Overview of ASP.NET Profilers
Almost a year ago, an article was published about using the application profiler in Visual Studio 2010. In the comments, it was suggested that it would be nice to compare this product with similar ones. I'll try to conduct a brief review and comparison of the 4 most famous .NET profilers.
The review involved:
What do we expect from a profiler? We need absolute (time) and relative (percent) statistics on the execution of individual sections of the code (if possible, accurate to the line). After optimizing some critical sections of the code, you need to start profiling again and compare the results.
Since by the nature of my activity I am associated with web development, the ASP.NET application will act as a test subject ( download ):
A slight lyrical digression. At first I wanted to choose a real application for profiling. The choice fell on Tailspin Spyworks , which is used as a steb-by-step guide. It would seem that the manual for beginners should be so polished in order to immediately interest the developer, teach him the right things. And what did I see there? A crooked layout, a mixture of business logic and markup, suboptimal queries to the database, select * even if 1-2 fields are stretched ... Performing all optimizations 4 times (for 4 profilers) was very time-consuming, so the test used in 3 minutes was written attachment. If anyone is interested, in future articles it will be possible to make out the bones of Tailspin Spyworks.
Enough lyrics, run profilers.
Launching is done from VS via the menu Analyze -> Launch Performance Wizard.
With a few simple steps, we select the type of profiling (I chose Instrumentation, because CPU sampling does not show the execution time, only in percent), the projects under study and whether it is necessary to profile database queries through ADO.NET.
After clicking the Finish button, the browser launches, while in the studio a splash screen with the Pause and Stop buttons will hang. After the page has finished loading in the browser, click Stop and get the result: We will not dwell on the profiler description, it’s done quite well here , here and here . Here is the statistics screen by method:

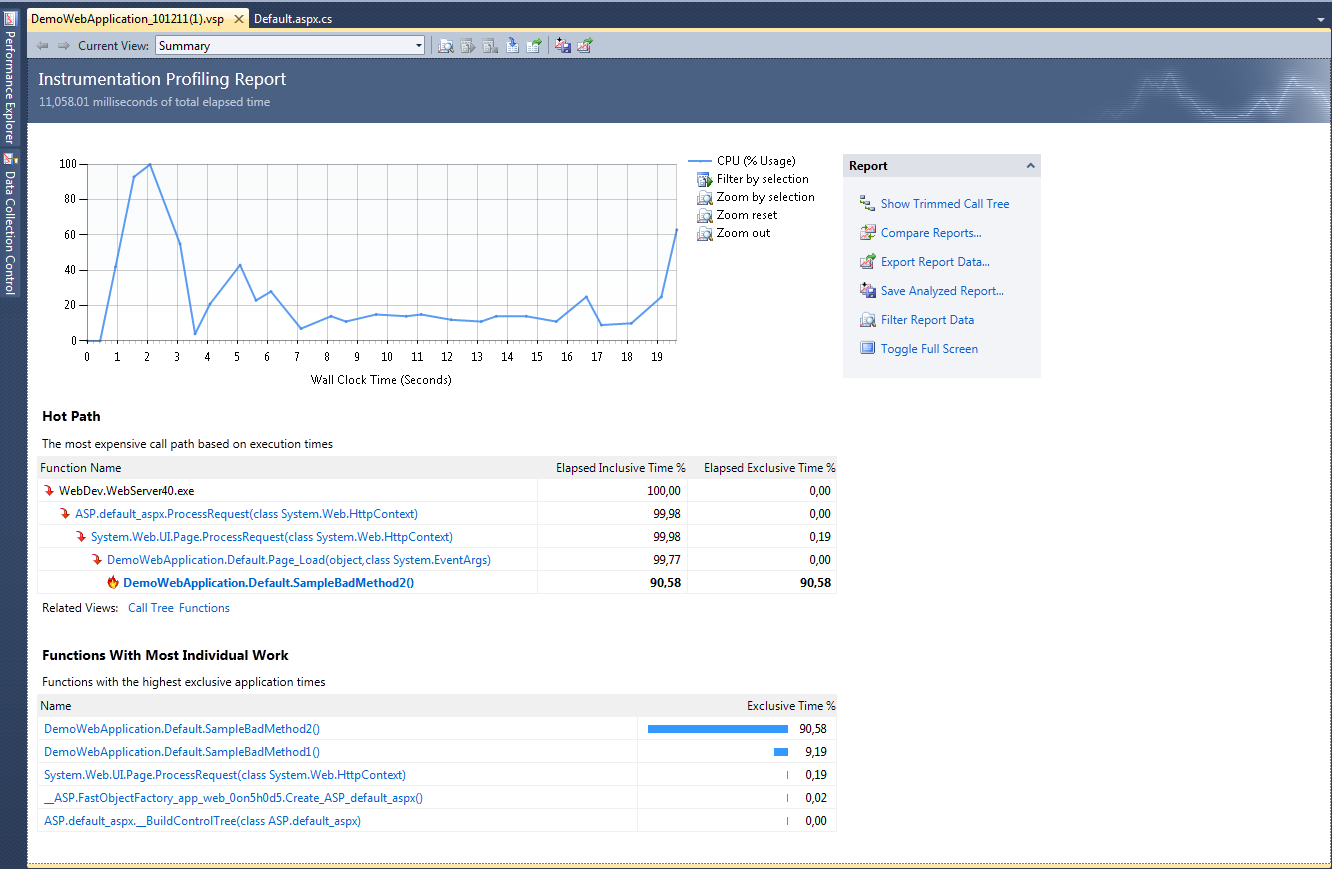

Now we need to optimize the critical sections of the code SampleBadMethod1 and SampleBadMethod2. As an “optimization”, we reduce the number of iterations in the loop (for example, from 100 to 50 and from 10000 to 1000).
We get the result again and through the menu item Analyze-> Compare Perfomance Reports we compare the result: Well, well done, we managed to speed up our application. Repeat the same steps with other profilers.

When creating a new profiling session, the following window appears: Here you can select the application type, profiling options and enter application data, for example, for an ASP.NET application on a dev server this is the path to the application, version .net, port number, etc. After clicking the Start Profiling button, the browser starts, in the ANTS Profiler at this time, a graph of the processor load along the time axis is drawn. Click the Stop Profiling button and get the result:


I won’t discuss the purpose and functions of the screen areas in detail (this is the topic of a separate article), I’ll briefly say that in the upper part you can see the timeline with CPU loading (or any other indicator that you can choose yourself), in the center there is a tree of methods with execution statistics by time and number of calls; at the bottom, see the source code of the methods (if the code is available).
There is some oddity here: we see that the total execution time of the SampleBadSubMethod2 method is 14 ms, although there is a 1 ms delay in it and it is called 10,000 times. Perhaps ANTS somehow incorrectly processes the Thread.Sleep method.
Now we “optimize” the application again, launch the profiler, get the result and ... we can’t compare it using ANTS ... The FAQ on the site suggests launching another profiler and switching between them, comparing the result. Well, thanks, what else to say :)
Joins the battle
When choosing File-> Profile, a window appears: Select the type of application, set the settings and Run! After starting profiling, a small window appears with which you can get the result (Get Snapshot) or complete the profiling. After receiving the result, we will see the following window: Here we see a tree of methods with percentages and runtimes. On the right side you can see the source of a particular method. We optimize the application, get the result and compare it with the original one: Well, the optimization was a success. And finally
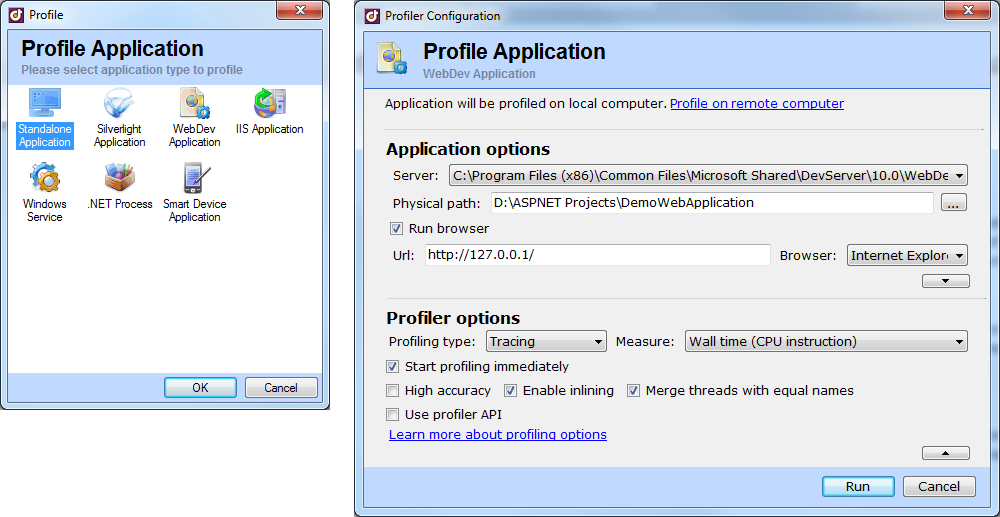



We start the profiler, we see the window: Here you need to select the path to the application bin folder, select the assemblies that we want to explore by clicking Build. Then launch your favorite browser and load the application page. If everything is fine, then the message “Profiled appication xxx started” appears in the log and the “Take snapshot” and “Reset counters” buttons become active. Next, click “Take snapshot”: And here is our snapshot: We see the call statistics, as well as representing the methods as blocks (in the lower part). These blocks are clickable, i.e. You can move up and down the call hierarchy. Now, once again, we optimize the application and compare the result: Well done again, we have accelerated the application many times.

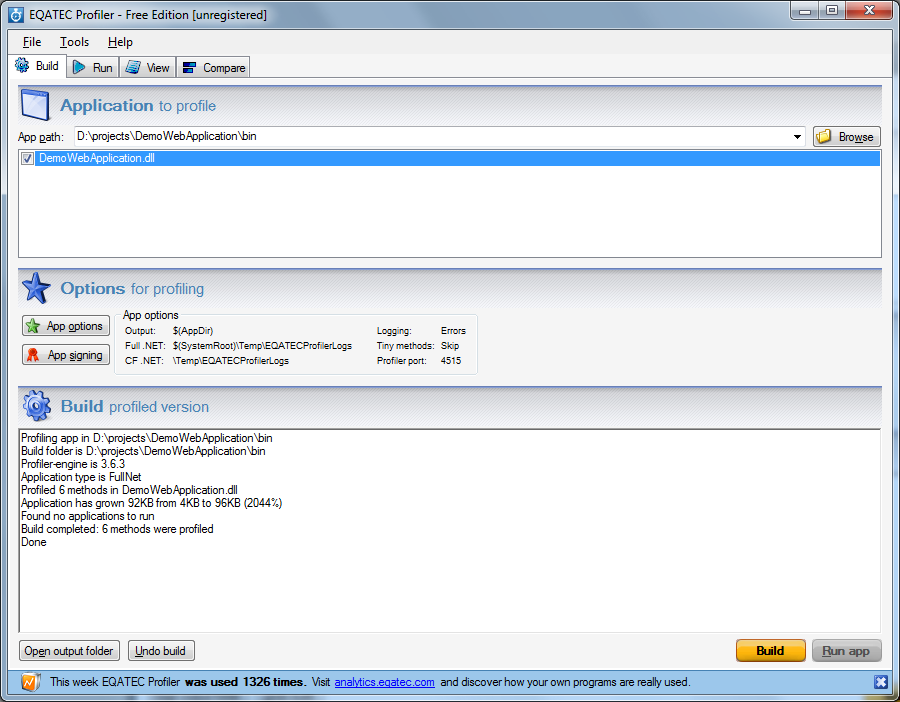




That's all, look at the summary table of the features of the presented profilers.
1) as part of VS Premium and above
2) depends on the edition
3) for open source projects free of charge
4) restriction on 10 simultaneously loaded dlls, for $ with less restrictions
Now we will consider the advantages and disadvantages of each profiler that I noticed separately, not listed in the comparative table.

Interaction with ADO.NET is built-in in VS (though in premium and ultimate)
, it’s capricious, sometimes profiling does not start without explaining the reasons
the appSettings section should be in web.config, and not put out in a separate file, because some service settings are written there, and the developers apparently did not foresee the location of this section in an external file
on a large project and a not very powerful machine noticeably slows down
the most detailed information on method calls, a bunch of performance counters
in SQL profile mode thinks that apart from ./SQLEXPRESS servers no longer exists :)
there is no comparison of the two measurement results
most of all I liked the documentation
in the tree view, some mess of numbers, method names, assemblies
did not start in IIS Application mode, although I did everything according to good documentation.
small and fast, suitable if you do not need to watch performance line by line and you do not need the source code;
this minus is a consequence of the plus: fewer opportunities than other competitors.
So, I will probably make a subjective conclusion. My choice is the EQATEC Profiler, for many performance assessment tasks it is more than enough. If you have the option of using VS Premium or Ultimate, the integrated profiler is a pretty good product. In this case, the need to purchase other profilers will disappear. Of the remaining two profilers, the ANTS profiler is striking in its power, although, of course, why there is no comparison of the results is unclear. DotTrace has an abundance of acquisition options with many features of the profiler itself.
Thanks for reading this review! Hope it helps you make a choice.
PS Proposals for a detailed review of each profiler are accepted.
The review involved:
- the profiler integrated in Visual Studio 2010 (starting with the Premium edition) ( MSDN , blog );
- ANTS Performance profiler from RedGate;
- dotTrace from JetBrains;
- EQATEC Profiler by EQATEC.
What do we expect from a profiler? We need absolute (time) and relative (percent) statistics on the execution of individual sections of the code (if possible, accurate to the line). After optimizing some critical sections of the code, you need to start profiling again and compare the results.
Since by the nature of my activity I am associated with web development, the ASP.NET application will act as a test subject ( download ):
- public partial class Default : System.Web.UI.Page
- {
- protected void Page_Load(object sender, EventArgs e)
- {
- SampleBadMethod1();
- SampleBadMethod2();
- }
-
- private void SampleBadMethod1()
- {
- for (int i = 0; i < 100; i++)
- {
- SampleBadSubMethod1();
- }
- }
-
- private void SampleBadSubMethod1()
- {
- Thread.Sleep(10);
- }
-
- private void SampleBadMethod2()
- {
- for (int i = 0; i < 10000; i++)
- {
- SampleBadSubMethod2();
- }
- }
-
- private void SampleBadSubMethod2()
- {
- Thread.Sleep(1);
- }
- }
* This source code was highlighted with Source Code Highlighter.
A slight lyrical digression. At first I wanted to choose a real application for profiling. The choice fell on Tailspin Spyworks , which is used as a steb-by-step guide. It would seem that the manual for beginners should be so polished in order to immediately interest the developer, teach him the right things. And what did I see there? A crooked layout, a mixture of business logic and markup, suboptimal queries to the database, select * even if 1-2 fields are stretched ... Performing all optimizations 4 times (for 4 profilers) was very time-consuming, so the test used in 3 minutes was written attachment. If anyone is interested, in future articles it will be possible to make out the bones of Tailspin Spyworks.
Enough lyrics, run profilers.
Visual Studio Performance Profiler
Launching is done from VS via the menu Analyze -> Launch Performance Wizard.
With a few simple steps, we select the type of profiling (I chose Instrumentation, because CPU sampling does not show the execution time, only in percent), the projects under study and whether it is necessary to profile database queries through ADO.NET.
After clicking the Finish button, the browser launches, while in the studio a splash screen with the Pause and Stop buttons will hang. After the page has finished loading in the browser, click Stop and get the result: We will not dwell on the profiler description, it’s done quite well here , here and here . Here is the statistics screen by method:

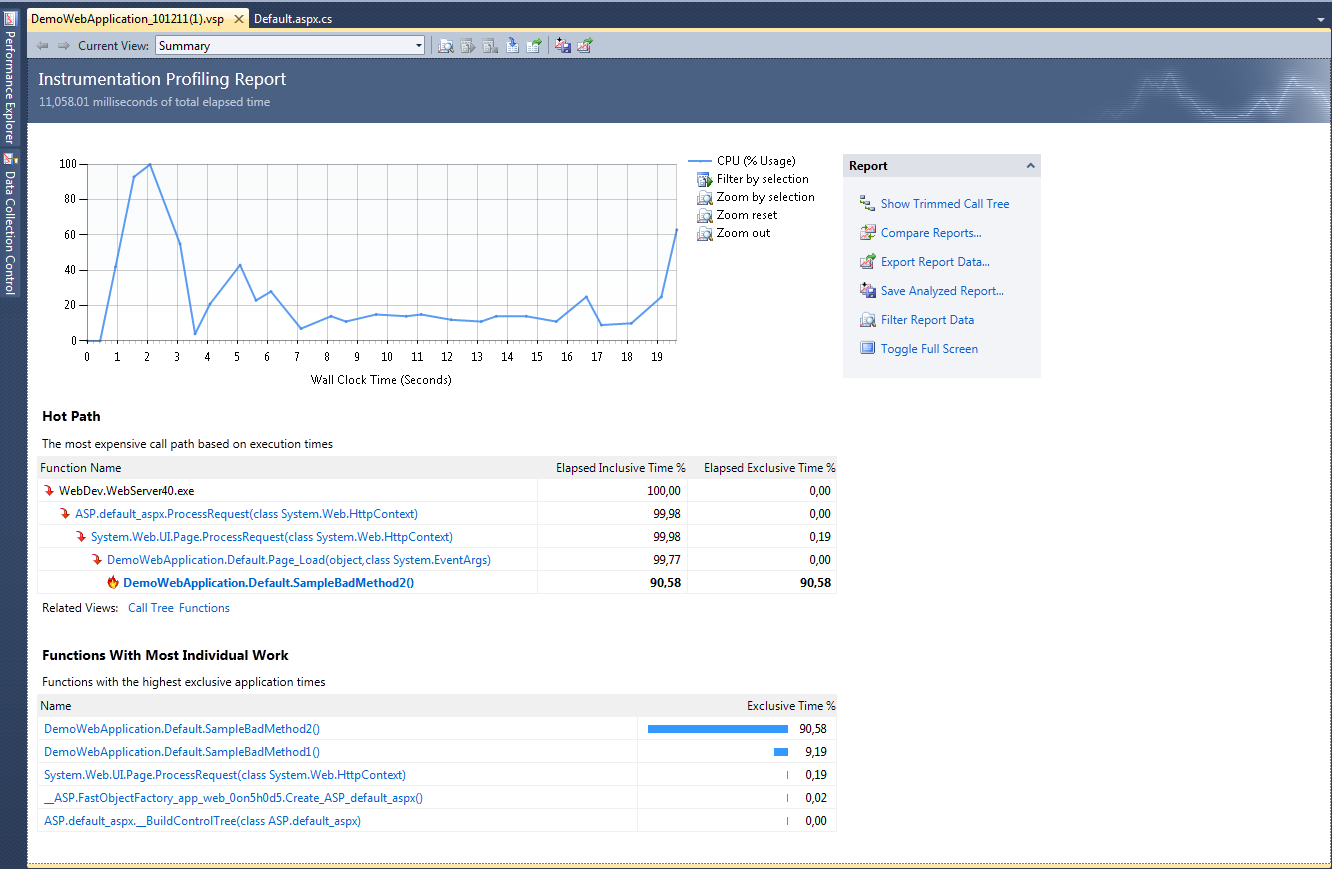

Now we need to optimize the critical sections of the code SampleBadMethod1 and SampleBadMethod2. As an “optimization”, we reduce the number of iterations in the loop (for example, from 100 to 50 and from 10000 to 1000).
We get the result again and through the menu item Analyze-> Compare Perfomance Reports we compare the result: Well, well done, we managed to speed up our application. Repeat the same steps with other profilers.

ANTS Profiler
When creating a new profiling session, the following window appears: Here you can select the application type, profiling options and enter application data, for example, for an ASP.NET application on a dev server this is the path to the application, version .net, port number, etc. After clicking the Start Profiling button, the browser starts, in the ANTS Profiler at this time, a graph of the processor load along the time axis is drawn. Click the Stop Profiling button and get the result:


I won’t discuss the purpose and functions of the screen areas in detail (this is the topic of a separate article), I’ll briefly say that in the upper part you can see the timeline with CPU loading (or any other indicator that you can choose yourself), in the center there is a tree of methods with execution statistics by time and number of calls; at the bottom, see the source code of the methods (if the code is available).
There is some oddity here: we see that the total execution time of the SampleBadSubMethod2 method is 14 ms, although there is a 1 ms delay in it and it is called 10,000 times. Perhaps ANTS somehow incorrectly processes the Thread.Sleep method.
Now we “optimize” the application again, launch the profiler, get the result and ... we can’t compare it using ANTS ... The FAQ on the site suggests launching another profiler and switching between them, comparing the result. Well, thanks, what else to say :)
Joins the battle
dotTrace
When choosing File-> Profile, a window appears: Select the type of application, set the settings and Run! After starting profiling, a small window appears with which you can get the result (Get Snapshot) or complete the profiling. After receiving the result, we will see the following window: Here we see a tree of methods with percentages and runtimes. On the right side you can see the source of a particular method. We optimize the application, get the result and compare it with the original one: Well, the optimization was a success. And finally
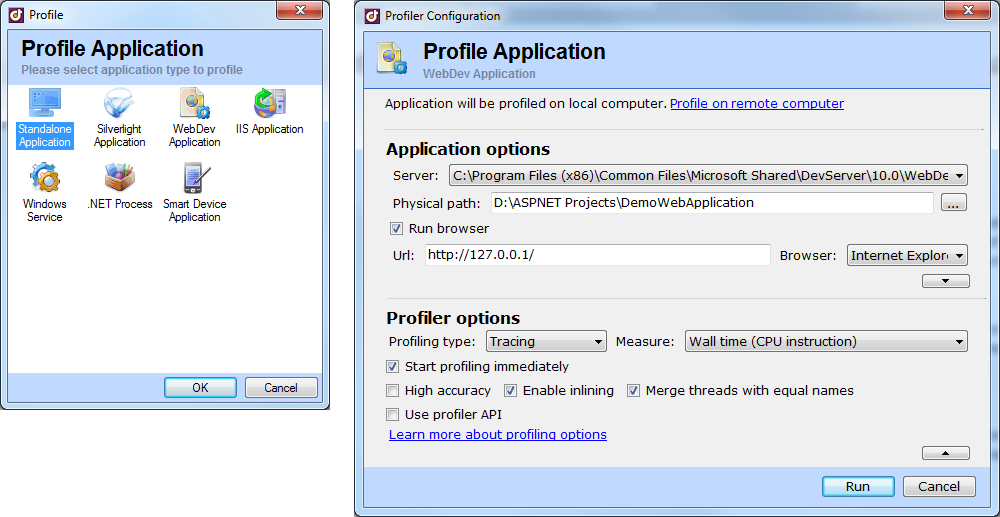



EQATEC Profiler
We start the profiler, we see the window: Here you need to select the path to the application bin folder, select the assemblies that we want to explore by clicking Build. Then launch your favorite browser and load the application page. If everything is fine, then the message “Profiled appication xxx started” appears in the log and the “Take snapshot” and “Reset counters” buttons become active. Next, click “Take snapshot”: And here is our snapshot: We see the call statistics, as well as representing the methods as blocks (in the lower part). These blocks are clickable, i.e. You can move up and down the call hierarchy. Now, once again, we optimize the application and compare the result: Well done again, we have accelerated the application many times.

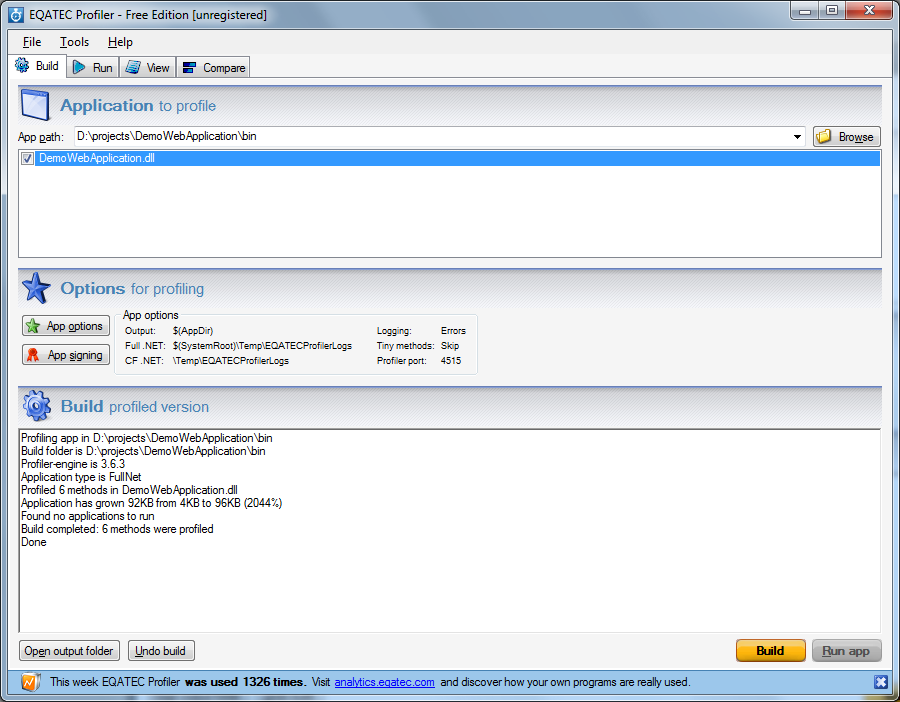




That's all, look at the summary table of the features of the presented profilers.
Summary
VS Profiler | ANTS | dotTrace | Eqatec | |
---|---|---|---|---|
Display of relative results of method execution (in%) | ![]() | ![]() | ![]() | ![]() |
Show absolute results of method execution (in seconds, ms, etc.) | ![]() | ![]() | ![]() | ![]() |
Display the number of method calls | ![]() | ![]() | ![]() | ![]() |
View method sources | ![]() | ![]() | ![]() | ![]() |
Comparison of the results of two measurements | ![]() | ![]() | ![]() | ![]() |
Price | > 5000 $ 1) | from 395 $ 2) | from 199 $ 3) | free 4) |
1) as part of VS Premium and above
2) depends on the edition
3) for open source projects free of charge
4) restriction on 10 simultaneously loaded dlls, for $ with less restrictions
Now we will consider the advantages and disadvantages of each profiler that I noticed separately, not listed in the comparative table.
VS Profiler





ANTS Profiler



dotTrace



Eqatec


So, I will probably make a subjective conclusion. My choice is the EQATEC Profiler, for many performance assessment tasks it is more than enough. If you have the option of using VS Premium or Ultimate, the integrated profiler is a pretty good product. In this case, the need to purchase other profilers will disappear. Of the remaining two profilers, the ANTS profiler is striking in its power, although, of course, why there is no comparison of the results is unclear. DotTrace has an abundance of acquisition options with many features of the profiler itself.
Thanks for reading this review! Hope it helps you make a choice.
PS Proposals for a detailed review of each profiler are accepted.