
Maps in your Android app
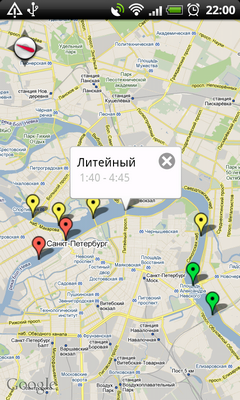
Quite often, the developer of a mobile application needs to display something on a map. What options does the developer have?
I considered two options.
Option 1. External application with a map
Perhaps the easiest option to implement. When necessary, you simply open a third-party application with a map - create a uri of the form geo: latitude, longitude , in the z parameter you can specify the scale (from 1 to 23):
String uri = String.format("geo:%s,%s?z=16", Double.toString(lat), Double.toString(lng));
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(uri));
startActivity(intent);
At the same time, if several applications with maps are installed on the user's device, the user will be asked to choose in which application the specified point should be shown:
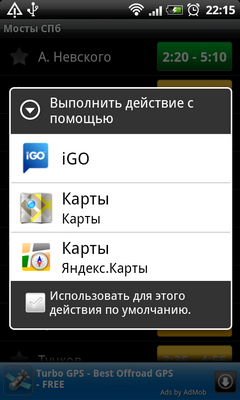
Yes, yes. In this way, it is the given point that is shown, and not just the map (although Yandex.Maps show just a map and, judging by my correspondence with them, they believe that it is necessary).
The advantages of this option:
- ease of implementation;
- all the features of an external application (for example, in iGo you can immediately get directions to the shown point; maps in some applications will be available offline).
- there is no way to display additional information (as, for example, in the first screenshot in this article - labels, tooltips, etc.).
Option 2. Built-in card
The integrated card is more difficult to implement. I will not dwell on all the details, the main thing is well described in the documentation .
I will dwell only on some subtleties.
Maps API Key
For the built-in card to work, you need to get the keys. Keys, not a key, because for the debug version and for the release version of your application, the keys will be different.
In order not to change the keys manually each time, you can create two layout'a with a map and write something like this in the code:
// choose layout with correct API key
if (debug) {
setContentView(R.layout.map_debug);
} else {
setContentView(R.layout.map_release);
}
Other options (including for determining the debug / release version of the assembly) can be found here .
current position
To display the current position on the map, you must write the following:
private MyLocationOverlay myLocationOverlay;
MapView mapView = (MapView) findViewById(R.id.mapview);
myLocationOverlay = new MyLocationOverlay(this, mapView);
myLocationOverlay.enableMyLocation();
mapView.getOverlays().add(myLocationOverlay);
Remember to turn off location when stopping / closing activity:
myLocationOverlay.disableMyLocation();
Markers
Markers are simply added to the map:
List mapOverlays;
Drawable drawable;
MapOverlay itemizedOverlay;
mapOverlays = mapView.getOverlays();
drawable = this.getResources().getDrawable(R.drawable.map_dot_green);
itemizedOverlay = new MapOverlay(drawable, mapView);
GeoPoint markerPoint = new GeoPoint(lat, lng);
OverlayItem overlayItem = new OverlayItem(markerPoint, "name", "description");
itemizedOverlay.addOverlay(overlayItem);
mapOverlays.add(itemizedOverlay);
Moreover, if you add several markers, then all the markers on the same layer will be the same. How to make markers different (as in the screenshot above - yellow, red, green)? Either create multiple layers, or use the SetMarker method:
int w = drawable.getIntrinsicWidth();
int h = drawable.getIntrinsicHeight();
drawable.setBounds(-w / 2, -h, w / 2, 0);
overlayItem.setMarker(drawable);
Without the first three lines, a void will be displayed instead of a marker.
If you do not use negative values of w and h and do not divide them in half, then the markers will have an incorrect shadow.
Advantages and disadvantages of this option
Benefits:
- the ability to create your own layers and labels;
- the ability to leave the user in his application (adding, for example, the window title).
- relative complexity of implementation;
- not all features of third-party applications;
- activity with a map has been running for a long time.
Conclusion
As a result, I could not make a choice for the user. Therefore, in my application both options are supported - in the settings the user can specify whether he wants to use external applications (by default, the built-in card is used).