Common IT tools: good or bad?
- Transfer
Would you like to always work in the same environment? So that you don’t have to go back and forth through development environments and using tools. The ideal, of course, is still far away, but the IT world is striving for it. Today we talk about a single cross-platform API library. Join now!

When developing applications for iOS and Android using Xamarin, developers can access any native API using C #. These bindings not only help you use the appropriate APIs in C #, but also provide the powerful functions of this language, such as async / await, events, delegates, etc.
This is a huge advantage for developers because they will never have to work outside of C #, regardless of whether they write general business logic, user interface, or access native functions. One of the key features that programmers often want to see when developing cross-platform applications on Xamarin is the ability to access common native functions from common code without having to write their own layers of abstractions or find a plug-in freely available created by the community.
Xamarin.Essentials embodies the dream of a single API for accessing features such as geolocation, sensor readings, secure storage, etc., for any application built on Xamarin!

In the evaluation version, available today, the Xamarin.Essentials APIs provide access to more than 25 native functions from a single cross-platform API library, which can be accessed from a common code, regardless of how the user interface is created. This means that you can use the Xamarin.Essentials API using a single Xamarin.Android application or Xamarin.Forms application adapted to work with iOS, Android and UWP. Despite the large number of functions, this library is fully optimized for the fastest possible operation and minimal impact on the size of the application, since it is protected from the linker. This means that only the APIs and functions that you use will be included in your application, and everything else will be deleted during compilation.
An evaluation version of the library that uses the basic set of cross-platform APIs can be installed into your application via NuGet:
The initial set of APIs was determined based on feedback from Xamarin developers on what features they would like to see in the cross-platform API library. Over time, we will expand this list.
Getting started using the Xamarin.Essentials API is easy in any existing application by following just a few simple steps:
1. Open an existing project or create a new one using the Blank App template in Visual Studio C # (for Android, iPhone and iPad or cross-platform).
2. Add the Xamarin.Essentials NuGet package to the project:
3. Add a reference to Xamarin.Essentials in any of the C # classes to reference the API.
4. Xamarin.Essentials requires a little additional configuration for Android projects in order to get access to special functions:
Xamarin.Essentials must be initialized using the OnCreate method within MainLauncher or any other Activity in the Android project:
To handle runtime permissions on Android, Xamarin.Essentials must get any OnRequestPermissionsResult. Add the following code to all Activity classes:
Done! Now you can start using the Xamarin.Essentials API in your application. Each API comes with complete documentation , including code snippets that you can copy into your application.
Be sure to read our Getting Started Guide for more information.
During one of my speeches on the Microsoft Build 2018, I showed how to combine the Xamarin.Forms and Xamarin.Essentials API features to assemble a cross-platform compass in less than 10 minutes. This is as easy as adding multiple images and a small compass user interface in the application:
I was able to use the Xamarin.Essentials compass API in the code above to record events when the compass changes position, update the label text and rotate the image. All this is done with a few lines of code:
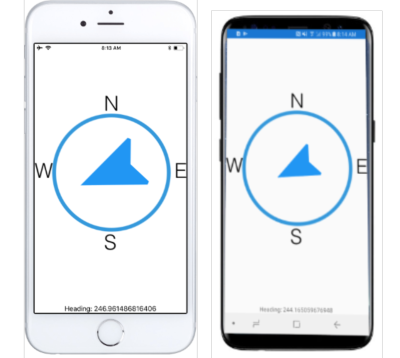
This is the simple way to create a compass application! You can find the complete source code and images on github.
Xamarin.Essentials has been thoroughly tested, but we nevertheless ask you to leave feedback during the validity period of our evaluation version. Try the library in your applications and report any problems you encounter when working with the Xamarin.Essentials GitHub repository .
We consider this library as the main element of the Xamarin platform and we know that it is an important building block for all developers and applications. Therefore, we will integrate the Xamarin.Essentials NuGet package into each iOS, Android and Xamarin.Forms template in Visual Studio and Visual Studio for Mac, so that developers have access to these APIs from the very first stages of their projects.
You can see a full review of Xamarin, Xamarin.Forms and Xamarin.Essentials as part of the presentation “Creating Mobile Applications with Visual Studio and Xamarin” on Microsoft Build 2018.
You can view our documentation containing a complete guide to getting started and using each Xamarin.Essentials feature. We also provided a complete list of API links that can be viewed online to learn about all the details of the Xamarin.Essentials APIs.

When developing applications for iOS and Android using Xamarin, developers can access any native API using C #. These bindings not only help you use the appropriate APIs in C #, but also provide the powerful functions of this language, such as async / await, events, delegates, etc.
This is a huge advantage for developers because they will never have to work outside of C #, regardless of whether they write general business logic, user interface, or access native functions. One of the key features that programmers often want to see when developing cross-platform applications on Xamarin is the ability to access common native functions from common code without having to write their own layers of abstractions or find a plug-in freely available created by the community.
Xamarin.Essentials embodies the dream of a single API for accessing features such as geolocation, sensor readings, secure storage, etc., for any application built on Xamarin!

APIs in Xamarin.Essentials
In the evaluation version, available today, the Xamarin.Essentials APIs provide access to more than 25 native functions from a single cross-platform API library, which can be accessed from a common code, regardless of how the user interface is created. This means that you can use the Xamarin.Essentials API using a single Xamarin.Android application or Xamarin.Forms application adapted to work with iOS, Android and UWP. Despite the large number of functions, this library is fully optimized for the fastest possible operation and minimal impact on the size of the application, since it is protected from the linker. This means that only the APIs and functions that you use will be included in your application, and everything else will be deleted during compilation.
An evaluation version of the library that uses the basic set of cross-platform APIs can be installed into your application via NuGet:
- Accelerometer : obtaining data on the acceleration of the movement of the device in three-dimensional space.
- Information about the application : the ability to obtain information about the application.
- Battery : determine the battery level, power source and its condition.
- Clipboard : Easy and quick editing or reading text on the clipboard.
- Compass : compass position tracking.
- Connection : monitor the status of the connection and its changes.
- Data transfer : sending text and website Uri to other applications.
- Information about the device screen : getting the device screen indicators and its orientation.
- Device Information : Easily retrieve device information.
- Email : easy emailing.
- File System Assistants : Easily save files to application data.
- Flashlight : a simple way to turn on / off the flashlight.
- Geocoding : easy forward and reverse geocoding.
- Geolocation : obtaining information about the location of the device by GPS.
- Gyroscope : obtaining information about the rotation of the device in three main axes.
- Magnetometer : Determine the orientation of the device relative to the magnetic field of the Earth.
- Browser opening : quick and easy opening of the browser with the transition to a specific website.
- Telephone dialing : open the panel to dial a telephone number.
- Settings : quick and easy change of permanent settings.
- Screen lock : Prevent device screen lock.
- Secure storage : secure data storage.
- SMS : easy SMS sending.
- Text-to-speech : Speaking text on the device.
- Version Tracking : track application version and build number.
- Vibration : Enable device vibration.
The initial set of APIs was determined based on feedback from Xamarin developers on what features they would like to see in the cross-platform API library. Over time, we will expand this list.
Beginning of work
Getting started using the Xamarin.Essentials API is easy in any existing application by following just a few simple steps:
1. Open an existing project or create a new one using the Blank App template in Visual Studio C # (for Android, iPhone and iPad or cross-platform).
2. Add the Xamarin.Essentials NuGet package to the project:
- Visual Studio. In the Solution Explorer pane, right-click on the solution name and select Manage NuGet Packages. Find Xamarin.Essentials and install the package into ALL projects, including iOS, Android, UWP and .NET Standard Libraries.
- Visual Studio for Mac. In the Solution Explorer panel, right-click on the project name and select Add> Add NuGet Packages ... Locate Xamarin.Essentials and install the package into ALL projects, including iOS, Android and .NET Standard Libraries.
3. Add a reference to Xamarin.Essentials in any of the C # classes to reference the API.
using Xamarin.Essentials;
4. Xamarin.Essentials requires a little additional configuration for Android projects in order to get access to special functions:
Xamarin.Essentials must be initialized using the OnCreate method within MainLauncher or any other Activity in the Android project:
Xamarin.Essentials.Platform.Init(this, bundle);
To handle runtime permissions on Android, Xamarin.Essentials must get any OnRequestPermissionsResult. Add the following code to all Activity classes:
publicoverridevoidOnRequestPermissionsResult(int requestCode, string[] permissions, [GeneratedEnum] Android.Content.PM.Permission[] grantResults)
{
Xamarin.Essentials.Platform.OnRequestPermissionsResult(requestCode, permissions, grantResults);
base.OnRequestPermissionsResult(requestCode, permissions, grantResults);
}
Done! Now you can start using the Xamarin.Essentials API in your application. Each API comes with complete documentation , including code snippets that you can copy into your application.
Be sure to read our Getting Started Guide for more information.
Compass assembly
During one of my speeches on the Microsoft Build 2018, I showed how to combine the Xamarin.Forms and Xamarin.Essentials API features to assemble a cross-platform compass in less than 10 minutes. This is as easy as adding multiple images and a small compass user interface in the application:
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="*"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<Image HorizontalOptions="FillAndExpand"
VerticalOptions="FillAndExpand"
Source="compass.png"/>
<Image HorizontalOptions="Center"
VerticalOptions="Center"
Source="arrow.png"
x:Name="ImageArrow"/>
<Label Grid.Row="1"
x:Name="LabelInfo"
VerticalOptions="Center"
HorizontalOptions="Center" />
</Grid>
I was able to use the Xamarin.Essentials compass API in the code above to record events when the compass changes position, update the label text and rotate the image. All this is done with a few lines of code:
using Xamarin.Forms;
using Xamarin.Essentials;
namespaceMyCompass
{
publicpartialclassMainPage : ContentPage
{
publicMainPage()
{
InitializeComponent();
// Register for reading changes
Compass.ReadingChanged += Compass_ReadingChanged;
}
voidCompass_ReadingChanged(CompassChangedEventArgs e)
{
LabelInfo.Text = $"Heading: {e.Reading.HeadingMagneticNorth}";
ImageArrow.Rotation = e.Reading.HeadingMagneticNorth;
}
protectedoverridevoidOnAppearing()
{
base.OnAppearing();
Compass.Start(SensorSpeed.Ui);
}
protectedoverridevoidOnDisappearing()
{
base.OnDisappearing();
Compass.Stop();
}
}
}
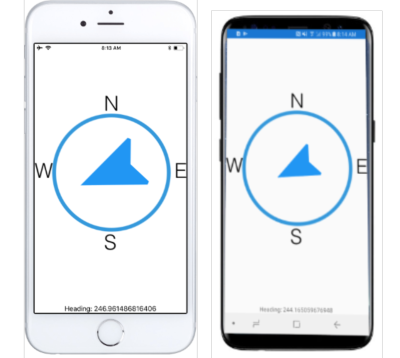
This is the simple way to create a compass application! You can find the complete source code and images on github.
Future plans
Xamarin.Essentials has been thoroughly tested, but we nevertheless ask you to leave feedback during the validity period of our evaluation version. Try the library in your applications and report any problems you encounter when working with the Xamarin.Essentials GitHub repository .
We consider this library as the main element of the Xamarin platform and we know that it is an important building block for all developers and applications. Therefore, we will integrate the Xamarin.Essentials NuGet package into each iOS, Android and Xamarin.Forms template in Visual Studio and Visual Studio for Mac, so that developers have access to these APIs from the very first stages of their projects.
See with my own eyes
You can see a full review of Xamarin, Xamarin.Forms and Xamarin.Essentials as part of the presentation “Creating Mobile Applications with Visual Studio and Xamarin” on Microsoft Build 2018.
Additional Information
You can view our documentation containing a complete guide to getting started and using each Xamarin.Essentials feature. We also provided a complete list of API links that can be viewed online to learn about all the details of the Xamarin.Essentials APIs.