clean # Cleans the build using the same build settings.
rake example:cleanbuild # Builds the specified target(s) from a clean slate.
Change build parameters:
XcodeProject::Tasks::BuildTask.new(proj) do |t|
t.target = "libexample"
t.configuration = "Release"
end
For more information on XcodeBuilder, click here .
In conclusion, a few words on the XcodeProject project. The project is under development and has only a little functionality presented in Xcode. The emergence of new opportunities will be associated with real portability in them. The project is available under the MIT license, you can use the project code as you wish. Any help in developing the project is appreciated.
Project address on github .