
How to create a green code

Each user would like to charge their mobile device as little as possible, whether it be a smartphone, netbook, ultrabook. Perhaps someday there will come a time when the device will need to be charged only once, after its purchase and used until it is bored or morally obsolete.
If you consider the enlarged model of any mobile platform, then it consists of 3 main parts.
Battery
It is the energy storage of a mobile device. Battery manufacturers every year try to increase capacity, reduce the time of a full charge.
Iron
It is the main direct consumer of energy. Here, progress also does not stand still. Manufacturers of "iron" are creating more and more energy-efficient chips that give more performance per watt of energy consumed, add various power modes that allow you to turn off unused iron, translate into low power modes, thereby saving battery.
Soft
It is an indirect consumer of energy. Direct software does not consume anything, it forces iron to consume energy. Here, too, have their own methods to extend the battery life. I would like to talk about the problem of energy-efficiency of software in this article.
How exactly does software affect energy consumption? If in a nutshell - he does not allow the iron to "sleep".
Consider one of the largest consumers of energy in the system - the processor.
The processor can control its power consumption using the so-called C-State . For those who are not familiar with these modes, I give a brief reference:
C0 - the working state of the processor, is divided into various P-States.
C1- a state where the processor does nothing, but is ready to start work, though with a slight delay. Many processors have various variations of this state.
C2 is almost the same as C1, but in this state the processor consumes less power, and has a longer delay for the transition to the working state.
C3 - the state of "sleep", passing into this state, the processor clears the cache of the second level. It is characterized by less power consumption, and a longer transition time to working condition.
... and so on depending on the processor.
In order to make it more clearly I will give an illustration:

The most energy-efficient option - the processor always sleeps. So the most effective program in terms of energy consumption is that program that is not running and will not "wake up". It does not produce any action, and generally does not consume anything. But nobody needs such software, the program should do something useful. A compromise solution is a program that does nothing when it should not do anything (“wakes up” only when needed), and if it does something, it does it as quickly as possible.
This is especially true for programs that perform any actions in the background. These programs should always sleep and wake up only when an event occurs.
Events rule or Event-driven approach
Let me give you an example of "wrong" code (unfortunately, this approach to writing code is used much more often than you think). This code example is used to receive data from a socket, for example, in some server application.
while(true)
{
// Получаем данные
result = recv(serverSocket, buffer, bufferLen, 0);
// Обработка полученных данных
if(result != 0)
{
HandleData(buffer);
}
// Спим секунду и повторяем
Sleep(1000);
}
What is "wrong" here? There is data or not data, the code will “wake up” the processor every 1000 ms. The code’s behavior resembles a donkey from Shrek: “Already arrived? Are you here now? Are you here now? ”
The "correct" code, for this task, will not ask anyone, he will fall asleep and will wait when they wake him. For this, in many operating systems, there are synchronization objects, such as events. With that said, the code should look like this (the code is incomplete, error handling and return codes are omitted, my task is simply to illustrate the principle):
WSANETWORKEVENTS NetworkEvents;
WSAEVENT wsaSocketEvent;
wsaSocketEvent = WSACreateEvent();
WSAEventSelect(serverSocket, wsaSocketEvent, FD_READ|FD_CLOSE);
while(true)
{
// Ждем наступления одного из событий - получения данных или закрытия сокета
WaitForSingleObject(wsaSocketEvent, INFINITE);
// Что произошло?
WSAEnumNetworkEvents(m_hServerSocket, wsaSocketEvent, &NetworkEvents);
// Пришли новые данные
if(NetworkEvents.lNetworkEvents & FD_READ)
{
// Читаем, обрабатываем
WSARecvFrom(serverSocket, &buffer, ...);
}
}
What is the beauty of the example above? He will sleep when he has nothing to do.
Timers, alarms of our code
Sometimes you can not do without timers, there are a lot of examples - playing audio, video, animation.
A little bit about timers. The Windows system timer interval, by default, is 15.6 ms. What does this mean for programs? Suppose you want the application to perform an action every 40 ms above. The first interval of 15.6 ms passes, too few, the second passes 31.1, again early, the third 46.8 - hit, the timer will work. In most cases, an extra 6.8 ms does not matter.
There is also a direct effect on Sleep , if you call Sleep (1), with a set interval of 15.6 ms, then the code will not sleep 1 ms, but all 15.6 ms.
But if it comes to playing video, then this behavior is not acceptable. In these cases, the developer can change the resolution of the system timer by calling the function from the Windows Multimedia API - timeBeginPeriod. This function allows you to change the timer period up to 1ms. For code, this is good, but greatly reduces battery life (up to 25%).
How to find a compromise? All just change the period of the system timer only when it is really necessary. For example, if you are developing an application that uses animation and you need less timer resolution, change the timer when the animation is displayed and occurs, and return if, for example, the window is minimized or the animation is stopped.
From the user's point of view, sometimes, to understand how to extend battery life, a utility will be interestingPowercfg . With it, you can find out some application has changed the period of the system timer, the value of the period of the system timer, information about driver problems that do not allow you to put hardware into low power mode, etc.
Timer Association
Windows 7 has a great opportunity to combine timers. What it is and how it works is presented in the figure below:
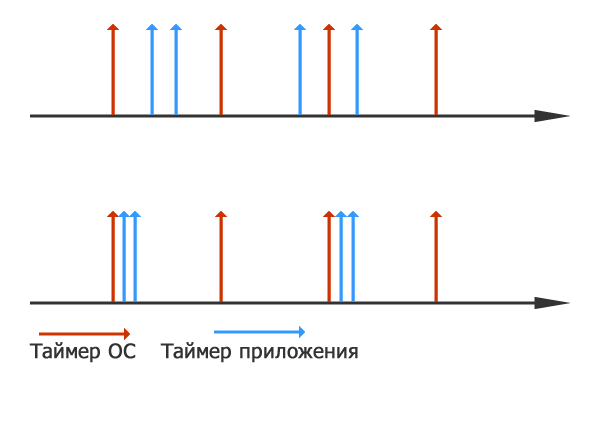
Those. Windows "adjusts" the application timers so that they coincide with the timer operations of the operating system itself.
In order to use this feature, you must call
BOOL WINAPI
SetWaitableTimerEx(
__in HANDLE hTimer,
__in const LARGE_INTEGER *lpDueTime,
__in LONG lPeriod,
__in_opt PTIMERAPCROUTINE pfnCompletionRoutine,
__in_opt LPVOID lpArgToCompletionRoutine,
__in_opt PREASON_CONTEXT WakeContext,
__in ULONG TolerableDelay
);
You can find the full description of the function in MSDN . In this article, we are only interested in the TolerableDelay parameter, which determines the maximum allowable deviation from a given interval.
For more information on timers in Windows, see Timers, Timer Resolution, and Development of Efficient Code
Do it fast
Another way to make a program more energy-efficient is to teach it how to do the right thing as quickly as possible. This can be achieved, for example, by optimizing the code by using SSE, AVX and other hardware capabilities of the platform. As an example, I want to use the Quick Sync in Sandy Bridge to encode and decode video. At Tom's Hardware you can see the results.
Suppose we have optimized our program, but how much more energy-efficient is it now, how to evaluate it? Very simple - using special programs and tools.
Energy Efficiency Analysis Tools
1. Intel Power Checker . Perhaps the easiest and fastest way to evaluate the energy efficiency of your program.
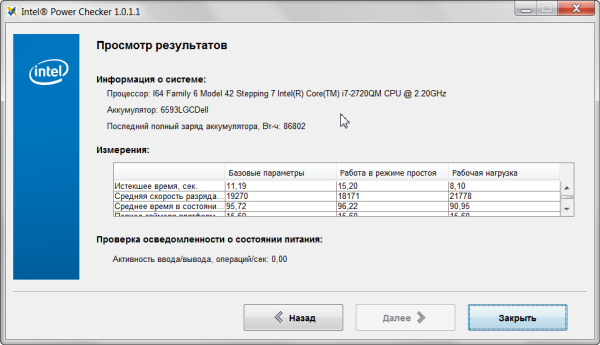
An overview and description of the program can be found on the ISN
2 blog . Intel Battery Life analyzer
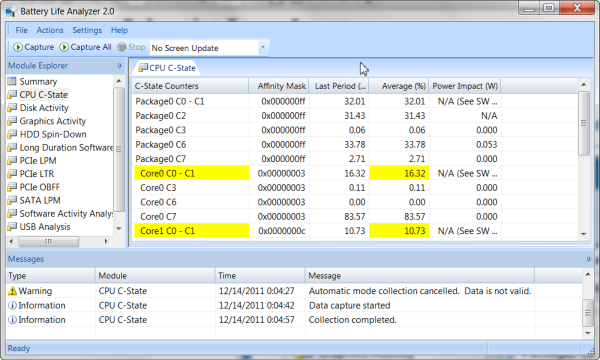
A more complex, but at the same time more informative tool, is used to track various activities of iron and software that affect battery life.
3. Microsoft Joulemeter
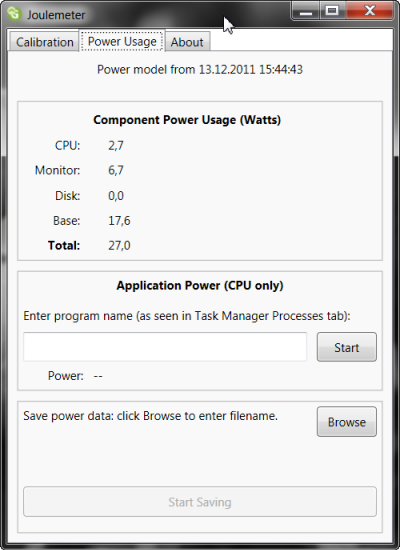
Also quite an interesting tool that determines the energy consumption of various components of the platform. It can work in conjunction with the WattsUp wattmeter .
Where to find out more
Intel Power Efficiency Community articles, how-tos, and tips for creating energy-efficient software.
Battery Life and Energy Efficiency collection of articles and recipes from Microsoft
Timers, Timer Resolution, and Development of Efficient Code The link is already given above, for those who begin to read the article from the end.
If you have questions, ask in the comments. You can also ask me your questions on the development of "green" software at the webinar , which will be held tomorrow, December 15 at 11 am.