
Android Development: Add Text to ProgressBar
The standard ProgressBar , as you know, is an indicator of the performance of lengthy processes. However, it is often used to visualize numerical values. Unfortunately, this element does not support the setText () method to place an inscription on it explaining the displayed value, which would significantly save the screen space. Remember the address bar in a standard browser, where a green bar is displayed behind the URI, showing the progress of the page loading. How to implement this behavior?
Under the cut - one of the options for solving this problem.
So, the statement of the problem: to implement an element that would perform the functions of a ProgressBar with a horizontal style (style = "@ android: style / Widget.ProgressBar.Horizontal"), and also have the ability to place text on top of itself.
Short action plan:
We will need a two-layer Drawable, which will be responsible for the graphic content of the element.
In the res / drawable folder, create a background.xml file with the following contents:
As you can see from the code, the background consists of two layers: the first is responsible for the container of the scale being created, and the second is actually for the scale that will characterize the displayed value. Both are rounded rectangles filled with a gray and yellow gradient. However, the second shape is enclosed in the clip tag, which means that only part of it will be displayed.
The trick of the described method is that we will not extend the ProgressBar class, but the TextView class. The key point is to create a method that draws a new background and sets a new label.
The setValue () method sets the label text of an element using the standard setText () function, and then changes the background image to the new value. To do this, use the setLevel () method, which is passed a parameter from 0 (the scale is not visible) to 10000 (the scale closes 100% of the container) as a parameter.
For convenience, the setMaxValue () method has also been created in the class, allowing you to set a value that corresponds to the full scale. Thus, it is possible to determine the range of possible values displayed by the element.
In the res / layout folder, modify the contents of the main.xml file, which is responsible for the appearance of the main Activity.
Here we indicate that the background of the element will be the Drawable we created with the name background (described in background.xml), well, do not forget to write the id by which it will be searched inside the Activity.
Do not forget to specify the namespace in which the AdvancedTextView class was created. In this example, it is ru.habrahabr.widgets.
The implementation and use of the element in the program is simple and straightforward:
The result will be such an element:
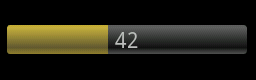
So, using our own Drawable for drawing the background and a class that extends TextView, we got the opportunity to use the progress bar with an explanatory inscription in the foreground.
Under the cut - one of the options for solving this problem.
So, the statement of the problem: to implement an element that would perform the functions of a ProgressBar with a horizontal style (style = "@ android: style / Widget.ProgressBar.Horizontal"), and also have the ability to place text on top of itself.
Short action plan:
- Create a Drawable responsible for drawing the background.
- Create a class that extends TextView, and write the necessary methods.
- Embed the created item in the Layout.
- Use an element in an Activity.
Create Drawable
We will need a two-layer Drawable, which will be responsible for the graphic content of the element.
In the res / drawable folder, create a background.xml file with the following contents:
As you can see from the code, the background consists of two layers: the first is responsible for the container of the scale being created, and the second is actually for the scale that will characterize the displayed value. Both are rounded rectangles filled with a gray and yellow gradient. However, the second shape is enclosed in the clip tag, which means that only part of it will be displayed.
Class creation
The trick of the described method is that we will not extend the ProgressBar class, but the TextView class. The key point is to create a method that draws a new background and sets a new label.
public class AdvancedTextView extends TextView {
// Максимальное значение шкалы
private int mMaxValue = 100;
// Конструкторы
public AdvancedTextView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
}
public AdvancedTextView(Context context, AttributeSet attrs) {
super(context, attrs);
}
public AdvancedTextView(Context context) {
super(context);
}
// Установка максимального значения
public void setMaxValue(int maxValue){
mMaxValue = maxValue;
}
// Установка значения
public synchronized void setValue(int value) {
// Установка новой надписи
this.setText(String.valueOf(value));
// Drawable, отвечающий за фон
LayerDrawable background = (LayerDrawable) this.getBackground();
// Достаём Clip, отвечающий за шкалу, по индексу 1
ClipDrawable barValue = (ClipDrawable) background.getDrawable(1);
// Устанавливаем уровень шкалы
int newClipLevel = (int) (value * 10000 / mMaxValue);
barValue.setLevel(newClipLevel);
// Уведомляем об изменении Drawable
drawableStateChanged();
}
}
The setValue () method sets the label text of an element using the standard setText () function, and then changes the background image to the new value. To do this, use the setLevel () method, which is passed a parameter from 0 (the scale is not visible) to 10000 (the scale closes 100% of the container) as a parameter.
For convenience, the setMaxValue () method has also been created in the class, allowing you to set a value that corresponds to the full scale. Thus, it is possible to determine the range of possible values displayed by the element.
Editing Layout
In the res / layout folder, modify the contents of the main.xml file, which is responsible for the appearance of the main Activity.
Here we indicate that the background of the element will be the Drawable we created with the name background (described in background.xml), well, do not forget to write the id by which it will be searched inside the Activity.
Do not forget to specify the namespace in which the AdvancedTextView class was created. In this example, it is ru.habrahabr.widgets.
Using an item in an Activity
The implementation and use of the element in the program is simple and straightforward:
AdvancedTextView advancedTextView = (AdvancedTextView) findViewById(R.id.advanced_text_view);
advancedTextView.setValue(42);
The result will be such an element:
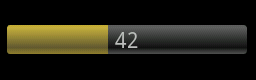
Conclusion
So, using our own Drawable for drawing the background and a class that extends TextView, we got the opportunity to use the progress bar with an explanatory inscription in the foreground.