
Parsing XML with the Simple Framework
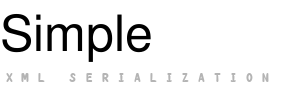
Hello readers Habrahabr!
This post is inspired by another post and the comment of the respected AnatolyB habrayuzer from there.
I think that many are familiar with this library, but, nevertheless, it turned out that it has not yet been reflected in the pages of Habra. We will deal with this misunderstanding today.
And, of course, I recommend to those who are not familiar with this beautiful library as soon as possible, I will try to help you with this.
Intro
Simple Framework is a library compatible with the Android virtual machine designed to serialize / deserialize Java objects in xml and vice versa.
The main advantage of Simple is its declarative approach to describing the relationships between classes with their contents and XML representation. Those. just set the appropriate attributes to the fields of the class and you can immediately serialize them in XML, and if desired, and vice versa. You don’t need to do any additional garbage, such as listing nodes, everything is very simple and transparent. All the work of comparing class fields, listing them, getting values, etc. takes on Simple, and helps her with this reflection.
On the official websiteThere is everything you need to start working with the library, as well as my favorite type of documentation: documentation with a large set of versatile examples .
First of all, download the library from here . We find the jar file and copy it to the lib folder inside our project. Next, add our jar to the Build Path via “Add JARs ...”, and end up with something like this:
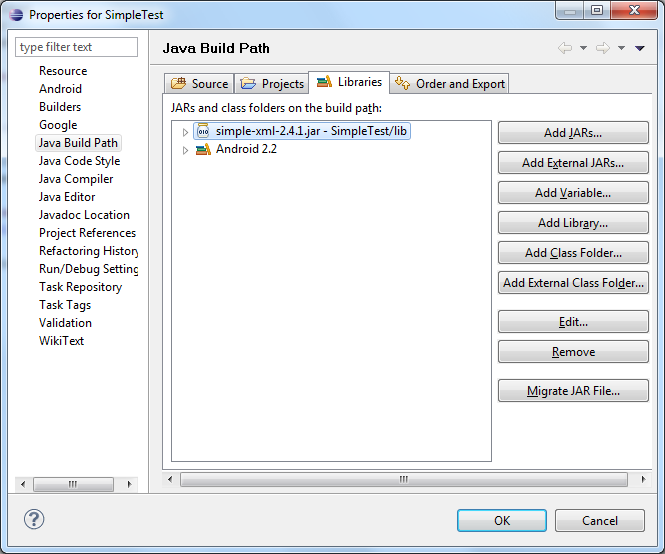
Examples of using
Now we can use Simple in our project. And the first thing we try to do is convert simple XML to a Java class.
Suppose we have the following XML:
Bobby 8 Lucky
It represents the essence of "pet", which has the required fields: name and age, as well as one optional: nickname.
In order to associate this XML with some class, we need to create a class and add special attributes to it so that Simple can understand what and how we want to do it. For our example, the class will look like this:
@Root(name="Pet")
public class MyPet
{
@Element(name="Name")
public String name;
@Element(name="Age")
public int age;
@Element(required=false, name="NickName")
public String nickName;
}
Where:
- Root - points to the root XML element.
- Element - Pet internal fields.
- required - Says this field may be optional.
- name - indicates the name of the element in the input XML, if this name and the class field are the same, then this attribute can be omitted.
And our class would look like this:
@Root(name="Pet")
public class MyPet
{
@Attribute(name="Name")
public String name;
@Attribute(name="Age")
public int age;
@Attribute(required=false, name="NickName")
public String nickName;
}
The deserialization code itself looks like this:
Reader reader = new StringReader(xml);
Persister serializer = new Persister();
try
{
MyPet pet = serializer.read(MyPet.class, reader, false);
Log.v("SimpleTest", "Pet Name" + pet.name);
}
catch (Exception e)
{
Log.e("SimpleTest", e.getMessage());
}
In the above code, we gave two parameters to the Simple serializer input: MyPet.class - a pointer to a class with a description of the attributes for deserialization and reader - a stream containing input XML. As you can see the code is not at all complicated and very compact.
The code for the inverse transform is also quite simple:
Writer writer = new StringWriter();
Serializer serializer = new Persister();
try
{
MyPet pet = new MyPet();
pet.name = "Bobby";
pet.age = 8;
pet.nickName = "Lucky";
serializer.write(pet, writer);
String xml = writer.toString();
}
catch (Exception e)
{
Log.e("SimpleTest", e.getMessage());
}
To comply with the principle of encapsulation, the fields of the class can be wrapped in get'ers and set'ers and Simple will work with them:
@Root(name="Pet")
public class MyPet
{
private String name;
private int age;
private String nickName;
@Attribute(name="Name")
public void setName(String name)
{
this.name = name;
}
@Attribute(name="Name")
public String getName()
{
return name;
}
@Attribute(name="Age")
public void setAge(int age)
{
this.age = age;
}
@Attribute(name="Age")
public int getAge()
{
return age;
}
@Attribute(required=false, name="NickName")
public void setNickName(String nickName)
{
this.nickName = nickName;
}
@Attribute(required=false, name="NickName")
public String getNickName()
{
return nickName;
}
}
If an XML element has its own attributes or nested elements, then it can be declared a separate class or a list of them. We modify our example, add the entity "nursery", which can contain an arbitrary number of objects "pet" (Pet). Example:
For this example, the only thing we need is to add a class for "Nursery":
@Root(name="Nursery")
public class MyNursery
{
@ElementList(inline=true, name="Pet")
public List pets;
}
As you can see, everything is just as simple. The inline keyword indicates that the "Pet" elements are contained immediately inside MyNursery, without using an intermediate parent element.
The code for downloading Nursery is similar to what we did for Pet:
Reader reader = new StringReader(xml);
Persister serializer = new Persister();
try
{
MyNursery nursery = serializer.read(MyNursery.class, reader, false);
Log.v("SimpleTest", "Pets in nursery: " + nursery.pets.size());
}
catch (Exception e)
{
Log.e("SimpleTest", e.getMessage());
}
I think these examples are enough to start an independent investigation with the Simple library. Moreover, a large number of examples are presented on the official website.
Conclusion
Simple offers solutions, probably for all constructions that are only possible in XML. Java features such as inheritance and interfaces are also supported.
When using Simple, the following positive and negative aspects can be noted.
Positive:
- Easy to use and understand.
- The amount of code with this approach is minimal.
- Android platform support.
- Rich support for various XML constructs.
- The ability to apply in non-Android applications, for example, in unit tests that are untied from devices.
- Apache license i.e. can be freely used in commercial software.
Negative:
- Simple attribute syntax overloads class representation.
- For the operation of Simple, the Reflection mechanism is used, and these are costly operations. Therefore, if you intend to use this Framework in a product demanding on performance, then it is worth considering the feasibility of such a solution.