React Tutorial, Part 13: Class Based Components
- Transfer
- Tutorial
Today we publish the translation of the next class of the React course. It is dedicated to class-based components. Such components are created using the class keyword.
→ Part 1: course overview, reasons for the popularity of React, ReactDOM and JSX
→ Part 2: functional components
→ Part 3: component files, project structure
→ Part 4: parent and child components
→ Part 5: starting work on a TODO application, basics of styling
→ Part 6: about some features of the course, JSX and JavaScript
→ Part 7: inline styles
→ Part 8: continued work on a TODO application, familiarity with the properties of components
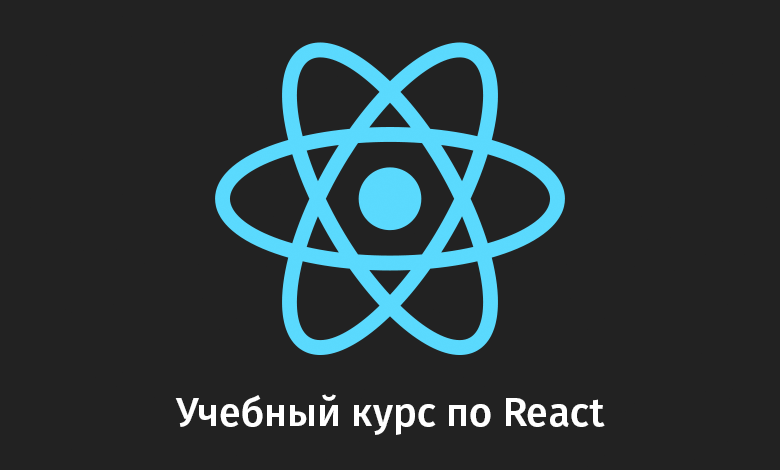
→ Part 9: properties of components
→ Part 10: workshop on working with properties of components and styling
→ Part 11: dynamic markup generation and the map array method
→ Part 12: workshop, the third stage of working on a TODO application
→ Part 13: components based on classes
→ Part 14: a workshop on components based on classes, the state of components
→ Part 15: workshops on working with the state of components
→ Part 16: the fourth stage of working on a TODO application, event handling
→ Original
If you, before you began to master this training course, studied React from the materials of some other courses, you may have a question about the fact that we use functional components here. The fact is that in many other courses this topic is either not covered, or the functional components are spoken of as something that is not particularly necessary. Some authors go even further and say that it is better not to use functional components, preferring components based on classes. This, in their opinion, saves the programmer from unnecessary load. I suppose that anyone who studies React will be useful to see the full picture and learn about the approaches that are popular today in working with components. In particular, the direction is now topical, according to which, wherever possible, use functional components, and components based on classes - only where they are really needed. It should be noted that all of this is only a recommendation. Each developer decides how he will design his applications.
When I teach courses on React, I prefer to start with functional components, since functions are understandable constructions. One glance at the functional component is enough to understand exactly what actions it performs. Say, here is the code of a functional component, which is a regular function that returns an element
But, as we delve into the study of React, become familiar with its capabilities, it turns out that functional components are not able to offer us all that we may need from React components. So today we’ll talk about class-based components. Namely, let's start by creating a component based on a class that performs the same actions as the above functional component. And in the following classes we will touch on the additional features that are given to us by class-based components. In particular, we are talking about the possibility of working with the state of components and with the methods of their life cycle.
Transform a functional component into a component based on a class. If you are not particularly familiar with the keyword
The description of a class-based component begins with a keyword
Classes in JavaScript are a superstructure over the traditional prototype inheritance model. The essence of the design
So, at this stage of working on a component based on classes, its code looks like this:
A class-based component must have at least one method. This is a method
Work with class-based components in the same way as functional components. That is, in our case, it is enough to replace the code of the functional component with a new code and the application will work in the same way as before.
Let's talk about the method
If you are familiar with classes, you can create your own method and place the code that prepares the component for visualization in it, and then call this method in the method
Namely, here we proceed from the assumption that the method
Now let's talk about how in class-based components to work with the properties passed to them when creating their instances.
When using functional components, we declared the corresponding function with a parameter
When working with a class-based component, the same goes like this:
As already mentioned, class-based components provide the developer with many features. We will talk about these opportunities. And now you can experiment with what you have learned today and prepare for a practical lesson on components that are based on classes.
Dear readers! If you use React professionally - please tell us about the situations in which you use functional components, and in which situations, components based on classes.

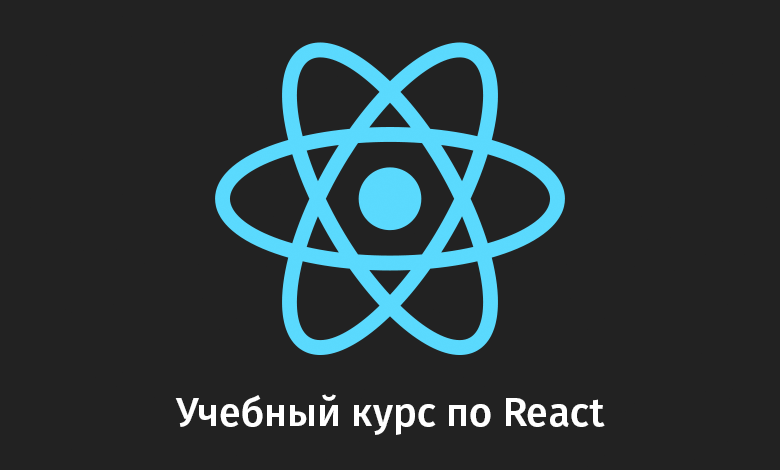
→ Part 9: properties of components
→ Part 10: workshop on working with properties of components and styling
→ Part 11: dynamic markup generation and the map array method
→ Part 12: workshop, the third stage of working on a TODO application
→ Part 13: components based on classes
→ Part 14: a workshop on components based on classes, the state of components
→ Part 15: workshops on working with the state of components
→ Part 16: the fourth stage of working on a TODO application, event handling
Lesson 24. Class Based Components
→ Original
If you, before you began to master this training course, studied React from the materials of some other courses, you may have a question about the fact that we use functional components here. The fact is that in many other courses this topic is either not covered, or the functional components are spoken of as something that is not particularly necessary. Some authors go even further and say that it is better not to use functional components, preferring components based on classes. This, in their opinion, saves the programmer from unnecessary load. I suppose that anyone who studies React will be useful to see the full picture and learn about the approaches that are popular today in working with components. In particular, the direction is now topical, according to which, wherever possible, use functional components, and components based on classes - only where they are really needed. It should be noted that all of this is only a recommendation. Each developer decides how he will design his applications.
When I teach courses on React, I prefer to start with functional components, since functions are understandable constructions. One glance at the functional component is enough to understand exactly what actions it performs. Say, here is the code of a functional component, which is a regular function that returns an element
<div>
containing an element <h1>
with some text.functionApp() {
return (
<div>
<h1>Code goes here</h1>
</div>
)
}
But, as we delve into the study of React, become familiar with its capabilities, it turns out that functional components are not able to offer us all that we may need from React components. So today we’ll talk about class-based components. Namely, let's start by creating a component based on a class that performs the same actions as the above functional component. And in the following classes we will touch on the additional features that are given to us by class-based components. In particular, we are talking about the possibility of working with the state of components and with the methods of their life cycle.
Transform a functional component into a component based on a class. If you are not particularly familiar with the keyword
class
, which appeared in ES6, and with the opportunities that it opens up for developers, it is recommended to take some time to get a closer look at the classes . The description of a class-based component begins with a keyword
class
. Then comes the name of the component, composed according to the same rules as the names of the functional components. In this case, after the construction, class App
something like a curly bracket, rather than a kind of curly bracket, will follow extends React.Component
. After it, put a pair of curly braces, which will describe the body of the class. Classes in JavaScript are a superstructure over the traditional prototype inheritance model. The essence of the design
class App extends React.Component
It comes down to the fact that we are declaring a new class and pointing out that its prototype should be React.Component
. The fact that our component has this prototype allows us to use all the useful features that are available in this component React.Component
. So, at this stage of working on a component based on classes, its code looks like this:
classAppextendsReact.Component{
}
A class-based component must have at least one method. This is a method
render()
. This method should return the same thing that we usually return from functional components. Here is the full code for a class-based component that implements the same features as the above functional component.classAppextendsReact.Component{
render() {
return (
<div>
<h1>Code goes here</h1>
</div>
)
}
}
Work with class-based components in the same way as functional components. That is, in our case, it is enough to replace the code of the functional component with a new code and the application will work in the same way as before.
Let's talk about the method
render()
. If, before forming the elements returned by this method, you need to perform some calculations, they are performed in this method, before the command return
. That is, if you have some code that determines the order of formation of the visual representation of the component, this code must be placed in the method render
. For example, here you can customize the styles if you use the inline styles. Here will be the code that implements the conditional rendering mechanism, and other similar constructions.If you are familiar with classes, you can create your own method and place the code that prepares the component for visualization in it, and then call this method in the method
render
. It looks like this:classAppextendsReact.Component{
yourMethodHere() {
}
render() {
const style = this.yourMethodHere()
return (
<div>
<h1>Code goes here</h1>
</div>
)
}
}
Namely, here we proceed from the assumption that the method
yourMethodHere()
produces the formation of styles, and what it returns is written into a constant style
declared in the method render()
. Please note that a keyword is used to refer to our own method this
. Later we will talk about the features of this keyword, but for now let's focus on the design presented here. Now let's talk about how in class-based components to work with the properties passed to them when creating their instances.
When using functional components, we declared the corresponding function with a parameter
props
representing an object, which got what was passed to the component when creating its instance. It looks like this:functionApp(props) {
return (
<div>
<h1>{props.whatever}</h1>
</div>
)
}
When working with a class-based component, the same goes like this:
classAppextendsReact.Component{
render() {
return (
<div>
<h1>{this.props.whatever}</h1>
</div>
)
}
}
Results
As already mentioned, class-based components provide the developer with many features. We will talk about these opportunities. And now you can experiment with what you have learned today and prepare for a practical lesson on components that are based on classes.
Dear readers! If you use React professionally - please tell us about the situations in which you use functional components, and in which situations, components based on classes.
