Xcode 10.2, macOS Mojave 10.14.4, iOS 12.1 and other beta versions

New Xcode beta, macOS Mojave and iOS have been released - and here are the main points that I learned.
Swift 5 for Xcode 10.2 beta
Swift
First of all, the latest beta version of Xcode comes bundled with the new version of Swift: Let's start with the most exciting news:
Apple Swift 5.0 (swiftlang-1001.0.45.7 clang-1001.0.37.7)
Target: x86_64-apple-darwin18.2.0
ABI version: 0.6
Swift apps no longer include the DLL for the Swift standard library and the Swift SDK overlay in versions for iOS 12.2, watchOS 5.2 and tvOS 12.2. As a result, Swift applications are reduced in size when deployed for testing in TestFlight or when compressing an archive for a distribution.ABI is finally going to be stable, and this is great news. In my opinion, today this is one of the key problems of Swift - not because of side effects, but because of unfulfilled promises. Some of my friends even rewrite extensions for Apple Watch on Objective-C to reduce the size of a binary file (from about 15 MB to 1 MB). Learn more about the ABI may be able to: Dashboard Swift ABI and manifest Swift ABI stability .
The @dynamicCallable attribute allows you to call named types as well as functions using simple syntactic sugar. The main purpose is to ensure compatibility with dynamic languages. ( SE-0216 )
Example:
@dynamicCallable struct ToyCallable {
func dynamicallyCall(withArguments: [Int]) {}
func dynamicallyCall(withKeywordArguments: KeyValuePairs<String, Int>) {}
}
let x = ToyCallable()
x(1, 2, 3)
// Desugars to `x.dynamicallyCall(withArguments: [1, 2, 3])`
x(label: 1, 2)
// Desugars to `x.dynamicallyCall(withKeywordArguments: ["label": 1, "": 2])
This topic is quite extensive, and the innovation makes me have mixed feelings. Paul Hudson was able to talk about it from a neutral position in the article " What's new in Swift 5.0 ".
Swift 3 compatibility mode is excluded. Supported flag values -swift-version
are 4, 4.2, and 5.
The era is leaving: source-level compatibility with Swift 3 is no longer. This step was expected, it was announced in Swift 5 Roadmap, and yet. I strongly advise you to refresh your memory by reading the Swift 5.0 Release Process . Swift 5 is already near - be prepared.
In Swift 5 mode, switch statements on enumerations declared in Objective-C or coming from system frameworks must process unknown values — that is, those that may be added in the future or that can be defined separately in the Objective-C implementation file.
Formally, Objective-C allows any value to be included in an enumeration if it corresponds to the base type. These unknown cases can be processed using the new case@unknown default
, which still gives a warning if any known cases in the switch are missing. They can be controlled with the help of the usualdefault
. If you specify an enumeration in Objective-C and do not want clients to have to handle unknown values, use a macroNS_CLOSED_ENUM
insteadNS_ENUM
. The Swift compiler recognizes it and does not require you to set itdefault
in a switch statement.
In Swift 4 and 4.2 modes you can also use@unknown default
. If you do not do this and skip the unknown value in the switch, the program will be interrupted - just as it was in Swift 4.2 and Xcode 10.1 ( SE-0192 )
This is a long-standing problem that still causes inconvenience, especially if you prefer not to set it in switches
default
. I remember how much torment the .provisional
type appeared in iOS 12 UNAuthorizationOptions
. Now, with the introduction of the case unknown
, to cope with such situations has become much easier.Swift Package Manager
Now packages can choose the minimum required version for the Apple platform (macOS, iOS) when using the Package.swift configuration file from Swift 5. Building a package is not possible if at least one of the dependencies the minimum platform version is greater than the version specified for the package itself. ( SE-0236 )
In my opinion, this is the main news regarding SPM. In fact, this feature can solve many problems that prevent SPM from becoming useful in the iOS world. In my last article, I tried to analyze the current state of SPM in the context of iOS development. It seems that now I will have to revise the conclusions.
But there are problems:
- in some projects there is a regression of the compile time compared to previous releases;
- projects for the command line crash at launch, giving an error
dyld: Library not loaded
(the library is not loaded). Crutch: Set custom build settingsSWIFT_FORCE_STATIC_LINK_STDLIB=YES
.
The change log lists many corrected problems and other information about Swift 5 that may be important to your business. Review them: you may decide to use inherited assigned initializers with a variable number of arguments. Or, suddenly, you are faced with the problem of interlocking due to complex recursive type definitions with classes and generics. Or generic pseudonyms in the method
@objc
cause you inconvenience.Xcode 10.2 beta
Clang compiler
There are many new alerts for the Clang compiler, and most of them are related to frameworks and modules. This fact is quite interesting, since it ( presumably ) speaks of integrating the Swift Package Manager as a tool for managing dependencies. Here, in my opinion, the most important points:
- A new diagnostic identifies framework headers that use inclusions with quotes instead of framework-style inclusions. By default, the alert is disabled, but you can turn it on by passing the
clang
option-Wquoted-include-in-framework-header
;- open framework headers may erroneously execute
#import
or#include
closed headers, which leads to disruption of the structure, and sometimes to the cyclic import of modules. About such violations will report a new diagnosis. It is disabled by defaultclang
and controlled by the flag-Wframework-include-private-from-public
;- using
@import
the framework in the headers does not allow using them without modules. New diagnostics searches@import
in the headers when passing the flag—fmodules
. By default, it is disabled and controlled by the flag-Watimport-in-framework-header
;- Previously, the loss of a keyword
framework
when declaring a module for a framework did not interfere with the compilation, but silently led to an incorrect result. New diagnostics-Wincomplete-framework-module-declaration
and new fix will offer you to add the desired key. This warning starts automatically when theclang
flag is transmitted—fmodules
.
First of all: how to turn them on? Go to the Build Settings menu for your project, select the Apple Clang - Custom Compiler Flags option and set the required flag in the Other C Flags item .
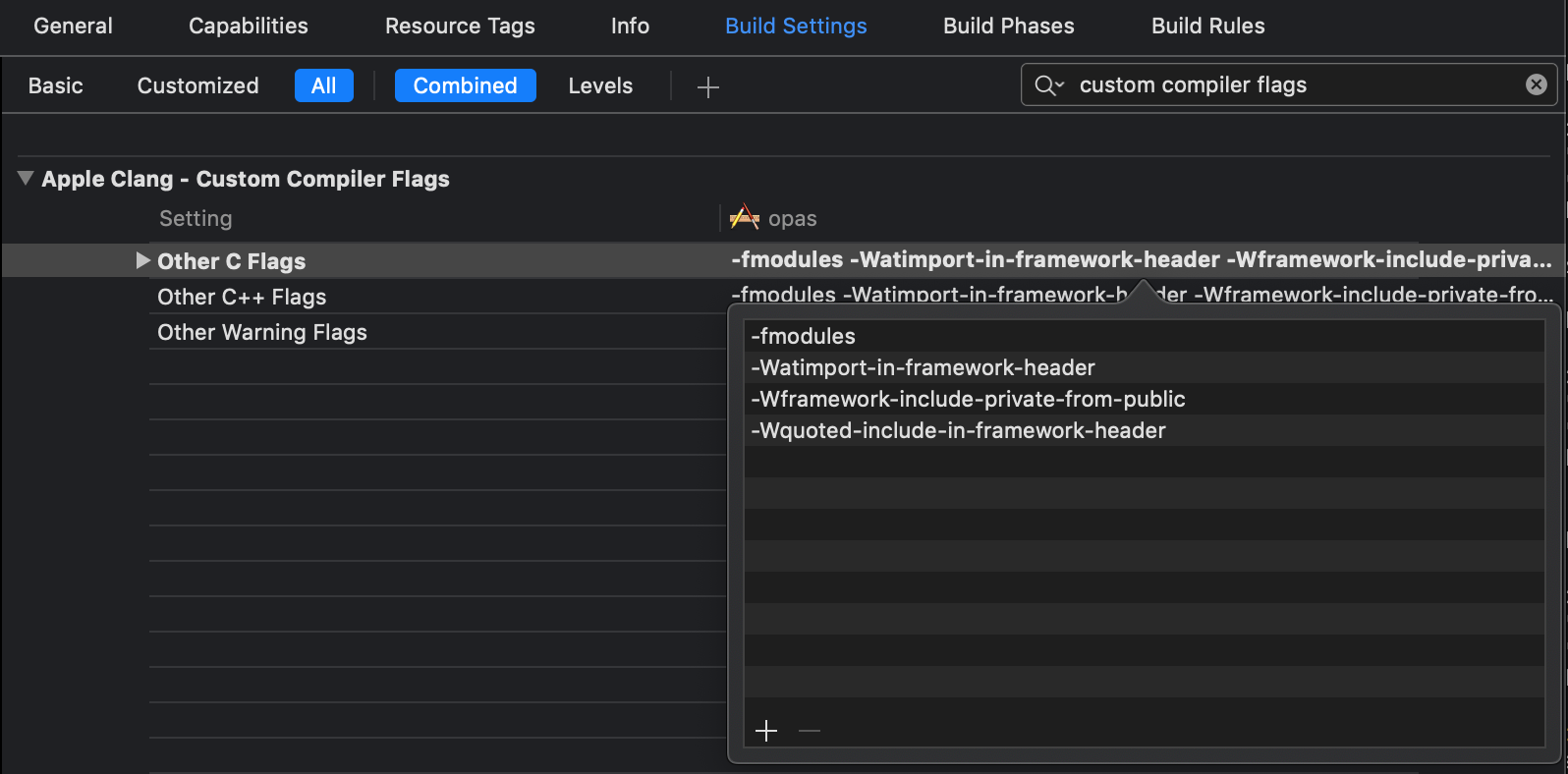
I tried to build an old Objective-C application and found a lot of problems with closed headers in the open framework headers:
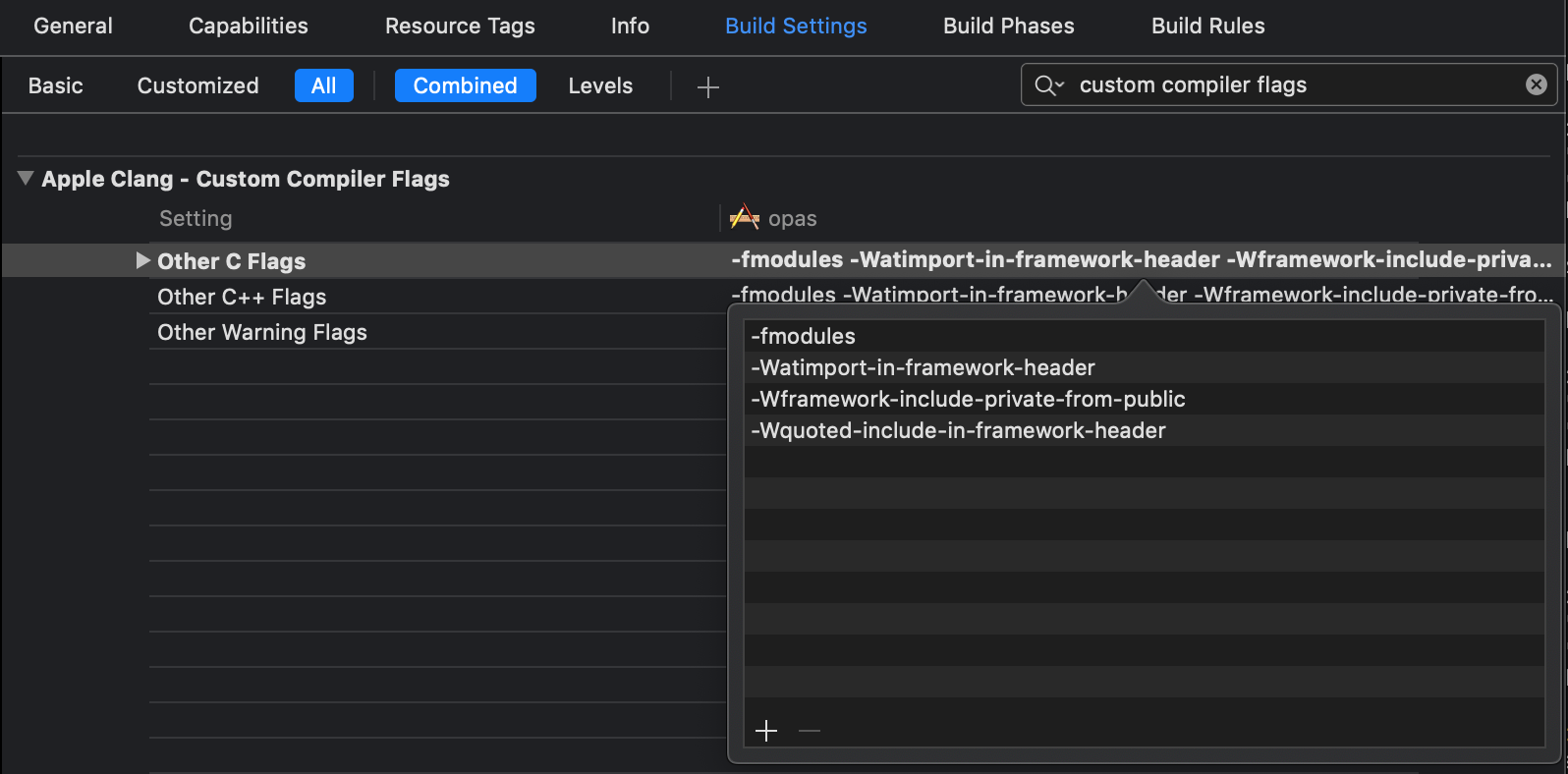
There were also problems with imported quotes inside the framework:
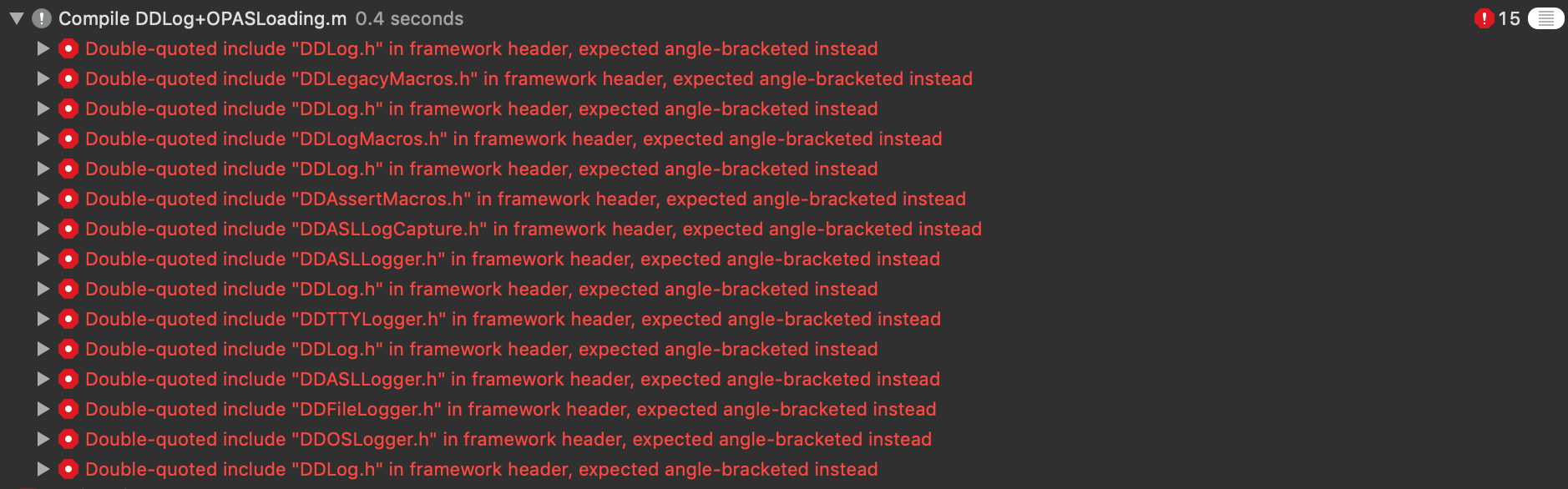
I advise you to make a similar diagnosis, or at least make a note for the future. Once these problems can cause a lot of trouble.
Assembly system
There is also a nice update in the code assembly system.
The search for implicit dependencies now works in the “other linker flags” section for related frameworks and libraries marked -framework, -weak_framework, -reexport_framework, -lazy_framework, -weak-l, -reexport-l, -lazy-l или —l.
This innovation is also very intriguing. In essence, it means that you can now define implicit dependencies using the option
.xcconfig
or even xcodebuild
by avoiding the Link / Embed stages in Xcode itself.Debugging
Debugger innovations:
Properties are UIStackView
now shown in the visual debugger inspector. The visual debugger has a more compact three-dimensional view.
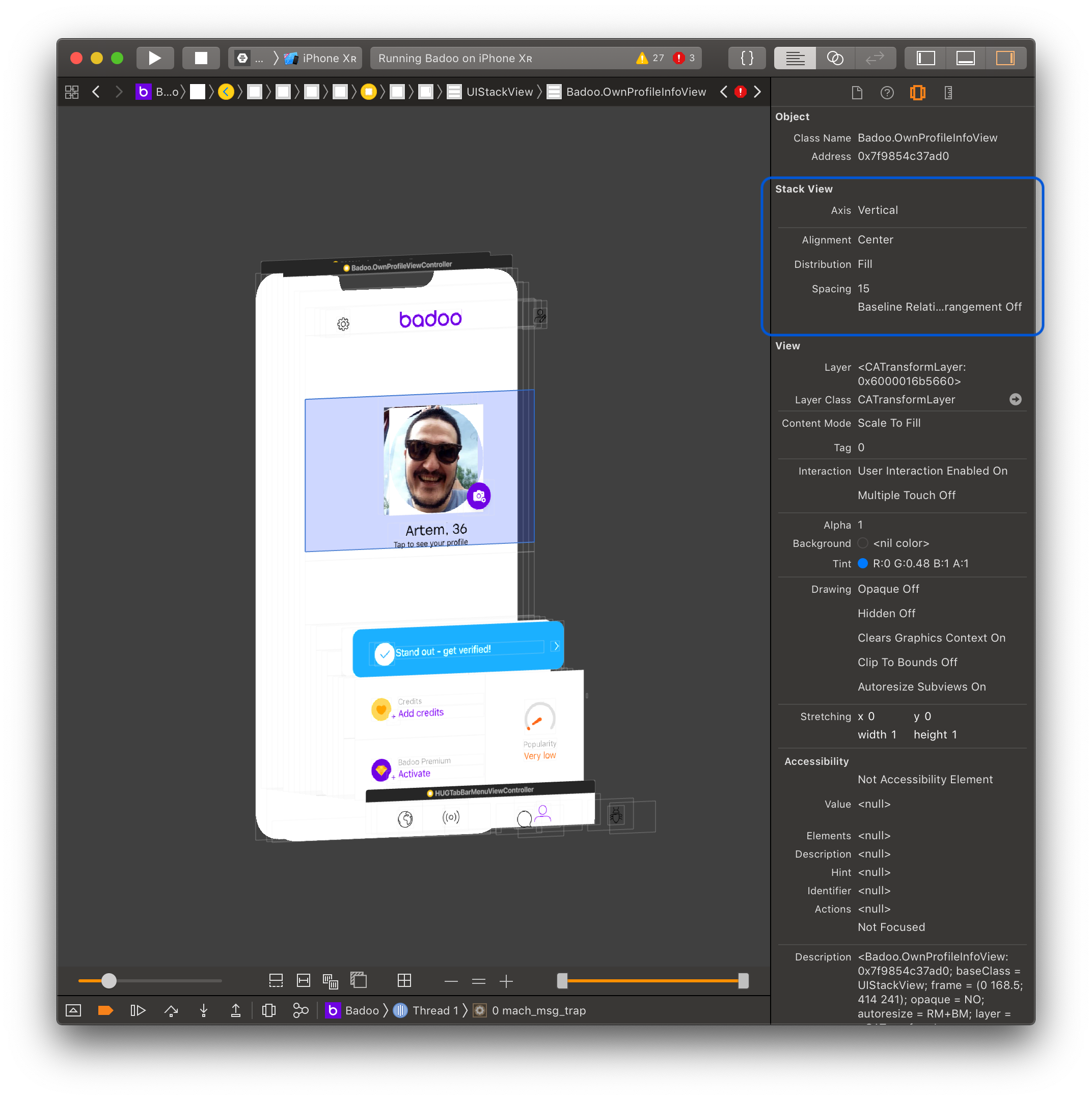
Now, if an error occurs due to insufficient memory, Xcode can automatically create a graph of memory usage. You can enable the creation of graphs in the diagnostics window in the schematic execution settings.
When approaching the limit of memory usage in iOS and watchOS Xcode displays the limit in the memory usage report.
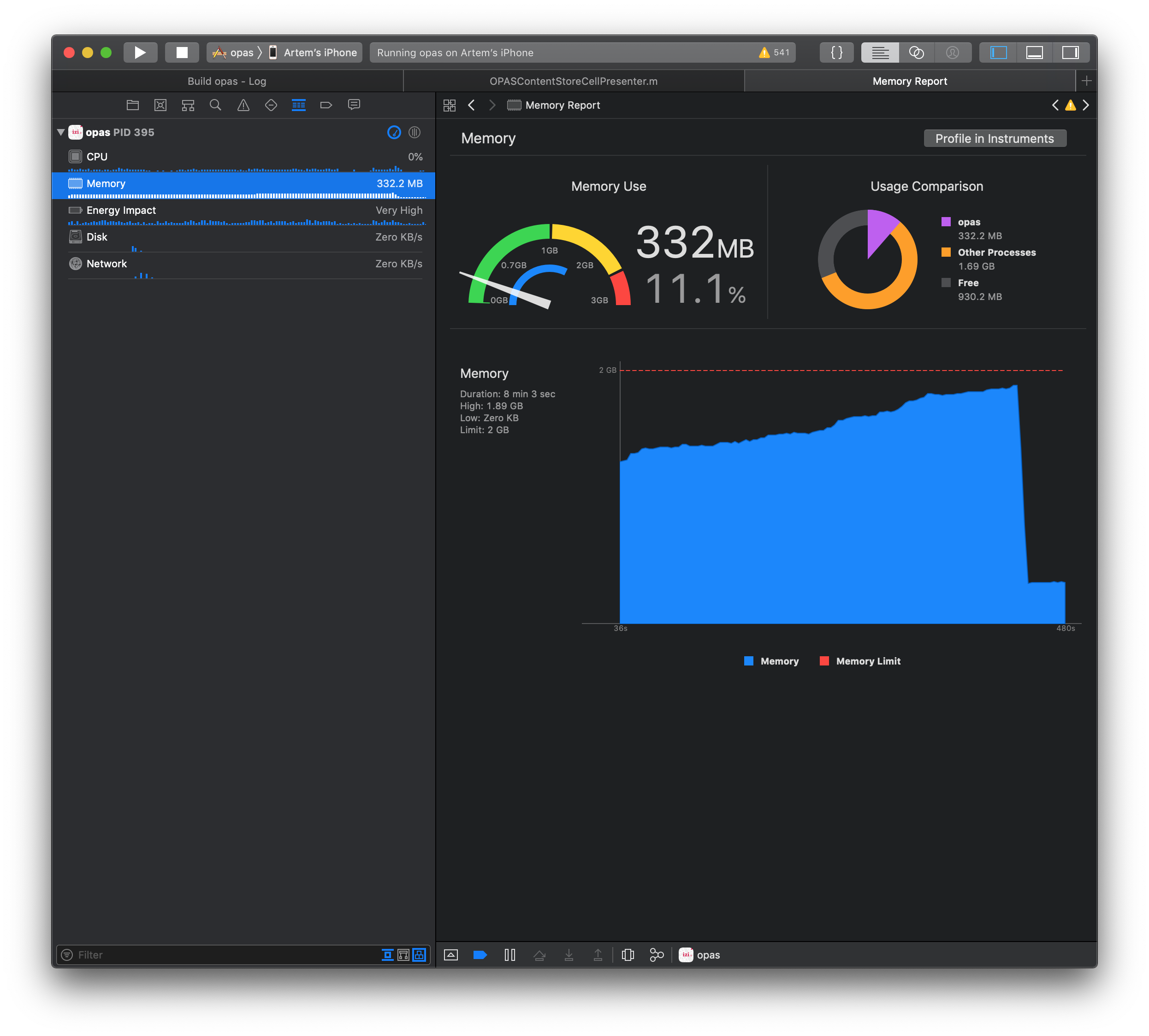
See the red line? When it reaches, Watchdog will send a notification
applicationDidReceiveMemoryWarning
. I thought that the update would be more useful, but for now it's just a little plus.LLDB debugger
New buns appeared in LLDB:
- Now you can use $ 0, $ 1, and other abbreviations when calculating expressions inside closures.
- LLDB now has a new alias
v
for the frame variable command, which is used to output variables in the current frame stack. Since this does not use the expression evaluation tool, itv
may be much faster thanp
orpo
, and therefore preferable.
I did not find an increase in performance, but in some cases
v
provides the best output — but it is not a direct replacement po
and works only with the current frame stack with certain limitations. Examples: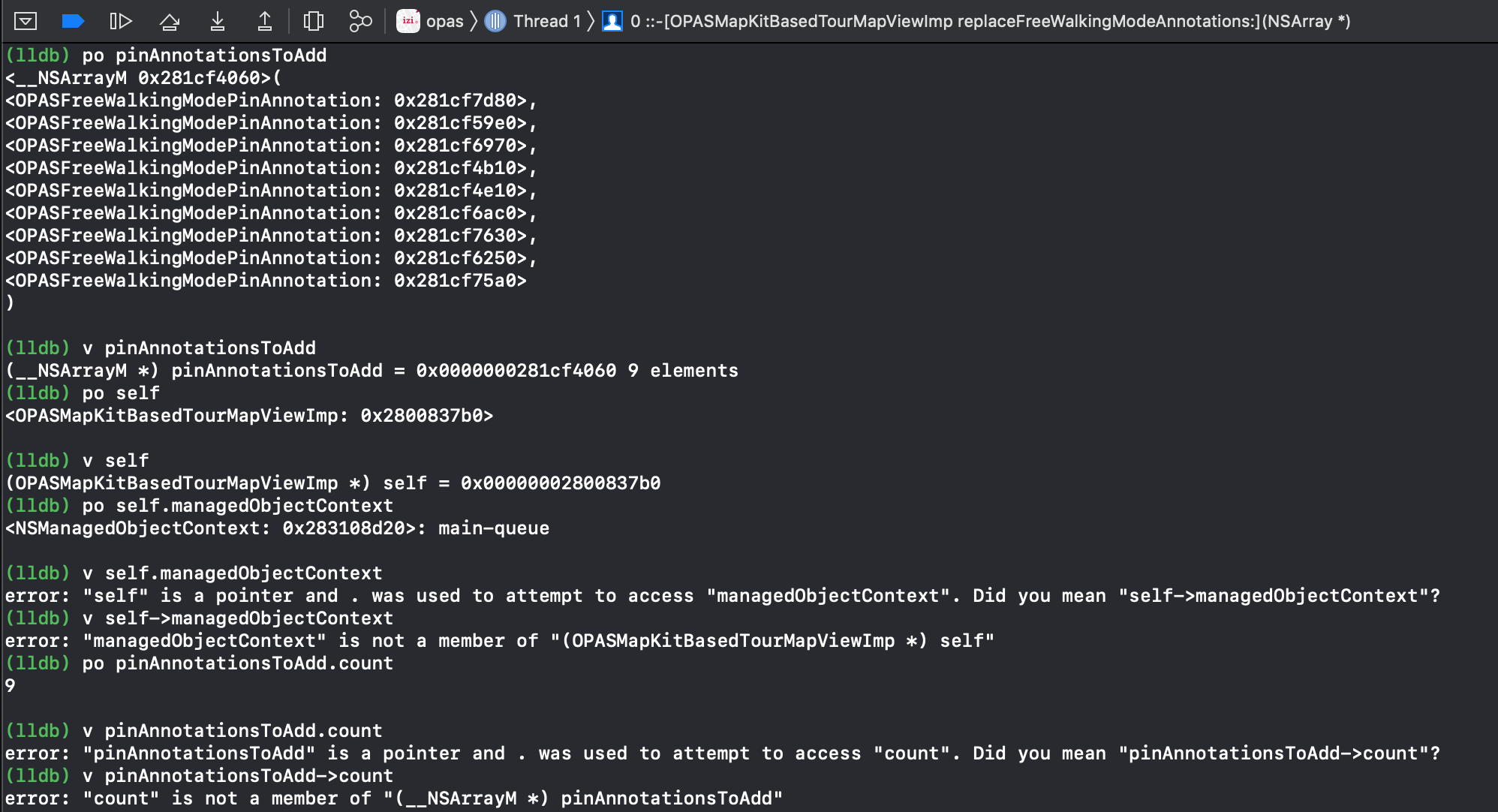
Playgrounds
My favorite section! Let's start with the bugs:
Playgrounds may not start
Unfortunately, there was no other news about the Playgrounds in the new beta.
Simulator
A few words about the simulator:
Siri does not work in watchOS and iOS simulators.
Pasteboard synchronization between macOS and simulated iOS devices has become more reliable.
I hope this is true.
The request to access the microphone on all simulated devices will not be repeated.
This is good news because, due to this problem, many people had difficulty with CI and build agents. Now you can build a crutch, or at least supplement your guides on working with assembly agents, including the “Run the simulator once” option.
Testing
xccov
supports merging multiple code coverage reports (and associated archives) into one general report and archive. The resulting report may contain inaccuracies, since there may be changes in the source files between the time the reports are created. If there are no changes, the report will be correct.- Now
xccov
reflects the difference between Xcode's different code coverage reports, which can be used to calculate the changes that have occurred in the coverage. For example, to highlight the difference betweenbefore.xccovreport
andafter.xccovreport
, call commandxccov
follows:xccov diff --json before.xccovreport after.xccovreport
.- Static libraries and framework targates now appear in the coverage report as top-level records — coverage metrics are collected across all targets that include a static library or framework. It also solves the problem when the source files of the static library or the framework framework are included in the report, even if the target itself is removed from the code coverage in the schema.
Great news for continuous integration, especially the demonstration of difference. Tell your release engineers or those who deal with such things.
However, there are several limitations regarding the parallelization of tests:
- writing on clones does not work when parallelization is enabled;
- project profiling from Xcode behaves incorrectly with the parallelization of tests enabled.
The rest is pleased with the correction of some bugs:
If testing fails due to a crash test runner on startup, Xcode will try to generate a detailed message describing the error. This error can be studied in the test log, and if you use itxcodebuild
, it will appear instdout
. It is also contained in the structured logs of the resulting package.
We often have similar problems - and we are wondering what happened. Sometimes it's in the wrong layout, sometimes in system overload. Now the reliability of the application will increase.
In the departure reports collected during testing, important sections such as the reason for departure or description are no longer lost.
Here, without comment, continuous delight.
The latest news, useful to many developers: now Xcode supports macOS content caching service . This means that you can create a caching server on the local network, which saves time and money when downloading new and old versions of Xcode on the local network.
Problems
In this beta, I encountered several problems. Basically, they dealt with tools from third-party developers. For example, it
carthage
gives the error "No simulators found for iOS were found." I checked the available simulator, and it seems to be the fault of the beta. In addition, download of other runtimes from Xcode is not available - the list of available simulators is empty:
$ xcrun simctl list devices --json | grep -A16 12.1
"com.apple.CoreSimulator.SimRuntime.iOS-12-1" : [
{
"availability" : "(unavailable, runtime profile not found)",
"state" : "Shutdown",
"isAvailable" : false,
"name" : "iPhone 5s",
"udid" : "DDD36346-A76F-42E8-80F4-6F11E1EE4BEB",
"availabilityError" : "runtime profile not found"
},
{
"availability" : "(unavailable, runtime profile not found)",
"state" : "Shutdown",
"isAvailable" : false,
"name" : "iPhone 6",
"udid" : "21794717-BC89-45E4-9F57-8CF9D14A87D1",
"availabilityError" : "runtime profile not found"
},
--
But this is just a beta, and the list of changes is huge. Stock up on patience. Carthage is already studying the situation, all the guesses are set out in this bug .
iOS 12.2 beta
Well well. Apple seems to be busy polishing technical debt and imposing security patches, so no serious news, but there are two problems:
- chances are that you will not be able to authenticate to the Wallet after choosing a card;
- chances are that you will not be able to purchase a prepaid plan using a mobile network.
And Apple News is now available in Canada.
Do not switch.
macOS Mojave 10.14.4 beta
The only update here is a possible issue with Safari 12.1 after upgrading from Safari 10.1.2.
After upgrading to Safari 12.1 from Safari 10.1.2, web pages may not display correctly. (47335741)
Workaround: enter a command in the terminal
defaults delete com.apple.Safari
. Please note that you lose all past Safari settings.Finally
The article came out much more voluminous than I expected. All my findings you will find above. And if in short - Swift 5 has come!
Thanks for attention.